As we speak, most functions can ship a whole lot of requests for a single web page.
For instance, my Twitter residence web page sends round 300 requests, and an Amazon
product particulars web page sends round 600 requests. A few of them are for static
property (JavaScript, CSS, font recordsdata, icons, and many others.), however there are nonetheless
round 100 requests for async knowledge fetching – both for timelines, associates,
or product suggestions, in addition to analytics occasions. That’s fairly a
lot.
The principle cause a web page could include so many requests is to enhance
efficiency and consumer expertise, particularly to make the applying really feel
sooner to the top customers. The period of clean pages taking 5 seconds to load is
lengthy gone. In trendy net functions, customers usually see a fundamental web page with
fashion and different components in lower than a second, with extra items
loading progressively.
Take the Amazon product element web page for instance. The navigation and prime
bar seem nearly instantly, adopted by the product photographs, transient, and
descriptions. Then, as you scroll, “Sponsored” content material, scores,
suggestions, view histories, and extra seem.Typically, a consumer solely desires a
fast look or to check merchandise (and verify availability), making
sections like “Prospects who purchased this merchandise additionally purchased” much less vital and
appropriate for loading by way of separate requests.
Breaking down the content material into smaller items and loading them in
parallel is an efficient technique, nevertheless it’s removed from sufficient in giant
functions. There are lots of different facets to contemplate in the case of
fetch knowledge appropriately and effectively. Information fetching is a chellenging, not
solely as a result of the character of async programming would not match our linear mindset,
and there are such a lot of components could cause a community name to fail, but additionally
there are too many not-obvious instances to contemplate below the hood (knowledge
format, safety, cache, token expiry, and many others.).
On this article, I want to talk about some frequent issues and
patterns you must take into account in the case of fetching knowledge in your frontend
functions.
We’ll start with the Asynchronous State Handler sample, which decouples
knowledge fetching from the UI, streamlining your utility structure. Subsequent,
we’ll delve into Fallback Markup, enhancing the intuitiveness of your knowledge
fetching logic. To speed up the preliminary knowledge loading course of, we’ll
discover methods for avoiding Request
Waterfall and implementing Parallel Information Fetching. Our dialogue will then cowl Code Splitting to defer
loading non-critical utility elements and Prefetching knowledge based mostly on consumer
interactions to raise the consumer expertise.
I consider discussing these ideas by means of a simple instance is
one of the best strategy. I intention to begin merely after which introduce extra complexity
in a manageable method. I additionally plan to maintain code snippets, significantly for
styling (I am using TailwindCSS for the UI, which can lead to prolonged
snippets in a React element), to a minimal. For these within the
full particulars, I’ve made them obtainable on this
repository.
Developments are additionally occurring on the server facet, with methods like
Streaming Server-Facet Rendering and Server Parts gaining traction in
numerous frameworks. Moreover, a variety of experimental strategies are
rising. Nevertheless, these matters, whereas doubtlessly simply as essential, is perhaps
explored in a future article. For now, this dialogue will focus
solely on front-end knowledge fetching patterns.
It is necessary to notice that the methods we’re protecting should not
unique to React or any particular frontend framework or library. I’ve
chosen React for illustration functions because of my in depth expertise with
it in recent times. Nevertheless, ideas like Code Splitting,
Prefetching are
relevant throughout frameworks like Angular or Vue.js. The examples I will share
are frequent situations you may encounter in frontend growth, regardless
of the framework you utilize.
That mentioned, let’s dive into the instance we’re going to make use of all through the
article, a Profile
display of a Single-Web page Software. It is a typical
utility you might need used earlier than, or at the very least the state of affairs is typical.
We have to fetch knowledge from server facet after which at frontend to construct the UI
dynamically with JavaScript.
Introducing the applying
To start with, on Profile
we’ll present the consumer’s transient (together with
title, avatar, and a brief description), after which we additionally need to present
their connections (much like followers on Twitter or LinkedIn
connections). We’ll must fetch consumer and their connections knowledge from
distant service, after which assembling these knowledge with UI on the display.
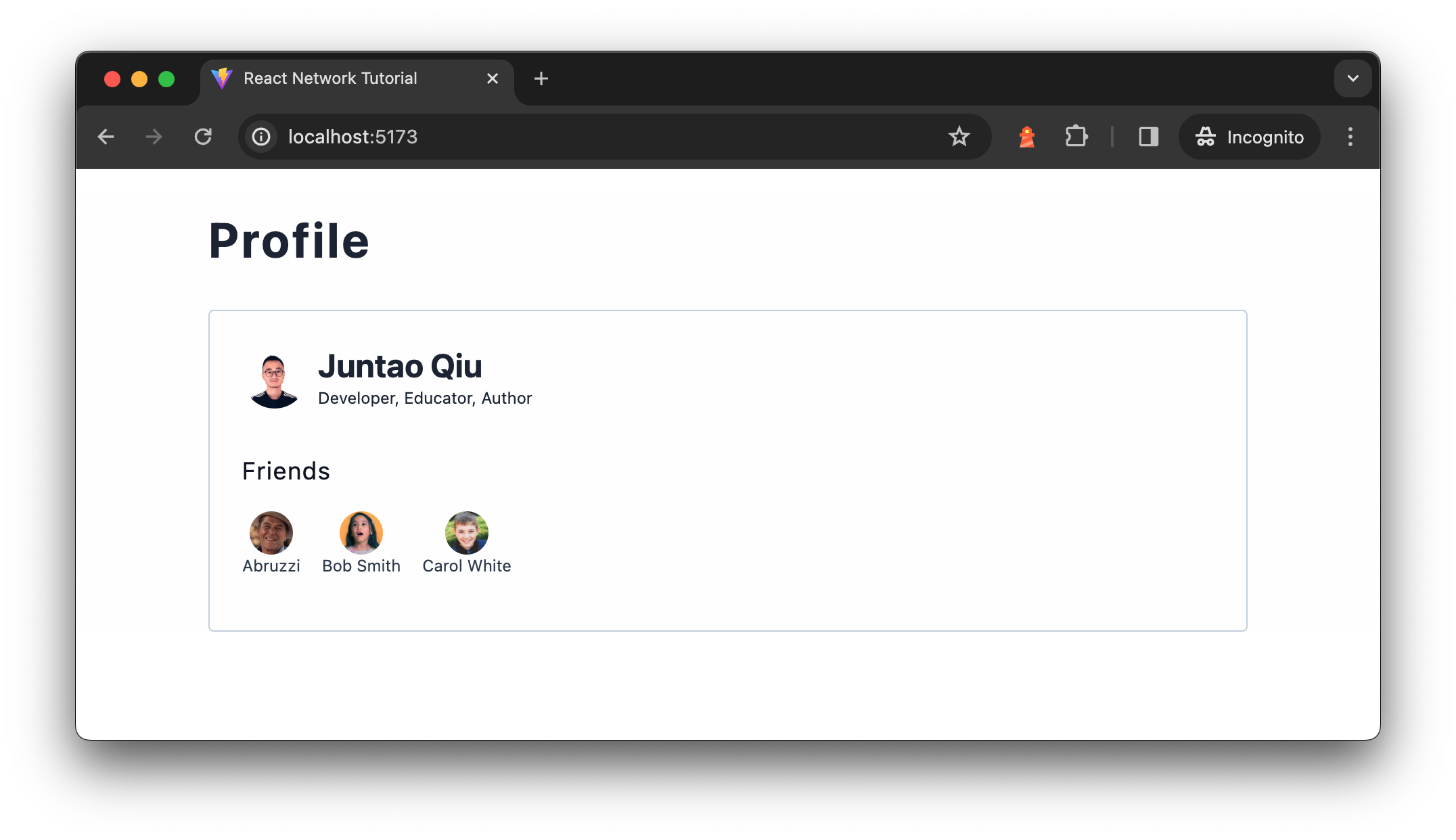
Determine 1: Profile display
The info are from two separate API calls, the consumer transient API
/customers/<id>
returns consumer transient for a given consumer id, which is a straightforward
object described as follows:
{ "id": "u1", "title": "Juntao Qiu", "bio": "Developer, Educator, Creator", "pursuits": [ "Technology", "Outdoors", "Travel" ] }
And the good friend API /customers/<id>/associates
endpoint returns an inventory of
associates for a given consumer, every record merchandise within the response is similar as
the above consumer knowledge. The explanation we’ve two endpoints as an alternative of returning
a associates
part of the consumer API is that there are instances the place one
may have too many associates (say 1,000), however most individuals do not have many.
This in-balance knowledge construction might be fairly difficult, particularly after we
must paginate. The purpose right here is that there are instances we have to deal
with a number of community requests.
A quick introduction to related React ideas
As this text leverages React for example numerous patterns, I do
not assume you recognize a lot about React. Moderately than anticipating you to spend so much
of time looking for the suitable elements within the React documentation, I’ll
briefly introduce these ideas we’ll make the most of all through this
article. In case you already perceive what React elements are, and the
use of the
useState
and useEffect
hooks, chances are you’ll
use this hyperlink to skip forward to the subsequent
part.
For these searching for a extra thorough tutorial, the new React documentation is a superb
useful resource.
What’s a React Element?
In React, elements are the elemental constructing blocks. To place it
merely, a React element is a operate that returns a chunk of UI,
which might be as easy as a fraction of HTML. Take into account the
creation of a element that renders a navigation bar:
import React from 'react'; operate Navigation() { return ( <nav> <ol> <li>Residence</li> <li>Blogs</li> <li>Books</li> </ol> </nav> ); }
At first look, the combination of JavaScript with HTML tags might sound
unusual (it is known as JSX, a syntax extension to JavaScript. For these
utilizing TypeScript, the same syntax known as TSX is used). To make this
code purposeful, a compiler is required to translate the JSX into legitimate
JavaScript code. After being compiled by Babel,
the code would roughly translate to the next:
operate Navigation() { return React.createElement( "nav", null, React.createElement( "ol", null, React.createElement("li", null, "Residence"), React.createElement("li", null, "Blogs"), React.createElement("li", null, "Books") ) ); }
Notice right here the translated code has a operate known as
React.createElement
, which is a foundational operate in
React for creating components. JSX written in React elements is compiled
right down to React.createElement
calls behind the scenes.
The fundamental syntax of React.createElement
is:
React.createElement(sort, [props], [...children])
sort
: A string (e.g., ‘div’, ‘span’) indicating the kind of
DOM node to create, or a React element (class or purposeful) for
extra refined buildings.props
: An object containing properties handed to the
component or element, together with occasion handlers, types, and attributes
likeclassName
andid
.youngsters
: These non-compulsory arguments might be extra
React.createElement
calls, strings, numbers, or any combine
thereof, representing the component’s youngsters.
As an example, a easy component might be created with
React.createElement
as follows:
React.createElement('div', { className: 'greeting' }, 'Hi there, world!');
That is analogous to the JSX model:
<div className="greeting">Hi there, world!</div>
Beneath the floor, React invokes the native DOM API (e.g.,
doc.createElement("ol")
) to generate DOM components as crucial.
You may then assemble your customized elements right into a tree, much like
HTML code:
import React from 'react'; import Navigation from './Navigation.tsx'; import Content material from './Content material.tsx'; import Sidebar from './Sidebar.tsx'; import ProductList from './ProductList.tsx'; operate App() { return <Web page />; } operate Web page() { return <Container> <Navigation /> <Content material> <Sidebar /> <ProductList /> </Content material> <Footer /> </Container>; }
In the end, your utility requires a root node to mount to, at
which level React assumes management and manages subsequent renders and
re-renders:
import ReactDOM from "react-dom/consumer"; import App from "./App.tsx"; const root = ReactDOM.createRoot(doc.getElementById('root')); root.render(<App />);
Producing Dynamic Content material with JSX
The preliminary instance demonstrates a simple use case, however
let’s discover how we are able to create content material dynamically. As an example, how
can we generate an inventory of information dynamically? In React, as illustrated
earlier, a element is basically a operate, enabling us to cross
parameters to it.
import React from 'react'; operate Navigation({ nav }) { return ( <nav> <ol> {nav.map(merchandise => <li key={merchandise}>{merchandise}</li>)} </ol> </nav> ); }
On this modified Navigation
element, we anticipate the
parameter to be an array of strings. We make the most of the map
operate to iterate over every merchandise, reworking them into
<li>
components. The curly braces {}
signify
that the enclosed JavaScript expression needs to be evaluated and
rendered. For these curious concerning the compiled model of this dynamic
content material dealing with:
operate Navigation(props) { var nav = props.nav; return React.createElement( "nav", null, React.createElement( "ol", null, nav.map(operate(merchandise) { return React.createElement("li", { key: merchandise }, merchandise); }) ) ); }
As an alternative of invoking Navigation
as an everyday operate,
using JSX syntax renders the element invocation extra akin to
writing markup, enhancing readability:
// As an alternative of this Navigation(["Home", "Blogs", "Books"]) // We do that <Navigation nav={["Home", "Blogs", "Books"]} />
Parts in React can obtain various knowledge, referred to as props, to
modify their habits, very like passing arguments right into a operate (the
distinction lies in utilizing JSX syntax, making the code extra acquainted and
readable to these with HTML information, which aligns nicely with the ability
set of most frontend builders).
import React from 'react'; import Checkbox from './Checkbox'; import BookList from './BookList'; operate App() { let showNewOnly = false; // This flag's worth is often set based mostly on particular logic. const filteredBooks = showNewOnly ? booksData.filter(e-book => e-book.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly}> Present New Printed Books Solely </Checkbox> <BookList books={filteredBooks} /> </div> ); }
On this illustrative code snippet (non-functional however supposed to
show the idea), we manipulate the BookList
element’s displayed content material by passing it an array of books. Relying
on the showNewOnly
flag, this array is both all obtainable
books or solely these which are newly printed, showcasing how props can
be used to dynamically modify element output.
Managing Inside State Between Renders: useState
Constructing consumer interfaces (UI) usually transcends the era of
static HTML. Parts incessantly must “keep in mind” sure states and
reply to consumer interactions dynamically. As an example, when a consumer
clicks an “Add” button in a Product element, it is necessary to replace
the ShoppingCart element to replicate each the entire worth and the
up to date merchandise record.
Within the earlier code snippet, trying to set the
showNewOnly
variable to true
inside an occasion
handler doesn’t obtain the specified impact:
operate App () { let showNewOnly = false; const handleCheckboxChange = () => { showNewOnly = true; // this does not work }; const filteredBooks = showNewOnly ? booksData.filter(e-book => e-book.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly} onChange={handleCheckboxChange}> Present New Printed Books Solely </Checkbox> <BookList books={filteredBooks}/> </div> ); };
This strategy falls brief as a result of native variables inside a operate
element don’t persist between renders. When React re-renders this
element, it does so from scratch, disregarding any adjustments made to
native variables since these don’t set off re-renders. React stays
unaware of the necessity to replace the element to replicate new knowledge.
