C++ strings are sequences of characters saved in a char array. Strings are used to retailer phrases and textual content. They’re additionally used to retailer information, equivalent to numbers and different forms of info. Strings in C++ could be outlined both utilizing the std::string class or the C-style character arrays.
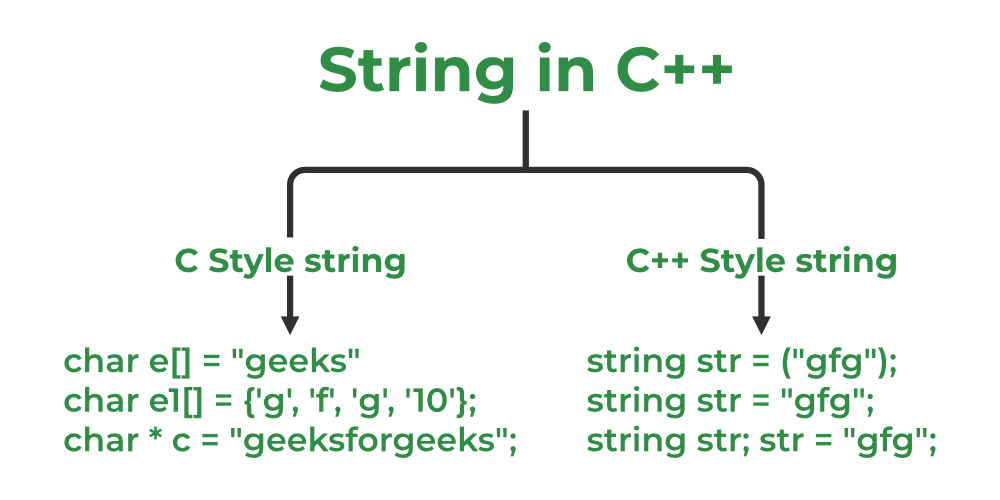
1. C Type Strings
These strings are saved because the plain outdated array of characters terminated by a null character ‘ ’. They’re the kind of strings that C++ inherited from C language.
Syntax:
char str[] = "GeeksforGeeks";
Instance:
C++
|
2. std::string Class
These are the brand new sort of strings which can be launched in C++ as std::string class outlined inside <string> header file. This supplies many benefits over typical C-Type strings equivalent to dynamic dimension, member capabilities, and many others.
Syntax:
std::string str("GeeksforGeeks");
Instance:
C++
|
Methods to Outline a String in C++
Strings could be outlined in a number of methods in C++. Strings could be accessed from the usual library utilizing the string class. Character arrays will also be used to outline strings. String supplies a wealthy set of options, equivalent to looking and manipulating, that are generally used strategies. Regardless of being much less superior than the string class, this technique continues to be broadly used, as it’s extra environment friendly and simpler to make use of. Methods to outline a string in C++ are:
- Utilizing String key phrase
- Utilizing C-style strings
1. Utilizing string Key phrase
It’s extra handy to outline a string with the string key phrase as an alternative of utilizing the array key phrase as a result of it’s simple to jot down and perceive.
Syntax:
string s = "GeeksforGeeks"; string s("GeeksforGeeks");
Instance:
C++
|
s = GeeksforGeeks str = GeeksforGeeks
2. Utilizing C-style strings
Utilizing C-style string libraries capabilities equivalent to strcpy(), strcmp(), and strcat() to outline strings. This technique is extra complicated and never as broadly used as the opposite two, however it may be helpful when coping with legacy code or if you want efficiency.
char s[] = {'g', 'f', 'g', ' '}; char s[4] = {'g', 'f', 'g', ' '}; char s[4] = "gfg"; char s[] = "gfg";
Instance:
C++
|
s1 = gfg s2 = gfg s3 = gfg s4 = gfg
How you can Take String Enter in C++
String enter means accepting a string from a consumer. In C++. We’ve got various kinds of taking enter from the consumer which depend upon the string. The most typical means is to take enter with cin key phrase with the extraction operator (>>) in C++. Strategies to take a string as enter are:
1. Utilizing Cin
The only method to take string enter is to make use of the cin command together with the stream extraction operator (>>).
Syntax:
cin>>s;
Instance:
C++
|
Output:
Enter String GeeksforGeeks String is: GeeksforGeeks
2. Utilizing getline
The getline() operate in C++ is used to learn a string from an enter stream. It’s declared within the <string> header file.
Syntax:
getline(cin,s);
Instance:
C++
|
Output:
Enter String GeeksforGeeks String is: GeeksforGeeks
3. Utilizing stringstream
The stringstream class in C++ is used to take a number of strings as enter without delay.
