Final Up to date on November 15, 2022
Two-dimensional tensors are analogous to two-dimensional metrics. Like a two-dimensional metric, a two-dimensional tensor additionally has $n$ variety of rows and columns.
Let’s take a gray-scale picture for instance, which is a two-dimensional matrix of numeric values, generally referred to as pixels. Starting from ‘0’ to ‘255’, every quantity represents a pixel depth worth. Right here, the bottom depth quantity (which is ‘0’) represents black areas within the picture whereas the best depth quantity (which is ‘255’) represents white areas within the picture. Utilizing the PyTorch framework, this two-dimensional picture or matrix will be transformed to a two-dimensional tensor.
Within the earlier put up, we realized about one-dimensional tensors in PyTorch and utilized some helpful tensor operations. On this tutorial, we’ll apply these operations to two-dimensional tensors utilizing the PyTorch library. Particularly, we’ll be taught:
- Find out how to create two-dimensional tensors in PyTorch and discover their sorts and shapes.
- About slicing and indexing operations on two-dimensional tensors intimately.
- To use numerous strategies to tensors akin to, tensor addition, multiplication, and extra.
Let’s get began.
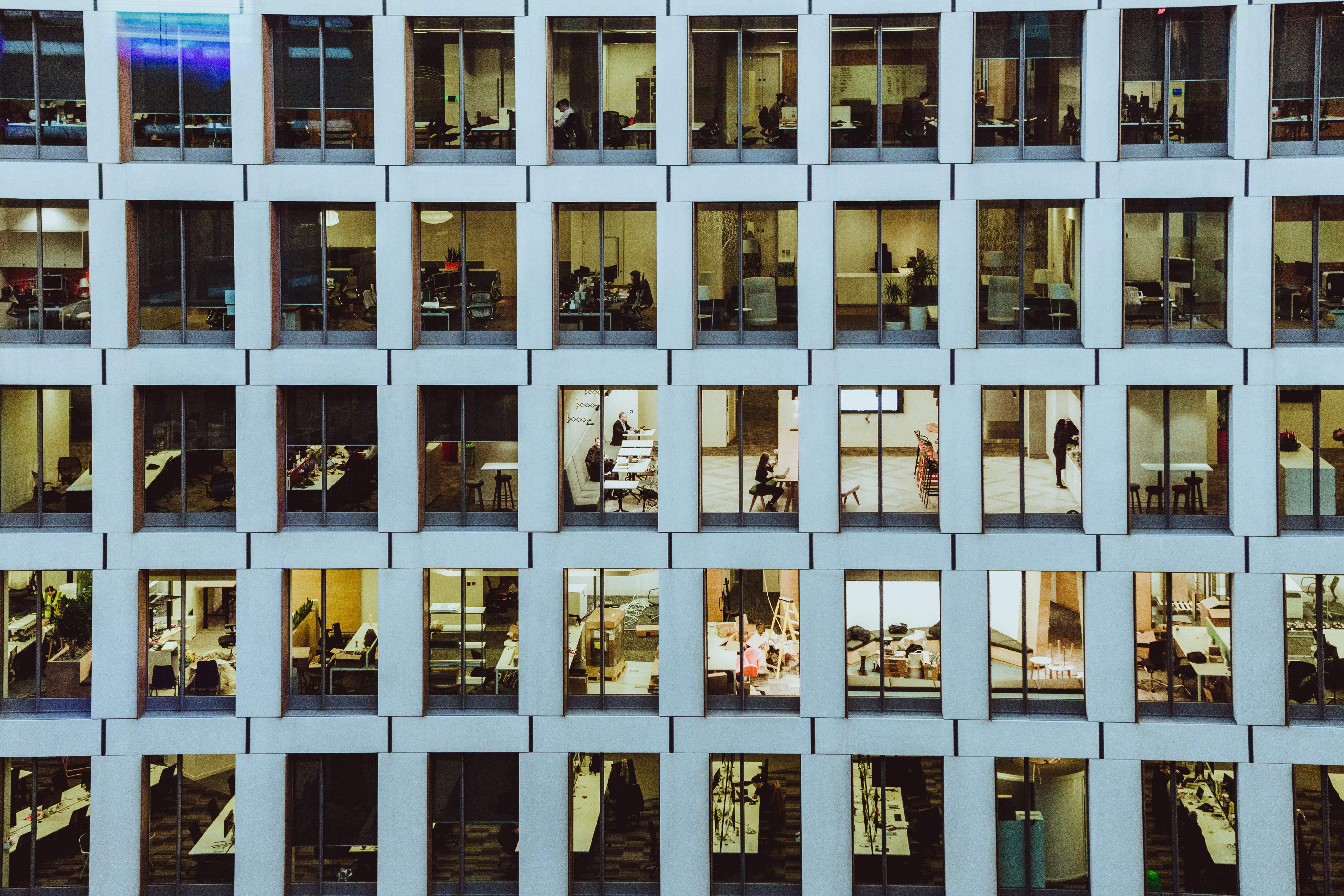
Two-Dimensional Tensors in Pytorch
Image by dylan dolte. Some rights reserved.