This limitation underscores the need for React’s
state
. Particularly, purposeful elements leverage the
useState
hook to recollect states throughout renders. Revisiting
the App
instance, we are able to successfully keep in mind the
showNewOnly
state as follows:
import React, { useState } from 'react'; import Checkbox from './Checkbox'; import BookList from './BookList'; operate App () { const [showNewOnly, setShowNewOnly] = useState(false); const handleCheckboxChange = () => { setShowNewOnly(!showNewOnly); }; const filteredBooks = showNewOnly ? booksData.filter(e-book => e-book.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly} onChange={handleCheckboxChange}> Present New Printed Books Solely </Checkbox> <BookList books={filteredBooks}/> </div> ); };
The useState
hook is a cornerstone of React’s Hooks system,
launched to allow purposeful elements to handle inner state. It
introduces state to purposeful elements, encapsulated by the next
syntax:
const [state, setState] = useState(initialState);
initialState
: This argument is the preliminary
worth of the state variable. It may be a easy worth like a quantity,
string, boolean, or a extra advanced object or array. The
initialState
is barely used throughout the first render to
initialize the state.- Return Worth:
useState
returns an array with
two components. The primary component is the present state worth, and the
second component is a operate that permits updating this worth. Through the use of
array destructuring, we assign names to those returned gadgets,
usuallystate
andsetState
, although you may
select any legitimate variable names. state
: Represents the present worth of the
state. It is the worth that can be used within the element’s UI and
logic.setState
: A operate to replace the state. This operate
accepts a brand new state worth or a operate that produces a brand new state based mostly
on the earlier state. When known as, it schedules an replace to the
element’s state and triggers a re-render to replicate the adjustments.
React treats state as a snapshot; updating it would not alter the
present state variable however as an alternative triggers a re-render. Throughout this
re-render, React acknowledges the up to date state, making certain the
BookList
element receives the proper knowledge, thereby
reflecting the up to date e-book record to the consumer. This snapshot-like
habits of state facilitates the dynamic and responsive nature of React
elements, enabling them to react intuitively to consumer interactions and
different adjustments.
Managing Facet Results: useEffect
Earlier than diving deeper into our dialogue, it is essential to deal with the
idea of unintended effects. Uncomfortable side effects are operations that work together with
the skin world from the React ecosystem. Widespread examples embody
fetching knowledge from a distant server or dynamically manipulating the DOM,
similar to altering the web page title.
React is primarily involved with rendering knowledge to the DOM and does
not inherently deal with knowledge fetching or direct DOM manipulation. To
facilitate these unintended effects, React gives the useEffect
hook. This hook permits the execution of unintended effects after React has
accomplished its rendering course of. If these unintended effects end in knowledge
adjustments, React schedules a re-render to replicate these updates.
The useEffect
Hook accepts two arguments:
- A operate containing the facet impact logic.
- An non-compulsory dependency array specifying when the facet impact needs to be
re-invoked.
Omitting the second argument causes the facet impact to run after
each render. Offering an empty array []
signifies that your impact
doesn’t rely on any values from props or state, thus not needing to
re-run. Together with particular values within the array means the facet impact
solely re-executes if these values change.
When coping with asynchronous knowledge fetching, the workflow inside
useEffect
entails initiating a community request. As soon as the information is
retrieved, it’s captured by way of the useState
hook, updating the
element’s inner state and preserving the fetched knowledge throughout
renders. React, recognizing the state replace, undertakes one other render
cycle to include the brand new knowledge.
This is a sensible instance about knowledge fetching and state
administration:
import { useEffect, useState } from "react"; sort Person = { id: string; title: string; }; const UserSection = ({ id }) => { const [user, setUser] = useState<Person | undefined>(); useEffect(() => { const fetchUser = async () => { const response = await fetch(`/api/customers/${id}`); const jsonData = await response.json(); setUser(jsonData); }; fetchUser(); }, tag:martinfowler.com,2024-05-29:Prefetching-in-Single-Web page-Purposes); return <div> <h2>{consumer?.title}</h2> </div>; };
Within the code snippet above, inside useEffect
, an
asynchronous operate fetchUser
is outlined after which
instantly invoked. This sample is important as a result of
useEffect
doesn’t immediately help async capabilities as its
callback. The async operate is outlined to make use of await
for
the fetch operation, making certain that the code execution waits for the
response after which processes the JSON knowledge. As soon as the information is obtainable,
it updates the element’s state by way of setUser
.
The dependency array tag:martinfowler.com,2024-05-29:Prefetching-in-Single-Web page-Purposes
on the finish of the
useEffect
name ensures that the impact runs once more provided that
id
adjustments, which prevents pointless community requests on
each render and fetches new consumer knowledge when the id
prop
updates.
This strategy to dealing with asynchronous knowledge fetching inside
useEffect
is a regular observe in React growth, providing a
structured and environment friendly method to combine async operations into the
React element lifecycle.
As well as, in sensible functions, managing completely different states
similar to loading, error, and knowledge presentation is crucial too (we’ll
see it the way it works within the following part). For instance, take into account
implementing standing indicators inside a Person element to replicate
loading, error, or knowledge states, enhancing the consumer expertise by
offering suggestions throughout knowledge fetching operations.
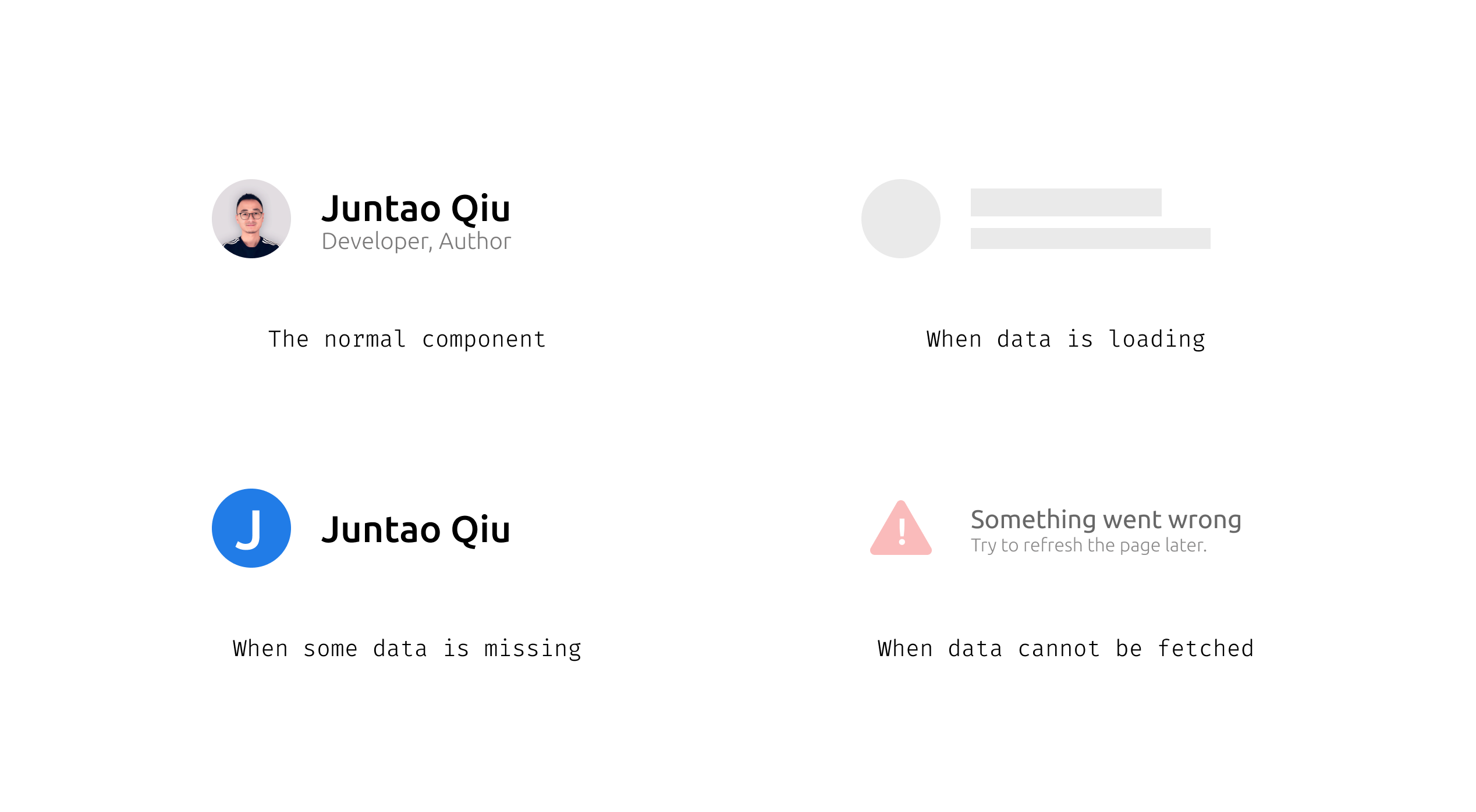
Determine 2: Totally different statuses of a
element
This overview affords only a fast glimpse into the ideas utilized
all through this text. For a deeper dive into extra ideas and
patterns, I like to recommend exploring the new React
documentation or consulting different on-line assets.
With this basis, you must now be geared up to affix me as we delve
into the information fetching patterns mentioned herein.
Implement the Profile element
Let’s create the Profile
element to make a request and
render the end result. In typical React functions, this knowledge fetching is
dealt with inside a useEffect
block. This is an instance of how
this is perhaps carried out:
import { useEffect, useState } from "react"; const Profile = ({ id }: { id: string }) => { const [user, setUser] = useState<Person | undefined>(); useEffect(() => { const fetchUser = async () => { const response = await fetch(`/api/customers/${id}`); const jsonData = await response.json(); setUser(jsonData); }; fetchUser(); }, tag:martinfowler.com,2024-05-29:Prefetching-in-Single-Web page-Purposes); return ( <UserBrief consumer={consumer} /> ); };
This preliminary strategy assumes community requests full
instantaneously, which is usually not the case. Actual-world situations require
dealing with various community circumstances, together with delays and failures. To
handle these successfully, we incorporate loading and error states into our
element. This addition permits us to offer suggestions to the consumer throughout
knowledge fetching, similar to displaying a loading indicator or a skeleton display
if the information is delayed, and dealing with errors once they happen.
Right here’s how the improved element appears to be like with added loading and error
administration:
import { useEffect, useState } from "react"; import { get } from "../utils.ts"; import sort { Person } from "../varieties.ts"; const Profile = ({ id }: { id: string }) => { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [user, setUser] = useState<Person | undefined>(); useEffect(() => { const fetchUser = async () => { attempt { setLoading(true); const knowledge = await get<Person>(`/customers/${id}`); setUser(knowledge); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; fetchUser(); }, tag:martinfowler.com,2024-05-29:Prefetching-in-Single-Web page-Purposes); if (loading || !consumer) { return <div>Loading...</div>; } return ( <> {consumer && <UserBrief consumer={consumer} />} </> ); };
Now in Profile
element, we provoke states for loading,
errors, and consumer knowledge with useState
. Utilizing
useEffect
, we fetch consumer knowledge based mostly on id
,
toggling loading standing and dealing with errors accordingly. Upon profitable
knowledge retrieval, we replace the consumer state, else show a loading
indicator.
The get
operate, as demonstrated under, simplifies
fetching knowledge from a selected endpoint by appending the endpoint to a
predefined base URL. It checks the response’s success standing and both
returns the parsed JSON knowledge or throws an error for unsuccessful requests,
streamlining error dealing with and knowledge retrieval in our utility. Notice
it is pure TypeScript code and can be utilized in different non-React elements of the
utility.
const baseurl = "https://icodeit.com.au/api/v2"; async operate get<T>(url: string): Promise<T> { const response = await fetch(`${baseurl}${url}`); if (!response.okay) { throw new Error("Community response was not okay"); } return await response.json() as Promise<T>; }
React will attempt to render the element initially, however as the information
consumer
isn’t obtainable, it returns “loading…” in a
div
. Then the useEffect
is invoked, and the
request is kicked off. As soon as sooner or later, the response returns, React
re-renders the Profile
element with consumer
fulfilled, so now you can see the consumer part with title, avatar, and
title.
If we visualize the timeline of the above code, you will notice
the next sequence. The browser firstly downloads the HTML web page, and
then when it encounters script tags and elegance tags, it would cease and
obtain these recordsdata, after which parse them to type the ultimate web page. Notice
that this can be a comparatively sophisticated course of, and I’m oversimplifying
right here, however the fundamental thought of the sequence is appropriate.
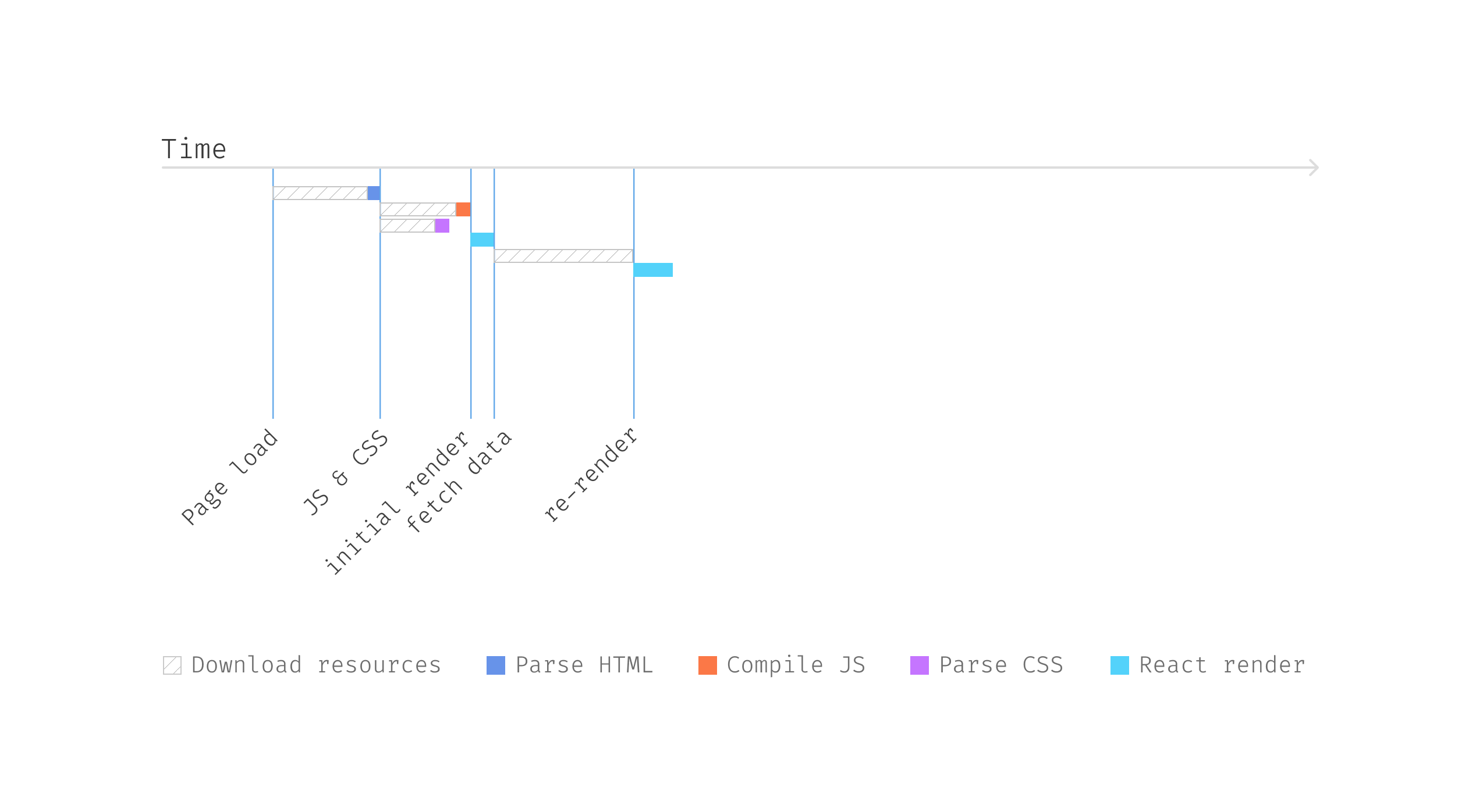
Determine 3: Fetching consumer
knowledge
So React can begin to render solely when the JS are parsed and executed,
after which it finds the useEffect
for knowledge fetching; it has to attend till
the information is obtainable for a re-render.
