Introduction
“Picture processing” could appear new to you. Nonetheless, all of us do picture processing in our day by day life, like blurring, cropping, de-noising, and likewise including totally different filters to reinforce a picture earlier than importing it to social media. Typically it’s the app that you just use has that process automated (i.e., deep studying), so you should utilize the improved picture proper after the shot. Like, processing the uncooked medical picture can also be a really essential step in any automated medical diagnostics e.g. classification of various sorts of tumors, pneumonia from a chest x-ray, mind strokes from a CT-Scan, inner hemorrhage from MRI, and so forth.
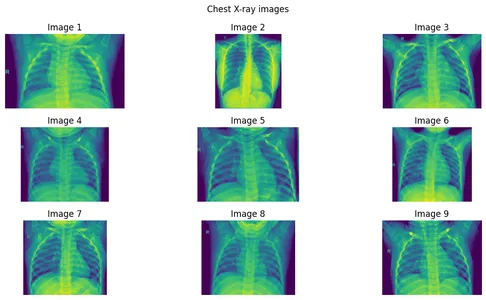
On this article, we’ll focus on several types of filters utilized in picture processing, particularly chest X-Ray, for extracting vital areas from the photographs.
Studying Aims
- Multidimensional picture processing approach.
- Utility of Laplace-Gaussian, Gaussian-Gradient-Magnitude edge detection operator on Chest X-ray photographs.
- The working of Sobel and Canny filter and its utility.
- Use of Numpy for masking a picture to separate vital segments.
- Use of Matplotlib library to plot a number of photographs collectively.
This text was printed as part of the Knowledge Science Blogathon.
Desk of Contents
Dataset
The dataset we use on this article is from Kaggle Pneumonia X-ray Picture.
Obtain Hyperlink
Chest X-Ray pneumonia picture.
Knowledge Description
- Whole variety of observations (photographs): 5,856
- Coaching observations: 4,192 (1,082 regular circumstances, 3,110 lung opacity circumstances)
- Validation observations: 1,040 (267 regular circumstances, 773 lung opacity circumstances)
- Testing observations: 624 (234 regular circumstances, 390 lung opacity circumstances)
- Setup Mission Atmosphere
We use anaconda for venture improvement. To create a brand new venture setting, observe the under instruction.
1. Open your terminal and kind these
$ conda create --name imgproc python=3.9
$ conda activate imgproc
2. Now, Set up the mandatory libraries
pip set up numpy matplotlib scipy imageio
Look at X-ray Photos
I downloaded and extracted the zip file within the “picture” folder. You’ll be able to see the picture knowledge is in JPEG format.
To learn the picture file, we use the ImageIO library. The medical business primarily works with DICOM format, and though “imageio” can learn DICOM format, we’ll solely work with JPEG format right this moment.
Create an inventory of all of the picture recordsdata within the prepare regular folder.
import os
import glob
# Supported picture file extensions
image_extensions = ['*.jpg', '*.jpeg', '*.png']
image_list = []
for ext in image_extensions:
image_files = glob.glob(os.path.be part of(image_dir, ext))
image_list.lengthen(image_files)
print("LIst of picture recordsdata in Practice/regular")
for image_path in image_list:
print(image_path)
Load a single picture
import os
import imageio.v3
FILE_PATH = "./photographs/x-rays/pneumonia-xray/prepare/regular/"
original_img = imageio.v3.imread(os.path.be part of(FILE_PATH, "IM-0115-0001.jpeg"))
Now see the form and knowledge sort of the picture
print(f"Form of the picture: ", original_img.form)
print(f"Knowledge Kind of the picture: ", original_img.dtype)

And the chest X-ray picture
import matplotlib.pyplot as plt
plt.imshow(original_img, cmap="grey")
plt.axis("off")
plt.present()
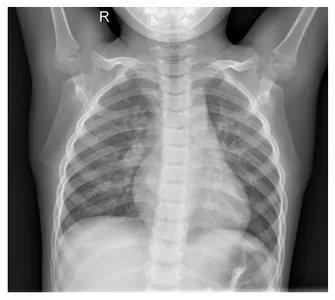
Create a GIF file to see the primary 10 photographs from the prepare folder.
