One widespread query in SwiftUI app improvement is the best way to work with Core Knowledge to save lots of information completely within the built-in database. Regardless of Appleās ongoing efforts to simplify the APIs of Core Knowledge, new comers usually discover the framework difficult to make use of. Nevertheless, there’s excellent news on the horizon. Apple will probably be releasing a brand new framework referred to as SwiftData in iOS 17 to exchange Core Knowledge. SwiftData is designed to be a lot simpler to make use of for information modelling and administration, providing a extra user-friendly method.
Whatās SwiftData
At the beginning, itās vital to notice that the SwiftData framework shouldn’t be confused with a database. Constructed on prime of Core Knowledge, SwiftData is definitely a framework designed to assist builders handle and work together with information on a persistent retailer. Whereas the default persistent retailer for iOS is often the SQLite database, itās price noting that persistent shops can take different types as nicely. For instance, Core Knowledge can be used to handle information in an area file, equivalent to an XML file.
No matter whether or not youāre utilizing Core Knowledge or the SwiftData framework, each instruments serve to protect builders from the complexities of the underlying persistent retailer. Think about the SQLite database, for example. With SwiftData, thereās no want to fret about connecting to the database or understanding SQL in an effort to retrieve information data. As an alternative, builders can deal with working with APIs and Swift Macros, equivalent to @Question
and @Mannequin
, to successfully handle information of their purposes.
The SwiftData framework is newly launched in iOS 17 to exchange the earlier framework referred to as Core Knowledge. Core Knowledge has lengthy been the information administration APIs for iOS improvement because the period of Goal-C. Regardless that builders can combine the framework into Swift tasks, Core Knowledge isn’t a local resolution for each Swift and SwiftUI.
In iOS 17, Apple lastly introduces a local framework referred to as SwiftData for Swift on persistent information administration and information modeling. Itās constructed on prime of Core Knowledge however the APIs are fully redesigned to take advantage of out of Swift.
Utilizing Code to Create the Knowledge Mannequin
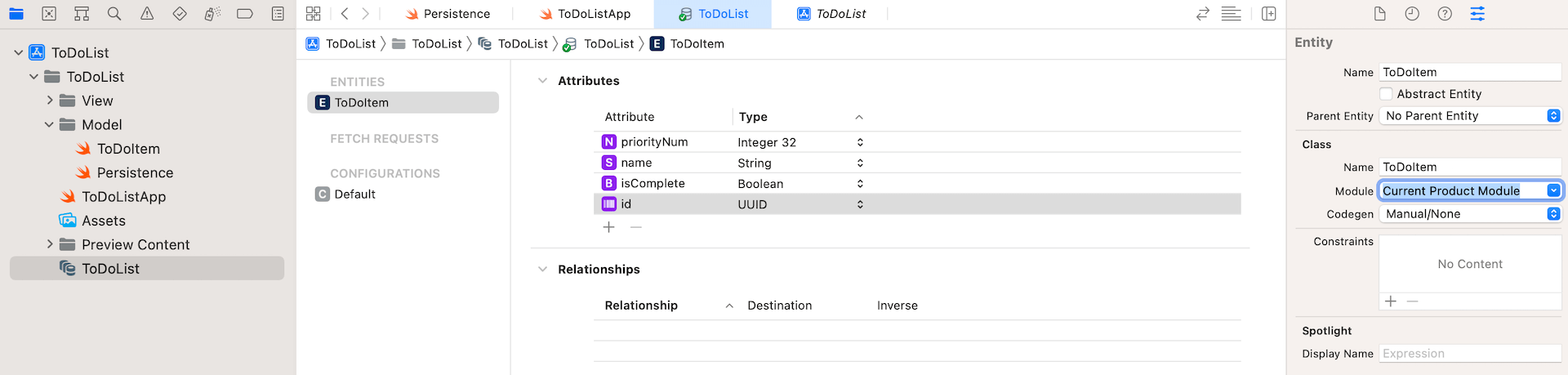
When you’ve got used Core Knowledge earlier than, it’s possible you’ll keep in mind that it’s important to create a knowledge mannequin (with a file extension .xcdatamodeld) utilizing a knowledge mannequin editor for information persistence. With the discharge of SwiftData, you not want to do this. SwiftData streamlines the entire course of with macros, one other new Swift characteristic in iOS 17. Say, for instance, you already outline a mannequin class for Tune as follows:
class Tune { Ā Ā var title: String Ā Ā var artist: String Ā Ā var album: String Ā Ā var style: String Ā Ā var ranking: Double } |
To make use of SwiftData, the brand new @Mannequin
macro is the important thing for storing persistent information utilizing SwiftUI. As an alternative of constructing the information mannequin with mannequin editor, SwiftData simply requires you to annotate the mannequin class with the @Mannequin
macro like this:
@Mannequin class Tune { Ā Ā var title: String Ā Ā var artist: String Ā Ā var album: String Ā Ā var style: String Ā Ā var ranking: Double } |
That is the way you outline the schema of the information mannequin in code. With this easy key phrase, SwiftData mechanically allows persistence for the information class and gives different information administration functionalities equivalent to iCloud sync. Attributes are inferred from properties and it helps fundamental worth sorts equivalent to Int and String.
