As a library developer, you could create a preferred utility that lots of of
hundreds of builders depend on day by day, corresponding to lodash or React. Over time,
utilization patterns may emerge that transcend your preliminary design. When this
occurs, you could want to increase an API by including parameters or modifying
operate signatures to repair edge circumstances. The problem lies in rolling out
these breaking modifications with out disrupting your customers’ workflows.
That is the place codemods are available in—a strong instrument for automating
large-scale code transformations, permitting builders to introduce breaking
API modifications, refactor legacy codebases, and preserve code hygiene with
minimal handbook effort.
On this article, we’ll discover what codemods are and the instruments you may
use to create them, corresponding to jscodeshift, hypermod.io, and codemod.com. We’ll stroll by way of real-world examples,
from cleansing up function toggles to refactoring element hierarchies.
You’ll additionally learn to break down advanced transformations into smaller,
testable items—a apply often known as codemod composition—to make sure
flexibility and maintainability.
By the tip, you’ll see how codemods can change into an important a part of your
toolkit for managing large-scale codebases, serving to you retain your code clear
and maintainable whereas dealing with even essentially the most difficult refactoring
duties.
Breaking Modifications in APIs
Returning to the situation of the library developer, after the preliminary
launch, new utilization patterns emerge, prompting the necessity to lengthen an
For easy modifications, a primary find-and-replace within the IDE may work. In
extra advanced circumstances, you may resort to utilizing instruments like sed
or awk
. Nevertheless, when your library is extensively adopted, the
scope of such modifications turns into tougher to handle. You possibly can’t ensure how
extensively the modification will affect your customers, and the very last thing
you need is to interrupt current performance that doesn’t want
updating.
A standard strategy is to announce the breaking change, launch a brand new
model, and ask customers emigrate at their very own tempo. However this workflow,
whereas acquainted, typically does not scale properly, particularly for main shifts.
Think about React’s transition from class parts to operate parts
with hooks—a paradigm shift that took years for giant codebases to totally
undertake. By the point groups managed emigrate, extra breaking modifications have been
typically already on the horizon.
For library builders, this case creates a burden. Sustaining
a number of older variations to help customers who haven’t migrated is each
expensive and time-consuming. For customers, frequent modifications threat eroding belief.
They could hesitate to improve or begin exploring extra secure alternate options,
which perpetuating the cycle.
However what if you happen to may assist customers handle these modifications routinely?
What if you happen to may launch a instrument alongside your replace that refactors
their code for them—renaming capabilities, updating parameter order, and
eradicating unused code with out requiring handbook intervention?
That’s the place codemods are available in. A number of libraries, together with React
and Subsequent.js, have already embraced codemods to clean the trail for model
bumps. For instance, React offers codemods to deal with the migration from
older API patterns, just like the outdated Context API, to newer ones.
So, what precisely is the codemod we’re speaking about right here?
What’s a Codemod?
A codemod (code modification) is an automatic script used to rework
code to observe new APIs, syntax, or coding requirements. Codemods use
Summary Syntax Tree (AST) manipulation to use constant, large-scale
modifications throughout codebases. Initially developed at Fb, codemods helped
engineers handle refactoring duties for giant tasks like React. As
Fb scaled, sustaining the codebase and updating APIs turned
more and more troublesome, prompting the event of codemods.
Manually updating hundreds of information throughout completely different repositories was
inefficient and error-prone, so the idea of codemods—automated scripts
that rework code—was launched to sort out this downside.
The method usually entails three fundamental steps:
- Parsing the code into an AST, the place every a part of the code is
represented as a tree construction. - Modifying the tree by making use of a metamorphosis, corresponding to renaming a
operate or altering parameters. - Rewriting the modified tree again into the supply code.
By utilizing this strategy, codemods be certain that modifications are utilized
persistently throughout each file in a codebase, decreasing the prospect of human
error. Codemods can even deal with advanced refactoring eventualities, corresponding to
modifications to deeply nested buildings or eradicating deprecated API utilization.