First, create an inventory of 10 photographs:
import numpy as np
num_img = 10
arr = []
for i, img in enumerate(image_list):
if(i == num_img):
break
else:
temp_img = imageio.v3.imread(f"{img}")
arr.append(temp_img)
Creating GIF:
GIF_PATH = os.path.be part of("./photographs/","x-ray_img.gif")
imageio.mimwrite(GIF_PATH, arr, format=".gif", fps=1)
The gif file is just not added right here, as a result of the editor renders it.
Now, dive deep into edge detection.
Edge Detection on the X-ray Picture in Picture Processing
What’s Edge Detection?
It’s a mathematical operation utilized to the directional adjustments in colour or picture gradient. It’s used for detecting the boundary of a picture. There are a lot of operators for detecting edges e.g., gradient approach, Sobel operator, Canny edge detection, and so forth. See under examples
Unique Picture
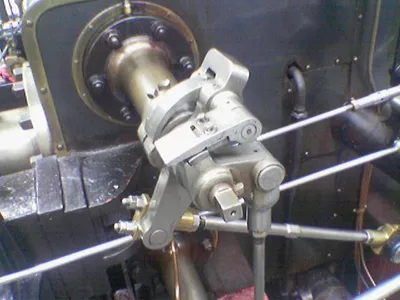
Supply: Wikipedia Sobel Operator
Detected Fringe of the Valve
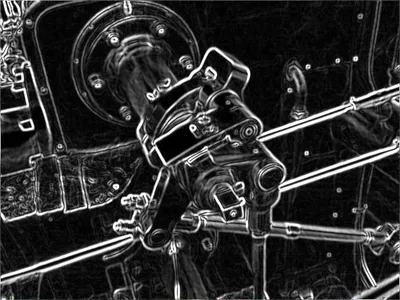
Supply: Wikipedia Sobel Operator
Varieties of Edge Detection Algorithms
Now we apply several types of edge detection algorithms to our Chest x-ray photographs. Let’s get this began!
1. The Laplace-Gaussian Filter
On this methodology, the Laplacian methodology focuses on altering the pixel depth, and the Gaussian methodology is used to take away noise from the photographs.
What’s Laplacian perform of the picture?
The Laplacian of the picture is outlined as the divergence of the gradient of the pixel depth perform, which is the same as the sum of the I(x,y) perform’s second spatial derivatives in cartesian coordinates.
If The Laplacian is L(x,y) and the pixel depth perform I(x,y). The formulation is
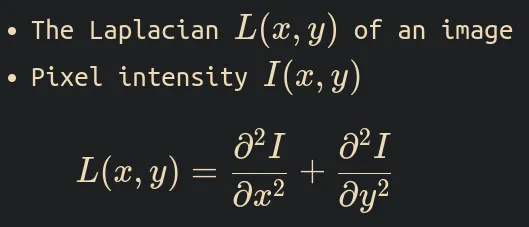
What’s Gaussian?
The Gaussian or regular distribution perform is a chance distribution utilized in statistics. Its form is sort of a bell. That’s why typically it’s known as the bell form curve. It’s vital that the curve is symmetric across the imply.
System:
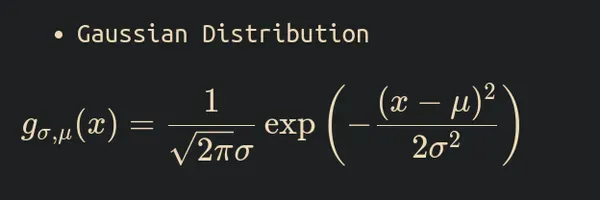
Right here, the Greek symbols sigma and mu are the usual deviation and imply of the distribution, respectively. Beneath is an instance plot.
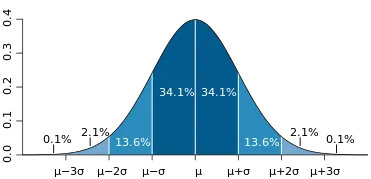
Supply: Wikipedia
In picture processing, Regular distribution is used to cut back noise from the picture. We are able to smoothen the picture by making use of a Gaussian filter a picture and decreasing the noise.
