Whereas I’ve put React utility, there is not such a factor as React utility. I imply, there are
front-end functions written in JavaScript or TypeScript that occur to
use React as their views. Nonetheless, I believe it is not truthful to name them React
functions, simply as we would not name a Java EE utility JSP
utility.
As a rule, individuals squeeze various things into React
elements or hooks to make the applying work. One of these
less-organised construction is not an issue if the applying is small or
principally with out a lot enterprise logic. Nonetheless, as extra enterprise logic shifted
to front-end in lots of circumstances, this everything-in-component exhibits issues. To
be extra particular, the trouble of understanding such kind of code is
comparatively excessive, in addition to the elevated danger to code modification.
On this article, I want to talk about just a few patterns and strategies
you need to use to reshape your “React utility” into a daily one, and solely
with React as its view (you’ll be able to even swap these views into one other view
libray with out an excessive amount of efforts).
The vital level right here is you need to analyse what function every a part of the
code is taking part in inside an utility (even on the floor, they is likely to be
packed in the identical file). Separate view from no-view logic, cut up the
no-view logic additional by their tasks and place them within the
proper locations.
The good thing about this separation is that it means that you can make modifications in
the underlying area logic with out worrying an excessive amount of concerning the floor
views, or vice versa. Additionally, it may well improve the reusability of the area
logic elsewhere as they don’t seem to be coupled to another elements.
React is a humble library for constructing views
It is simple to neglect that React, at its core, is a library (not a
framework) that helps you construct the person interface.
On this context, it’s emphasised that React is a JavaScript library
that concentrates on a selected side of net improvement, particularly UI
elements, and gives ample freedom by way of the design of the
utility and its total construction.
A JavaScript library for constructing person interfaces
It could sound fairly simple. However I’ve seen many circumstances the place
individuals write the info fetching, reshaping logic proper within the place the place
it is consumed. For instance, fetching information inside a React part, within the
useEffect
block proper above the rendering, or performing information
mapping/reworking as soon as they obtained the response from the server aspect.
useEffect(() => { fetch("https://handle.service/api") .then((res) => res.json()) .then((information) => { const addresses = information.map((merchandise) => ({ road: merchandise.streetName, handle: merchandise.streetAddress, postcode: merchandise.postCode, })); setAddresses(addresses); }); }); // the precise rendering...
Maybe as a result of there’s but to be a common commonplace within the frontend
world, or it is only a dangerous programming behavior. Frontend functions ought to
not be handled too otherwise from common software program functions. Within the
frontend world, you continue to use separation of issues generally to rearrange
the code construction. And all of the confirmed helpful design patterns nonetheless
apply.
Welcome to the actual world React utility
Most builders had been impressed by React’s simplicity and the concept
a person interface could be expressed as a pure perform to map information into the
DOM. And to a sure extent, it IS.
However builders begin to battle when they should ship a community
request to a backend or carry out web page navigation, as these uncomfortable side effects
make the part much less “pure”. And when you think about these completely different
states (both international state or native state), issues shortly get
difficult, and the darkish aspect of the person interface emerges.
Other than the person interface
React itself doesn’t care a lot about the place to place calculation or
enterprise logic, which is truthful because it’s solely a library for constructing person
interfaces. And past that view layer, a frontend utility has different
elements as properly. To make the applying work, you have to a router,
native storage, cache at completely different ranges, community requests, Third-party
integrations, Third-party login, safety, logging, efficiency tuning,
and many others.
With all this further context, attempting to squeeze the whole lot into
React elements or hooks is usually not a good suggestion. The reason being
mixing ideas in a single place typically results in extra confusion. At
first, the part units up some community request for order standing, and
then there’s some logic to trim off main area from a string and
then navigate some other place. The reader should always reset their
logic circulation and bounce backwards and forwards from completely different ranges of particulars.
Packing all of the code into elements may match in small functions
like a Todo or one-form utility. Nonetheless, the efforts to grasp
such utility will probably be vital as soon as it reaches a sure stage.
