Mixins
, HOC
, render props,
and Hooks
are 4 methods to reuse elements
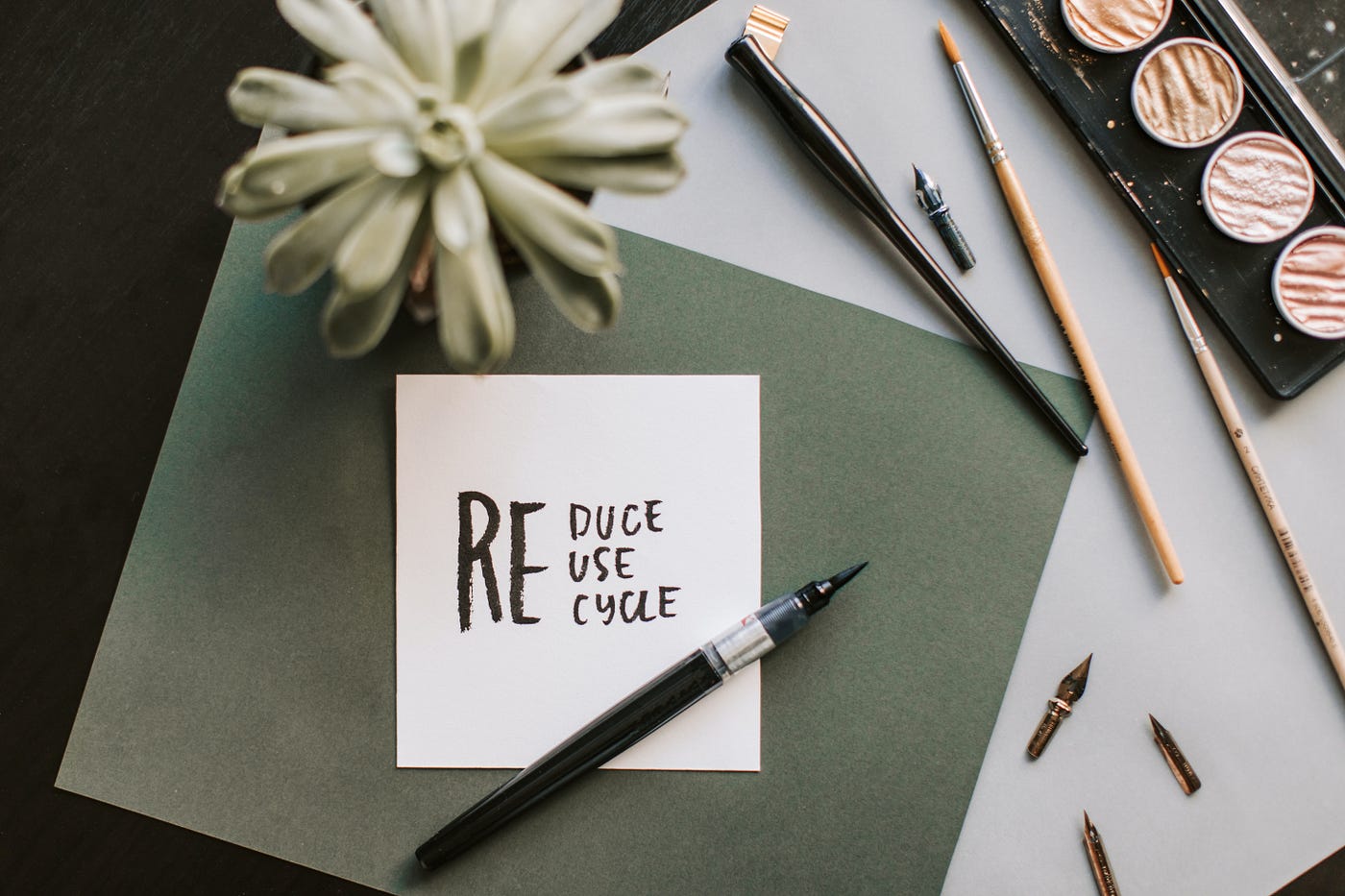
Now frontend engineering is an increasing number of essential. Though Ctrl+C and Ctrl+V may also be used to finish necessities, as soon as they’re modified, it turns into an enormous process. Subsequently, copying of code is diminished, and the packaging and reuse capabilities are elevated to realize maintainability and reversibility. The code used turns into significantly essential.
