Whereas I’ve put React software, there is not such a factor as React software. I imply, there are
front-end functions written in JavaScript or TypeScript that occur to
use React as their views. Nonetheless, I feel it is not truthful to name them React
functions, simply as we would not name a Java EE software JSP
software.
Most of the time, folks squeeze various things into React
parts or hooks to make the applying work. One of these
less-organised construction is not an issue if the applying is small or
largely with out a lot enterprise logic. Nonetheless, as extra enterprise logic shifted
to front-end in lots of circumstances, this everything-in-component reveals issues. To
be extra particular, the hassle of understanding such kind of code is
comparatively excessive, in addition to the elevated threat to code modification.
On this article, I want to talk about a couple of patterns and strategies
you should use to reshape your “React software” into an everyday one, and solely
with React as its view (you may even swap these views into one other view
library with out an excessive amount of efforts).
The essential level right here is you must analyse what function every a part of the
code is enjoying inside an software (even on the floor, they could be
packed in the identical file). Separate view from no-view logic, break up the
no-view logic additional by their obligations and place them within the
proper locations.
The advantage of this separation is that it lets you make adjustments in
the underlying area logic with out worrying an excessive amount of in regards to the floor
views, or vice versa. Additionally, it may enhance the reusability of the area
logic somewhere else as they aren’t coupled to some other components.
React is a humble library for constructing views
It is simple to overlook that React, at its core, is a library (not a
framework) that helps you construct the person interface.
On this context, it’s emphasised that React is a JavaScript library
that concentrates on a selected facet of internet growth, specifically UI
parts, and affords ample freedom by way of the design of the
software and its total construction.
A JavaScript library for constructing person interfaces
It might sound fairly easy. However I’ve seen many circumstances the place
folks write the information fetching, reshaping logic proper within the place the place
it is consumed. For instance, fetching knowledge inside a React element, within the
useEffect
block proper above the rendering, or performing knowledge
mapping/reworking as soon as they received the response from the server facet.
useEffect(() => { fetch("https://deal with.service/api") .then((res) => res.json()) .then((knowledge) => { const addresses = knowledge.map((merchandise) => ({ avenue: merchandise.streetName, deal with: merchandise.streetAddress, postcode: merchandise.postCode, })); setAddresses(addresses); }); }, []); // the precise rendering...
Maybe as a result of there may be but to be a common commonplace within the frontend
world, or it is only a unhealthy programming behavior. Frontend functions ought to
not be handled too otherwise from common software program functions. Within the
frontend world, you continue to use separation of considerations basically to rearrange
the code construction. And all of the confirmed helpful design patterns nonetheless
apply.
Welcome to the true world React software
Most builders had been impressed by React’s simplicity and the concept
a person interface may be expressed as a pure perform to map knowledge into the
DOM. And to a sure extent, it IS.
However builders begin to wrestle when they should ship a community
request to a backend or carry out web page navigation, as these negative effects
make the element much less “pure”. And when you take into account these totally different
states (both international state or native state), issues rapidly get
sophisticated, and the darkish facet of the person interface emerges.
Aside from the person interface
React itself doesn’t care a lot about the place to place calculation or
enterprise logic, which is truthful because it’s solely a library for constructing person
interfaces. And past that view layer, a frontend software has different
components as effectively. To make the applying work, you have to a router,
native storage, cache at totally different ranges, community requests, Third-party
integrations, Third-party login, safety, logging, efficiency tuning,
and so forth.
With all this additional context, attempting to squeeze the whole lot into
React parts or hooks is usually not a good suggestion. The reason being
mixing ideas in a single place usually results in extra confusion. At
first, the element units up some community request for order standing, and
then there may be some logic to trim off main house from a string and
then navigate someplace else. The reader should consistently reset their
logic circulation and bounce forwards and backwards from totally different ranges of particulars.
Packing all of the code into parts may match in small functions
like a Todo or one-form software. Nonetheless, the efforts to grasp
such software can be important as soon as it reaches a sure degree.
To not point out including new options or fixing current defects.
If we may separate totally different considerations into information or folders with
buildings, the psychological load required to grasp the applying would
be considerably diminished. And also you solely need to deal with one factor at a
time. Fortunately, there are already some well-proven patterns again to the
pre-web time. These design rules and patterns are explored and
mentioned effectively to resolve the widespread person interface issues – however within the
desktop GUI software context.
Martin Fowler has an awesome abstract of the idea of view-model-data
layering.
On the entire I’ve discovered this to be an efficient type of
modularization for a lot of functions and one which I usually use and
encourage. It is largest benefit is that it permits me to extend my
focus by permitting me to consider the three matters (i.e., view,
mannequin, knowledge) comparatively independently.
Layered architectures have been used to manage the challenges in massive
GUI functions, and positively we will use these established patterns of
front-end group in our “React functions”.
The evolution of a React software
For small or one-off initiatives, you would possibly discover that every one logic is simply
written inside React parts. You might even see one or only some parts
in whole. The code seems just about like HTML, with just some variable or
state used to make the web page “dynamic”. Some would possibly ship requests to fetch
knowledge on useEffect
after the parts render.
As the applying grows, and increasingly code are added to codebase.
And not using a correct strategy to organise them, quickly the codebase will flip into
unmaintainable state, which means that even including small options may be
time-consuming as builders want extra time to learn the code.
So I’ll checklist a couple of steps that may assist to aid the maintainable
downside. It usually require a bit extra efforts, however it is going to repay to
have the construction in you software. Let’s have a fast assessment of those
steps to construct front-end functions that scale.
Single Part Software
It may be referred to as just about a Single Part Software:
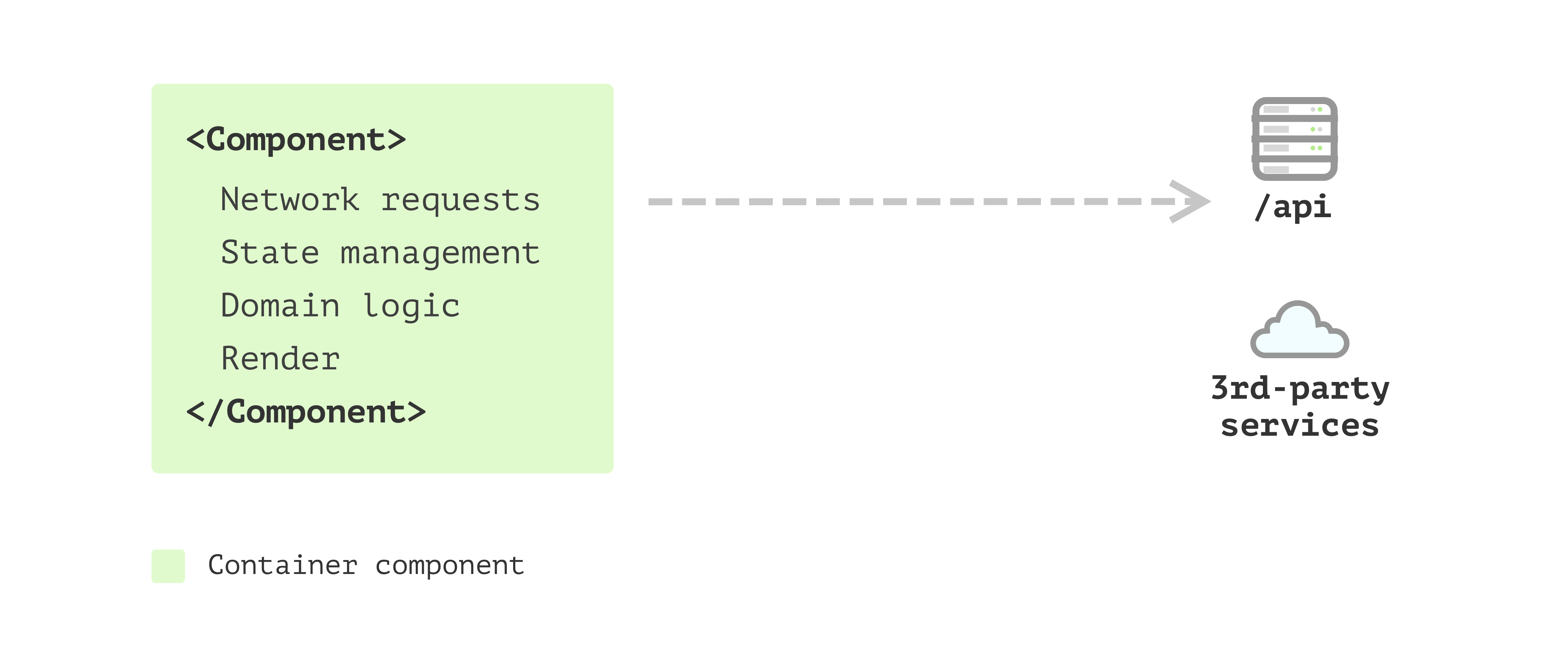
Determine 1: Single Part Software
However quickly, you realise one single element requires a variety of time
simply to learn what’s going on. For instance, there may be logic to iterate
by way of an inventory and generate every merchandise. Additionally, there may be some logic for
utilizing Third-party parts with only some configuration code, aside
from different logic.
