Introduction
Renaming column names in Pandas refers back to the course of of adjusting the names of a number of columns in a DataFrame. By renaming columns, we are able to make our knowledge extra readable, significant, and constant. It’s a quite common activity in knowledge manipulation and evaluation, and so, should be identified to all. On this article, we are going to discover the varied strategies used to rename columns in Pandas, together with the very best practices and examples.
The Significance of Renaming Column Names
Column names play a vital position in knowledge evaluation as they supply context and that means to the information. Renaming column names could make our code extra readable and comprehensible, particularly when working with giant datasets. It additionally helps in sustaining consistency throughout completely different datasets and facilitates simpler knowledge merging and manipulation.
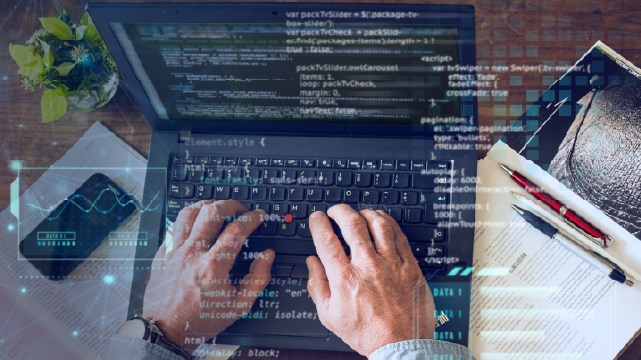
Overview of Pandas Library in Python
Earlier than diving into the small print of renaming column names in Pandas, let’s have a short overview of the Pandas library in Python. Pandas is a strong open-source knowledge manipulation and evaluation library that gives easy-to-use knowledge constructions and knowledge evaluation instruments. It’s constructed on high of the NumPy library and is broadly utilized in knowledge science and analytics.
Renaming Columns in Pandas
Pandas gives a number of strategies to rename column names in a DataFrame. Let’s discover a few of these strategies:
Utilizing the rename() Operate
The rename() perform in Pandas permits us to rename column names by offering a dictionary-like object or a mapping perform. We will specify the outdated column title as the important thing and the brand new column title as the worth within the dictionary. Right here’s an instance:
Instance 1:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df = df.rename(columns={'A': 'Column1', 'B': 'Column2'})
Utilizing the rename_axis() Operate
The rename_axis() perform in Pandas permits us to rename the index or column labels of a DataFrame. We will specify the brand new label utilizing the `columns` parameter. Right here’s an instance:
Instance 2:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df = df.rename_axis(columns="NewColumn")
Renaming Columns Primarily based on Particular Standards
In some instances, we could wish to rename columns primarily based on particular standards, such because the column index or title. Pandas gives strategies to rename columns primarily based on these standards.
Renaming Columns by Index
To rename columns primarily based on their index, we are able to use the `set_axis()` perform in Pandas. We have to specify the brand new column names as a listing and cross the `axis` parameter as 1. Right here’s an instance:
Instance 3:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df = df.set_axis(['Column1', 'Column2'], axis=1)
Renaming Columns by Identify
To rename columns primarily based on their title, we are able to use the `rename()` perform in Pandas. We have to specify the outdated and new column names as a dictionary-like object. Right here’s an instance:
Instance 4:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df = df.rename(columns={'A': 'Column1', 'B': 'Column2'})
Renaming Columns Utilizing a Dictionary
Pandas additionally permits us to rename columns utilizing a dictionary. We will specify the outdated and new column names as key-value pairs within the dictionary. Right here’s an instance:
Instance 5:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df = df.rename(columns={'A': 'Column1', 'B': 'Column2'})
Renaming Columns Whereas Studying a CSV File
One other methodology of renaming columns in Pandas entails renaming columns whereas studying a CSV file. This may be accomplished utilizing the rename parameter of the read_csv perform.
Instance 6:
import pandas as pd
# Learn the CSV file and rename columns
df = pd.read_csv("your_file.csv", names=['NewColumn1', 'NewColumn2', 'NewColumn3'], header=None)
On this instance, the names parameter is used to supply a listing of column names that will probably be used as a substitute of the names current within the CSV file. The header=None parameter is used to point that the CSV file doesn’t have a header row with column names.
Dealing with Duplicate Column Names
Duplicate column names may cause confusion and result in errors in knowledge evaluation. Pandas gives strategies to determine and rename duplicate column names.
Figuring out Duplicate Column Names
To determine duplicate column names in a DataFrame, we are able to use the `duplicated()` perform in Pandas. It returns a boolean Collection indicating whether or not every column title is duplicated or not. Right here’s an instance:
Instance 7:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'A': [7, 8, 9]})
duplicated_columns = df.columns[df.columns.duplicated()]
Renaming Duplicate Column Names
To rename duplicate column names, we are able to append a suffix or prefix to the column names utilizing the `add_suffix()` or `add_prefix()` capabilities in Pandas. Right here’s an instance:
Instance 8:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'A': [7, 8, 9]})
df = df.add_suffix('_duplicate')
Examples and Use Circumstances
Let’s discover some examples and use instances to know rename column names in Pandas.
Renaming Columns in a Pandas DataFrame
Instance 9:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df = df.rename(columns={'A': 'Column1', 'B': 'Column2'})
Renaming Columns in a MultiIndex DataFrame
Instance 10:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df.columns = pd.MultiIndex.from_tuples([('Column1', 'SubColumn1'), ('Column2', 'SubColumn2')])
Conclusion
Renaming column names in Pandas is a vital step in knowledge manipulation and evaluation. By following the strategies and practices mentioned on this article, you possibly can successfully rename column names in your Pandas DataFrame. Bear in mind to decide on descriptive and constant names, keep away from reserved key phrases and particular characters, and deal with duplicate column names appropriately. Blissful coding!