Tutorial Overview
This tutorial is split into elements; they’re:
- Varieties and shapes of two-dimensional tensors
- Changing two-dimensional tensors into NumPy arrays
- Changing pandas collection to two-dimensional tensors
- Indexing and slicing operations on two-dimensional tensors
- Operations on two-dimensional tensors
Varieties and Shapes of Two-Dimensional Tensors
Let’s first import a couple of obligatory libraries we’ll use on this tutorial.
import torch import numpy as np import pandas as pd |
To test the categories and shapes of the two-dimensional tensors, we’ll use the identical strategies from PyTorch, launched beforehand for one-dimensional tensors. However, ought to it work the identical approach it did for the one-dimensional tensors?
Let’s show by changing a 2D checklist of integers to a 2D tensor object. For instance, we’ll create a 2D checklist and apply torch.tensor()
for conversion.
example_2D_list = [[5, 10, 15, 20], [25, 30, 35, 40], [45, 50, 55, 60]] list_to_tensor = torch.tensor(example_2D_list) print(“Our New 2D Tensor from 2D Listing is: “, list_to_tensor) |
Our New 2D Tensor from 2D Listing is: tensor([[ 5, 10, 15, 20], [25, 30, 35, 40], [45, 50, 55, 60]]) |
As you possibly can see, the torch.tensor()
methodology additionally works properly for the two-dimensional tensors. Now, let’s use form()
, dimension()
, and ndimension()
strategies to return the form, dimension, and dimensions of a tensor object.
print(“Getting the form of tensor object: “, list_to_tensor.form) print(“Getting the scale of tensor object: “, list_to_tensor.dimension()) print(“Getting the scale of tensor object: “, list_to_tensor.ndimension()) |
print(“Getting the form of tensor object: “, list_to_tensor.form) print(“Getting the scale of tensor object: “, list_to_tensor.dimension()) print(“Getting the scale of tensor object: “, list_to_tensor.ndimension()) |
Changing Two-Dimensional Tensors to NumPy Arrays
PyTorch permits us to transform a two-dimensional tensor to a NumPy array after which again to a tensor. Let’s learn how.
# Changing two_D tensor to numpy array
twoD_tensor_to_numpy = list_to_tensor.numpy() print(“Changing two_Dimensional tensor to numpy array:”) print(“Numpy array after conversion: “, twoD_tensor_to_numpy) print(“Knowledge kind after conversion: “, twoD_tensor_to_numpy.dtype)
print(“***************************************************************”)
# Changing numpy array again to a tensor
back_to_tensor = torch.from_numpy(twoD_tensor_to_numpy) print(“Changing numpy array again to two_Dimensional tensor:”) print(“Tensor after conversion:”, back_to_tensor) print(“Knowledge kind after conversion: “, back_to_tensor.dtype) |
Changing two_Dimensional tensor to numpy array: Numpy array after conversion: [[ 5 10 15 20] [25 30 35 40] [45 50 55 60]] Knowledge kind after conversion: int64 *************************************************************** Changing numpy array again to two_Dimensional tensor: Tensor after conversion: tensor([[ 5, 10, 15, 20], [25, 30, 35, 40], [45, 50, 55, 60]]) Knowledge kind after conversion: torch.int64 |
Changing Pandas Sequence to Two-Dimensional Tensors
Equally, we are able to additionally convert a pandas DataFrame to a tensor. As with the one-dimensional tensors, we’ll use the identical steps for the conversion. Utilizing values attribute we’ll get the NumPy array after which use torch.from_numpy
that means that you can convert a pandas DataFrame to a tensor.
Right here is how we’ll do it.
# Changing Pandas Dataframe to a Tensor
dataframe = pd.DataFrame({‘x’:[22,24,26],‘y’:[42,52,62]})
print(“Pandas to numpy conversion: “, dataframe.values) print(“Knowledge kind earlier than tensor conversion: “, dataframe.values.dtype)
print(“***********************************************”)
pandas_to_tensor = torch.from_numpy(dataframe.values) print(“Getting new tensor: “, pandas_to_tensor) print(“Knowledge kind after conversion to tensor: “, pandas_to_tensor.dtype) |
Pandas to numpy conversion: [[22 42] [24 52] [26 62]] Knowledge kind earlier than tensor conversion: int64 *********************************************** Getting new tensor: tensor([[22, 42], [24, 52], [26, 62]]) Knowledge kind after conversion to tensor: torch.int64 |
Indexing and Slicing Operations on Two-Dimensional Tensors
For indexing operations, totally different components in a tensor object will be accessed utilizing sq. brackets. You’ll be able to merely put corresponding indices in sq. brackets to entry the specified components in a tensor.