A number of Part Software
You determined to separate the element into a number of parts, with
these buildings reflecting what’s taking place on the consequence HTML is a
good thought, and it lets you deal with one element at a time.
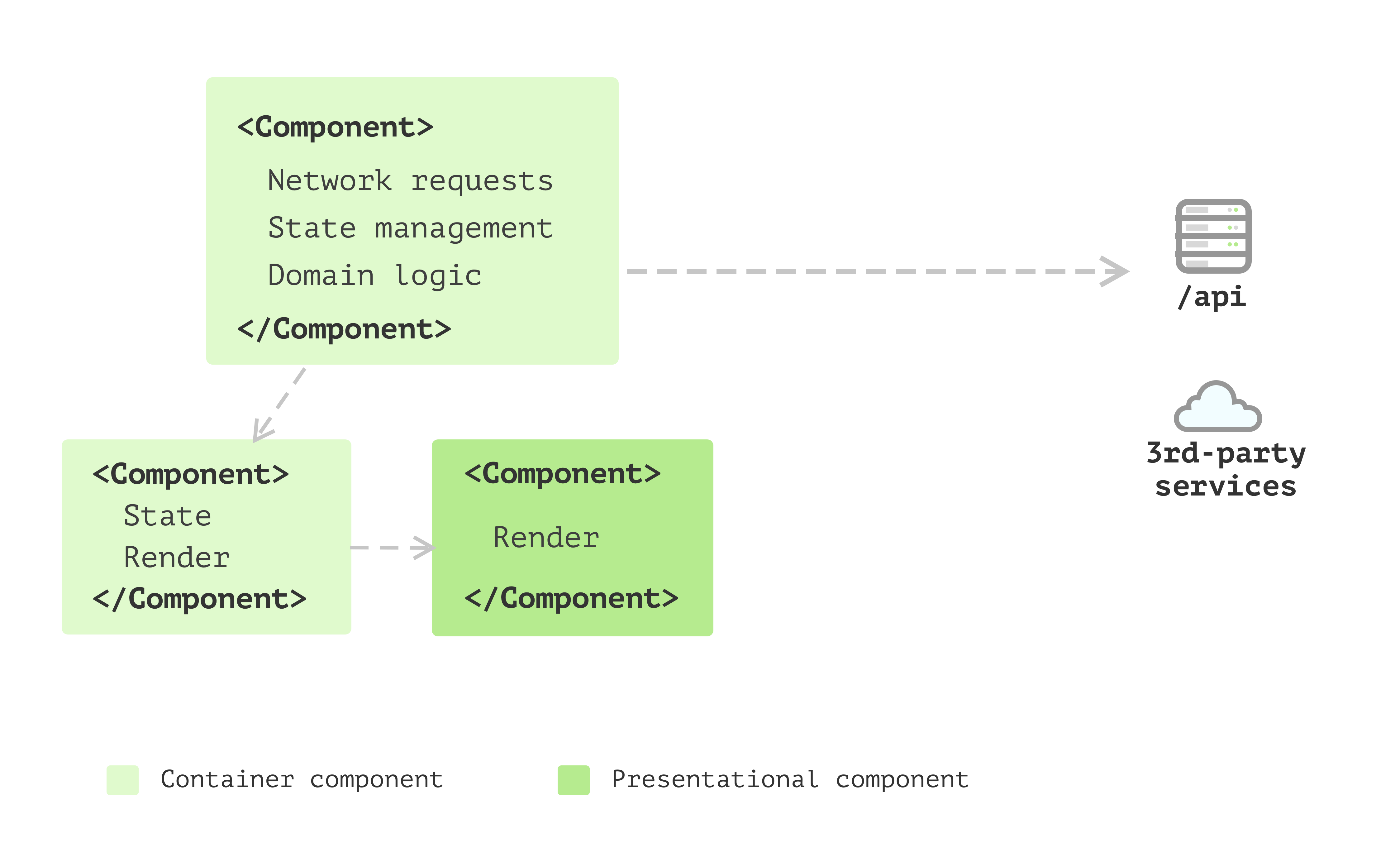
Determine 2: A number of Part Software
And as your software grows, other than the view, there are issues
like sending community requests, changing knowledge into totally different shapes for
the view to eat, and gathering knowledge to ship again to the server. And
having this code inside parts doesn’t really feel proper as they’re not
actually about person interfaces. Additionally, some parts have too many
inside states.
State administration with hooks
It’s a greater thought to separate this logic right into a separate locations.
Fortunately in React, you may outline your personal hooks. This can be a nice strategy to
share these state and the logic of every time states change.
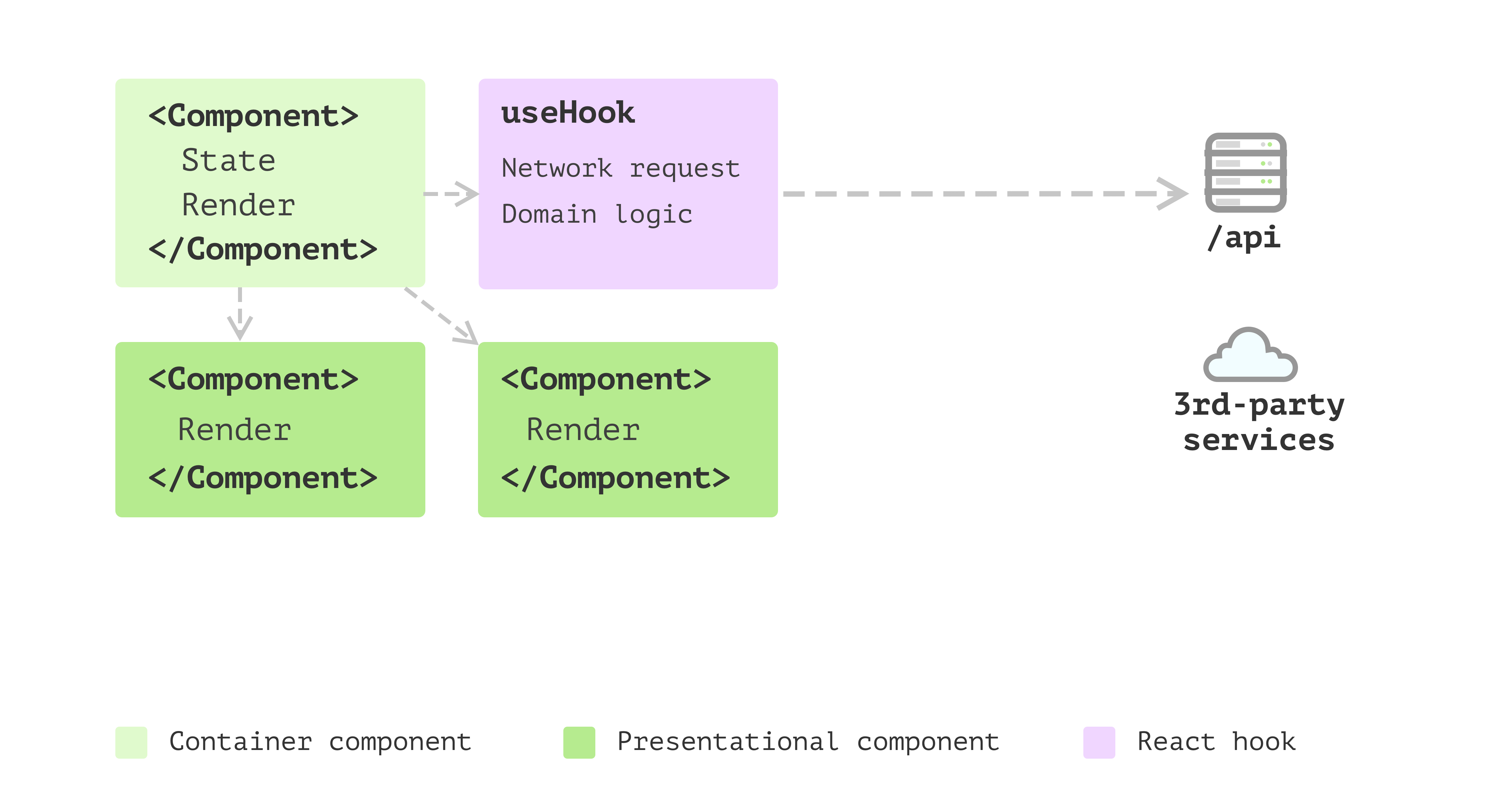
Determine 3: State administration with hooks
That’s superior! You’ve gotten a bunch of components extracted out of your
single element software, and you’ve got a couple of pure presentational
parts and a few reusable hooks that make different parts stateful.