If we visualize the method, it will look one thing like this:
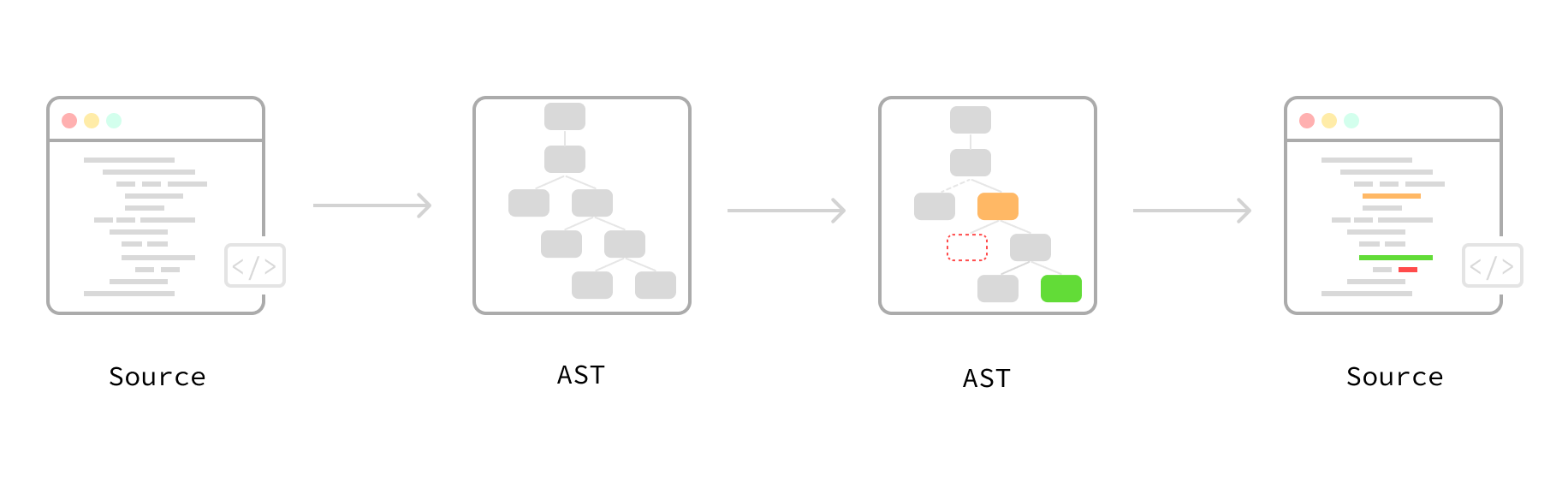
Determine 1: The three steps of a typical codemod course of
The thought of a program that may “perceive” your code after which carry out
computerized transformations isn’t new. That’s how your IDE works if you
run refactorings like
Basically, your IDE parses the supply code into ASTs and applies
predefined transformations to the tree, saving the consequence again into your
information.
For contemporary IDEs, many issues occur underneath the hood to make sure modifications
are utilized accurately and effectively, corresponding to figuring out the scope of
the change and resolving conflicts like variable title collisions. Some
refactorings even immediate you to enter parameters, corresponding to when utilizing
order of parameters or default values earlier than finalizing the change.
Use jscodeshift in JavaScript Codebases
Let’s take a look at a concrete instance to grasp how we may run a
codemod in a JavaScript undertaking. The JavaScript neighborhood has a number of
instruments that make this work possible, together with parsers that convert supply
code into an AST, in addition to transpilers that may rework the tree into
different codecs (that is how TypeScript works). Moreover, there are
instruments that assist apply codemods to total repositories routinely.
Probably the most common instruments for writing codemods is jscodeshift, a toolkit maintained by Fb.
It simplifies the creation of codemods by offering a strong API to
manipulate ASTs. With jscodeshift, builders can seek for particular
patterns within the code and apply transformations at scale.
You should use jscodeshift
to determine and substitute deprecated API calls
with up to date variations throughout a whole undertaking.
Let’s break down a typical workflow for composing a codemod
manually.
Clear a Stale Characteristic Toggle
Let’s begin with a easy but sensible instance to display the
energy of codemods. Think about you’re utilizing a function
toggle in your
codebase to regulate the discharge of unfinished or experimental options.
As soon as the function is reside in manufacturing and dealing as anticipated, the subsequent
logical step is to scrub up the toggle and any associated logic.
As an illustration, think about the next code:
const information = featureToggle('feature-new-product-list') ? { title: 'Product' } : undefined;
As soon as the function is absolutely launched and not wants a toggle, this
may be simplified to:
const information = { title: 'Product' };
The duty entails discovering all cases of featureToggle
within the
codebase, checking whether or not the toggle refers to
feature-new-product-list
, and eradicating the conditional logic surrounding
it. On the identical time, different function toggles (like
feature-search-result-refinement
, which can nonetheless be in improvement)
ought to stay untouched. The codemod must perceive the construction
of the code to use modifications selectively.
Understanding the AST
Earlier than we dive into writing the codemod, let’s break down how this
particular code snippet seems to be in an AST. You should use instruments like AST
Explorer to visualise how supply code and AST
are mapped. It’s useful to grasp the node sorts you are interacting
with earlier than making use of any modifications.