In React, elements are the principle unit of code reuse. The mix-based element reuse mechanism is kind of elegant, however for extra fine-grained logic (state logic, conduct logic, and so on.), reuse isn’t really easy. It’s troublesome to disassemble the state logic as a reusable operate or element. In reality, earlier than the looks of Hooks, there was a scarcity of a easy and direct manner of element conduct extension, which is taken into account to be mixins, higher-order elements (HOC), and render props. The upper-level mannequin explored below the present (element mechanism) sport guidelines has not solved the issue of logic reuse between elements from the basis. That is my thirty eighth Medium article.
After all, React not recommends utilizing mixins as a reuse resolution for a very long time, however it may well nonetheless present assist for mixins by way of create-react-class
. Observe that mixins usually are not supported when declaring elements in ES6 courses.
Mixins permit a number of React elements to share code. They’re similar to mixins in Python or traits in PHP. The emergence of the mixin resolution comes from an OOP instinct. Within the early days, it solely offered React.createClass()
API to outline elements. (In React v15.5.0, it’s formally deserted and moved to create-react-class
). Naturally, (class) inheritance has develop into an intuitive try, and in JavaScript
prototype-based extension mode, it’s much like the inherited mixin
scheme. It has develop into a great resolution. Mixin
is especially used to unravel the reuse drawback of life cycle logic and state logic, and permits the element life cycle to be prolonged from the surface. That is particularly essential in Flux
and different modes, however many defects have additionally appeared in steady observe:
- There’s an implicit dependency between the element and the
mixin
(Mixin
typically relies on the precise technique of the element, however the dependency isn’t identified when the element is outlined). - There could also be conflicts between a number of
mixin
(similar to defining the identicalstate
subject). Mixin
tends so as to add extra states, which reduces the predictability of the applying and results in a pointy enhance in complexity.- Implicit dependencies result in opaque dependencies, and upkeep prices and understanding prices are rising quickly.
- It’s troublesome to shortly perceive the conduct of elements, and it’s essential to completely perceive all of the extension behaviors that depend on
mixin
and their mutual affect. - The tactic and
state
subject of the element itself is afraid to be simply deleted as a result of it’s troublesome to find out whether or notmixin
relies on it. Mixin
can also be troublesome to take care of, as a result ofMixin
logic will ultimately be flattened and merged collectively, and it’s troublesome to determine the enter and output of aMixin
.
There isn’t a doubt that these issues are deadly, so Reactv0.13.0
deserted Mixin
static crosscutting (much like inherited reuse) and moved to HOC
higher-order elements (much like mixed reuse).
Instance
The instance of the traditional model, a standard state of affairs is: A element must be up to date frequently. It’s simple to do it with setInterval(), however it is extremely essential to cancel the timer when it’s not wanted to save lots of reminiscence. React offers a lifecycle technique to tell the element. The time of creation or destruction, the next Mixin, use setInterval() and be certain that the timer is cleaned up when the element is destroyed.
After Mixin
, HOC high-order elements tackle the heavy duty and develop into the really useful resolution for logical reuse between elements. Excessive-order elements reveal a high-order ambiance from their names. In reality, this idea needs to be derived from high-order capabilities of JavaScript
. The high-order operate is a operate that accepts a operate as enter or output. It may be thought that currying is a higher-order operate. The definition of higher-order elements can also be given within the React
doc. Greater-order elements obtain elements and return new elements. operate. The precise that means is: Excessive-order elements will be seen as an implementation of React
ornament sample. Excessive-order elements are a operate, and the operate accepts a element as a parameter and returns a brand new element. It should return an enhanced React
elements. Excessive-order elements could make our code extra reusable, logical and summary, can hijack the render
technique, and may also management props
and state
.