The one downside is that in hooks, other than the facet impact and state
administration, some logic doesn’t appear to belong to the state administration
however pure calculations.
Enterprise fashions emerged
So that you’ve began to change into conscious that extracting this logic into but
one other place can convey you a lot advantages. For instance, with that break up,
the logic may be cohesive and impartial of any views. Then you definately extract
a couple of area objects.
These easy objects can deal with knowledge mapping (from one format to
one other), test nulls and use fallback values as required. Additionally, because the
quantity of those area objects grows, you discover you want some inheritance
or polymorphism to make issues even cleaner. Thus you utilized many
design patterns you discovered useful from different locations into the front-end
software right here.
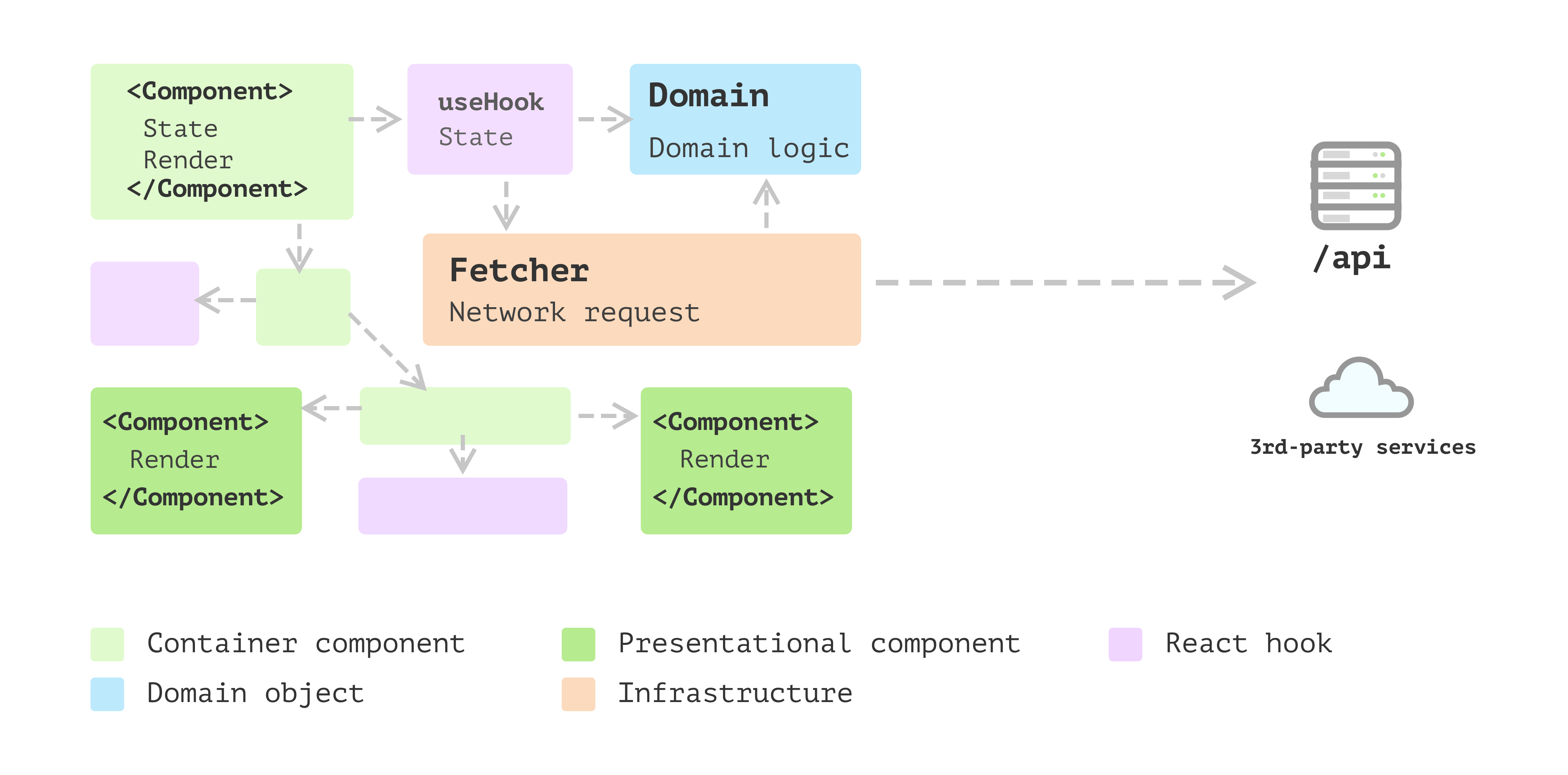
Determine 4: Enterprise fashions
Layered frontend software
The appliance retains evolving, and then you definately discover some patterns
emerge. There are a bunch of objects that don’t belong to any person
interface, they usually additionally don’t care about whether or not the underlying knowledge is
from distant service, native storage or cache. After which, you need to break up
them into totally different layers. Here’s a detailed clarification in regards to the layer
splitting Presentation Area Knowledge Layering.
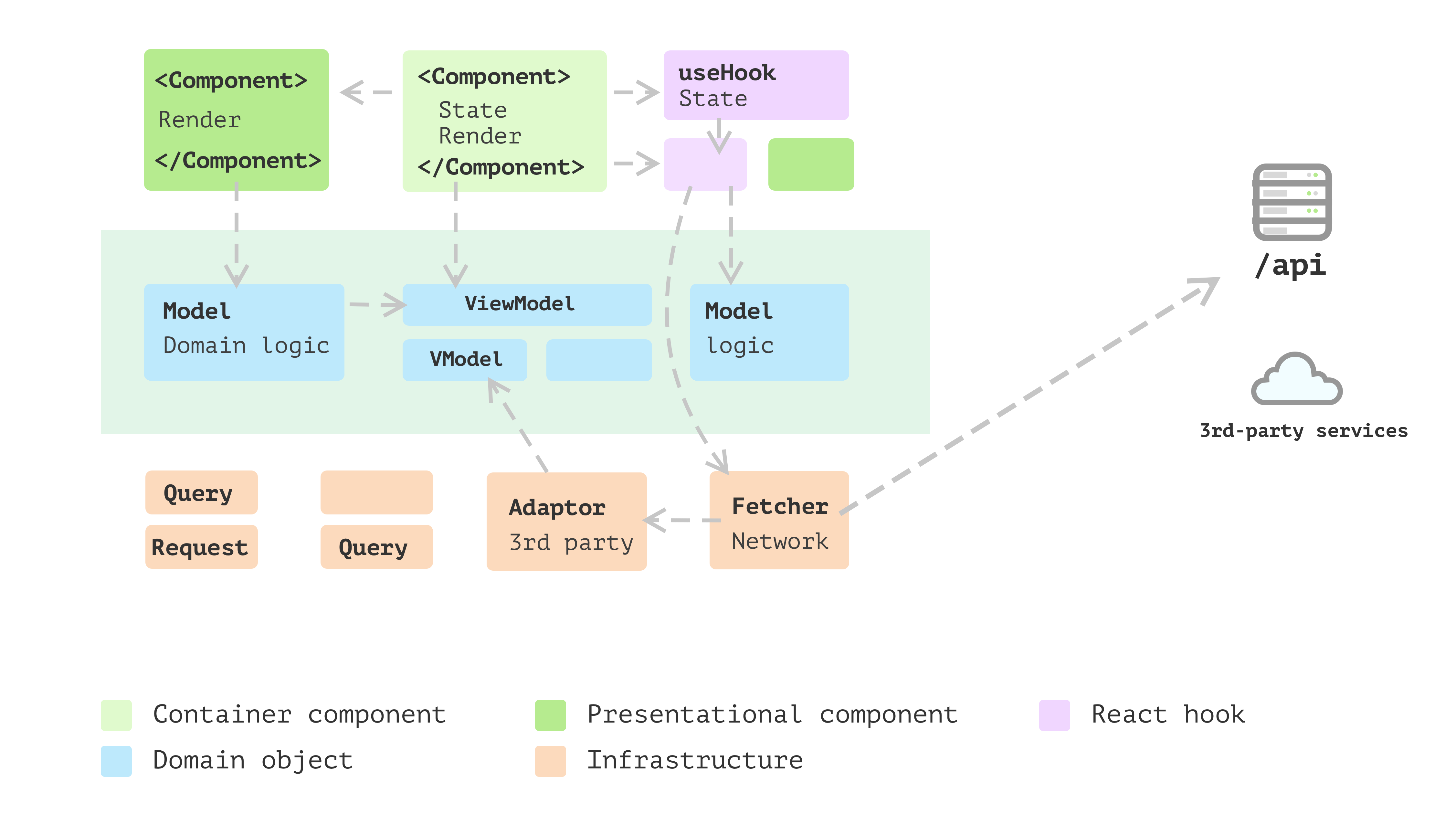
Determine 5: Layered frontend software
The above evolution course of is a high-level overview, and you must
have a style of how you must construction your code or at the least what the
path ought to be. Nonetheless, there can be many particulars it is advisable to
take into account earlier than making use of the speculation in your software.