Now within the browser, we are able to see a “loading…” when the applying
begins, after which after a number of seconds (we are able to simulate such case by add
some delay within the API endpoints) the consumer transient part exhibits up when knowledge
is loaded.
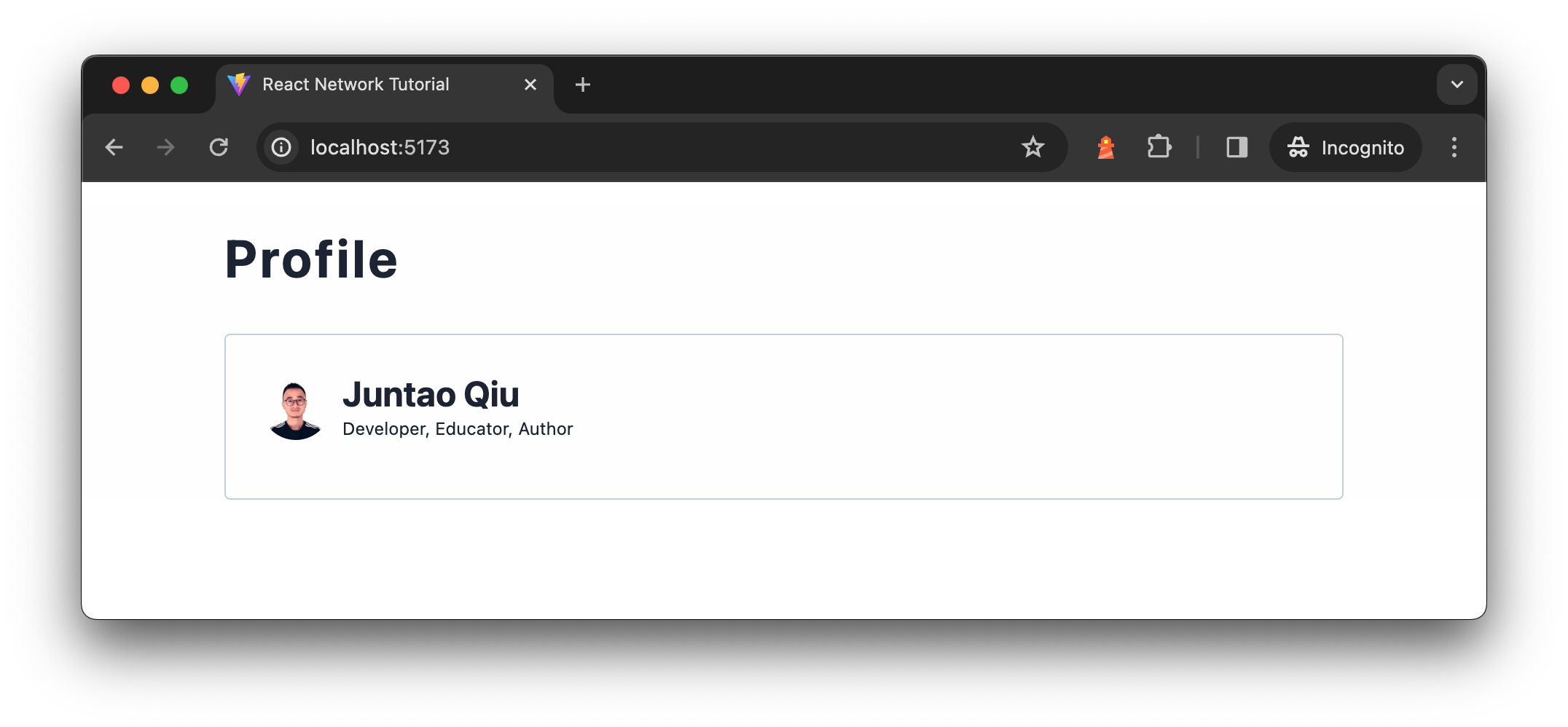
Determine 4: Person transient element
This code construction (in useEffect to set off request, and replace states
like loading
and error
correspondingly) is
extensively used throughout React codebases. In functions of standard dimension, it is
frequent to seek out quite a few situations of such similar data-fetching logic
dispersed all through numerous elements.
Asynchronous State Handler
Wrap asynchronous queries with meta-queries for the state of the
question.
Distant calls might be gradual, and it is important to not let the UI freeze
whereas these calls are being made. Due to this fact, we deal with them asynchronously
and use indicators to indicate {that a} course of is underway, which makes the
consumer expertise higher – realizing that one thing is occurring.
Moreover, distant calls may fail because of connection points,
requiring clear communication of those failures to the consumer. Due to this fact,
it is best to encapsulate every distant name inside a handler module that
manages outcomes, progress updates, and errors. This module permits the UI
to entry metadata concerning the standing of the decision, enabling it to show
various data or choices if the anticipated outcomes fail to
materialize.
A easy implementation could possibly be a operate getAsyncStates
that
returns these metadata, it takes a URL as its parameter and returns an
object containing data important for managing asynchronous
operations. This setup permits us to appropriately reply to completely different
states of a community request, whether or not it is in progress, efficiently
resolved, or has encountered an error.
const { loading, error, knowledge } = getAsyncStates(url); if (loading) { // Show a loading spinner } if (error) { // Show an error message } // Proceed to render utilizing the information
The idea right here is that getAsyncStates
initiates the
community request routinely upon being known as. Nevertheless, this won’t
at all times align with the caller’s wants. To supply extra management, we are able to additionally
expose a fetch
operate inside the returned object, permitting
the initiation of the request at a extra applicable time, in line with the
caller’s discretion. Moreover, a refetch
operate may
be offered to allow the caller to re-initiate the request as wanted,
similar to after an error or when up to date knowledge is required. The
fetch
and refetch
capabilities might be similar in
implementation, or refetch
may embody logic to verify for
cached outcomes and solely re-fetch knowledge if crucial.
const { loading, error, knowledge, fetch, refetch } = getAsyncStates(url); const onInit = () => { fetch(); }; const onRefreshClicked = () => { refetch(); }; if (loading) { // Show a loading spinner } if (error) { // Show an error message } // Proceed to render utilizing the information
This sample gives a flexible strategy to dealing with asynchronous
requests, giving builders the pliability to set off knowledge fetching
explicitly and handle the UI’s response to loading, error, and success
states successfully. By decoupling the fetching logic from its initiation,
functions can adapt extra dynamically to consumer interactions and different
runtime circumstances, enhancing the consumer expertise and utility
reliability.
Implementing Asynchronous State Handler in React with hooks
The sample might be carried out in numerous frontend libraries. For
occasion, we may distill this strategy right into a customized Hook in a React
utility for the Profile element:
import { useEffect, useState } from "react"; import { get } from "../utils.ts"; const useUser = (id: string) => { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [user, setUser] = useState<Person | undefined>(); useEffect(() => { const fetchUser = async () => { attempt { setLoading(true); const knowledge = await get<Person>(`/customers/${id}`); setUser(knowledge); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; fetchUser(); }, tag:martinfowler.com,2024-05-29:Prefetching-in-Single-Web page-Purposes); return { loading, error, consumer, }; };
Please be aware that within the customized Hook, we have no JSX code –
which means it’s very UI free however sharable stateful logic. And the
useUser
launch knowledge routinely when known as. Inside the Profile
element, leveraging the useUser
Hook simplifies its logic:
import { useUser } from './useUser.ts'; import UserBrief from './UserBrief.tsx'; const Profile = ({ id }: { id: string }) => { const { loading, error, consumer } = useUser(id); if (loading || !consumer) { return <div>Loading...</div>; } if (error) { return <div>One thing went flawed...</div>; } return ( <> {consumer && <UserBrief consumer={consumer} />} </> ); };
Generalizing Parameter Utilization
In most functions, fetching various kinds of knowledge—from consumer
particulars on a homepage to product lists in search outcomes and
suggestions beneath them—is a typical requirement. Writing separate
fetch capabilities for every sort of information might be tedious and troublesome to
keep. A greater strategy is to summary this performance right into a
generic, reusable hook that may deal with numerous knowledge varieties
effectively.
Take into account treating distant API endpoints as companies, and use a generic
useService
hook that accepts a URL as a parameter whereas managing all
the metadata related to an asynchronous request:
import { get } from "../utils.ts"; operate useService<T>(url: string) { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [data, setData] = useState<T | undefined>(); const fetch = async () => { attempt { setLoading(true); const knowledge = await get<T>(url); setData(knowledge); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; return { loading, error, knowledge, fetch, }; }
This hook abstracts the information fetching course of, making it simpler to
combine into any element that should retrieve knowledge from a distant
supply. It additionally centralizes frequent error dealing with situations, similar to
treating particular errors otherwise:
import { useService } from './useService.ts'; const { loading, error, knowledge: consumer, fetch: fetchUser, } = useService(`/customers/${id}`);
Through the use of useService, we are able to simplify how elements fetch and deal with
knowledge, making the codebase cleaner and extra maintainable.
Variation of the sample
A variation of the useUser
could be expose the
fetchUsers
operate, and it doesn’t set off the information
fetching itself:
import { useState } from "react"; const useUser = (id: string) => { // outline the states const fetchUser = async () => { attempt { setLoading(true); const knowledge = await get<Person>(`/customers/${id}`); setUser(knowledge); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; return { loading, error, consumer, fetchUser, }; };
After which on the calling web site, Profile
element use
useEffect
to fetch the information and render completely different
states.
const Profile = ({ id }: { id: string }) => { const { loading, error, consumer, fetchUser } = useUser(id); useEffect(() => { fetchUser(); }, []); // render correspondingly };
The benefit of this division is the power to reuse these stateful
logics throughout completely different elements. As an example, one other element
needing the identical knowledge (a consumer API name with a consumer ID) can merely import
the useUser
Hook and make the most of its states. Totally different UI
elements may select to work together with these states in numerous methods,
maybe utilizing various loading indicators (a smaller spinner that
matches to the calling element) or error messages, but the elemental
logic of fetching knowledge stays constant and shared.
When to make use of it
Separating knowledge fetching logic from UI elements can typically
introduce pointless complexity, significantly in smaller functions.
Protecting this logic built-in inside the element, much like the
css-in-js strategy, simplifies navigation and is simpler for some
builders to handle. In my article, Modularizing
React Purposes with Established UI Patterns, I explored
numerous ranges of complexity in utility buildings. For functions
which are restricted in scope — with only a few pages and several other knowledge
fetching operations — it is usually sensible and in addition really helpful to
keep knowledge fetching inside the UI elements.
Nevertheless, as your utility scales and the event crew grows,
this technique could result in inefficiencies. Deep element bushes can gradual
down your utility (we’ll see examples in addition to learn how to deal with
them within the following sections) and generate redundant boilerplate code.
Introducing an Asynchronous State Handler can mitigate these points by
decoupling knowledge fetching from UI rendering, enhancing each efficiency
and maintainability.
It’s essential to steadiness simplicity with structured approaches as your
challenge evolves. This ensures your growth practices stay
efficient and aware of the applying’s wants, sustaining optimum
efficiency and developer effectivity whatever the challenge
scale.
Implement the Pals record
Now let’s take a look on the second part of the Profile – the good friend
record. We are able to create a separate element Pals
and fetch knowledge in it
(through the use of a useService customized hook we outlined above), and the logic is
fairly much like what we see above within the Profile
element.