Within the beneath instance, we’ll create a tensor and entry sure components utilizing two totally different strategies. Observe that the index worth ought to all the time be one lower than the place the ingredient is positioned in a two-dimensional tensor.
example_tensor = torch.tensor([[10, 20, 30, 40], [50, 60, 70, 80], [90, 100, 110, 120]]) print(“Accessing ingredient in 2nd row and 2nd column: “, example_tensor[1, 1]) print(“Accessing ingredient in 2nd row and 2nd column: “, example_tensor[1][1])
print(“********************************************************”)
print(“Accessing ingredient in third row and 4th column: “, example_tensor[2, 3]) print(“Accessing ingredient in third row and 4th column: “, example_tensor[2][3]) |
Accessing ingredient in 2nd row and 2nd column: tensor(60) Accessing ingredient in 2nd row and 2nd column: tensor(60) ******************************************************** Accessing ingredient in third row and 4th column: tensor(120) Accessing ingredient in third row and 4th column: tensor(120) |
What if we have to entry two or extra components on the identical time? That’s the place tensor slicing comes into play. Let’s use the earlier instance to entry first two components of the second row and first three components of the third row.
example_tensor = torch.tensor([[10, 20, 30, 40], [50, 60, 70, 80], [90, 100, 110, 120]]) print(“Accessing first two components of the second row: “, example_tensor[1, 0:2]) print(“Accessing first two components of the second row: “, example_tensor[1][0:2])
print(“********************************************************”)
print(“Accessing first three components of the third row: “, example_tensor[2, 0:3]) print(“Accessing first three components of the third row: “, example_tensor[2][0:3]) |
example_tensor = torch.tensor([[10, 20, 30, 40], [50, 60, 70, 80], [90, 100, 110, 120]]) print(“Accessing first two components of the second row: “, example_tensor[1, 0:2]) print(“Accessing first two components of the second row: “, example_tensor[1][0:2])
print(“********************************************************”)
print(“Accessing first three components of the third row: “, example_tensor[2, 0:3]) print(“Accessing first three components of the third row: “, example_tensor[2][0:3]) |
Operations on Two-Dimensional Tensors
Whereas there are lots of operations you possibly can apply on two-dimensional tensors utilizing the PyTorch framework, right here, we’ll introduce you to tensor addition, and scalar and matrix multiplication.
Including Two-Dimensional Tensors
Including two tensors is just like matrix addition. It’s fairly a straight ahead course of as you merely want an addition (+) operator to carry out the operation. Let’s add two tensors within the beneath instance.
A = torch.tensor([[5, 10], [50, 60], [100, 200]]) B = torch.tensor([[10, 20], [60, 70], [200, 300]]) add = A + B print(“Including A and B to get: “, add) |
Including A and B to get: tensor([[ 15, 30], [110, 130], [300, 500]]) |
Scalar and Matrix Multiplication of Two-Dimensional Tensors
Scalar multiplication in two-dimensional tensors can also be an identical to scalar multiplication in matrices. As an example, by multiplying a tensor with a scalar, say a scalar 4, you’ll be multiplying each ingredient in a tensor by 4.
new_tensor = torch.tensor([[1, 2, 3], [4, 5, 6]]) mul_scalar = 4 * new_tensor print(“results of scalar multiplication: “, mul_scalar) |
results of scalar multiplication: tensor([[ 4, 8, 12], [16, 20, 24]]) |
Coming to the multiplication of the two-dimensional tensors, torch.mm()
in PyTorch makes issues simpler for us. Much like the matrix multiplication in linear algebra, variety of columns in tensor object A (i.e. 2×3) should be equal to the variety of rows in tensor object B (i.e. 3×2).
A = torch.tensor([[3, 2, 1], [1, 2, 1]]) B = torch.tensor([[3, 2], [1, 1], [2, 1]]) A_mult_B = torch.mm(A, B) print(“multiplying A with B: “, A_mult_B) |
multiplying A with B: tensor([[13, 9], [ 7, 5]]) |
Additional Studying
Developed similtaneously TensorFlow, PyTorch used to have an easier syntax till TensorFlow adopted Keras in its 2.x model. To be taught the fundamentals of PyTorch, you could wish to learn the PyTorch tutorials:
Particularly the fundamentals of PyTorch tensor will be discovered within the Tensor tutorial web page:
There are additionally fairly a couple of books on PyTorch which might be appropriate for rookies. A extra just lately revealed e book needs to be beneficial because the instruments and syntax are actively evolving. One instance is
Abstract
On this tutorial, you realized about two-dimensional tensors in PyTorch.
Particularly, you realized:
- Find out how to create two-dimensional tensors in PyTorch and discover their sorts and shapes.
- About slicing and indexing operations on two-dimensional tensors intimately.
- To use numerous strategies to tensors akin to, tensor addition, multiplication, and extra.