Within the following sections, I’ll stroll you thru a characteristic I
extracted from an actual mission to show all of the patterns and design
rules I feel helpful for giant frontend functions.
Introduction of the Fee characteristic
I’m utilizing an oversimplified on-line ordering software as a beginning
level. On this software, a buyer can decide up some merchandise and add
them to the order, after which they might want to choose one of many cost
strategies to proceed.
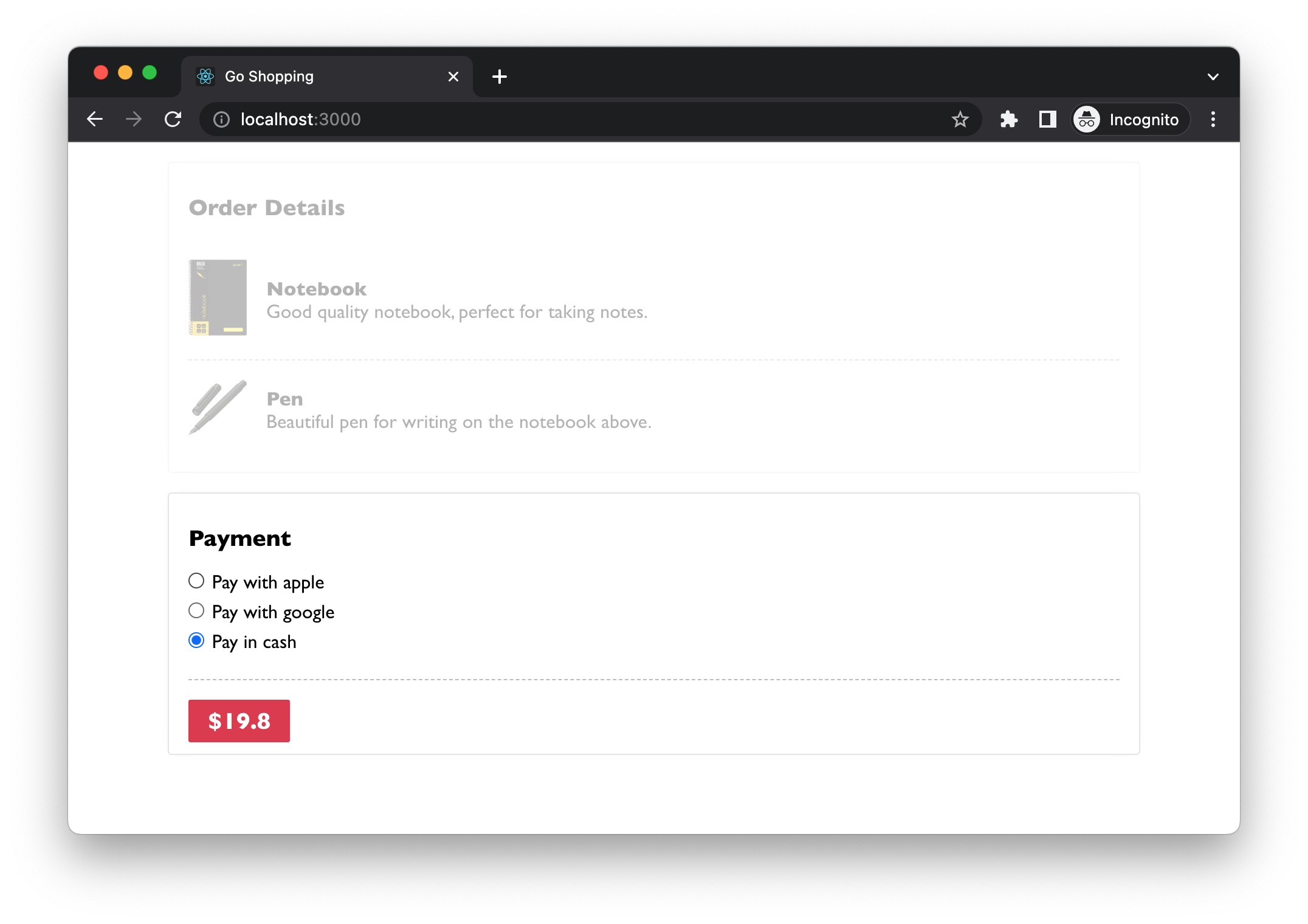
Determine 6: Fee part
These cost methodology choices are configured on the server facet, and
clients from totally different international locations might even see different choices. For instance,
Apple Pay might solely be fashionable in some international locations. The radio buttons are
data-driven – no matter is fetched from the backend service can be
surfaced. The one exception is that when no configured cost strategies
are returned, we don’t present something and deal with it as “pay in money” by
default.
For simplicity, I’ll skip the precise cost course of and deal with the
Fee
element. Let’s say that after studying the React hiya world
doc and a few stackoverflow searches, you got here up with some code
like this:
src/Fee.tsx…
export const Fee = ({ quantity }: { quantity: quantity }) => { const [paymentMethods, setPaymentMethods] = useState<LocalPaymentMethod[]>( [] ); useEffect(() => { const fetchPaymentMethods = async () => { const url = "https://online-ordering.com/api/payment-methods"; const response = await fetch(url); const strategies: RemotePaymentMethod[] = await response.json(); if (strategies.size > 0) { const prolonged: LocalPaymentMethod[] = strategies.map((methodology) => ({ supplier: methodology.identify, label: `Pay with ${methodology.identify}`, })); prolonged.push({ supplier: "money", label: "Pay in money" }); setPaymentMethods(prolonged); } else { setPaymentMethods([]); } }; fetchPaymentMethods(); }, []); return ( <div> <h3>Fee</h3> <div> {paymentMethods.map((methodology) => ( <label key={methodology.supplier}> <enter kind="radio" identify="cost" worth={methodology.supplier} defaultChecked={methodology.supplier === "money"} /> <span>{methodology.label}</span> </label> ))} </div> <button>${quantity}</button> </div> ); };
The code above is fairly typical. You might need seen it within the get
began tutorial someplace. And it is not vital unhealthy. Nonetheless, as we
talked about above, the code has blended totally different considerations all in a single
element and makes it a bit troublesome to learn.
The issue with the preliminary implementation
The primary subject I want to deal with is how busy the element
is. By that, I imply Fee
offers with various things and makes the
code troublesome to learn as you must change context in your head as you
learn.
With a view to make any adjustments you must comprehend
easy methods to initialise community request
,
easy methods to map the information to a neighborhood format that the element can perceive
,
easy methods to render every cost methodology
,
and
the rendering logic for Fee
element itself
.
src/Fee.tsx…
export const Fee = ({ quantity }: { quantity: quantity }) => { const [paymentMethods, setPaymentMethods] = useState<LocalPaymentMethod[]>( [] ); useEffect(() => { const fetchPaymentMethods = async () => { const url = "https://online-ordering.com/api/payment-methods"; const response = await fetch(url); const strategies: RemotePaymentMethod[] = await response.json(); if (strategies.size > 0) { const prolonged: LocalPaymentMethod[] = strategies.map((methodology) => ({ supplier: methodology.identify, label: `Pay with ${methodology.identify}`, })); prolonged.push({ supplier: "money", label: "Pay in money" }); setPaymentMethods(prolonged); } else { setPaymentMethods([]); } }; fetchPaymentMethods(); }, []); return ( <div> <h3>Fee</h3> <div> {paymentMethods.map((methodology) => ( <label key={methodology.supplier}> <enter kind="radio" identify="cost" worth={methodology.supplier} defaultChecked={methodology.supplier === "money"} /> <span>{methodology.label}</span> </label> ))} </div> <button>${quantity}</button> </div> ); };
It is not a giant downside at this stage for this easy instance.