const Pals = ({ id }: { id: string }) => { const { loading, error, knowledge: associates } = useService(`/customers/${id}/associates`); // loading & error dealing with... return ( <div> <h2>Pals</h2> <div> {associates.map((consumer) => ( // render consumer record ))} </div> </div> ); };
After which within the Profile element, we are able to use Pals as an everyday
element, and cross in id
as a prop:
const Profile = ({ id }: { id: string }) => { //... return ( <> {consumer && <UserBrief consumer={consumer} />} <Pals id={id} /> </> ); };
The code works effective, and it appears to be like fairly clear and readable,
UserBrief
renders a consumer
object handed in, whereas
Pals
handle its personal knowledge fetching and rendering logic
altogether. If we visualize the element tree, it will be one thing like
this:
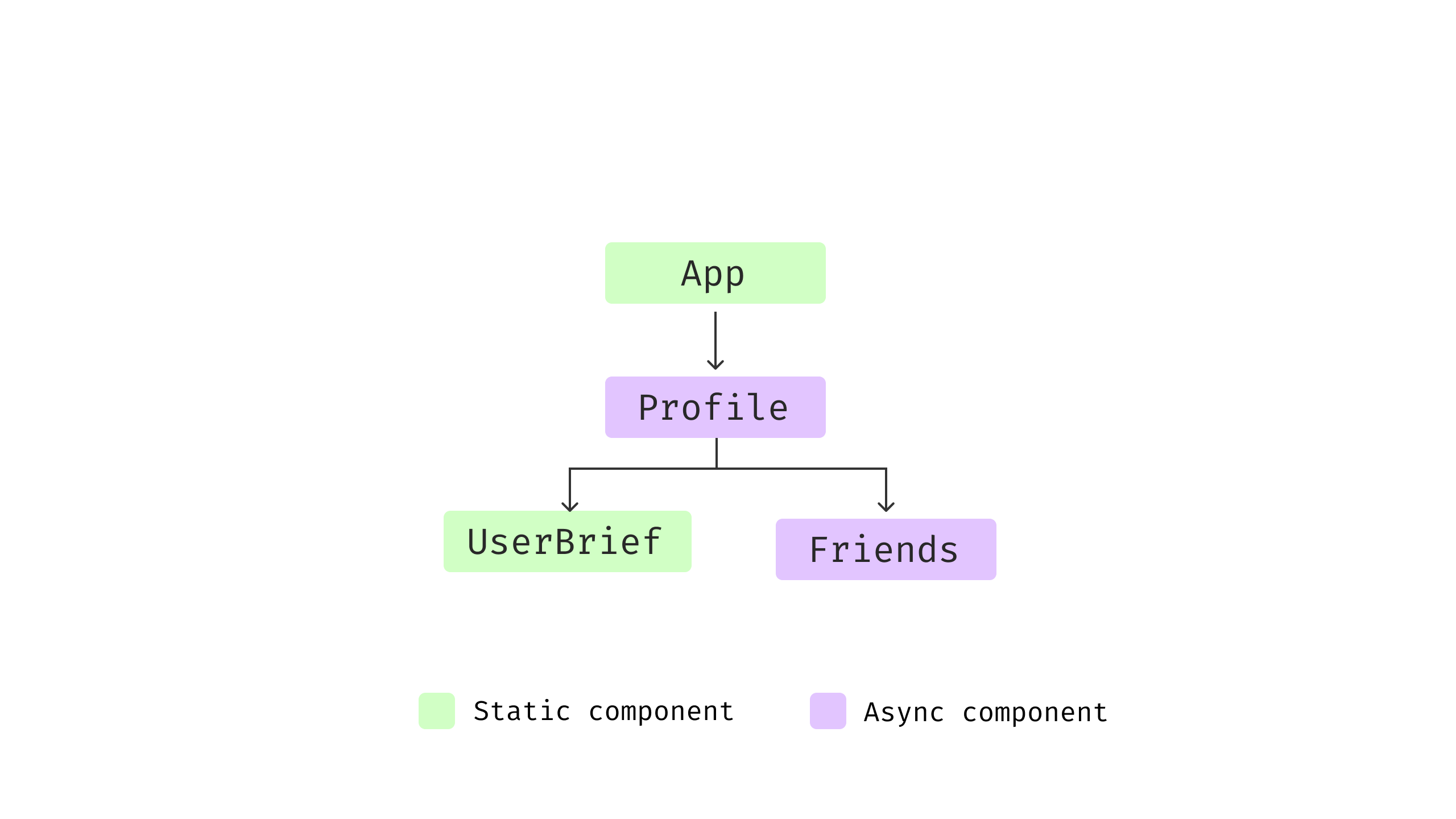
Determine 5: Element construction
Each the Profile
and Pals
have logic for
knowledge fetching, loading checks, and error dealing with. Since there are two
separate knowledge fetching calls, and if we have a look at the request timeline, we
will discover one thing fascinating.
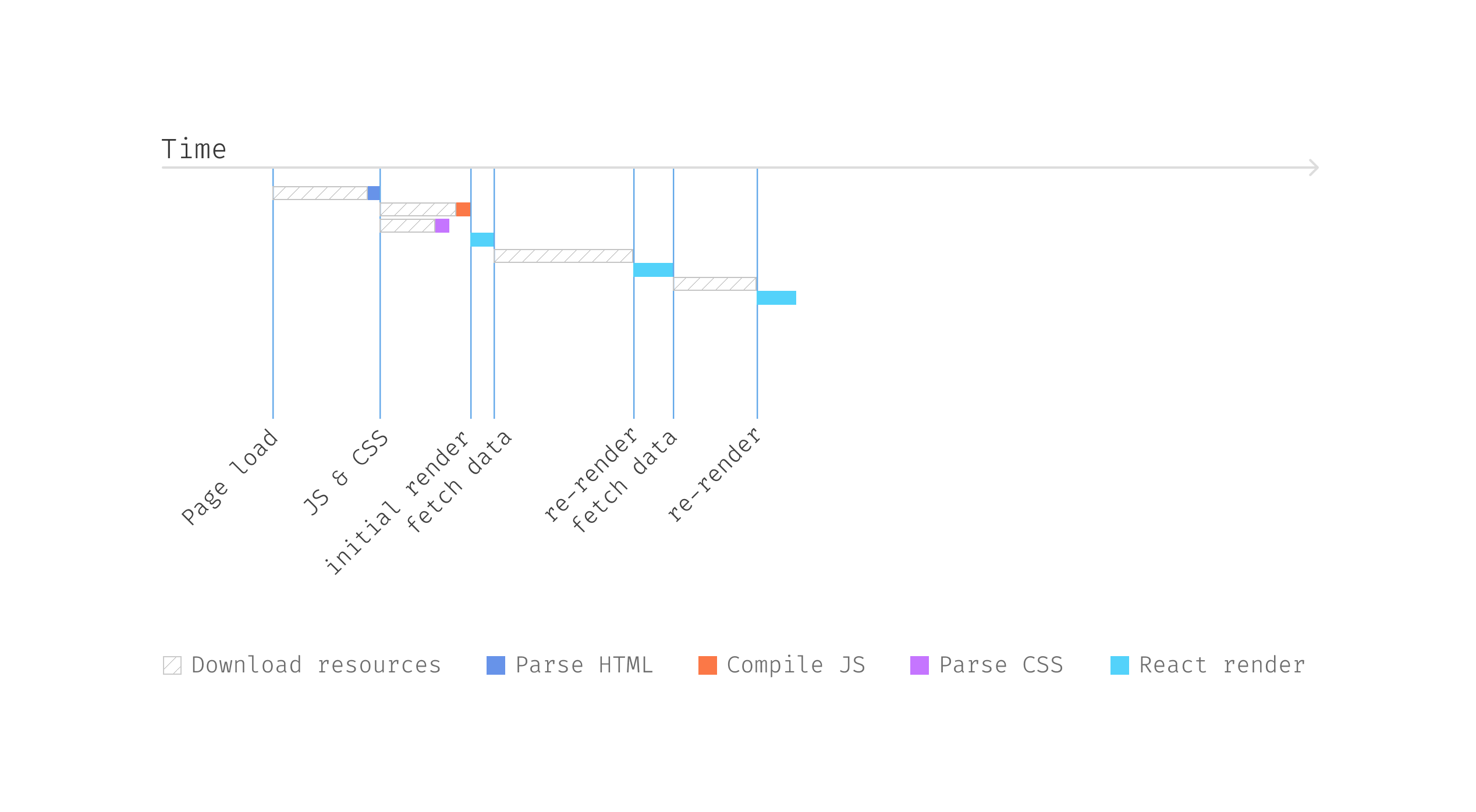
Determine 6: Request waterfall
The Pals
element will not provoke knowledge fetching till the consumer
state is ready. That is known as the Fetch-On-Render strategy,
the place the preliminary rendering is paused as a result of the information is not obtainable,
requiring React to attend for the information to be retrieved from the server
facet.
This ready interval is considerably inefficient, contemplating that whereas
React’s rendering course of solely takes a number of milliseconds, knowledge fetching can
take considerably longer, usually seconds. In consequence, the Pals
element spends most of its time idle, ready for knowledge. This state of affairs
results in a typical problem referred to as the Request Waterfall, a frequent
prevalence in frontend functions that contain a number of knowledge fetching
operations.
Parallel Information Fetching
Run distant knowledge fetches in parallel to reduce wait time
Think about after we construct a bigger utility {that a} element that
requires knowledge might be deeply nested within the element tree, to make the
matter worse these elements are developed by completely different groups, it’s onerous
to see whom we’re blocking.
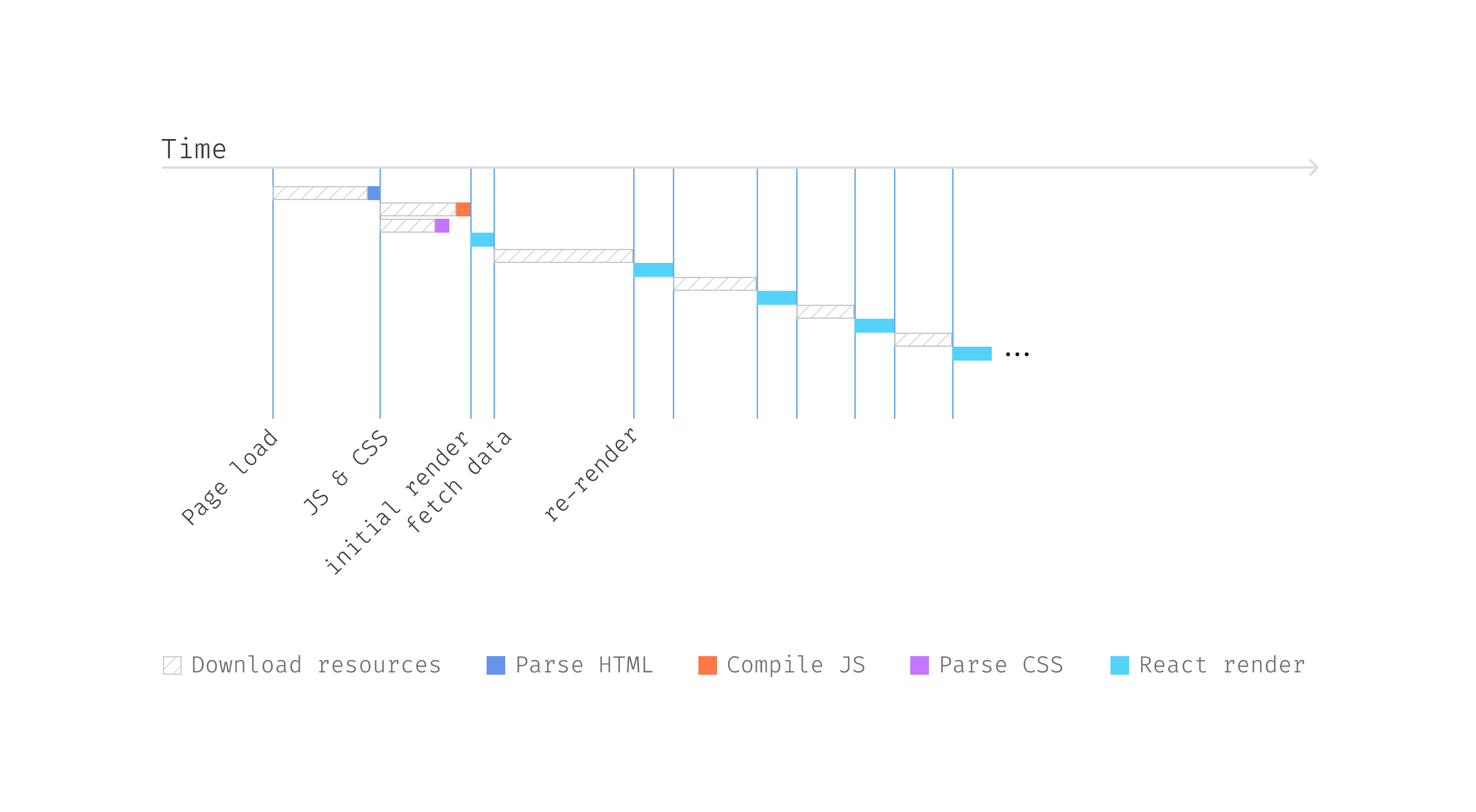
Determine 7: Request waterfall
Request Waterfalls can degrade consumer
expertise, one thing we intention to keep away from. Analyzing the information, we see that the
consumer API and associates API are impartial and might be fetched in parallel.
Initiating these parallel requests turns into vital for utility
efficiency.
One strategy is to centralize knowledge fetching at a better degree, close to the
root. Early within the utility’s lifecycle, we begin all knowledge fetches
concurrently. Parts depending on this knowledge wait just for the
slowest request, usually leading to sooner general load occasions.
We may use the Promise API Promise.all
to ship
each requests for the consumer’s fundamental data and their associates record.
Promise.all
is a JavaScript methodology that permits for the
concurrent execution of a number of guarantees. It takes an array of guarantees
as enter and returns a single Promise that resolves when all the enter
guarantees have resolved, offering their outcomes as an array. If any of the
guarantees fail, Promise.all
instantly rejects with the
cause of the primary promise that rejects.
As an example, on the utility’s root, we are able to outline a complete
knowledge mannequin:
sort ProfileState = { consumer: Person; associates: Person[]; }; const getProfileData = async (id: string) => Promise.all([ get<User>(`/users/${id}`), get<User[]>(`/customers/${id}/associates`), ]); const App = () => { // fetch knowledge on the very begining of the applying launch const onInit = () => { const [user, friends] = await getProfileData(id); } // render the sub tree correspondingly }
Implementing Parallel Information Fetching in React
Upon utility launch, knowledge fetching begins, abstracting the
fetching course of from subcomponents. For instance, in Profile element,
each UserBrief and Pals are presentational elements that react to
the handed knowledge. This manner we may develop these element individually
(including types for various states, for instance). These presentational
elements usually are simple to check and modify as we’ve separate the
knowledge fetching and rendering.
We are able to outline a customized hook useProfileData
that facilitates
parallel fetching of information associated to a consumer and their associates through the use of
Promise.all
. This methodology permits simultaneous requests, optimizing the
loading course of and structuring the information right into a predefined format identified
as ProfileData
.
Right here’s a breakdown of the hook implementation:
import { useCallback, useEffect, useState } from "react"; sort ProfileData = { consumer: Person; associates: Person[]; }; const useProfileData = (id: string) => { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(undefined); const [profileState, setProfileState] = useState<ProfileData>(); const fetchProfileState = useCallback(async () => { attempt { setLoading(true); const [user, friends] = await Promise.all([ get<User>(`/users/${id}`), get<User[]>(`/customers/${id}/associates`), ]); setProfileState({ consumer, associates }); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }, tag:martinfowler.com,2024-05-29:Prefetching-in-Single-Web page-Purposes); return { loading, error, profileState, fetchProfileState, }; };
This hook gives the Profile
element with the
crucial knowledge states (loading
, error
,
profileState
) together with a fetchProfileState
operate, enabling the element to provoke the fetch operation as
wanted. Notice right here we use useCallback
hook to wrap the async
operate for knowledge fetching. The useCallback hook in React is used to
memoize capabilities, making certain that the identical operate occasion is
maintained throughout element re-renders except its dependencies change.
Just like the useEffect, it accepts the operate and a dependency
array, the operate will solely be recreated if any of those dependencies
change, thereby avoiding unintended habits in React’s rendering
cycle.
The Profile
element makes use of this hook and controls the information fetching
timing by way of useEffect
:
const Profile = ({ id }: { id: string }) => { const { loading, error, profileState, fetchProfileState } = useProfileData(id); useEffect(() => { fetchProfileState(); }, [fetchProfileState]); if (loading) { return <div>Loading...</div>; } if (error) { return <div>One thing went flawed...</div>; } return ( <> {profileState && ( <> <UserBrief consumer={profileState.consumer} /> <Pals customers={profileState.associates} /> </> )} </> ); };
This strategy is also referred to as Fetch-Then-Render, suggesting that the intention
is to provoke requests as early as doable throughout web page load.
Subsequently, the fetched knowledge is utilized to drive React’s rendering of
the applying, bypassing the necessity to handle knowledge fetching amidst the
rendering course of. This technique simplifies the rendering course of,
making the code simpler to check and modify.