To not point out including new options or fixing present defects.
If we may separate completely different issues into recordsdata or folders with
constructions, the psychological load required to grasp the applying would
be considerably lowered. And also you solely need to concentrate on one factor at a
time. Fortunately, there are already some well-proven patterns again to the
pre-web time. These design ideas and patterns are explored and
mentioned properly to resolve the widespread person interface issues – however within the
desktop GUI utility context.
Martin Fowler has an important abstract of the idea of view-model-data
layering.
On the entire I’ve discovered this to be an efficient type of
modularization for a lot of functions and one which I recurrently use and
encourage. It is largest benefit is that it permits me to extend my
focus by permitting me to consider the three subjects (i.e., view,
mannequin, information) comparatively independently.
Layered architectures have been used to manage the challenges in giant
GUI functions, and positively we are able to use these established patterns of
front-end group in our “React functions”.
The evolution of a React utility
For small or one-off tasks, you would possibly discover that every one logic is simply
written inside React elements. You may even see one or just a few elements
in whole. The code appears just about like HTML, with just some variable or
state used to make the web page “dynamic”. Some would possibly ship requests to fetch
information on useEffect
after the elements render.
As the applying grows, and an increasing number of code are added to codebase.
With out a correct approach to organise them, quickly the codebase will flip into
unmaintainable state, which means that even including small options could be
time-consuming as builders want extra time to learn the code.
So I’ll listing just a few steps that may assist to reduction the maintainable
downside. It typically require a bit extra efforts, however it’ll repay to
have the construction in you utility. Let’s have a fast evaluation of those
steps to construct front-end functions that scale.
Single Part Software
It may be known as just about a Single Part Software:
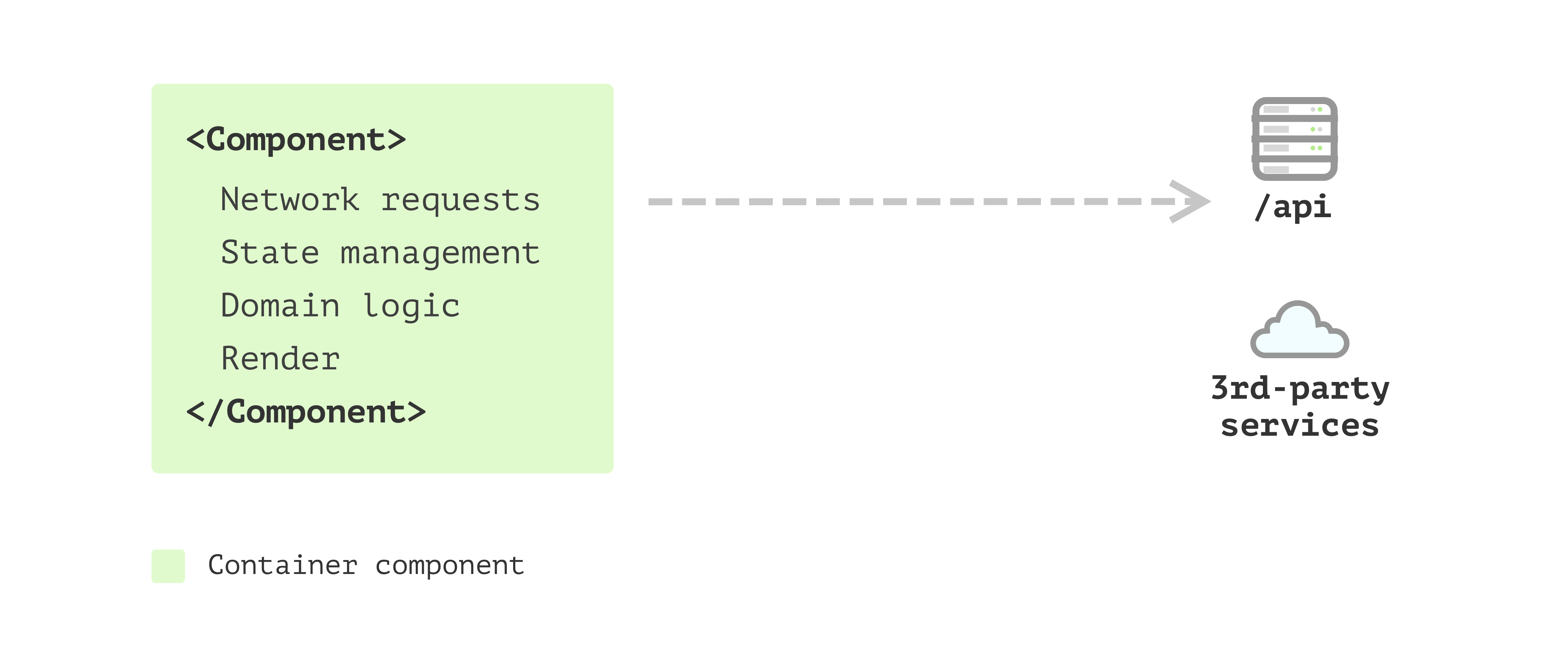
Determine 1: Single Part Software
However quickly, you realise one single part requires plenty of time