Nonetheless, because the code will get greater and extra advanced, we’ll have to
refactoring them a bit.
It’s good follow to separate view and non-view code into separate
locations. The reason being, basically, views are altering extra continuously than
non-view logic. Additionally, as they take care of totally different facets of the
software, separating them lets you deal with a selected
self-contained module that’s way more manageable when implementing new
options.
The break up of view and non-view code
In React, we will use a customized hook to take care of state of a element
whereas holding the element itself kind of stateless. We will
use
to create a perform referred to as usePaymentMethods
(the
prefix use
is a conference in React to point the perform is a hook
and dealing with some states in it):
src/Fee.tsx…
const usePaymentMethods = () => {
const [paymentMethods, setPaymentMethods] = useState<LocalPaymentMethod[]>(
[]
);
useEffect(() => {
const fetchPaymentMethods = async () => {
const url = "https://online-ordering.com/api/payment-methods";
const response = await fetch(url);
const strategies: RemotePaymentMethod[] = await response.json();
if (strategies.size > 0) {
const prolonged: LocalPaymentMethod[] = strategies.map((methodology) => ({
supplier: methodology.identify,
label: `Pay with ${methodology.identify}`,
}));
prolonged.push({ supplier: "money", label: "Pay in money" });
setPaymentMethods(prolonged);
} else {
setPaymentMethods([]);
}
};
fetchPaymentMethods();
}, []);
return {
paymentMethods,
};
};
This returns a paymentMethods
array (in kind LocalPaymentMethod
) as
inside state and is prepared for use in rendering. So the logic in
Fee
may be simplified as:
src/Fee.tsx…
export const Fee = ({ quantity }: { quantity: quantity }) => {
const { paymentMethods } = usePaymentMethods();
return (
<div>
<h3>Fee</h3>
<div>
{paymentMethods.map((methodology) => (
<label key={methodology.supplier}>
<enter
kind="radio"
identify="cost"
worth={methodology.supplier}
defaultChecked={methodology.supplier === "money"}
/>
<span>{methodology.label}</span>
</label>
))}
</div>
<button>${quantity}</button>
</div>
);
};
This helps relieve the ache within the Fee
element. Nonetheless, in case you
have a look at the block for iterating by way of paymentMethods
, it appears a
idea is lacking right here. In different phrases, this block deserves its personal
element. Ideally, we would like every element to deal with, just one
factor.
Knowledge modelling to encapsulate logic
Up to now, the adjustments we’ve made are all about splitting view and
non-view code into totally different locations. It really works effectively. The hook handles knowledge
fetching and reshaping. Each Fee
and PaymentMethods
are comparatively
small and straightforward to grasp.
Nonetheless, in case you look carefully, there may be nonetheless room for enchancment. To
begin with, within the pure perform element PaymentMethods
, we’ve a bit
of logic to test if a cost methodology ought to be checked by default:
src/Fee.tsx…
const PaymentMethods = ({
paymentMethods,
}: {
paymentMethods: LocalPaymentMethod[];
}) => (
<>
{paymentMethods.map((methodology) => (
<label key={methodology.supplier}>
<enter
kind="radio"
identify="cost"
worth={methodology.supplier}
defaultChecked={methodology.supplier === "money"}
/>
<span>{methodology.label}</span>
</label>
))}
</>
);
These take a look at statements in a view may be thought of a logic leak, and
progressively they are often scatted elsewhere and make modification
more durable.
One other level of potential logic leakage is within the knowledge conversion
the place we fetch knowledge:
src/Fee.tsx…
const usePaymentMethods = () => { const [paymentMethods, setPaymentMethods] = useState<LocalPaymentMethod[]>( [] ); useEffect(() => { const fetchPaymentMethods = async () => { const url = "https://online-ordering.com/api/payment-methods"; const response = await fetch(url); const strategies: RemotePaymentMethod[] = await response.json(); if (strategies.size > 0) { const prolonged: LocalPaymentMethod[] = strategies.map((methodology) => ({ supplier: methodology.identify, label: `Pay with ${methodology.identify}`, })); prolonged.push({ supplier: "money", label: "Pay in money" }); setPaymentMethods(prolonged); } else { setPaymentMethods([]); } }; fetchPaymentMethods(); }, []); return { paymentMethods, }; };
Be aware the nameless perform inside strategies.map
does the conversion
silently, and this logic, together with the methodology.supplier === "money"
above may be extracted into a category.
We may have a category PaymentMethod
with the information and behavior
centralised right into a single place:
src/PaymentMethod.ts…
class PaymentMethod {
personal remotePaymentMethod: RemotePaymentMethod;
constructor(remotePaymentMethod: RemotePaymentMethod) {
this.remotePaymentMethod = remotePaymentMethod;
}
get supplier() {
return this.remotePaymentMethod.identify;
}
get label() {
if(this.supplier === 'money') {
return `Pay in ${this.supplier}`
}
return `Pay with ${this.supplier}`;
}
get isDefaultMethod() {
return this.supplier === "money";
}
}
With the category, I can outline the default money cost methodology:
const payInCash = new PaymentMethod({ identify: "money" });
And through the conversion – after the cost strategies are fetched from
the distant service – I can assemble the PaymentMethod
object in-place. And even
extract a small perform referred to as convertPaymentMethods
:
src/usePaymentMethods.ts…
const convertPaymentMethods = (strategies: RemotePaymentMethod[]) => {
if (strategies.size === 0) {
return [];
}
const prolonged: PaymentMethod[] = strategies.map(
(methodology) => new PaymentMethod(methodology)
);
prolonged.push(payInCash);
return prolonged;
};
Additionally, within the PaymentMethods
element, we don’t use the
methodology.supplier === "money"
to test anymore, and as an alternative name the
getter
:
src/PaymentMethods.tsx…
export const PaymentMethods = ({ choices }: { choices: PaymentMethod[] }) => (
<>
{choices.map((methodology) => (
<label key={methodology.supplier}>
<enter
kind="radio"
identify="cost"
worth={methodology.supplier}
defaultChecked={methodology.isDefaultMethod}
/>
<span>{methodology.label}</span>
</label>
))}
</>
);
Now we’re restructuring our Fee
element right into a bunch of smaller
components that work collectively to complete the work.