The Gaussian filter course of is a convolutional operation that replaces every pixel worth of the picture by taking the weighted common of the neighboring pixel values. e.g., the blurred picture under is produced after making use of the Gaussian Filter on the highest picture.
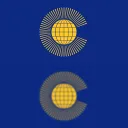
Supply: Wikipedia
And also you would possibly marvel why it’s known as the Gaussian. The reply is to take the weighted common of the neighboring pixels of the picture. The Gaussian distribution determines the burden.
The formulation of the 2nd by-product of Gaussian distribution is

The Laplace-Gaussian operator formulation is
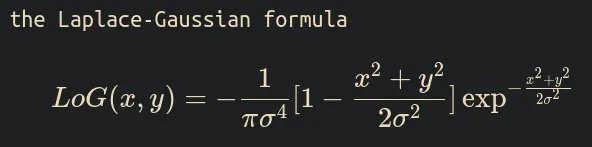
Sufficient math is finished now implementation.
Implementation
We implement the Laplace Gaussian operation on an X-ray picture utilizing SciPy ndimage’s gaussina_laplace() perform with a normal deviation of 1.
from scipy import ndimage
xray_LG = ndimage.gaussian_laplace(
original_img,
sigma=1
)
Now, we evaluate each the unique and filtered photographs aspect by aspect. So, first, we create a perform to plot the photographs utilizing the Matplolib pyplot library.
def plot_xray(image1, image2, title1="Unique", title2="Image2"):
fig, axes = plt.subplots(nrows=1, ncols=2, figsize=(10, 10))
axes[0].set_title(title1)
axes[0].imshow(image1, cmap="grey")
axes[1].set_title(title2)
axes[1].imshow(image2, cmap="grey")
for i in axes:
i.axis("off")
plt.present()
This perform will create the plot of each photographs.
Now see the outcomes.
plot_xray(original_img,xray_LG, title2="Laplace gaussian edges")
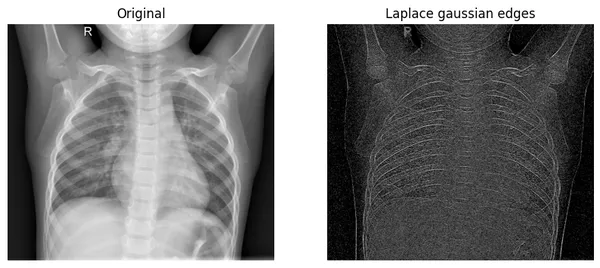
The operator particularly focuses on the place the pixel depth is modified very quickly. When you have a look at the unique picture’s bones, you discover that there are pixels in fast change. Our edges of the filtered picture are precisely exhibiting these adjustments.
Now proceed to the subsequent filter.
2. Gaussian Gradient Magnitude
What’s Gaussian gradient magnitude?
We already know what Gaussian or regular distribution within the Laplace-Gaussian filter is. Now, the Gradient of a picture is the adjustments within the depth or colour to a sure path.
Picture gradient instance.
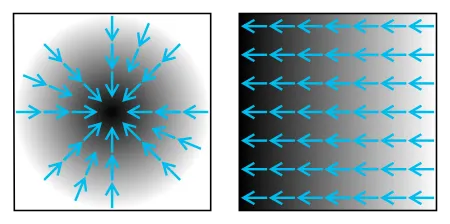
Mathematically, first, the algorithm applies the Gaussian filter on the picture in each the x-axis and y-axis or horizontally and vertically. It blurs the picture, however the total construction of the picture is unbroken.
Second, The magnitude is calculated by taking each gradient instructions’ sq. root or Euclidean distance.
On this methodology of edge detection, we take away the high-frequency parts from the picture utilizing multidimensional gradient magnitude with Gaussian by-product.
In picture processing, high-frequency means the sting half, and low-frequency means the physique a part of that picture.