simply to learn what’s going on. For instance, there’s logic to iterate
by way of an inventory and generate every merchandise. Additionally, there’s some logic for
utilizing Third-party elements with just a few configuration code, aside
from different logic.
A number of Part Software
You determined to separate the part into a number of elements, with
these constructions reflecting what’s taking place on the end result HTML is a
good thought, and it lets you concentrate on one part at a time.
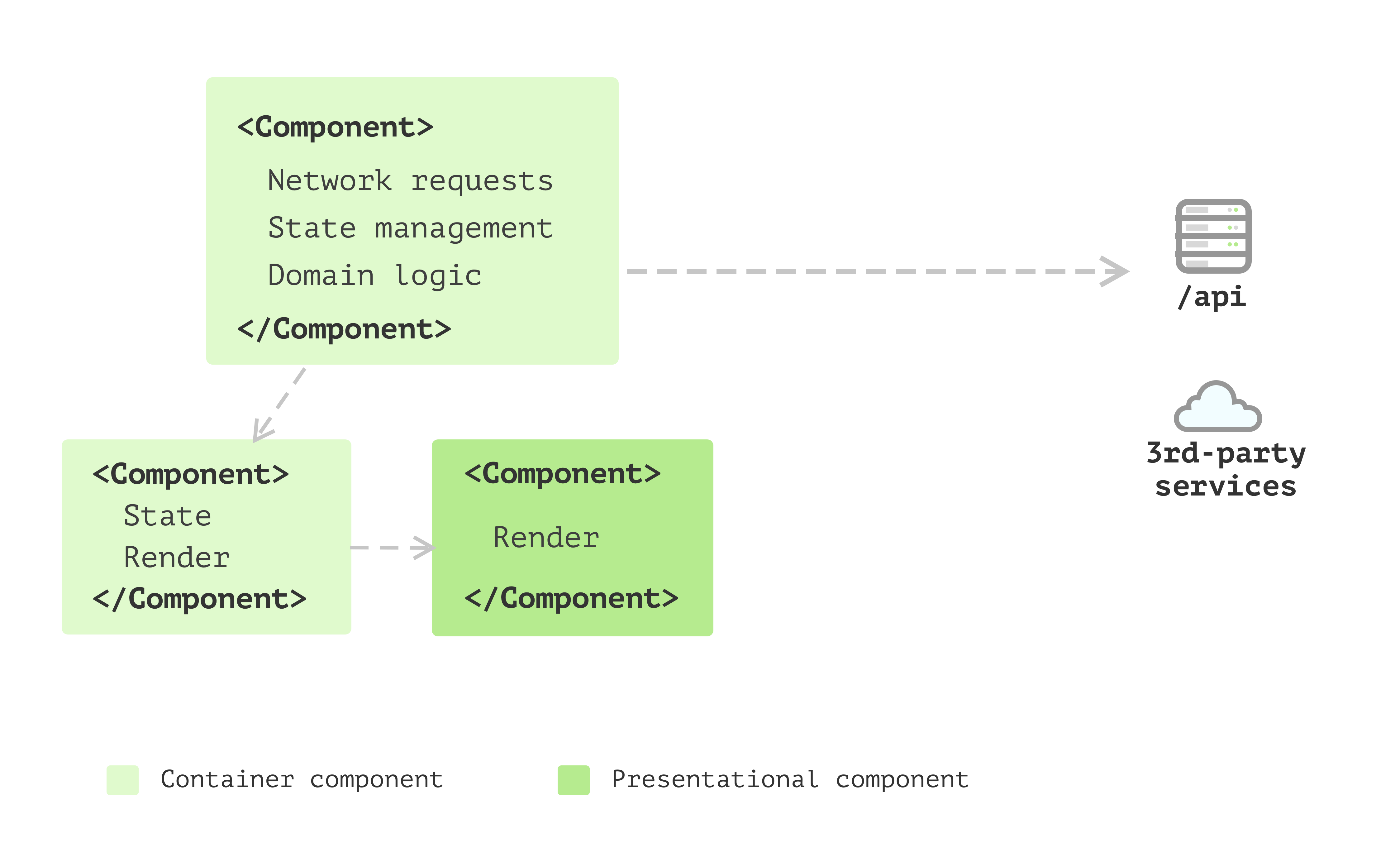
Determine 2: A number of Part Software
And as your utility grows, aside from the view, there are issues
like sending community requests, changing information into completely different shapes for
the view to eat, and gathering information to ship again to the server. And
having this code inside elements doesn’t really feel proper as they’re not
actually about person interfaces. Additionally, some elements have too many
inside states.
State administration with hooks
It’s a greater thought to separate this logic right into a separate locations.
Fortunately in React, you’ll be able to outline your individual hooks. It is a nice approach to
share these state and the logic of at any time when states change.