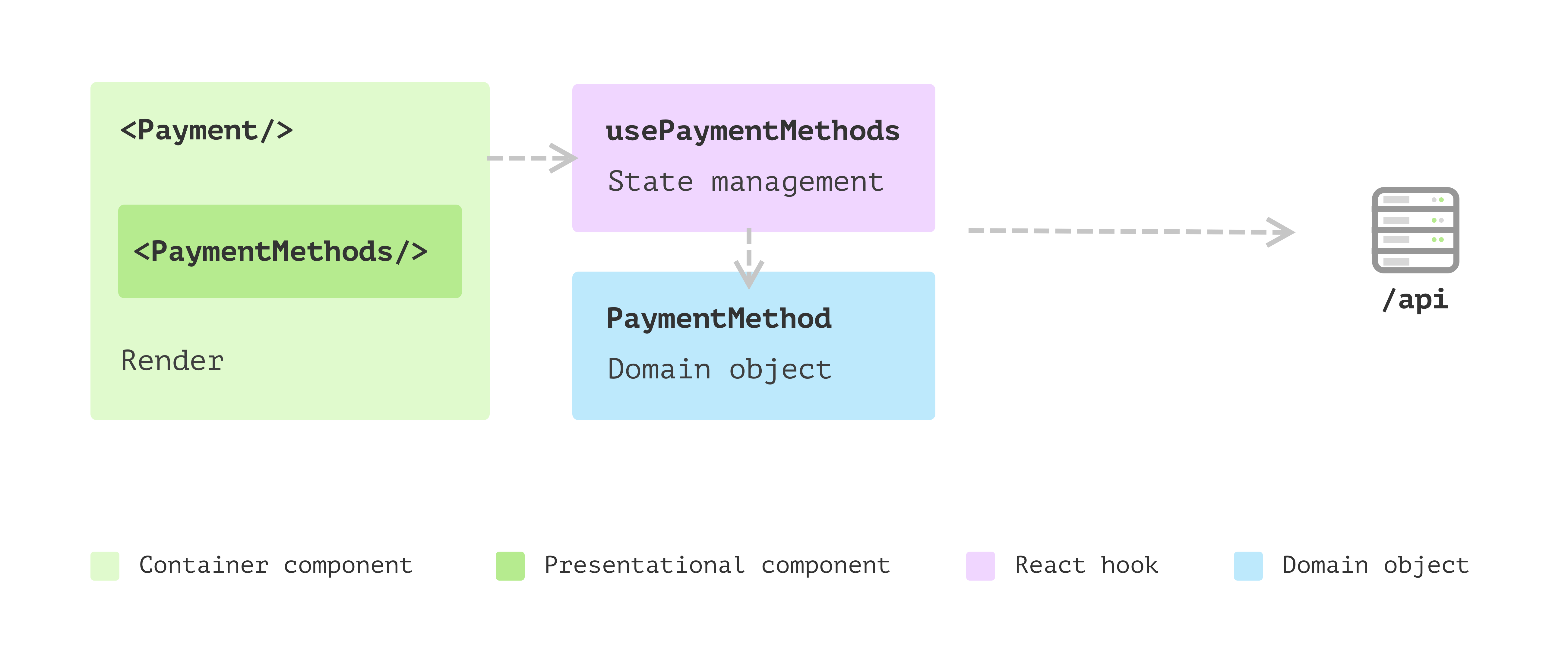
Determine 7: Refactored Fee with extra components that may be composed simply
The advantages of the brand new construction
- Having a category encapsulates all of the logic round a cost methodology. It’s a
area object and doesn’t have any UI-related info. So testing and
doubtlessly modifying logic right here is far simpler than when embedded in a
view. - The brand new extracted element
PaymentMethods
is a pure perform and solely
relies on a site object array, which makes it tremendous simple to check and reuse
elsewhere. We would have to cross in aonSelect
callback to it, however even in
that case, it’s a pure perform and doesn’t have to the touch any exterior
states. - Every a part of the characteristic is obvious. If a brand new requirement comes, we will
navigate to the appropriate place with out studying all of the code.
I’ve to make the instance on this article sufficiently advanced in order that
many patterns may be extracted. All these patterns and rules are
there to assist simplify our code’s modifications.
New requirement: donate to a charity
Let’s look at the speculation right here with some additional adjustments to the
software. The brand new requirement is that we need to supply an possibility for
clients to donate a small amount of cash as a tip to a charity alongside
with their order.
For instance, if the order quantity is $19.80, we ask if they want
to donate $0.20. And if a person agrees to donate it, we’ll present the full
quantity on the button.
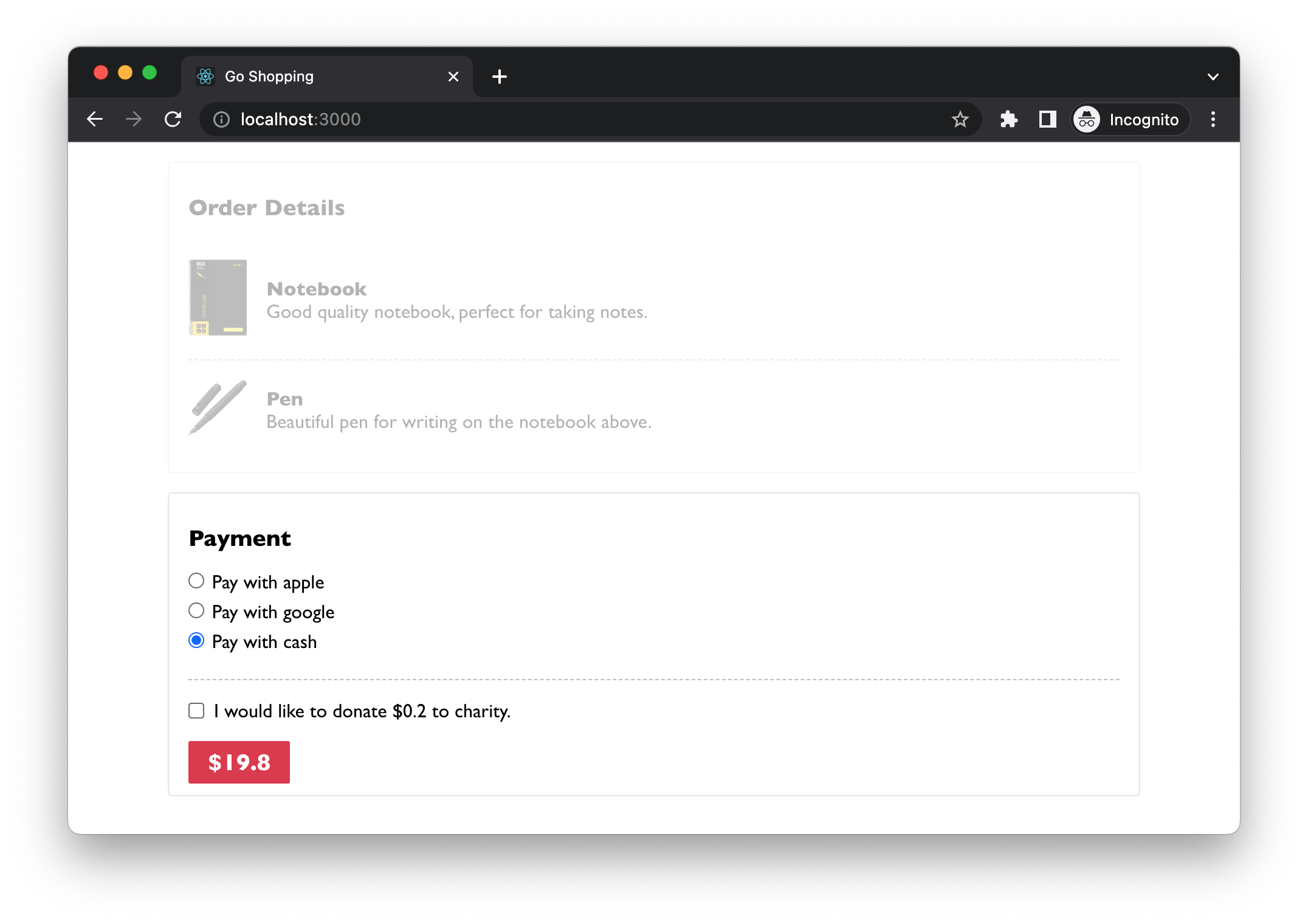
Determine 8: Donate to a charity
Earlier than we make any adjustments, let’s have a fast have a look at the present code
construction. I want have totally different components of their folder so it is simple for
me to navigate when it grows greater.
src ├── App.tsx ├── parts │ ├── Fee.tsx │ └── PaymentMethods.tsx ├── hooks │ └── usePaymentMethods.ts ├── fashions │ └── PaymentMethod.ts └── sorts.ts
App.tsx
is the primary entry, it makes use of Fee
element, and Fee
makes use of PaymentMethods
for rendering totally different cost choices. The hook
usePaymentMethods
is chargeable for fetching knowledge from distant service
after which convert it to a PaymentMethod
area object that’s used to
maintain label
and the isDefaultChecked
flag.
Inside state: comply with donation
To make these adjustments in Fee
, we want a boolean state
agreeToDonate
to point whether or not a person chosen the checkbox on the
web page.
src/Fee.tsx…
const [agreeToDonate, setAgreeToDonate] = useState<boolean>(false); const { whole, tip } = useMemo( () => ({ whole: agreeToDonate ? Math.ground(quantity + 1) : quantity, tip: parseFloat((Math.ground(quantity + 1) - quantity).toPrecision(10)), }), [amount, agreeToDonate] );
The perform Math.ground
will around the quantity down so we will get the
right amount when the person selects agreeToDonate
, and the distinction
between the rounded-up worth and the unique quantity can be assigned to tip
.
And for the view, the JSX can be a checkbox plus a brief
description:
src/Fee.tsx…
return ( <div> <h3>Fee</h3> <PaymentMethods choices={paymentMethods} /> <div> <label> <enter kind="checkbox" onChange={handleChange} checked={agreeToDonate} /> <p> {agreeToDonate ? "Thanks in your donation." : `I want to donate $${tip} to charity.`} </p> </label> </div> <button>${whole}</button> </div> );
With these new adjustments, our code begins dealing with a number of issues once more.