Evaluating Mixin
and HOC
, Mixin
is a mixed-in mode. In precise use, Mixin
continues to be very highly effective, permitting us to share the identical technique in a number of elements, however it is going to additionally proceed so as to add new strategies and attributes to the elements. The element itself can’t solely understand but in addition must do associated processing (similar to naming conflicts, state upkeep, and so on.). As soon as the blended modules enhance, the whole element turns into troublesome to take care of. Mixin
might introduce invisible attributes, similar to within the Mixin
technique used within the rendering element brings invisible property props
and states
to the element. Mixin
might rely on one another and is coupled with one another, which isn’t conducive to code upkeep. As well as, the strategies in numerous Mixin
might battle with one another. Beforehand React
formally really useful utilizing Mixin
to unravel issues associated to cross-cutting issues, however as a result of utilizing Mixin
might trigger extra hassle, the official advice is now to make use of HOC
. Excessive-order element HOC
belong to the thought of purposeful programming
. The wrapped elements won’t concentrate on the existence of high-order elements, and the elements returned by high-order elements can have a purposeful enhancement impact on the unique elements. Primarily based on this, React
formally recommends using high-order elements.
Though HOC
doesn’t have so many deadly issues, it additionally has some minor flaws:
- Scalability restriction:
HOC
can not utterly changeMixin
. In some situations,Mixin
can howeverHOC
can not. For instance,PureRenderMixin
, as a result ofHOC
can not entry theState
of subcomponents from the surface, and on the similar time filter out pointless updates by way ofshouldComponentUpdate
. Subsequently,React
After supportingES6Class
,React.PureComponent
is offered to unravel this drawback. Ref
switch drawback:Ref
is minimize off. The switch drawback ofRef
is kind of annoying below the layers of packaging. The operateRef
can alleviate a part of it (permittingHOC
to study node creation and destruction), so theReact.forwardRef API
API was launched later.WrapperHell
:HOC
is flooded, andWrapperHell
seems (there is no such thing as a drawback that can’t be solved by one layer, if there’s, then two layers). Multi-layer abstraction additionally will increase complexity and value of understanding. That is probably the most essential defect. InHOC
mode There isn’t a good resolution.
Instance
Particularly, a high-order element is a operate whose parameter is a element and the return worth is a brand new element. A element converts props
right into a UI
however a high-order element converts a element into one other element. HOC
is quite common in React
third-party libraries, similar to Redux
’s join
and Relay
’s createFragmentContainer
.
Consideration needs to be paid right here, don’t attempt to modify the element prototype within the HOC
in any manner, however ought to use the mix technique to understand the operate by packaging the element within the container element. Below regular circumstances, there are two methods to implement high-order elements:
- Property agent
Props Proxy
. - Reverse inheritance
Inheritance Inversion
.
Property Agent
For instance, we will add a saved id
attribute worth to the incoming element. We will add a props
to this element by way of high-order elements. After all, we will additionally function on the props
within the WrappedComponent
element in JSX
. Observe that it’s not to govern the incoming WrappedComponent
class, we must always circuitously modify the incoming element, however can function on it within the technique of mixture.
We will additionally use high-order elements to load the state of recent elements into the packaged elements. For instance, we will use high-order elements to transform uncontrolled elements into managed elements.
Or our function is to wrap it with different elements to realize the aim of format or fashion.
Reverse inheritance
Reverse inheritance signifies that the returned element inherits the earlier element. In reverse inheritance, we will do a whole lot of operations, modify state
, props
and even flip the Aspect Tree
. There is a crucial level within the reverse inheritance that reverse inheritance can not be certain that the whole sub-component tree is parsed. Which means if the parsed component tree comprises elements (operate
kind or Class
kind), the sub-components of the element can not be manipulated.
Once we use reverse inheritance to implement high-order elements, we will management rendering by way of rendering hijacking. Particularly, we will consciously management the rendering technique of WrappedComponent
to regulate the outcomes of rendering management. For instance, we will resolve whether or not to render elements in line with some parameters.
We will even hijack the life cycle of the unique element by rewriting.