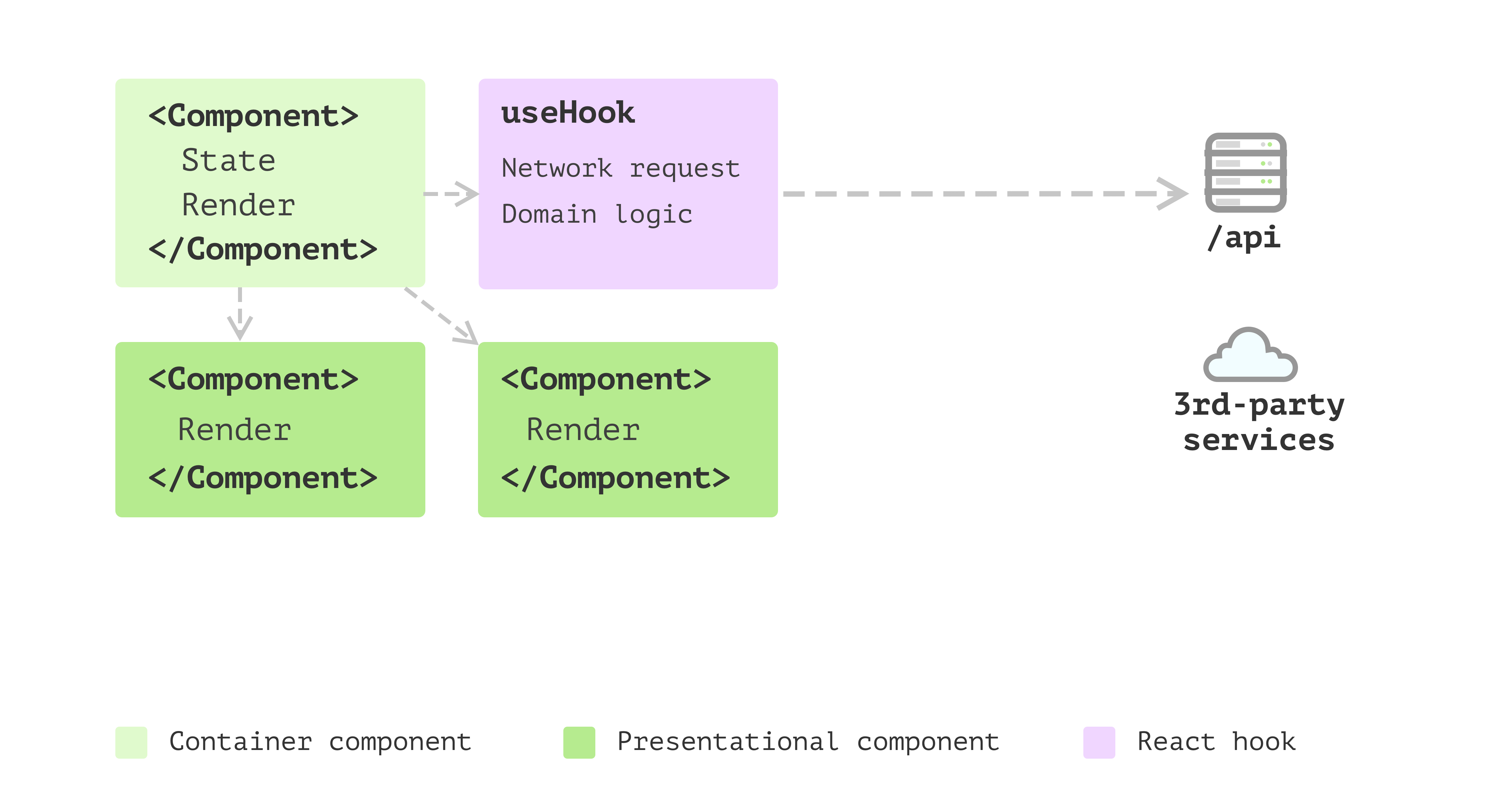
Determine 3: State administration with hooks
That’s superior! You’ve got a bunch of components extracted out of your
single part utility, and you’ve got just a few pure presentational
elements and a few reusable hooks that make different elements stateful.
The one downside is that in hooks, aside from the aspect impact and state
administration, some logic doesn’t appear to belong to the state administration
however pure calculations.
Enterprise fashions emerged
So that you’ve began to turn out to be conscious that extracting this logic into but
one other place can deliver you a lot advantages. For instance, with that cut up,
the logic could be cohesive and impartial of any views. You then extract
just a few area objects.
These easy objects can deal with information mapping (from one format to
one other), test nulls and use fallback values as required. Additionally, because the
quantity of those area objects grows, you discover you want some inheritance
or polymorphism to make issues even cleaner. Thus you utilized many
design patterns you discovered useful from different locations into the front-end
utility right here.