Implementation
We use Scipy ndimage’s gaussian_gradient_magnitude() with a normal deviation(sigma) of two.
xray_GM = ndimage.gaussian_gradient_magnitude(
original_img,
sigma=2
)
Now, see the consequence by plotting
plot_xray(original_img, xray_GM, title2="Gaussian Gradient Magazine")
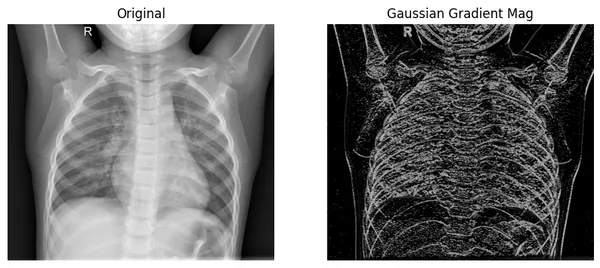
Right here, we will see that the filtered picture extracts the perimeters from the unique picture, the place colour values change quickly. And as compared with the Laplace-Gaussian methodology, it reveals edges higher.
3. Sobel Filter
The Sobel filter, also referred to as the Soble-Feldman operator utilized in picture processing. It makes use of convolution operation on the picture to calculate the approximation of the gradient.
How does it work?
There are two separate [3 by 3] kernels for every path. One for the x-axis or horizontal and one for the y-axis or vertical. These kernels convolve on the unique picture to calculate the gradient approximation of the unique picture in each horizontal and vertical instructions.
Then, It’ll calculate the magnitude by taking the sq. root of each directional gradients.
If A is a picture and G_x and G_y are horizontal and vertical by-product approximations at every level.
The computation shall be like

Compute the gradient with smoothi

Calculating the magnitude of each gradients.

Now, utilizing all the knowledge, we will calculate the gradient path utilizing

For instance, the path angle massive theta shall be 0 for the vertical edge.
Implementation
a. To implement the Sobel filter, we use ndimage’s sobel() perform. We should apply the Sobel filter on the x-axis and y-axis of the unique picture individually.
x_sb = ndimage.sobel(original_img, axis=0)
y_sb = ndimage.sobel(original_img, axis=1)
b. Then, we use np.hypot() to calculate euclidean distance of sobel_x and sobel_y
c. Final, normalize the picture.
# taking magnitude
sobel_img = np.hypot(x_sb, y_sb)
# Normalization
sobel_img *= 255.0 / np.max(sobel_img)
Now, the picture turns into float16 format, so we should rework it into float32 format for higher compatibility with the matplotlib.
print("The information sort - earlier than: ", sobel_img.dtype)
sobel_img = sobel_img.astype("float32")
print("The information sort - earlier than: ", sobel_img.dtype)
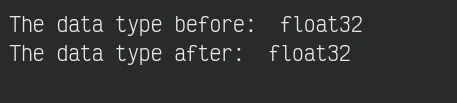
Let’s see the outcomes
We are going to plot the unique picture together with each grayscale and CMRmap colormap scale for higher visualization of filtered photographs.
fig, axes = plt.subplots(nrows=1, ncols=3, figsize=(15, 15))
axes[0].set_title("Unique")
axes[0].imshow(original_img, cmap="grey")
axes[1].set_title("Sobel_edges: grayscale")
axes[1].imshow(sobel_img, cmap="grey")
axes[2].set_title("Sobel_edges: CMRmap")
axes[2].imshow(sobel_img, cmap="CMRmap")
for i in axes:
i.axis("off")
plt.present()
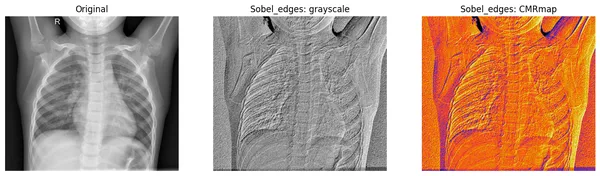
Within the ensuing plot, the Sobel filter focuses extra on the lung space of the chest X-ray.
4. The Canny Filter
In keeping with Wikipedia, The canny filter or canny edge detection is a method that applies a number of algorithms to the photographs to get the outcomes. It’s developed by John.F.Canny in 1986.