It’s important to remain alert for potential mixing of view and non-view
code. If you happen to discover any pointless mixing, search for methods to separate them.
Be aware that it is not a set-in-stone rule. Preserve issues all collectively good
and tidy for small and cohesive parts, so you do not have to look in
a number of locations to grasp the general behaviour. Typically, you must
remember to keep away from the element file rising too huge to grasp.
Extra adjustments about round-up logic
The round-up seems good to this point, and because the enterprise expands to different
international locations, it comes with new necessities. The identical logic doesn’t work in
Japan market as 0.1 Yen is just too small as a donation, and it must spherical
as much as the closest hundred for the Japanese foreign money. And for Denmark, it
must spherical as much as the closest tens.
It appears like a straightforward repair. All I want is a countryCode
handed into
the Fee
element, proper?
<Fee quantity={3312} countryCode="JP" />;
And since all the logic is now outlined within the useRoundUp
hook, I
may also cross the countryCode
by way of to the hook.
const useRoundUp = (quantity: quantity, countryCode: string) => { //... const { whole, tip } = useMemo( () => ({ whole: agreeToDonate ? countryCode === "JP" ? Math.ground(quantity / 100 + 1) * 100 : Math.ground(quantity + 1) : quantity, //... }), [amount, agreeToDonate, countryCode] ); //... };
You’ll discover that the if-else can go on and on as a brand new
countryCode
is added within the useEffect
block. And for the
getTipMessage
, we want the identical if-else checks as a special nation
might use different foreign money signal (as an alternative of a greenback signal by default):
const formatCheckboxLabel = ( agreeToDonate: boolean, tip: quantity, countryCode: string ) => { const currencySign = countryCode === "JP" ? "¥" : "$"; return agreeToDonate ? "Thanks in your donation." : `I want to donate ${currencySign}${tip} to charity.`; };
One final thing we additionally want to alter is the foreign money signal on the
button:
<button> {countryCode === "JP" ? "¥" : "$"} {whole} </button>;
The shotgun surgical procedure downside
This situation is the well-known “shotgun surgical procedure” odor we see in
many locations (not notably in React functions). This basically
says that we’ll have to the touch a number of modules every time we have to modify
the code for both a bug fixing or including a brand new characteristic. And certainly, it’s
simpler to make errors with this many adjustments, particularly when your checks
are inadequate.
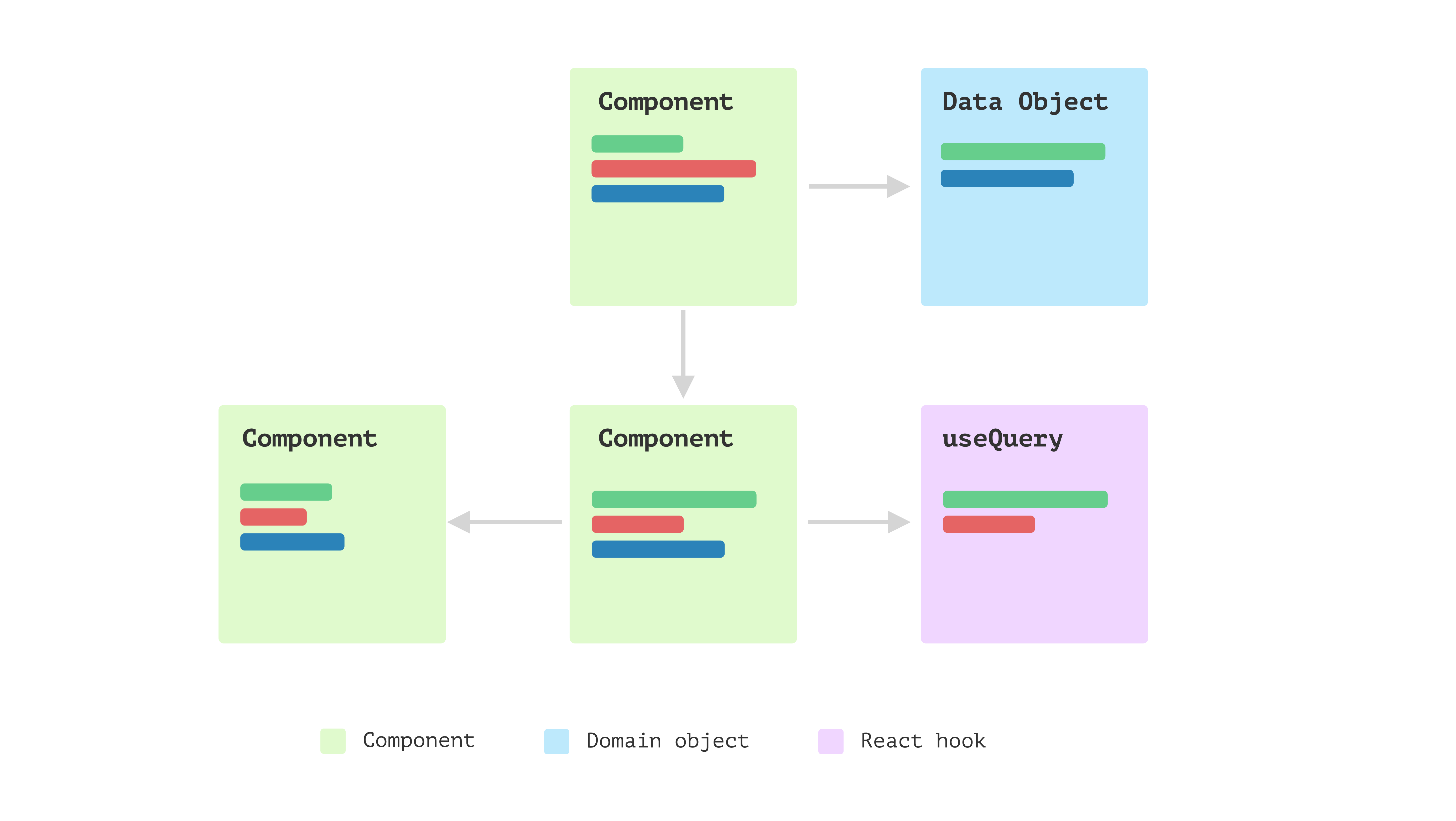
Determine 10: The shotgun surgical procedure odor
As illustrated above, the colored strains point out branches of nation
code checks that cross many information. In views, we’ll have to do separate
issues for various nation code, whereas in hooks, we’ll want related
branches. And every time we have to add a brand new nation code, we’ll need to
contact all these components.
For instance, if we take into account Denmark as a brand new nation the enterprise is
increasing to, we’ll find yourself with code in lots of locations like:
const currencySignMap = { JP: "¥", DK: "Kr.", AU: "$", }; const getCurrencySign = (countryCode: CountryCode) => currencySignMap[countryCode];
One potential resolution for the issue of getting branches scattered in
totally different locations is to make use of polymorphism to switch these change circumstances or
desk look-up logic. We will use Extract Class on these
properties after which Substitute Conditional with Polymorphism.
Polymorphism to the rescue
The very first thing we will do is look at all of the variations to see what
should be extracted into a category. For instance, totally different international locations have
totally different foreign money indicators, so getCurrencySign
may be extracted right into a
public interface. Additionally ,international locations might need totally different round-up
algorithms, thus getRoundUpAmount
and getTip
can go to the
interface.
export interface PaymentStrategy { getRoundUpAmount(quantity: quantity): quantity; getTip(quantity: quantity): quantity; }
A concrete implementation of the technique interface could be like
following the code snippet: PaymentStrategyAU
.
export class PaymentStrategyAU implements PaymentStrategy {
get currencySign(): string {
return "$";
}
getRoundUpAmount(quantity: quantity): quantity {
return Math.ground(quantity + 1);
}
getTip(quantity: quantity): quantity {
return parseFloat((this.getRoundUpAmount(quantity) - quantity).toPrecision(10));
}
}
Be aware right here the interface and courses don’t have anything to do with the UI
straight. This logic may be shared somewhere else within the software or
even moved to backend providers (if the backend is written in Node, for
instance).