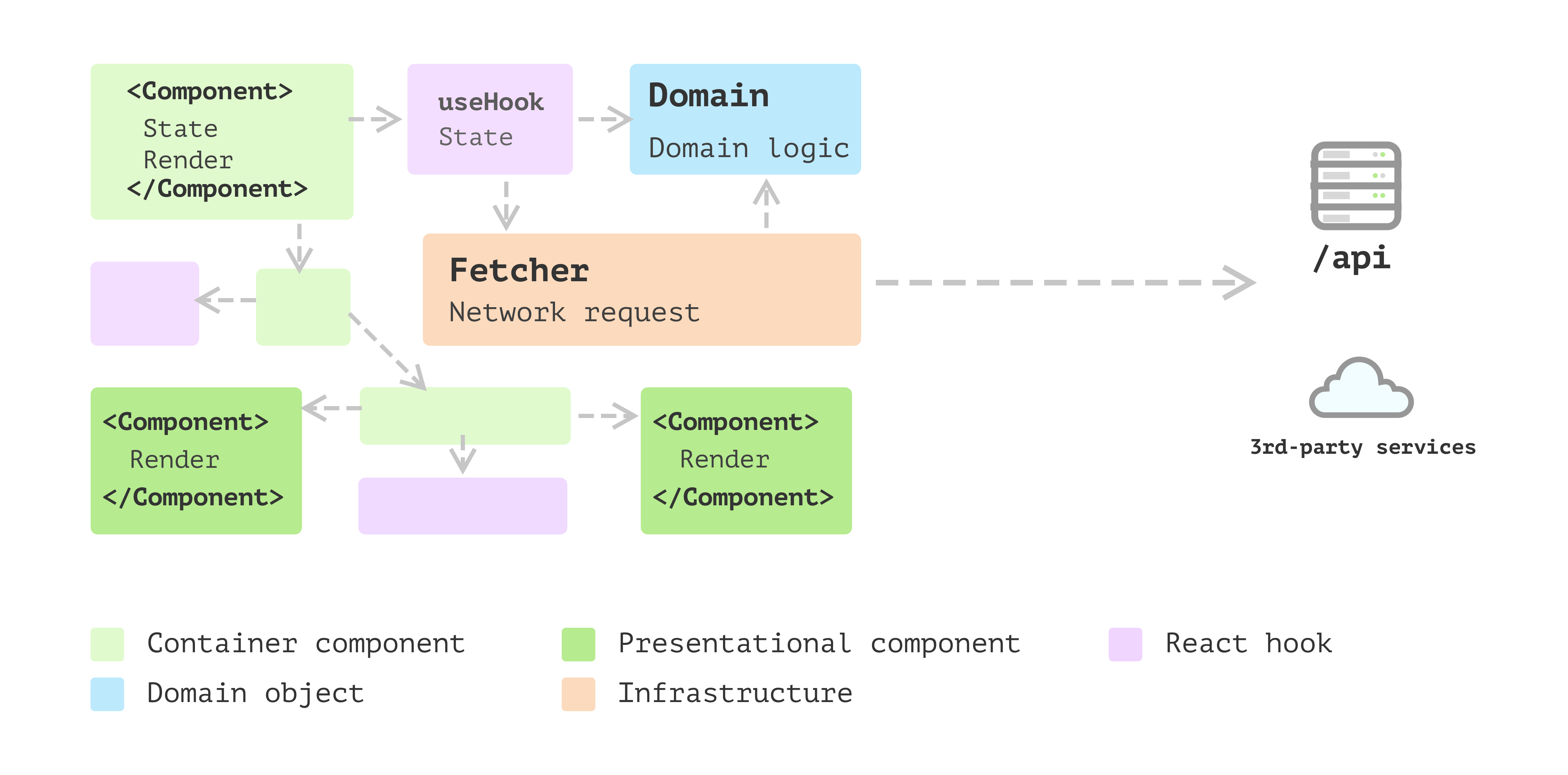
Determine 4: Enterprise fashions
Layered frontend utility
The appliance retains evolving, and then you definately discover some patterns
emerge. There are a bunch of objects that don’t belong to any person
interface, and so they additionally don’t care about whether or not the underlying information is
from distant service, native storage or cache. After which, you need to cut up
them into completely different layers. Here’s a detailed clarification concerning the layer
splitting Presentation Area Information Layering.