And the element construction, if visualized, could be just like the
following illustration
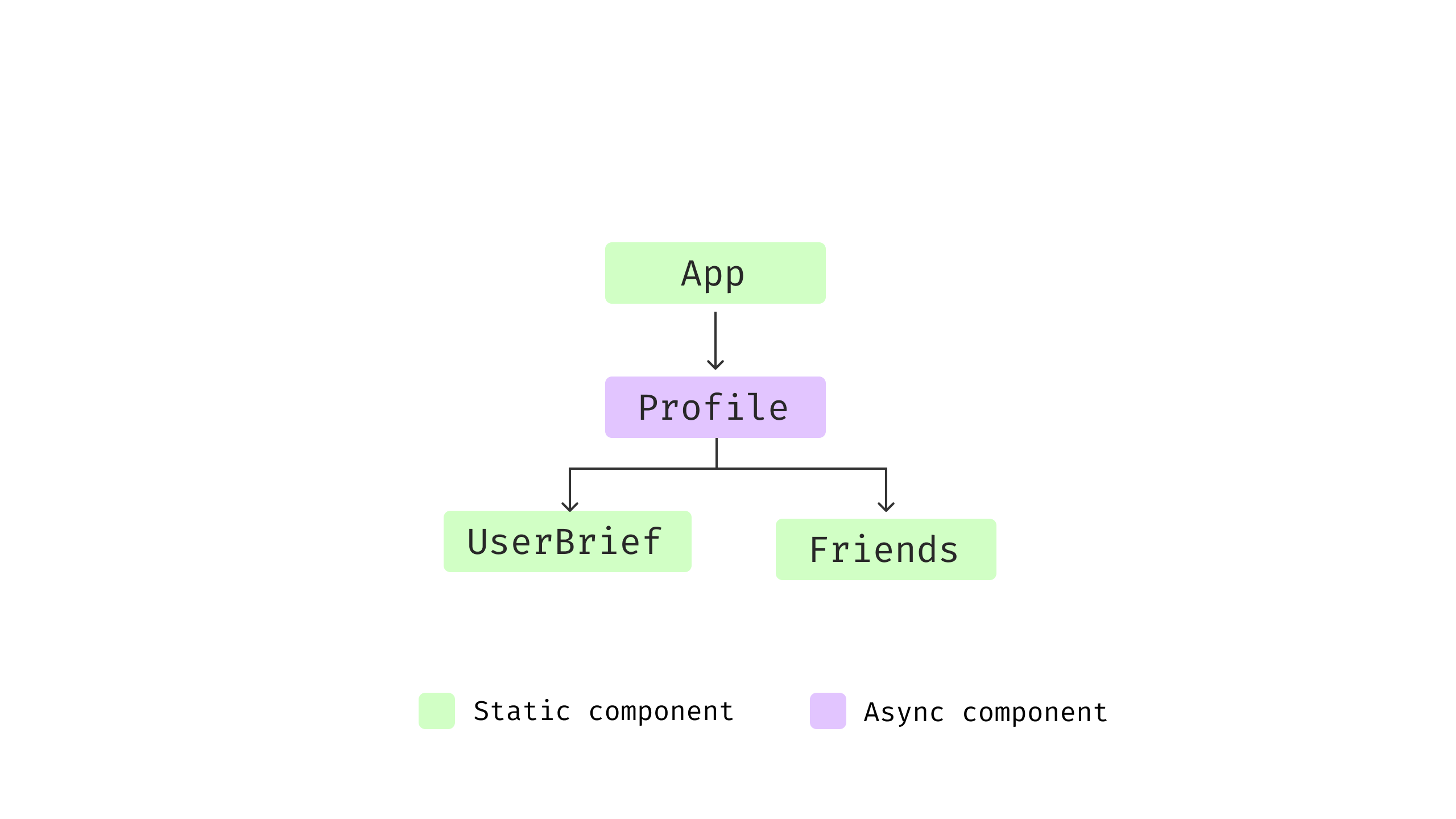
Determine 8: Element construction after refactoring
And the timeline is way shorter than the earlier one as we ship two
requests in parallel. The Pals
element can render in a number of
milliseconds as when it begins to render, the information is already prepared and
handed in.
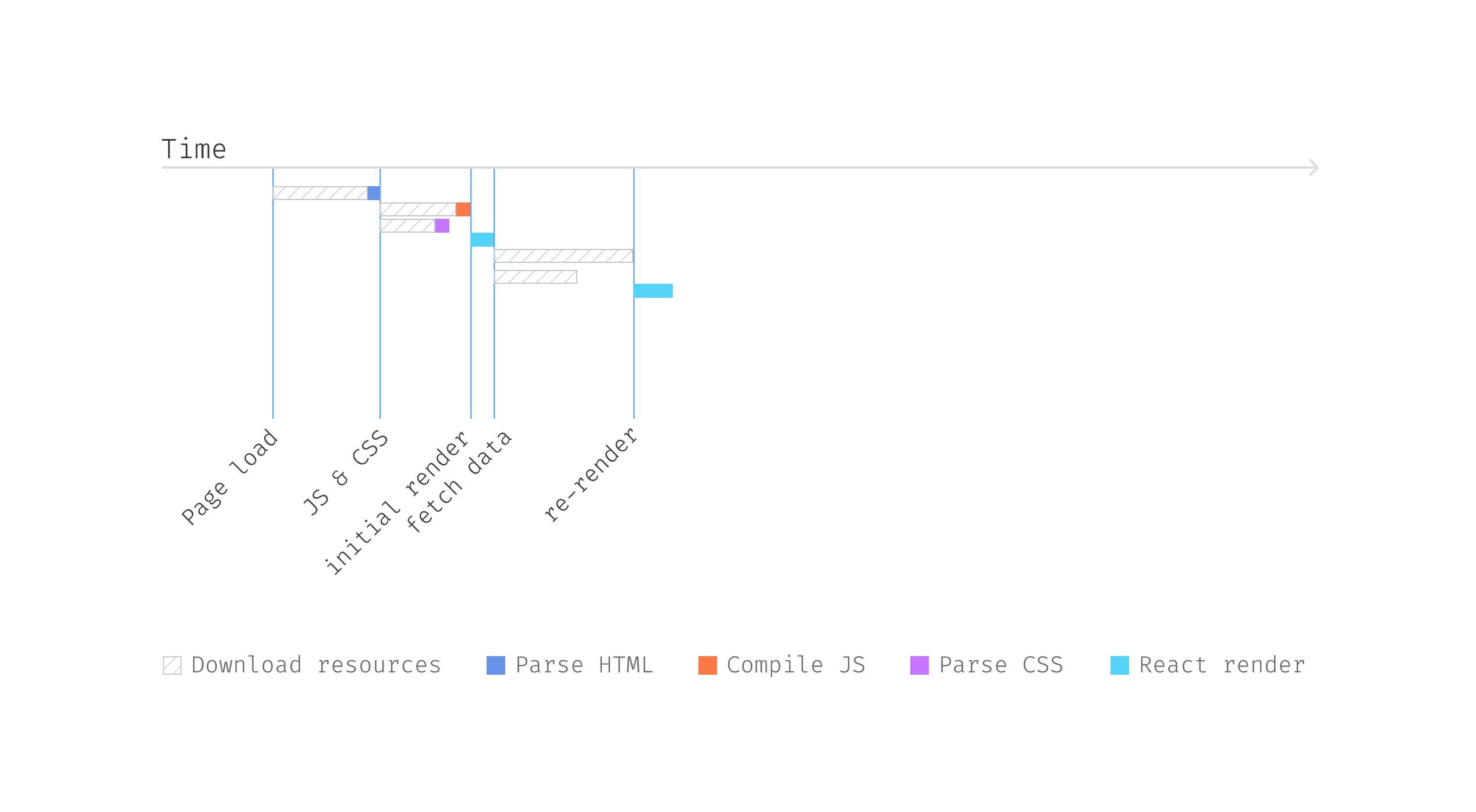
Determine 9: Parallel requests
Notice that the longest wait time will depend on the slowest community
request, which is way sooner than the sequential ones. And if we may
ship as many of those impartial requests on the similar time at an higher
degree of the element tree, a greater consumer expertise might be
anticipated.
As functions increase, managing an rising variety of requests at
root degree turns into difficult. That is significantly true for elements
distant from the foundation, the place passing down knowledge turns into cumbersome. One
strategy is to retailer all knowledge globally, accessible by way of capabilities (like
Redux or the React Context API), avoiding deep prop drilling.
When to make use of it
Operating queries in parallel is beneficial each time such queries could also be
gradual and do not considerably intrude with every others’ efficiency.
That is often the case with distant queries. Even when the distant
machine’s I/O and computation is quick, there’s at all times potential latency
points within the distant calls. The principle drawback for parallel queries
is setting them up with some type of asynchronous mechanism, which can be
troublesome in some language environments.
The principle cause to not use parallel knowledge fetching is after we do not
know what knowledge must be fetched till we have already fetched some
knowledge. Sure situations require sequential knowledge fetching because of
dependencies between requests. As an example, take into account a state of affairs on a
Profile
web page the place producing a personalised suggestion feed
will depend on first buying the consumer’s pursuits from a consumer API.
This is an instance response from the consumer API that features
pursuits:
{ "id": "u1", "title": "Juntao Qiu", "bio": "Developer, Educator, Creator", "pursuits": [ "Technology", "Outdoors", "Travel" ] }
In such instances, the advice feed can solely be fetched after
receiving the consumer’s pursuits from the preliminary API name. This
sequential dependency prevents us from using parallel fetching, as
the second request depends on knowledge obtained from the primary.
Given these constraints, it turns into necessary to debate various
methods in asynchronous knowledge administration. One such technique is
Fallback Markup. This strategy permits builders to specify what
knowledge is required and the way it needs to be fetched in a method that clearly
defines dependencies, making it simpler to handle advanced knowledge
relationships in an utility.
One other instance of when arallel Information Fetching will not be relevant is
that in situations involving consumer interactions that require real-time
knowledge validation.
Take into account the case of an inventory the place every merchandise has an “Approve” context
menu. When a consumer clicks on the “Approve” possibility for an merchandise, a dropdown
menu seems providing selections to both “Approve” or “Reject.” If this
merchandise’s approval standing could possibly be modified by one other admin concurrently,
then the menu choices should replicate essentially the most present state to keep away from
conflicting actions.
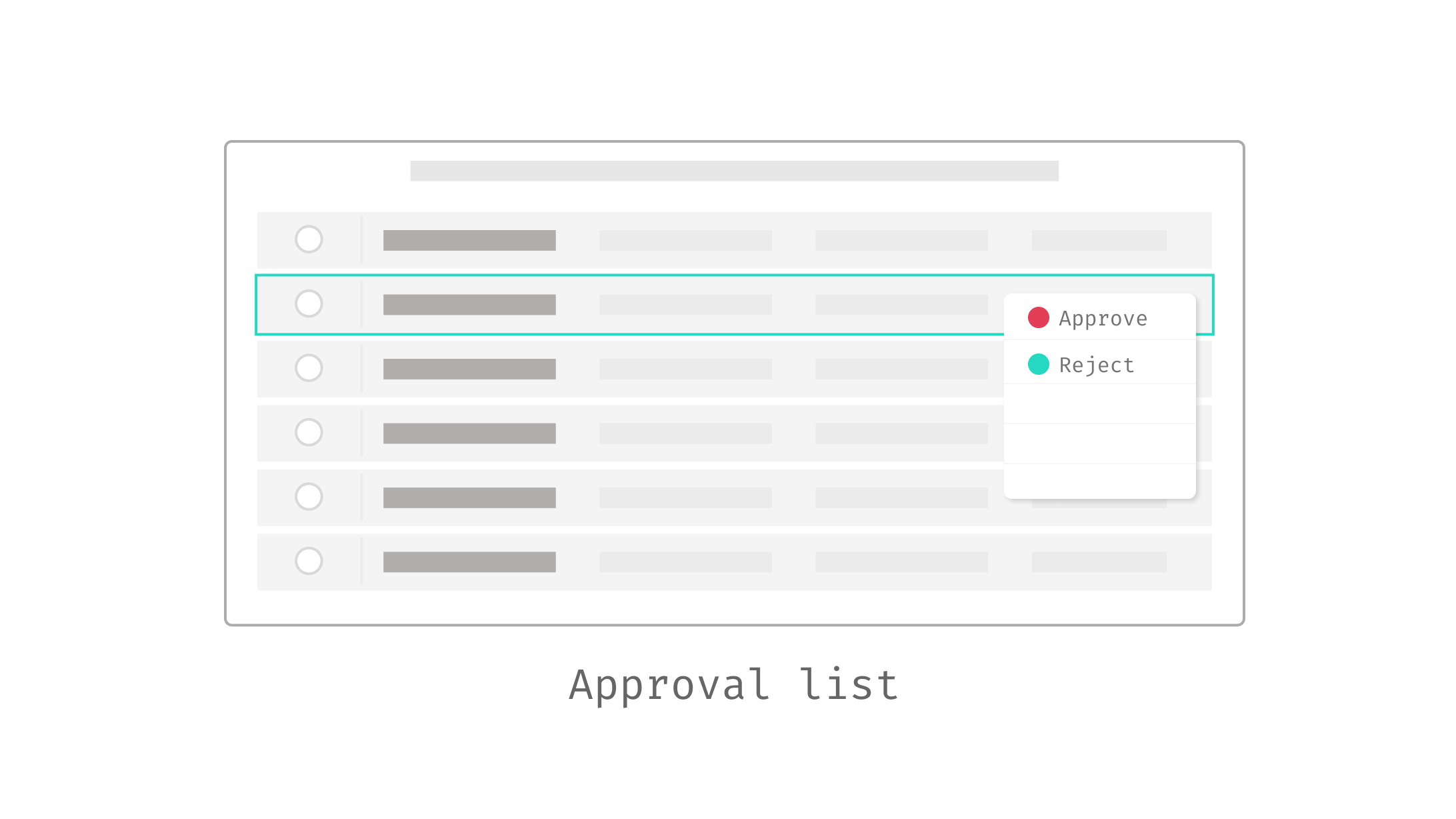
Determine 10: The approval record that require in-time
states
To deal with this, a service name is initiated every time the context
menu is activated. This service fetches the most recent standing of the merchandise,
making certain that the dropdown is constructed with essentially the most correct and
present choices obtainable at that second. In consequence, these requests
can’t be made in parallel with different data-fetching actions because the
dropdown’s contents rely solely on the real-time standing fetched from
the server.
Fallback Markup
Specify fallback shows within the web page markup
This sample leverages abstractions offered by frameworks or libraries
to deal with the information retrieval course of, together with managing states like
loading, success, and error, behind the scenes. It permits builders to
concentrate on the construction and presentation of information of their functions,
selling cleaner and extra maintainable code.