We may have subclasses for every nation, and every has the nation particular
round-up logic. Nonetheless, as perform is first-class citizen in JavaScript, we
can cross within the round-up algorithm into the technique implementation to make the
code much less overhead with out subclasses. And becaues we’ve just one
implementation of the interface, we will use Inline Class to
cut back the single-implementation-interface.
src/fashions/CountryPayment.ts…
export class CountryPayment {
personal readonly _currencySign: string;
personal readonly algorithm: RoundUpStrategy;
public constructor(currencySign: string, roundUpAlgorithm: RoundUpStrategy) {
this._currencySign = currencySign;
this.algorithm = roundUpAlgorithm;
}
get currencySign(): string {
return this._currencySign;
}
getRoundUpAmount(quantity: quantity): quantity {
return this.algorithm(quantity);
}
getTip(quantity: quantity): quantity {
return calculateTipFor(this.getRoundUpAmount.bind(this))(quantity);
}
}
As illustrated under, as an alternative of rely upon scattered logic in
parts and hooks, they now solely depend on a single class
PaymentStrategy
. And at runtime, we will simply substitute one occasion
of PaymentStrategy
for one more (the pink, inexperienced and blue sq. signifies
totally different situations of PaymentStrategy
class).
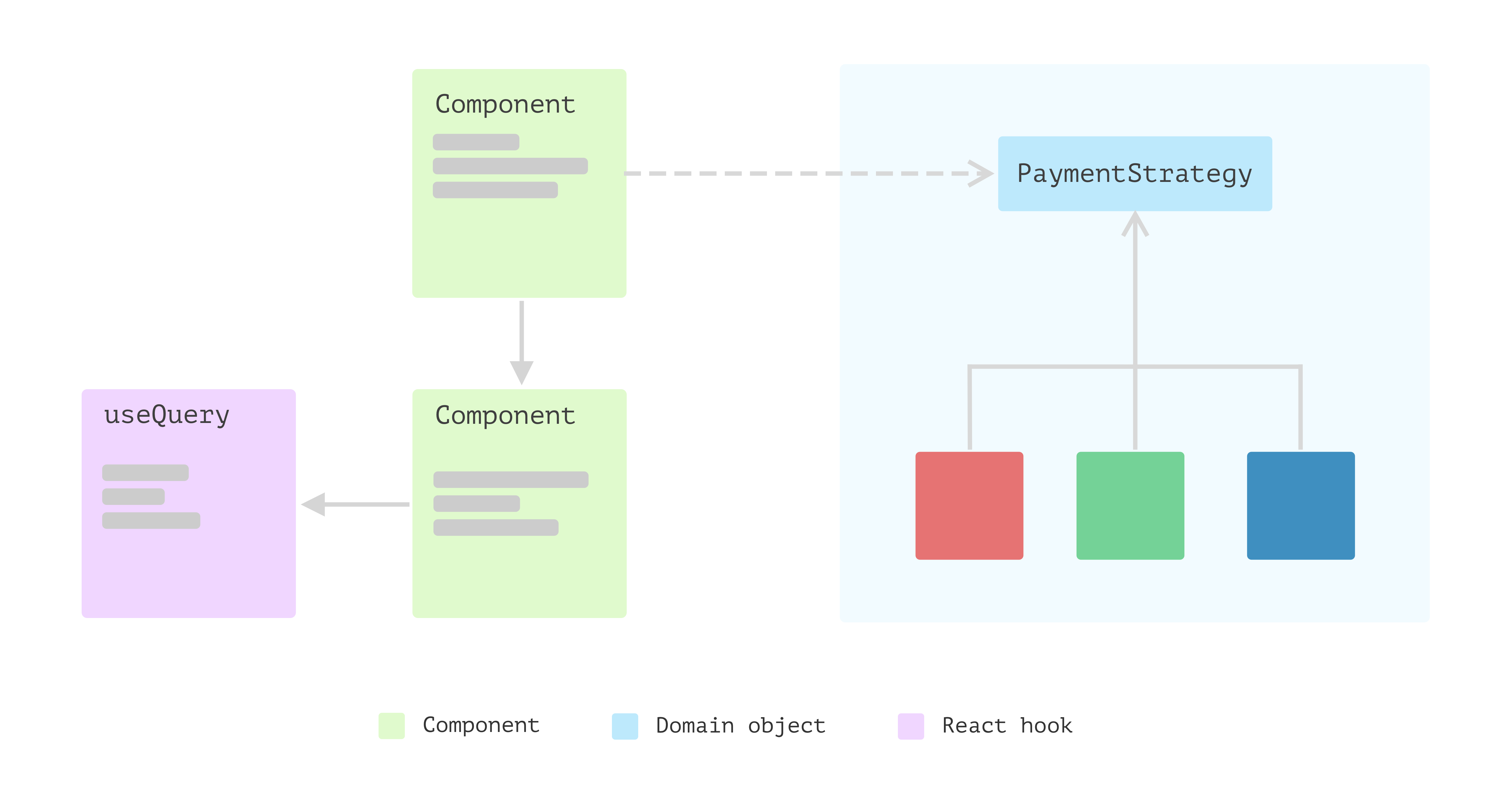
Determine 11: Extract class to encapsulate logic
And the useRoundUp
hook, the code could possibly be simplified as:
src/hooks/useRoundUp.ts…
export const useRoundUp = (quantity: quantity, technique: PaymentStrategy) => { const [agreeToDonate, setAgreeToDonate] = useState<boolean>(false); const { whole, tip } = useMemo( () => ({ whole: agreeToDonate ? technique.getRoundUpAmount(quantity) : quantity, tip: technique.getTip(quantity), }), [agreeToDonate, amount, strategy] ); const updateAgreeToDonate = () => { setAgreeToDonate((agreeToDonate) => !agreeToDonate); }; return { whole, tip, agreeToDonate, updateAgreeToDonate, }; };
Within the Fee
element, we cross the technique from props
by way of
to the hook:
src/parts/Fee.tsx…
export const Fee = ({ quantity, technique = new PaymentStrategy("$", roundUpToNearestInteger), }: { quantity: quantity; technique?: PaymentStrategy; }) => { const { paymentMethods } = usePaymentMethods(); const { whole, tip, agreeToDonate, updateAgreeToDonate } = useRoundUp( quantity, technique ); return ( <div> <h3>Fee</h3> <PaymentMethods choices={paymentMethods} /> <DonationCheckbox onChange={updateAgreeToDonate} checked={agreeToDonate} content material={formatCheckboxLabel(agreeToDonate, tip, technique)} /> <button>{formatButtonLabel(technique, whole)}</button> </div> ); };
And I then did a bit clear as much as extract a couple of helper features for
producing the labels:
src/utils.ts…
export const formatCheckboxLabel = ( agreeToDonate: boolean, tip: quantity, technique: CountryPayment ) => { return agreeToDonate ? "Thanks in your donation." : `I want to donate ${technique.currencySign}${tip} to charity.`; };
I hope you may have seen that we’re attempting to straight extract non-view
code into separate locations or summary new mechanisms to reform it to be
extra modular.
You’ll be able to consider it this fashion: the React view is barely one of many
shoppers of your non-view code. For instance, in case you would construct a brand new
interface – perhaps with Vue or perhaps a command line software – how a lot code
are you able to reuse along with your present implementation?
The advantages of getting these layers
As demonstrated above, these layers brings us many benefits:
- Enhanced maintainability: by separating a element into distinct components,
it’s simpler to find and repair defects in particular components of the code. This could
save time and cut back the danger of introducing new bugs whereas making adjustments. - Elevated modularity: the layered construction is extra modular, which might
make it simpler to reuse code and construct new options. Even in every layer, take
views for instance, are usually extra composable. - Enhanced readability: it is a lot simpler to grasp and observe the logic
of the code. This may be particularly useful for different builders who’re studying
and dealing with the code. That is the core of constructing adjustments to the
codebase. - Improved scalability: with diminished complixity in every particular person module,
the applying is commonly extra scalable, as it’s simpler so as to add new options or
make adjustments with out affecting all the system. This may be particularly
vital for giant, advanced functions which might be anticipated to evolve over
time. - Migrate to different techstack: if we’ve to (even impossible in most
initiatives), we will exchange the view layer with out altering the underlying fashions
and logic. All as a result of the area logic is encapsulated in pure JavaScript (or
TypeScript) code and is not conscious of the existence of views.
Conclusion
Constructing React software, or a frontend software with React as its
view, shouldn’t be handled as a brand new kind of software program. A lot of the patterns
and rules for constructing the standard person interface nonetheless apply. Even
the patterns for setting up a headless service within the backend are additionally
legitimate within the frontend discipline. We will use layers within the frontend and have the
person interface as skinny as potential, sink the logic right into a supporting mannequin
layer, and knowledge entry into one other.
The advantage of having these layers in frontend functions is that you just
solely want to grasp one piece with out worrying about others. Additionally, with
the development of reusability, making adjustments to current code could be
comparatively extra manageable than earlier than.