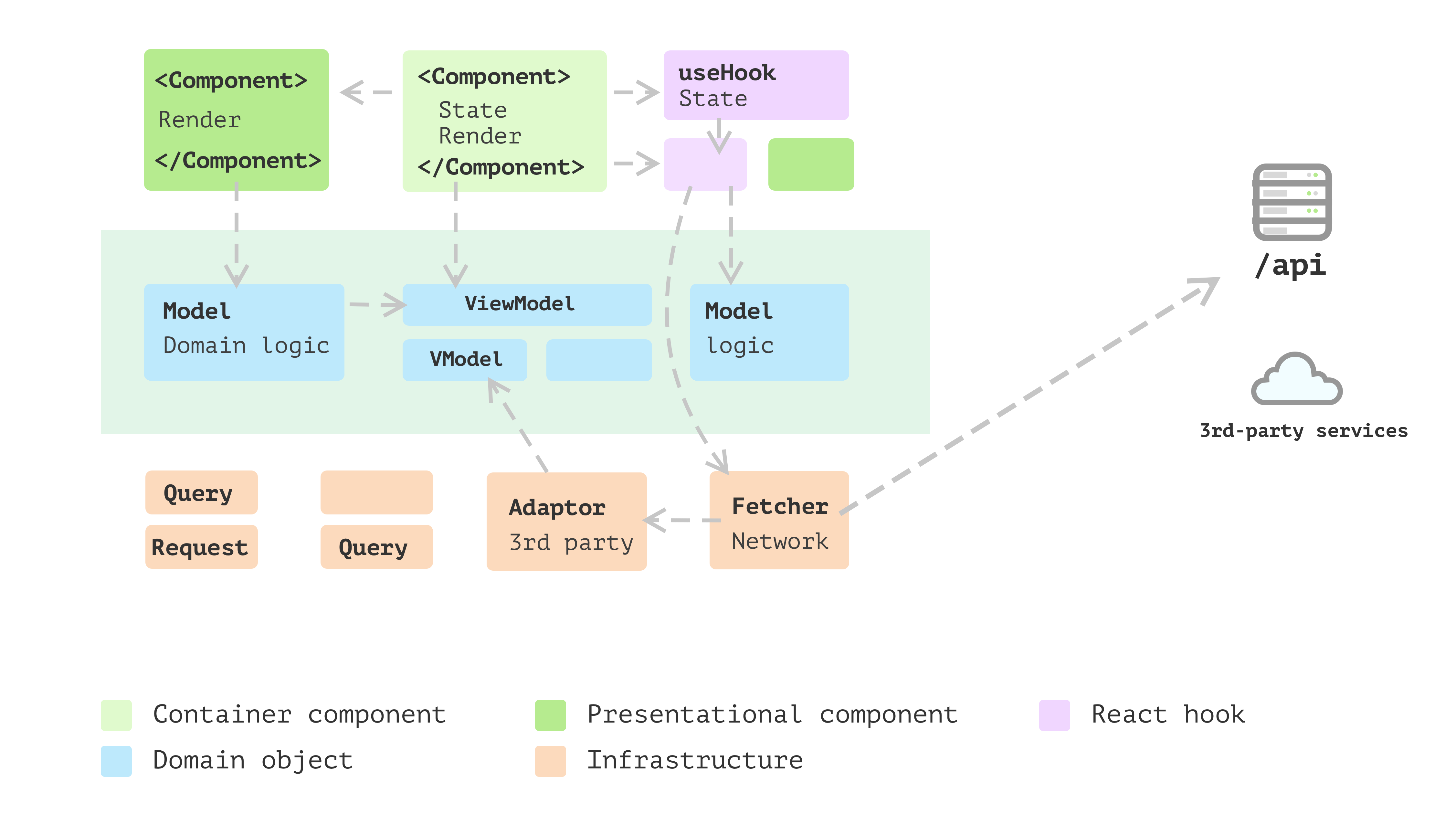
Determine 5: Layered frontend utility
The above evolution course of is a high-level overview, and you need to
have a style of how you need to construction your code or at the least what the
route ought to be. Nonetheless, there will probably be many particulars it’s worthwhile to
think about earlier than making use of the speculation in your utility.
Within the following sections, I’ll stroll you thru a characteristic I
extracted from an actual venture to exhibit all of the patterns and design
ideas I believe helpful for giant frontend functions.