Let’s take one other have a look at the Pals
element within the above
part. It has to take care of three completely different states and register the
callback in useEffect
, setting the flag appropriately on the proper time,
prepare the completely different UI for various states:
const Pals = ({ id }: { id: string }) => { //... const { loading, error, knowledge: associates, fetch: fetchFriends, } = useService(`/customers/${id}/associates`); useEffect(() => { fetchFriends(); }, []); if (loading) { // present loading indicator } if (error) { // present error message element } // present the acutal good friend record };
You’ll discover that inside a element we’ve to cope with
completely different states, even we extract customized Hook to scale back the noise in a
element, we nonetheless must pay good consideration to dealing with
loading
and error
inside a element. These
boilerplate code might be cumbersome and distracting, usually cluttering the
readability of our codebase.
If we consider declarative API, like how we construct our UI with JSX, the
code might be written within the following method that permits you to concentrate on
what the element is doing – not learn how to do it:
<WhenError fallback={<ErrorMessage />}> <WhenInProgress fallback={<Loading />}> <Pals /> </WhenInProgress> </WhenError>
Within the above code snippet, the intention is straightforward and clear: when an
error happens, ErrorMessage
is displayed. Whereas the operation is in
progress, Loading is proven. As soon as the operation completes with out errors,
the Pals element is rendered.
And the code snippet above is fairly similiar to what already be
carried out in a number of libraries (together with React and Vue.js). For instance,
the brand new Suspense
in React permits builders to extra successfully handle
asynchronous operations inside their elements, bettering the dealing with of
loading states, error states, and the orchestration of concurrent
duties.
Implementing Fallback Markup in React with Suspense
Suspense
in React is a mechanism for effectively dealing with
asynchronous operations, similar to knowledge fetching or useful resource loading, in a
declarative method. By wrapping elements in a Suspense
boundary,
builders can specify fallback content material to show whereas ready for the
element’s knowledge dependencies to be fulfilled, streamlining the consumer
expertise throughout loading states.
Whereas with the Suspense API, within the Pals
you describe what you
need to get after which render:
import useSWR from "swr"; import { get } from "../utils.ts"; operate Pals({ id }: { id: string }) { const { knowledge: customers } = useSWR("/api/profile", () => get<Person[]>(`/customers/${id}/associates`), { suspense: true, }); return ( <div> <h2>Pals</h2> <div> {associates.map((consumer) => ( <Buddy consumer={consumer} key={consumer.id} /> ))} </div> </div> ); }
And declaratively whenever you use the Pals
, you utilize
Suspense
boundary to wrap across the Pals
element:
<Suspense fallback={<FriendsSkeleton />}> <Pals id={id} /> </Suspense>
Suspense
manages the asynchronous loading of the
Pals
element, exhibiting a FriendsSkeleton
placeholder till the element’s knowledge dependencies are
resolved. This setup ensures that the consumer interface stays responsive
and informative throughout knowledge fetching, bettering the general consumer
expertise.
Use the sample in Vue.js
It is value noting that Vue.js can be exploring the same
experimental sample, the place you may make use of Fallback Markup utilizing:
<Suspense> <template #default> <AsyncComponent /> </template> <template #fallback> Loading... </template> </Suspense>
Upon the primary render, <Suspense>
makes an attempt to render
its default content material behind the scenes. Ought to it encounter any
asynchronous dependencies throughout this part, it transitions right into a
pending state, the place the fallback content material is displayed as an alternative. As soon as all
the asynchronous dependencies are efficiently loaded,
<Suspense>
strikes to a resolved state, and the content material
initially supposed for show (the default slot content material) is
rendered.
Deciding Placement for the Loading Element
You could surprise the place to position the FriendsSkeleton
element and who ought to handle it. Usually, with out utilizing Fallback
Markup, this choice is easy and dealt with immediately inside the
element that manages the information fetching:
const Pals = ({ id }: { id: string }) => { // Information fetching logic right here... if (loading) { // Show loading indicator } if (error) { // Show error message element } // Render the precise good friend record };
On this setup, the logic for displaying loading indicators or error
messages is of course located inside the Pals
element. Nevertheless,
adopting Fallback Markup shifts this duty to the
element’s shopper:
<Suspense fallback={<FriendsSkeleton />}> <Pals id={id} /> </Suspense>
In real-world functions, the optimum strategy to dealing with loading
experiences relies upon considerably on the specified consumer interplay and
the construction of the applying. As an example, a hierarchical loading
strategy the place a mother or father element ceases to indicate a loading indicator
whereas its youngsters elements proceed can disrupt the consumer expertise.
Thus, it is essential to fastidiously take into account at what degree inside the
element hierarchy the loading indicators or skeleton placeholders
needs to be displayed.
Consider Pals
and FriendsSkeleton
as two
distinct element states—one representing the presence of information, and the
different, the absence. This idea is considerably analogous to utilizing a Speical Case sample in object-oriented
programming, the place FriendsSkeleton
serves because the ‘null’
state dealing with for the Pals
element.
The secret’s to find out the granularity with which you need to
show loading indicators and to take care of consistency in these
selections throughout your utility. Doing so helps obtain a smoother and
extra predictable consumer expertise.
When to make use of it
Utilizing Fallback Markup in your UI simplifies code by enhancing its readability
and maintainability. This sample is especially efficient when using
normal elements for numerous states similar to loading, errors, skeletons, and
empty views throughout your utility. It reduces redundancy and cleans up
boilerplate code, permitting elements to focus solely on rendering and
performance.
Fallback Markup, similar to React’s Suspense, standardizes the dealing with of
asynchronous loading, making certain a constant consumer expertise. It additionally improves
utility efficiency by optimizing useful resource loading and rendering, which is
particularly helpful in advanced functions with deep element bushes.
Nevertheless, the effectiveness of Fallback Markup will depend on the capabilities of
the framework you’re utilizing. For instance, React’s implementation of Suspense for
knowledge fetching nonetheless requires third-party libraries, and Vue’s help for
related options is experimental. Furthermore, whereas Fallback Markup can scale back
complexity in managing state throughout elements, it could introduce overhead in
less complicated functions the place managing state immediately inside elements may
suffice. Moreover, this sample could restrict detailed management over loading and
error states—conditions the place completely different error varieties want distinct dealing with may
not be as simply managed with a generic fallback strategy.
Introducing UserDetailCard element
Let’s say we want a characteristic that when customers hover on prime of a Buddy
,
we present a popup to allow them to see extra particulars about that consumer.
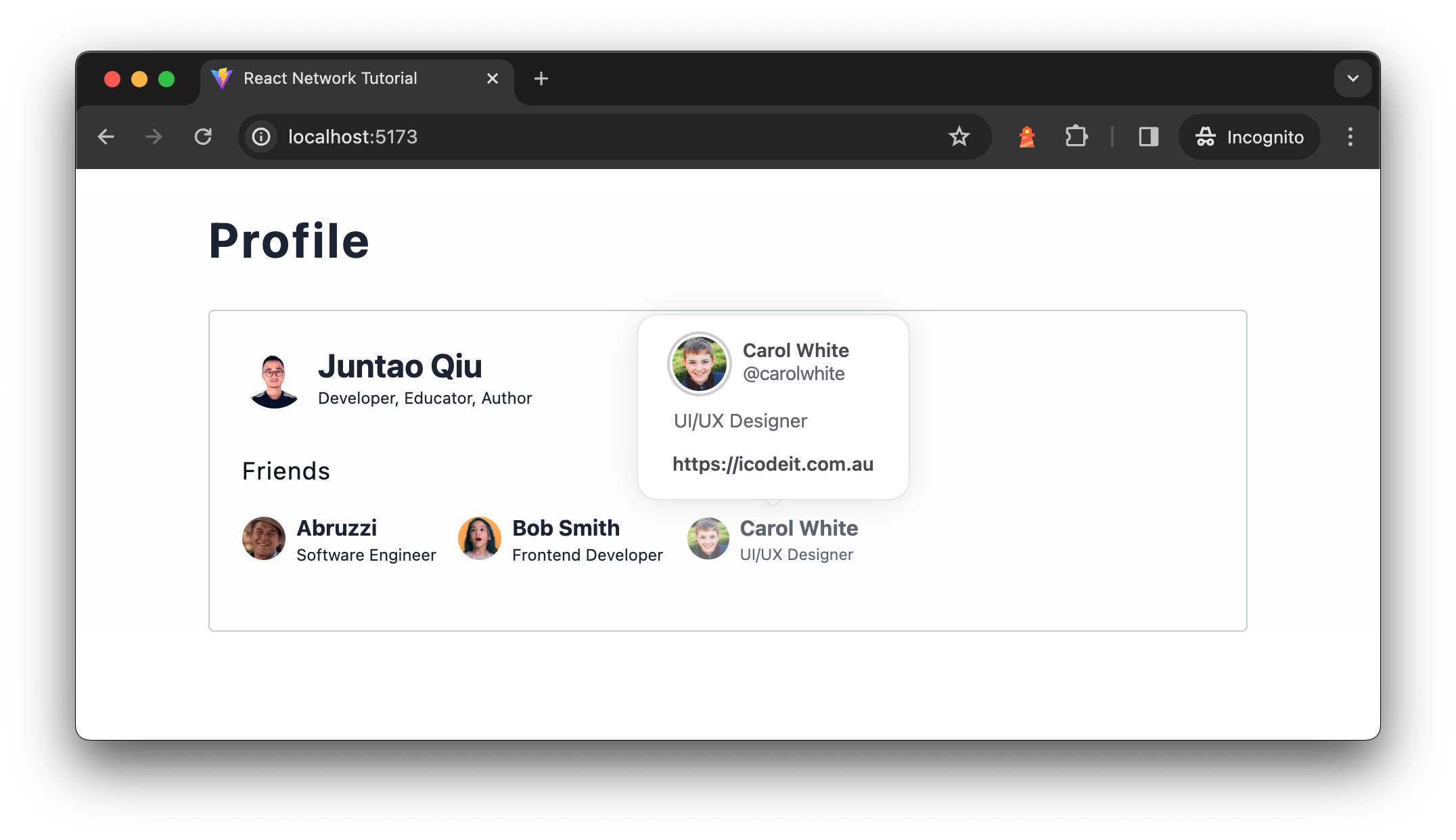
Determine 11: Exhibiting consumer element
card element when hover
When the popup exhibits up, we have to ship one other service name to get
the consumer particulars (like their homepage and variety of connections, and many others.). We
might want to replace the Buddy
element ((the one we use to
render every merchandise within the Pals record) ) to one thing just like the
following.
import { Popover, PopoverContent, PopoverTrigger } from "@nextui-org/react"; import { UserBrief } from "./consumer.tsx"; import UserDetailCard from "./user-detail-card.tsx"; export const Buddy = ({ consumer }: { consumer: Person }) => { return ( <Popover placement="backside" showArrow offset={10}> <PopoverTrigger> <button> <UserBrief consumer={consumer} /> </button> </PopoverTrigger> <PopoverContent> <UserDetailCard id={consumer.id} /> </PopoverContent> </Popover> ); };
The UserDetailCard
, is fairly much like the
Profile
element, it sends a request to load knowledge after which
renders the end result as soon as it will get the response.
export operate UserDetailCard({ id }: { id: string }) { const { loading, error, element } = useUserDetail(id); if (loading || !element) { return <div>Loading...</div>; } return ( <div> {/* render the consumer element*/} </div> ); }
We’re utilizing Popover
and the supporting elements from
nextui
, which gives a number of lovely and out-of-box
elements for constructing trendy UI. The one drawback right here, nonetheless, is that
the package deal itself is comparatively huge, additionally not everybody makes use of the characteristic
(hover and present particulars), so loading that further giant package deal for everybody
isn’t preferrred – it will be higher to load the UserDetailCard
on demand – each time it’s required.
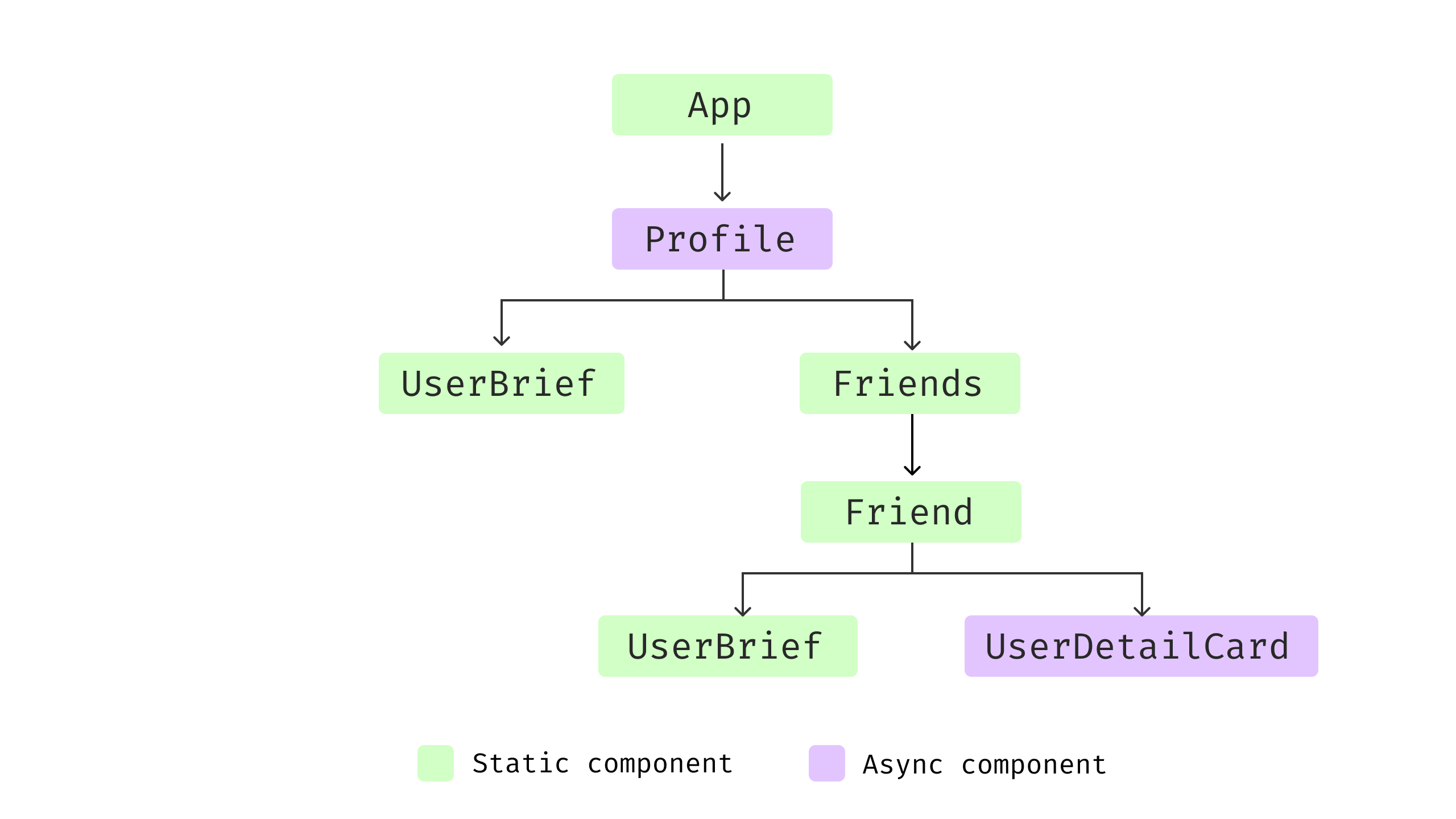
Determine 12: Element construction with
UserDetailCard
Code Splitting
Divide code into separate modules and dynamically load them as
wanted.