The picture under exhibits the syntax tree by way of ECMAScript syntax. It
comprises nodes like Identifier
(for variables), StringLiteral
(for the
toggle title), and extra summary nodes like CallExpression
and
ConditionalExpression
.
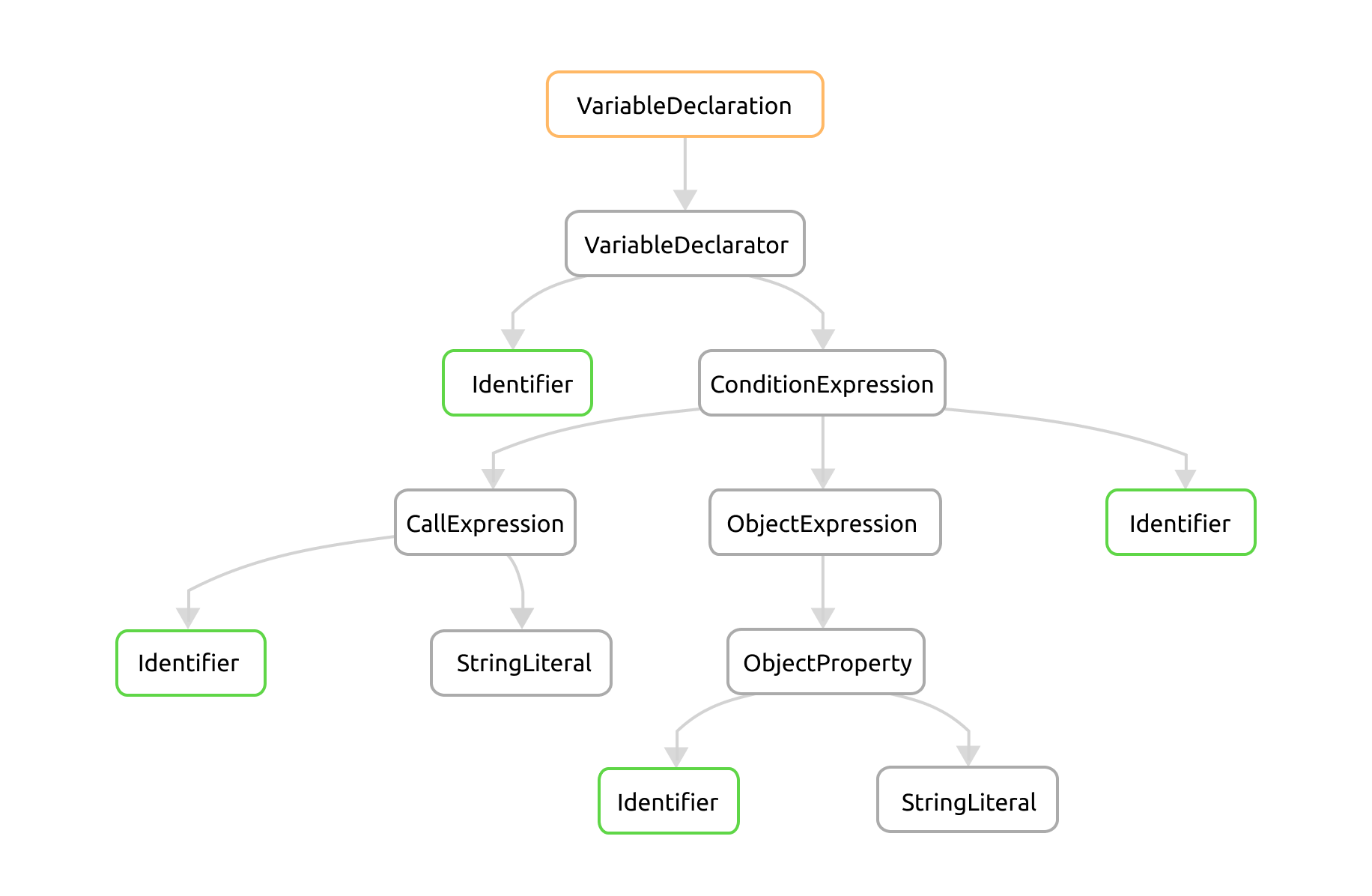
Determine 2: The Summary Syntax Tree illustration of the function toggle test
On this AST illustration, the variable information
is assigned utilizing a
ConditionalExpression
. The take a look at a part of the expression calls
featureToggle('feature-new-product-list')
. If the take a look at returns true
,
the consequent department assigns { title: 'Product' }
to information
. If
false
, the alternate department assigns undefined
.