Normally, the algorithm works as
a. Noise Discount
It makes use of the Gaussian filter (deep studying) to cut back noise from the photographs. Right here, it makes use of the 5 by 5 Gaussian kernel for convolution.
b. Calculate Depth Gradient
We already know the way to discover the depth gradient within the Sobel filter part. In brief, It applies the Sobel filter on the smoothened picture within the horizontal and vertical path. After that, it calculates the gradient magnitude and gradient path.
c. Non-maximum Suppression
It’s a deep studying approach to take away the pixel which doesn’t represent the sting.
d. Hysteresis Thresholding
On this part, the deep studying algorithm calculates the perimeters and people not. There are two threshold values, min_val and max_val, for the perimeters. If the sure edge is greater than max_val, then it’s thought of a certain edge, and if the sting is under the min_val, then it’s thought of certain not edge, so it will likely be discarded.
The selection of min_val and max_val is vital for getting appropriate outcomes.
Implementation
To get the canny picture,
first, apply the Fourier Gaussian filter on the unique picture to get a smoother picture.
fourier_gau = ndimage.fourier_gaussian(
original_img,
sigma=0.05
)
Second, we calculate each the directional gradient utilizing Prewitt from Scipy ndimage.
x_prewitt = ndimage.prewitt(fourier_gau, axis=0)
y_prewitt = ndimage.prewitt(fourier_gau, axis=1)
And the final, We calculate the gradient by taking the sq. root of each gradients. After which normalizing the picture.
xray_canny = np.hypot(x_prewitt, y_prewitt)
xray_canny *= 255.0 / np.max(xray_canny)
The information sort of the ensuing picture
print(f"the info sort - {xray_canny.dtype}")
Let’s see the outcomes
fig, axes = plt.subplots(nrows=1, ncols=4, figsize=(20, 15))
axes[0].set_title("Unique")
axes[0].imshow(original_img, cmap="grey")
axes[1].set_title("Canny edge: prism")
axes[1].imshow(xray_canny, cmap="prism")
axes[2].set_title("Canny edge: nipy_spectral")
axes[2].imshow(xray_canny, cmap="nipy_spectral")
axes[3].set_title("Canny edges: terrain")
axes[3].imshow(xray_canny, cmap="terrain")
for i in axes:
i.axis("off")
plt.present()
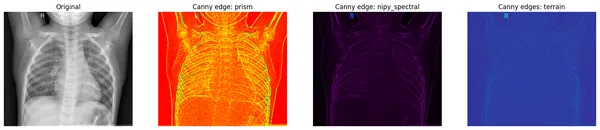
By masking sure pixels of a picture, we will extract the options from the unique photographs.
let’s present some photographs first.
Create a picture array of 9 photographs.
photographs = [
imageio.v3.imread(image_list[i]) for i in vary(9)
]
Plotting the Photos
n_images = len(photographs)
n_rows = 3
n_cols = (n_images + 1) // n_rows
fig, axes = plt.subplots(n_rows, n_cols, figsize=(12, 6))
axes = axes.flatten()
for i in vary(n_images):
if i < n_images:
axes[i].imshow(photographs[i])
axes[i].axis('off')
axes[i].set_title(f"Picture {i+1}")
else:
axes[i].axis("off")
for i in vary(n_images, n_rows * n_cols):
axes[i].axis("off")
fig.suptitle("Chest X-ray photographs")
plt.tight_layout()
plt.present()
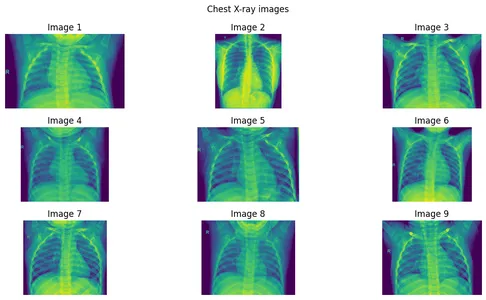
Now, See some primary statistics of pixel values
print("The information sort of the X-ray picture is: ", original_img.dtype)
print("The minimal pixel worth is: ", np.min(original_img))
print("The utmost pixel worth is: ", np.max(original_img))
print("The common pixel worth is: ", np.imply(original_img))
print("The median pixel worth is: ", np.median(original_img))
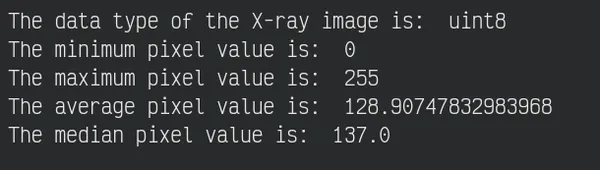
Plotting the Pixel density distribution of all of the above photographs.