Code Splitting addresses the difficulty of enormous bundle sizes in net
functions by dividing the bundle into smaller chunks which are loaded as
wanted, moderately than abruptly. This improves preliminary load time and
efficiency, particularly necessary for big functions or these with
many routes.
This optimization is often carried out at construct time, the place advanced
or sizable modules are segregated into distinct bundles. These are then
dynamically loaded, both in response to consumer interactions or
preemptively, in a way that doesn’t hinder the vital rendering path
of the applying.
Leveraging the Dynamic Import Operator
The dynamic import operator in JavaScript streamlines the method of
loading modules. Although it could resemble a operate name in your code,
similar to import("./user-detail-card.tsx")
, it is necessary to
acknowledge that import
is definitely a key phrase, not a
operate. This operator permits the asynchronous and dynamic loading of
JavaScript modules.
With dynamic import, you may load a module on demand. For instance, we
solely load a module when a button is clicked:
button.addEventListener("click on", (e) => { import("/modules/some-useful-module.js") .then((module) => { module.doSomethingInteresting(); }) .catch(error => { console.error("Did not load the module:", error); }); });
The module will not be loaded throughout the preliminary web page load. As an alternative, the
import()
name is positioned inside an occasion listener so it solely
be loaded when, and if, the consumer interacts with that button.
You should utilize dynamic import operator in React and libraries like
Vue.js. React simplifies the code splitting and lazy load by means of the
React.lazy
and Suspense
APIs. By wrapping the
import assertion with React.lazy
, and subsequently wrapping
the element, as an example, UserDetailCard
, with
Suspense
, React defers the element rendering till the
required module is loaded. Throughout this loading part, a fallback UI is
introduced, seamlessly transitioning to the precise element upon load
completion.
import React, { Suspense } from "react"; import { Popover, PopoverContent, PopoverTrigger } from "@nextui-org/react"; import { UserBrief } from "./consumer.tsx"; const UserDetailCard = React.lazy(() => import("./user-detail-card.tsx")); export const Buddy = ({ consumer }: { consumer: Person }) => { return ( <Popover placement="backside" showArrow offset={10}> <PopoverTrigger> <button> <UserBrief consumer={consumer} /> </button> </PopoverTrigger> <PopoverContent> <Suspense fallback={<div>Loading...</div>}> <UserDetailCard id={consumer.id} /> </Suspense> </PopoverContent> </Popover> ); };
This snippet defines a Buddy
element displaying consumer
particulars inside a popover from Subsequent UI, which seems upon interplay.
It leverages React.lazy
for code splitting, loading the
UserDetailCard
element solely when wanted. This
lazy-loading, mixed with Suspense
, enhances efficiency
by splitting the bundle and exhibiting a fallback throughout the load.
If we visualize the above code, it renders within the following
sequence.
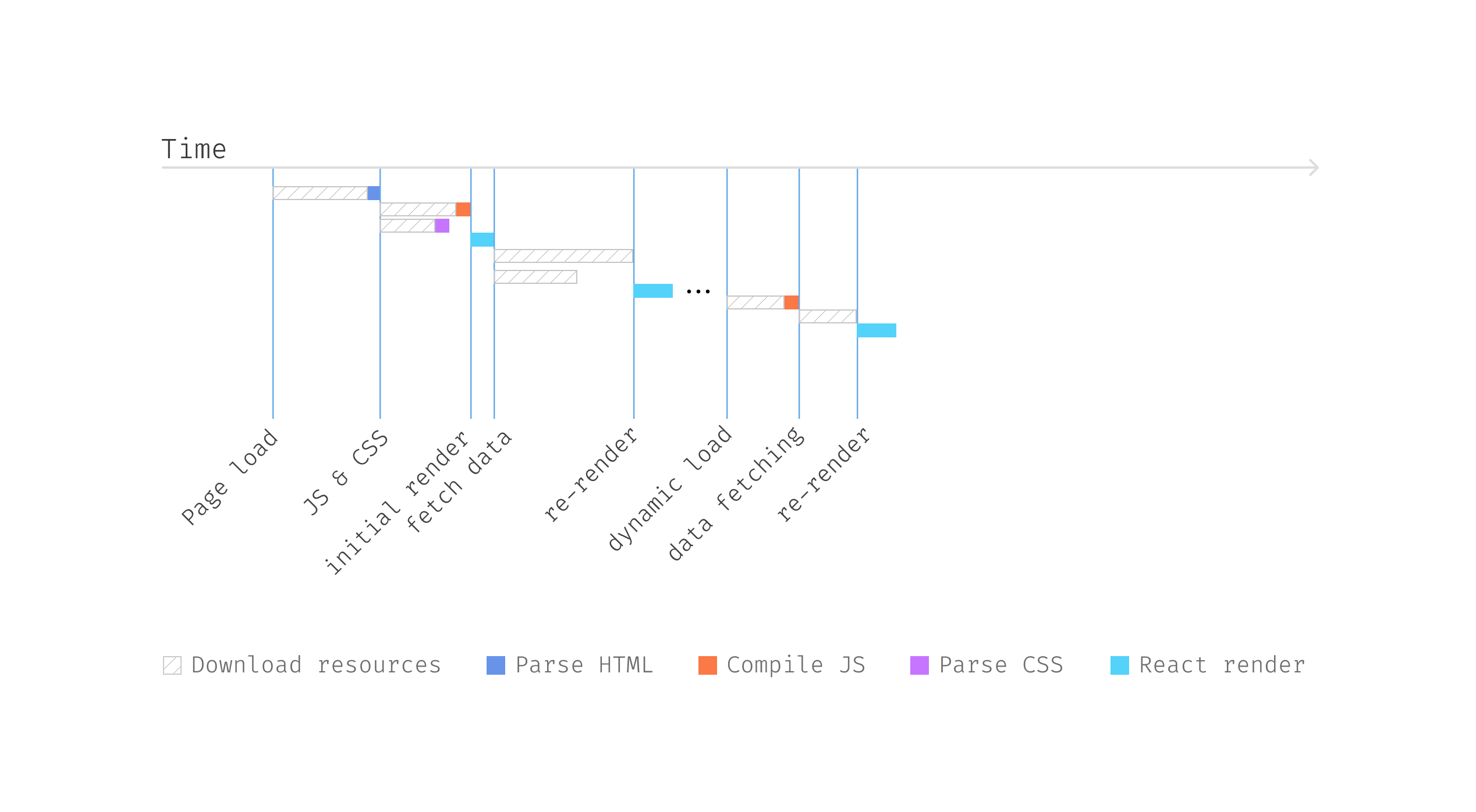
Determine 13: Dynamic load element
when wanted
Notice that when the consumer hovers and we obtain
the JavaScript bundle, there can be some further time for the browser to
parse the JavaScript. As soon as that a part of the work is completed, we are able to get the
consumer particulars by calling /customers/<id>/particulars
API.
Ultimately, we are able to use that knowledge to render the content material of the popup
UserDetailCard
.
When to make use of it
Splitting out further bundles and loading them on demand is a viable
technique, nevertheless it’s essential to contemplate the way you implement it. Requesting
and processing a further bundle can certainly save bandwidth and lets
customers solely load what they want. Nevertheless, this strategy may also gradual
down the consumer expertise in sure situations. For instance, if a consumer
hovers over a button that triggers a bundle load, it may take a number of
seconds to load, parse, and execute the JavaScript crucial for
rendering. Although this delay happens solely throughout the first
interplay, it won’t present the perfect expertise.
To enhance perceived efficiency, successfully utilizing React Suspense to
show a skeleton or one other loading indicator can assist make the
loading course of appear faster. Moreover, if the separate bundle is
not considerably giant, integrating it into the primary bundle could possibly be a
extra easy and cost-effective strategy. This manner, when a consumer
hovers over elements like UserBrief
, the response might be
fast, enhancing the consumer interplay with out the necessity for separate
loading steps.
Lazy load in different frontend libraries
Once more, this sample is extensively adopted in different frontend libraries as
nicely. For instance, you should utilize defineAsyncComponent
in Vue.js to
obtain the samiliar end result – solely load a element whenever you want it to
render:
<template> <Popover placement="backside" show-arrow offset="10"> <!-- the remainder of the template --> </Popover> </template> <script> import { defineAsyncComponent } from 'vue'; import Popover from 'path-to-popover-component'; import UserBrief from './UserBrief.vue'; const UserDetailCard = defineAsyncComponent(() => import('./UserDetailCard.vue')); // rendering logic </script>
The operate defineAsyncComponent
defines an async
element which is lazy loaded solely when it’s rendered similar to the
React.lazy
.
As you might need already seen the observed, we’re working right into a Request Waterfall right here once more: we load the
JavaScript bundle first, after which when it execute it sequentially name
consumer particulars API, which makes some further ready time. We may request
the JavaScript bundle and the community request parallely. That means,
each time a Buddy
element is hovered, we are able to set off a
community request (for the information to render the consumer particulars) and cache the
end result, in order that by the point when the bundle is downloaded, we are able to use
the information to render the element instantly.
Prefetching
Prefetch knowledge earlier than it could be wanted to scale back latency whether it is.
Prefetching includes loading assets or knowledge forward of their precise
want, aiming to lower wait occasions throughout subsequent operations. This
method is especially helpful in situations the place consumer actions can
be predicted, similar to navigating to a special web page or displaying a modal
dialog that requires distant knowledge.
In observe, prefetching might be
carried out utilizing the native HTML <hyperlink>
tag with a
rel="preload"
attribute, or programmatically by way of the
fetch
API to load knowledge or assets upfront. For knowledge that
is predetermined, the best strategy is to make use of the
<hyperlink>
tag inside the HTML <head>
:
<!doctype html> <html lang="en"> <head> <hyperlink rel="preload" href="https://martinfowler.com/bootstrap.js" as="script"> <hyperlink rel="preload" href="https://martinfowler.com/customers/u1" as="fetch" crossorigin="nameless"> <hyperlink rel="preload" href="https://martinfowler.com/customers/u1/associates" as="fetch" crossorigin="nameless"> <script sort="module" src="https://martinfowler.com/app.js"></script> </head> <physique> <div id="root"></div> </physique> </html>
With this setup, the requests for bootstrap.js
and consumer API are despatched
as quickly because the HTML is parsed, considerably sooner than when different
scripts are processed. The browser will then cache the information, making certain it
is prepared when your utility initializes.
Nevertheless, it is usually not doable to know the exact URLs forward of
time, requiring a extra dynamic strategy to prefetching. That is usually
managed programmatically, usually by means of occasion handlers that set off
prefetching based mostly on consumer interactions or different circumstances.
For instance, attaching a mouseover
occasion listener to a button can
set off the prefetching of information. This methodology permits the information to be fetched
and saved, maybe in a neighborhood state or cache, prepared for fast use
when the precise element or content material requiring the information is interacted with
or rendered. This proactive loading minimizes latency and enhances the
consumer expertise by having knowledge prepared forward of time.
doc.getElementById('button').addEventListener('mouseover', () => { fetch(`/consumer/${consumer.id}/particulars`) .then(response => response.json()) .then(knowledge => { sessionStorage.setItem('userDetails', JSON.stringify(knowledge)); }) .catch(error => console.error(error)); });
And within the place that wants the information to render, it reads from
sessionStorage
when obtainable, in any other case exhibiting a loading indicator.
Usually the consumer experiense could be a lot sooner.
Implementing Prefetching in React
For instance, we are able to use preload
from the
swr
package deal (the operate title is a bit deceptive, nevertheless it
is performing a prefetch right here), after which register an
onMouseEnter
occasion to the set off element of
Popover
,
import { preload } from "swr"; import { getUserDetail } from "../api.ts"; const UserDetailCard = React.lazy(() => import("./user-detail-card.tsx")); export const Buddy = ({ consumer }: { consumer: Person }) => { const handleMouseEnter = () => { preload(`/consumer/${consumer.id}/particulars`, () => getUserDetail(consumer.id)); }; return ( <Popover placement="backside" showArrow offset={10}> <PopoverTrigger> <button onMouseEnter={handleMouseEnter}> <UserBrief consumer={consumer} /> </button> </PopoverTrigger> <PopoverContent> <Suspense fallback={<div>Loading...</div>}> <UserDetailCard id={consumer.id} /> </Suspense> </PopoverContent> </Popover> ); };
That method, the popup itself can have a lot much less time to render, which
brings a greater consumer expertise.
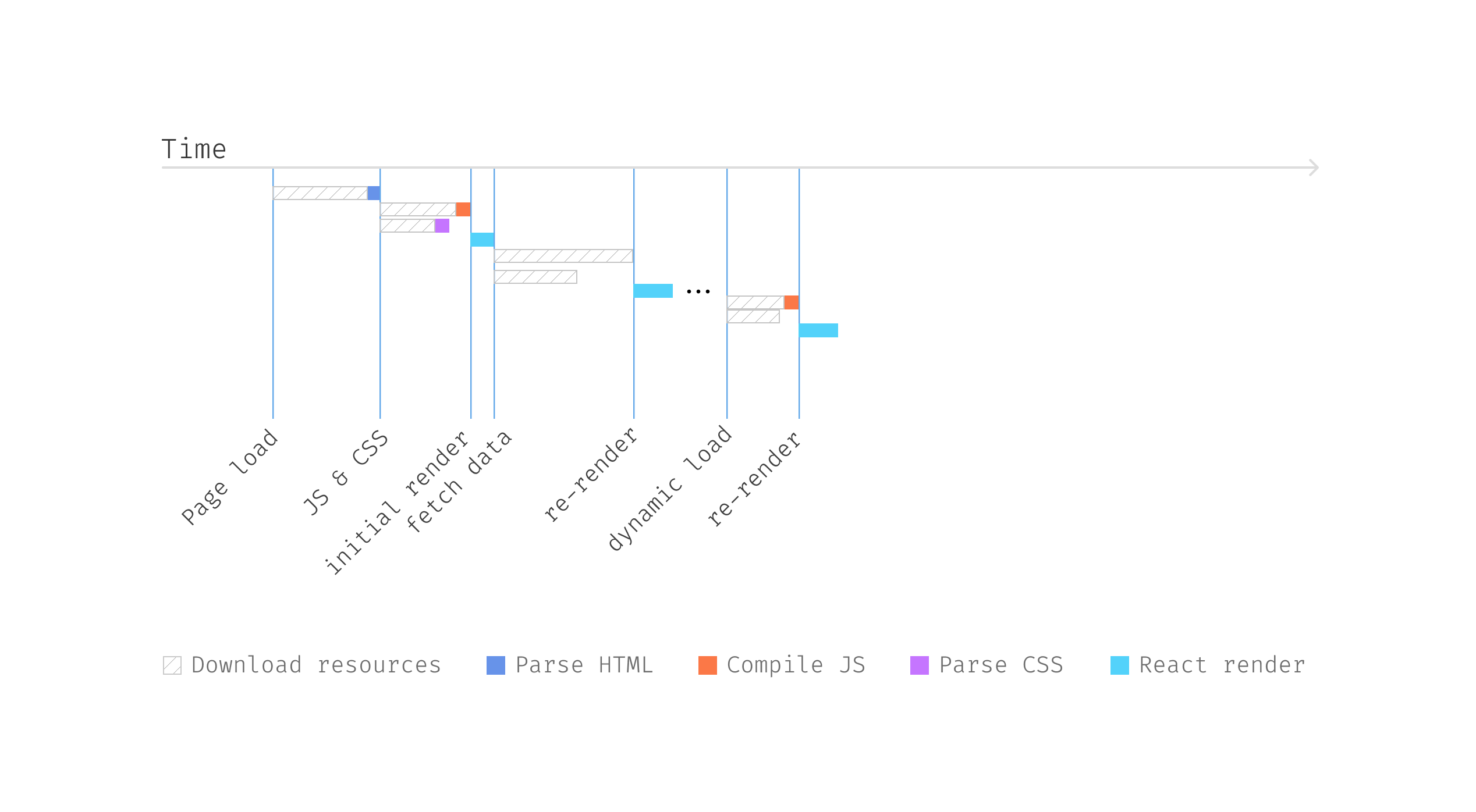
Determine 14: Dynamic load with prefetch
in parallel
So when a consumer hovers on a Buddy
, we obtain the
corresponding JavaScript bundle in addition to obtain the information wanted to
render the UserDetailCard, and by the point UserDetailCard
renders, it sees the present knowledge and renders instantly.