Syntax:
stringstream stringstream_object(string_name);
Instance:
C++
|
GeeksforGeeks to the Moon
How you can Go Strings to Features?
In the identical means that we go an array to a operate, strings in C++ could be handed to capabilities as character arrays. Right here is an instance program:
Instance:
C++
|
Handed String is: GeeksforGeeks
Pointers and Strings
Pointers in C++ are symbolic representations of addresses. They allow packages to simulate call-by-reference in addition to to create and manipulate dynamic information constructions. Through the use of pointers we are able to get the primary character of the string, which is the beginning deal with of the string. As proven beneath, the given string could be accessed and printed by means of the pointers.
Instance:
C++
|
Distinction between String and Character array in C++
The primary distinction between a string and a personality array is that strings are immutable, whereas character arrays should not.
String |
Character Array |
---|---|
Strings outline objects that may be represented as string streams. | The null character terminates a personality array of characters. |
No Array decay happens in strings as strings are represented as objects. | The specter of array decay is current within the case of the character array |
A string class supplies quite a few capabilities for manipulating strings. | Character arrays don’t provide inbuilt capabilities to govern strings. |
Reminiscence is allotted dynamically. | The dimensions of the character array must be allotted statically. |
Know extra concerning the distinction between strings and character arrays in C++
C++ String Features
C++ supplies some inbuilt capabilities that are used for string manipulation, such because the strcpy() and strcat() capabilities for copying and concatenating strings. A few of them are:
Perform |
Description |
---|---|
size() | This operate returns the size of the string. |
swap() | This operate is used to swap the values of two strings. |
dimension() | Used to search out the dimensions of string |
resize() | This operate is used to resize the size of the string as much as the given variety of characters. |
discover() | Used to search out the string which is handed in parameters |
push_back() | This operate is used to push the handed character on the finish of the string |
pop_back() | This operate is used to pop the final character from the string |
clear() | This operate is used to take away all the weather of the string. |
strncmp() | This operate compares at most the primary num bytes of each handed strings. |
strncpy() | This operate is just like strcpy() operate, besides that at most n bytes of src are copied |
strrchr() | This operate locates the final incidence of a personality within the string. |
strcat() | This operate appends a replica of the supply string to the tip of the vacation spot string |
discover() | This operate is used to seek for a sure substring inside a string and returns the place of the primary character of the substring. |
substr() | This operate is used to create a substring from a given string. |
evaluate() | This operate is used to match two strings and returns the consequence within the type of an integer. |
erase() | This operate is used to take away a sure a part of a string. |
C++ Strings iterator capabilities
In C++ inbuilt string iterator capabilities present the programmer with a simple method to modify and traverse string parts. These capabilities are:
Features | Description |
---|---|
start() | This operate returns an iterator pointing to the start of the string. |
finish() | This operate returns an iterator that factors to the tip of the string. |
rfind() | This operate is used to search out the string’s final incidence. |
rbegin() | This operate returns a reverse iterator pointing to the tip of the string. |
rend() | This operate returns a reverse iterator pointing to the start of the string. |
cbegin() | This operate returns a const_iterator pointing to the start of the string. |
cend() | This operate returns a const_iterator pointing to the tip of the string. |
crbegin() | This operate returns a const_reverse_iterator pointing to the tip of the string. |
crend() | This operate returns a const_reverse_iterator pointing to the start of the string. |
Instance:
C++
|
Pointing to the beginning of the string: G Pointing to the tip of the string: s Pointing to the final character of the string: s Pointing to the primary character of the string: G
String Capability Features
In C++, string capability capabilities are used to handle string dimension and capability. Main capabilities of capability embrace:
Perform | Description |
---|---|
size() | This operate is used to return the dimensions of the string |
capability() | This operate returns the capability which is allotted to the string by the compiler |
resize() | This operate permits us to extend or lower the string dimension |
shrink_to_fit() | This operate decreases the capability and makes it equal to the minimal. |
Instance:
C++
|
The size of the string is 13 The capability of string is 13 The string after utilizing resize funtion is GeeksforGe The capability of string earlier than utilizing shrink_to_fit operate is 26 The capability of string after utilizing shrink_to_fit operate is 20
In conclusion, this text explains how strings could be defied in C++ utilizing character arrays and string lessons. The string class supplies extra superior options, whereas the character array supplies fundamental options however is environment friendly and straightforward to make use of. On this article, we additionally mentioned the assorted strategies to take enter from the consumer.
To know extra about std::string class, seek advice from the article – std::string class in C++