fig, axes = plt.subplots(n_rows, n_cols, figsize=(12, 6))
axes = axes.flatten()
for i in vary(n_images):
if i < n_images:
pixel_int_dist = ndimage.histogram(photographs[i],
min=np.min(photographs[i]),
max=np.max(photographs[i]),
bins=256)
axes[i].plot(pixel_int_dist)
axes[i].set_xlim(0, 255)
axes[i].set_ylim(0, np.max(pixel_int_dist))
axes[i].set_title(f"PDD of img_{i+1}")
fig.suptitle("Pixel density distribution of Chest X-ray photographs")
plt.tight_layout()
plt.present()
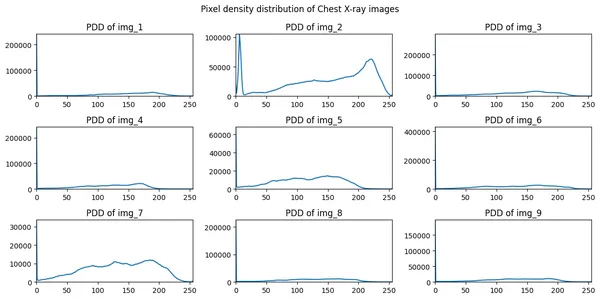
Extracting options from photographs utilizing masking
We use np.the place() with the edge worth of 150. This implies the pixel larger than 150 will keep in any other case will develop into 0.
fig, axes = plt.subplots(n_rows, n_cols, figsize=(12, 6))
axes = axes.flatten()
for i in vary(n_images):
if i < n_images:
noisy_image = np.the place(photographs[i] >150, photographs[i], 0 )
axes[i].imshow(noisy_image, cmap="grey")
axes[i].set_title(f"Picture {i+1}")
else:
axes[i].axis("off")
for i in vary(n_images, n_rows * n_cols):
axes[i].axis("off")
fig.suptitle("Chest X-ray photographs")
plt.tight_layout()
plt.present()
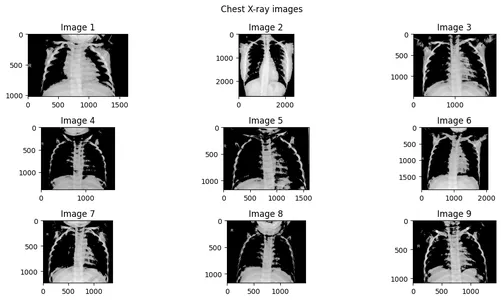
Now, we will see that the masked pixel is just not exhibiting or blackened by np.the place() methodology.
Conclusion
Edge detection is an important process for healthcare industries, deep studying picture classification initiatives, and Object detection initiatives. On this article, we study a lot of methods right this moment. You’ll be able to apply these methods in any picture classification venture on Kaggle competitions. And likewise you may additional research the topic to provide you with higher methods.
Key takeaways
- Studying about picture processing in deep studying.
- How fashionable arithmetic is utilized in Picture processing of deep studying.
- Laplacian gradient and utility of Gaussian distribution on picture processing.
- Sobel and canny filter operation.
- Utility of masking approach for options extraction in deep studying.
All of the codes of this text can be found right here (search for the x-ray-process.ipynb file)
Thanks for studying, When you just like the article please like and share it along with your fellow learners. Comply with me on LinkedIn
The media proven on this article is just not owned by Analytics Vidhya and is used on the Creator’s discretion.