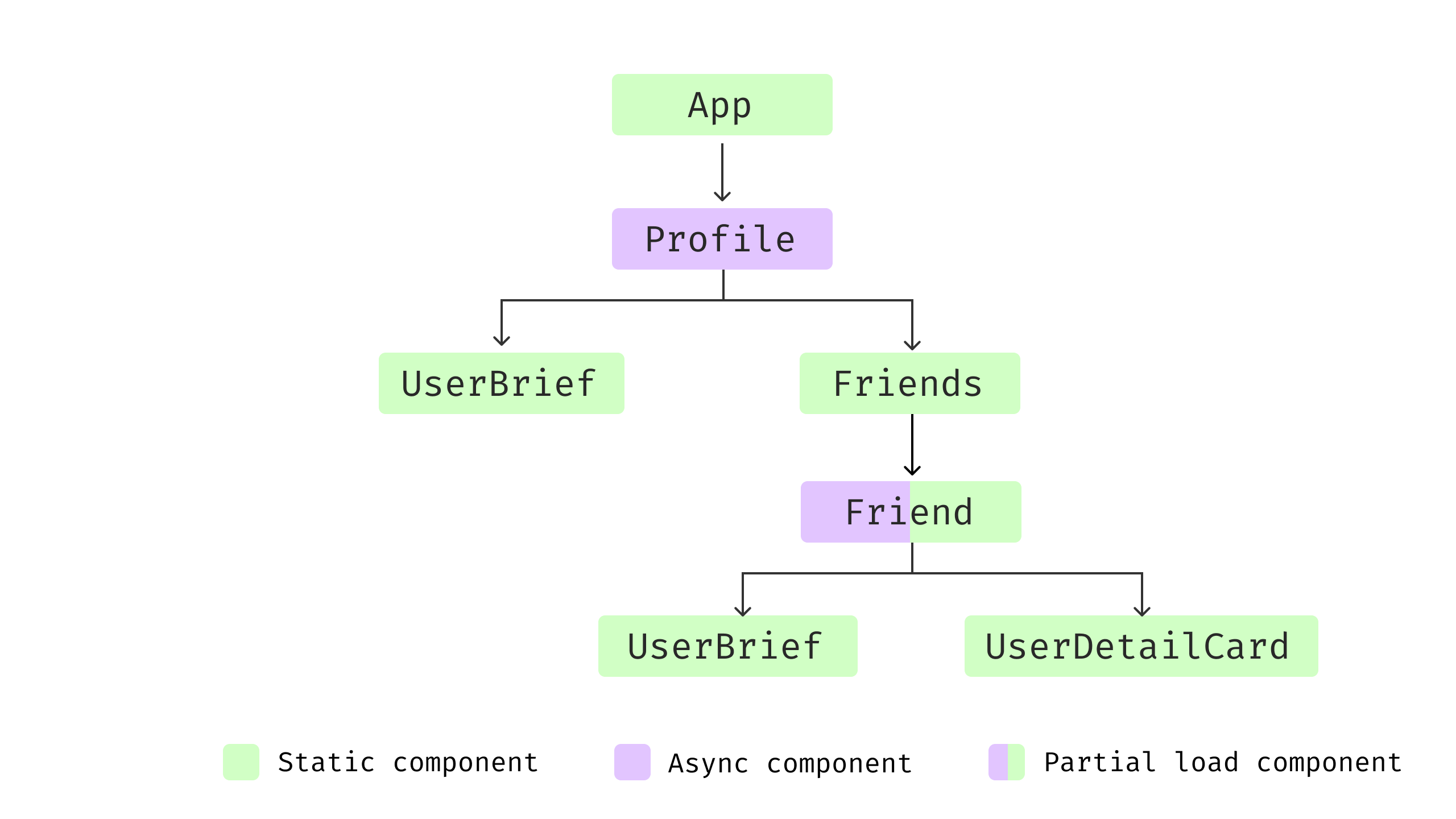
Determine 15: Element construction with
dynamic load
As the information fetching and loading is shifted to Buddy
element, and for UserDetailCard
, it reads from the native
cache maintained by swr
.
import useSWR from "swr"; export operate UserDetailCard({ id }: { id: string }) { const { knowledge: element, isLoading: loading } = useSWR( `/consumer/${id}/particulars`, () => getUserDetail(id) ); if (loading || !element) { return <div>Loading...</div>; } return ( <div> {/* render the consumer element*/} </div> ); }
This element makes use of the useSWR
hook for knowledge fetching,
making the UserDetailCard
dynamically load consumer particulars
based mostly on the given id
. useSWR
affords environment friendly
knowledge fetching with caching, revalidation, and automated error dealing with.
The element shows a loading state till the information is fetched. As soon as
the information is obtainable, it proceeds to render the consumer particulars.
In abstract, we have already explored vital knowledge fetching methods:
Asynchronous State Handler , Parallel Information Fetching ,
Fallback Markup , Code Splitting and Prefetching . Elevating requests for parallel execution
enhances effectivity, although it is not at all times easy, particularly
when coping with elements developed by completely different groups with out full
visibility. Code splitting permits for the dynamic loading of
non-critical assets based mostly on consumer interplay, like clicks or hovers,
using prefetching to parallelize useful resource loading.
When to make use of it
Take into account making use of prefetching whenever you discover that the preliminary load time of
your utility is turning into gradual, or there are a lot of options that are not
instantly crucial on the preliminary display however could possibly be wanted shortly after.
Prefetching is especially helpful for assets which are triggered by consumer
interactions, similar to mouse-overs or clicks. Whereas the browser is busy fetching
different assets, similar to JavaScript bundles or property, prefetching can load
extra knowledge upfront, thus making ready for when the consumer really must
see the content material. By loading assets throughout idle occasions, prefetching makes use of the
community extra effectively, spreading the load over time moderately than inflicting spikes
in demand.
It’s sensible to comply with a normal guideline: do not implement advanced patterns like
prefetching till they’re clearly wanted. This is perhaps the case if efficiency
points turn into obvious, particularly throughout preliminary hundreds, or if a big
portion of your customers entry the app from cellular gadgets, which generally have
much less bandwidth and slower JavaScript engines. Additionally, take into account that there are different
efficiency optimization techniques similar to caching at numerous ranges, utilizing CDNs
for static property, and making certain property are compressed. These strategies can improve
efficiency with less complicated configurations and with out extra coding. The
effectiveness of prefetching depends on precisely predicting consumer actions.
Incorrect assumptions can result in ineffective prefetching and even degrade the
consumer expertise by delaying the loading of really wanted assets.
Selecting the best sample
Deciding on the suitable sample for knowledge fetching and rendering in
net growth will not be one-size-fits-all. Typically, a number of methods are
mixed to fulfill particular necessities. For instance, you may must
generate some content material on the server facet – utilizing Server-Facet Rendering
methods – supplemented by client-side
Fetch-Then-Render for dynamic
content material. Moreover, non-essential sections might be break up into separate
bundles for lazy loading, probably with Prefetching triggered by consumer
actions, similar to hover or click on.
Take into account the Jira difficulty web page for instance. The highest navigation and
sidebar are static, loading first to provide customers fast context. Early
on, you are introduced with the difficulty’s title, description, and key particulars
just like the Reporter and Assignee. For much less fast data, similar to
the Historical past part at a problem’s backside, it hundreds solely upon consumer
interplay, like clicking a tab. This makes use of lazy loading and knowledge
fetching to effectively handle assets and improve consumer expertise.
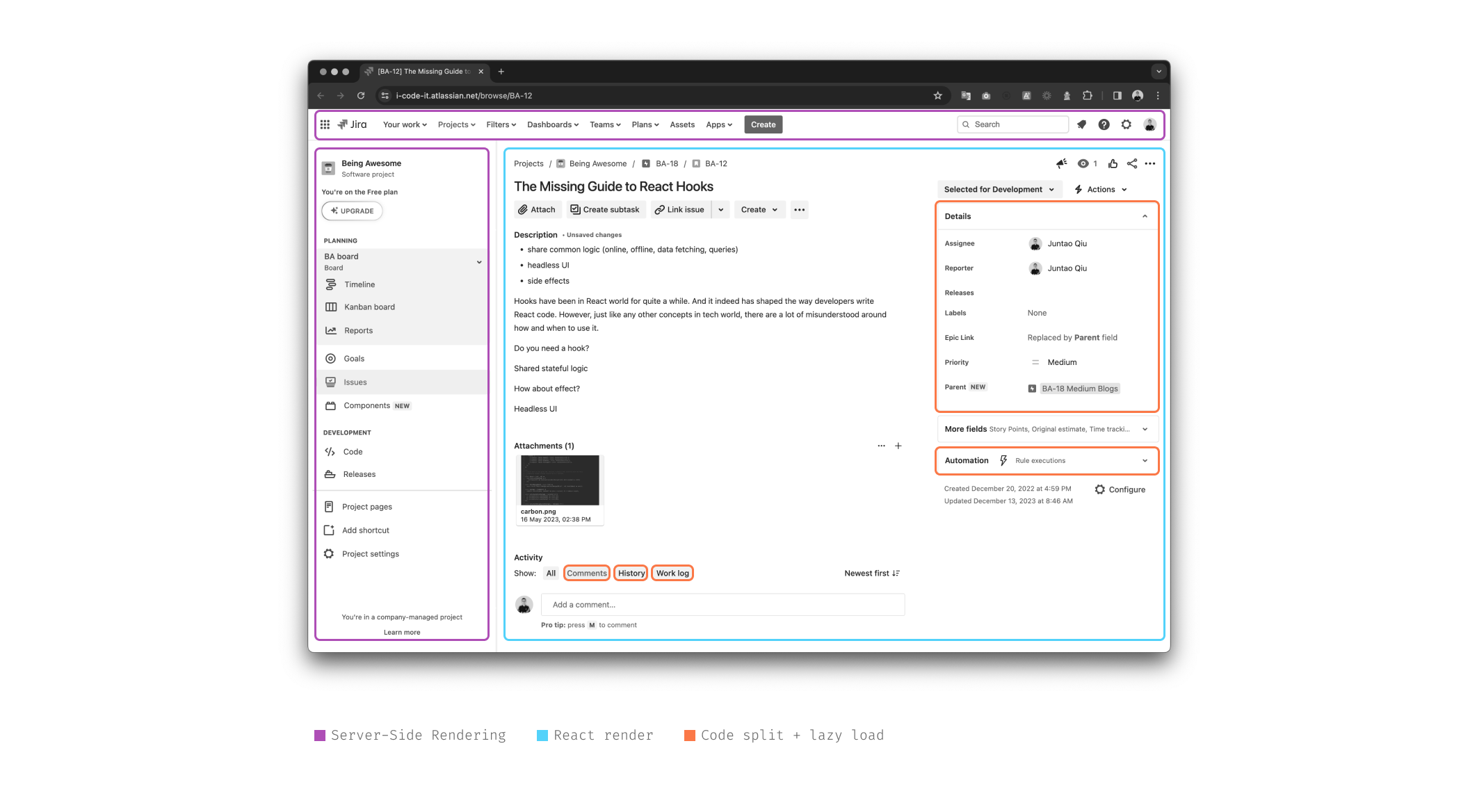
Determine 16: Utilizing patterns collectively
Furthermore, sure methods require extra setup in comparison with
default, much less optimized options. As an example, implementing Code Splitting requires bundler help. In case your present bundler lacks this
functionality, an improve could also be required, which could possibly be impractical for
older, much less secure techniques.
We have coated a variety of patterns and the way they apply to numerous
challenges. I understand there’s fairly a bit to soak up, from code examples
to diagrams. In case you’re searching for a extra guided strategy, I’ve put
collectively a complete tutorial on my
web site, or when you solely need to take a look on the working code, they’re
all hosted on this github repo.
Conclusion
Information fetching is a nuanced facet of growth, but mastering the
applicable methods can vastly improve our functions. As we conclude
our journey by means of knowledge fetching and content material rendering methods inside
the context of React, it is essential to focus on our most important insights:
- Asynchronous State Handler: Make the most of customized hooks or composable APIs to
summary knowledge fetching and state administration away out of your elements. This
sample centralizes asynchronous logic, simplifying element design and
enhancing reusability throughout your utility. - Fallback Markup: React’s enhanced Suspense mannequin helps a extra
declarative strategy to fetching knowledge asynchronously, streamlining your
codebase. - Parallel Information Fetching: Maximize effectivity by fetching knowledge in
parallel, decreasing wait occasions and boosting the responsiveness of your
utility. - Code Splitting: Make use of lazy loading for non-essential
elements throughout the preliminary load, leveraging Suspense for sleek
dealing with of loading states and code splitting, thereby making certain your
utility stays performant. - Prefetching: By preemptively loading knowledge based mostly on predicted consumer
actions, you may obtain a clean and quick consumer expertise.
Whereas these insights had been framed inside the React ecosystem, it is
important to acknowledge that these patterns should not confined to React
alone. They’re broadly relevant and helpful methods that may—and
ought to—be tailored to be used with different libraries and frameworks. By
thoughtfully implementing these approaches, builders can create
functions that aren’t simply environment friendly and scalable, but additionally provide a
superior consumer expertise by means of efficient knowledge fetching and content material
rendering practices.