For a activity with clear enter and output, I want writing assessments first,
then implementing the codemod. I begin by defining a damaging case to
guarantee we don’t by chance change issues we wish to depart untouched,
adopted by an actual case that performs the precise conversion. I start with
a easy situation, implement it, then add a variation (like checking if
featureToggle is known as inside an if assertion), implement that case, and
guarantee all assessments cross.
This strategy aligns properly with Take a look at-Pushed Growth (TDD), even
if you happen to don’t apply TDD often. Realizing precisely what the
transformation’s inputs and outputs are earlier than coding improves security and
effectivity, particularly when tweaking codemods.
With jscodeshift, you may write assessments to confirm how the codemod
behaves:
const rework = require("../remove-feature-new-product-list"); defineInlineTest( rework, {}, ` const information = featureToggle('feature-new-product-list') ? { title: 'Product' } : undefined; `, ` const information = { title: 'Product' }; `, "delete the toggle feature-new-product-list in conditional operator" );
The defineInlineTest
operate from jscodeshift permits you to outline
the enter, anticipated output, and a string describing the take a look at’s intent.
Now, operating the take a look at with a traditional jest
command will fail as a result of the
codemod isn’t written but.
The corresponding damaging case would make sure the code stays unchanged
for different function toggles:
defineInlineTest( rework, {}, ` const information = featureToggle('feature-search-result-refinement') ? { title: 'Product' } : undefined; `, ` const information = featureToggle('feature-search-result-refinement') ? { title: 'Product' } : undefined; `, "don't change different function toggles" );
Writing the Codemod
Let’s begin by defining a easy rework operate. Create a file
referred to as rework.js
with the next code construction:
module.exports = operate(fileInfo, api, choices) { const j = api.jscodeshift; const root = j(fileInfo.supply); // manipulate the tree nodes right here return root.toSource(); };
This operate reads the file right into a tree and makes use of jscodeshift’s API to
question, modify, and replace the nodes. Lastly, it converts the AST again to
supply code with .toSource()
.
Now we will begin implementing the rework steps:
- Discover all cases of
featureToggle
. - Confirm that the argument handed is
'feature-new-product-list'
. - Substitute all the conditional expression with the consequent half,
successfully eradicating the toggle.
Right here’s how we obtain this utilizing jscodeshift
:
module.exports = operate (fileInfo, api, choices) { const j = api.jscodeshift; const root = j(fileInfo.supply); // Discover ConditionalExpression the place the take a look at is featureToggle('feature-new-product-list') root .discover(j.ConditionalExpression, { take a look at: { callee: { title: "featureToggle" }, arguments: [{ value: "feature-new-product-list" }], }, }) .forEach((path) => { // Substitute the ConditionalExpression with the 'consequent' j(path).replaceWith(path.node.consequent); }); return root.toSource(); };
The codemod above:
- Finds
ConditionalExpression
nodes the place the take a look at calls
featureToggle('feature-new-product-list')
. - Replaces all the conditional expression with the resultant (i.e.,
{
), eradicating the toggle logic and leaving simplified code
title: 'Product' }
behind.
This instance demonstrates how simple it’s to create a helpful
transformation and apply it to a big codebase, considerably decreasing
handbook effort.
You’ll want to jot down extra take a look at circumstances to deal with variations like
if-else
statements, logical expressions (e.g.,
!featureToggle('feature-new-product-list')
), and so forth to make the
codemod strong in real-world eventualities.
As soon as the codemod is prepared, you may check it out on a goal codebase,
such because the one you are engaged on. jscodeshift offers a command-line
instrument that you should utilize to use the codemod and report the outcomes.
$ jscodeshift -t transform-name src/
After validating the outcomes, test that each one purposeful assessments nonetheless
cross and that nothing breaks—even if you happen to’re introducing a breaking change.
As soon as glad, you may commit the modifications and lift a pull request as
a part of your regular workflow.
Codemods Enhance Code High quality and Maintainability
Codemods aren’t simply helpful for managing breaking API modifications—they will
considerably enhance code high quality and maintainability. As codebases
evolve, they typically accumulate technical debt, together with outdated function
toggles, deprecated strategies, or tightly coupled parts. Manually
refactoring these areas may be time-consuming and error-prone.
By automating refactoring duties, codemods assist preserve your codebase clear
and freed from legacy patterns. Commonly making use of codemods permits you to
implement new coding requirements, take away unused code, and modernize your
codebase with out having to manually modify each file.