Since it’s really an inheritance relationship, we will learn the props
and state
of the element. If essential, we will even add, modify, and delete the props
and state
. After all, the premise is that the dangers attributable to the modification should be managed by your self. In some circumstances, we might must cross in some parameters for the high-order attributes, then we will cross within the parameters within the type of currying, and cooperate with the high-order elements to finish the operation much like the closure of the element.
notice
Don’t change the unique elements
Don’t attempt to modify the element prototype in HOC
, or change it in different methods.
Doing so can have some undesirable penalties. One is that the enter element can not be used as earlier than the HOC
enhancement. What’s extra severe is that in case you use one other HOC
that additionally modifies componentDidUpdate
to reinforce it, the earlier HOC
will probably be invalid, and this HOC
can’t be utilized to purposeful elements that haven’t any life cycle.
Modifying the HOC
of the incoming element is a foul abstraction, and the caller should know the way they’re carried out to keep away from conflicts with different HOC
. HOC
shouldn’t modify the incoming elements, however ought to use a mix of elements to realize capabilities by packaging the elements in container elements.
Filter props
HOC
provides options to elements and shouldn’t considerably change the conference itself. The elements returned by HOC
ought to keep related interfaces with the unique elements. HOC
ought to transparently transmit props
that don’t have anything to do with itself, and most HOC
ought to embrace a render
technique much like the next.
Most composability
Not all HOCs
are the identical. Generally it solely accepts one parameter, which is the packaged element.
const NavbarWithRouter = withRouter(Navbar);
HOC
can often obtain a number of parameters. For instance, in Relay
, HOC moreover receives a configuration object to specify the info dependency of the element.
const CommentWithRelay = Relay.createContainer(Remark, config);
The commonest HOC signatures are as follows, join is a higher-order operate that returns higher-order elements.
This kind could seem complicated or pointless, nevertheless it has a helpful property, just like the single-parameter HOC
returned by the join
operate has the signature Part => Part
, and capabilities with the identical output kind and enter kind will be simply mixed. The identical attributes additionally permit join
and different HOCs
to imagine the position of decorator. As well as, many third-party libraries present compose device capabilities, together with lodash
, Redux
, and Ramda
.
Don’t use HOC within the render technique
React
’s diff
algorithm makes use of the element identifier to find out whether or not it ought to replace the present subtree or discard it and mount the brand new subtree. If the element returned from the render
is similar because the element within the earlier render ===
, React
passes The subtree is distinguished from the brand new subtree to recursively replace the subtree, and if they don’t seem to be equal, the earlier subtree is totally unloaded.
Often, you don’t want to contemplate this when utilizing it, however it is extremely essential for HOC
, as a result of it signifies that you shouldn’t apply HOC
to a element within the render
technique of the element.
This isn’t only a efficiency concern. Re-mounting the element will trigger the state of the element and all its subcomponents to be misplaced. If the HOC
is created outdoors the element, the element will solely be created as soon as. So each time you render
it is going to be the identical element. Typically talking, that is constant together with your anticipated efficiency. In uncommon circumstances, it is advisable name HOC
dynamically, you may name it within the element’s lifecycle technique or its constructor.
You’ll want to copy static strategies
Generally it’s helpful to outline static strategies on React
elements. For instance, the Relay
container exposes a static technique getFragment
to facilitate the composition of GraphQL
fragments. However once you apply HOC
to a element, the unique element will probably be packaged with a container element, which signifies that the brand new element doesn’t have any static strategies of the unique element.
To unravel this drawback, you may copy these strategies to the container element earlier than returning.
However to do that, it is advisable know which strategies needs to be copied. You should utilize hoist-non-react-statics
to routinely copy all non-React
static strategies.
Along with exporting elements, one other possible resolution is to moreover export this static technique.
Refs won’t be handed
Though the conference of high-level elements is to cross all props
to the packaged element, this doesn’t apply to refs
, as a result of ref
isn’t really a prop
, similar to a key
, it’s particularly dealt with by React
. If the ref
is added to the return element of the HOC
, the ref
reference factors to the container element, not the packaged element. This drawback will be explicitly forwarded to the inner element by way of the React.forwardRefAPI
refs
.