SwiftData permits you to customise how your schema is constructed utilizing property metadata. You may add uniqueness constraints through the use of the @Attribute
annotation, and delete propagation guidelines with the @Relationship
annotation. If there are particular properties you do not need included, you should use the @Transient
macro to inform SwiftData to exclude them. Right here is an instance:
// The cascade relationship instructs SwiftData to delete all
// songs when the album is deleted.
@Attribute(.cascade) var songs: [Song]? = []
}
@Mannequin class Album { Ā Ā @Attribute(.distinctive) var identify: String Ā Ā var artist: String Ā Ā var style: String Ā Ā Ā // The cascade relationship instructs SwiftData to delete all Ā Ā Ā Ā // songs when the album is deleted. Ā Ā @Attribute(.cascade) var songs: [Song]? = [] } |
To drive the information persistent operations, there are two key objects of SwiftData that try to be conversant in: ModelContainer
and ModelContext
. The ModelContainer
serves because the persistent backend in your mannequin sorts. To create a ModelContaine
r, you merely have to instantiate an occasion of it.
// With configuration
let container = attempt ModelContainer(for: [Song.self, Album.self],
configurations: ModelConfiguration(url: URL(“path”))))
// Primary let container = attempt ModelContainer(for: [Song.self, Album.self]) Ā // With configuration let container = attempt ModelContainer(for: [Song.self, Album.self], Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā configurations: ModelConfiguration(url: URL(“path”)))) |
In SwiftUI, you’ll be able to arrange the mannequin container on the root of the applying:
@essential
struct MusicApp: App {
var physique: some Scene {
WindowGroup {
ContentView()
}
.modelContainer (for: [Song.self, Album.self]))
}
}
import SwiftData import SwiftUI Ā @essential struct MusicApp: App { Ā Ā Ā Ā var physique: some Scene { Ā Ā Ā Ā Ā Ā Ā Ā WindowGroup { Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā ContentView() Ā Ā Ā Ā Ā Ā Ā Ā } Ā Ā Ā Ā Ā Ā Ā Ā .modelContainer (for: [Song.self, Album.self])) Ā Ā Ā Ā } } |
After you have arrange the mannequin container, you’ll be able to start utilizing the mannequin context to fetch and save information. The context serves as your interface for monitoring updates, fetching information, saving modifications, and even undoing these modifications. When working with SwiftUI, you’ll be able to sometimes acquire the mannequin context out of your viewās atmosphere:
struct ContextView: View { Ā Ā Ā Ā @Surroundings(.modelContext) non-public var modelContext } |
With the context, you might be able to fetch information. The only method is to make use of the @Question
property wrapper. You may simply load and filter something saved in your database with a single line of code.
@Question(type: .artist, order: .reverse) var songs: [Song] |
To insert merchandise within the persistent retailer, you’ll be able to name the insert methodology of the mannequin context and move it the mannequin objects to insert.
modelContext.insert(track) |
Equally, you’ll be able to delete the merchandise by way of the mannequin context like this:
modelContext.delete(track) |
It is a transient introduction of SwiftData. In case youāre nonetheless feeling confused about the best way to use SwiftData? No worries. You’ll perceive its utilization after constructing a ToDO app.
Constructing a Easy To Do App
Now that you’ve got a fundamental understanding of SwiftData, I wish to show the best way to construct a easy to-do app utilizing this framework. Please notice that the app isn’t absolutely useful and solely permits customers so as to add a random process to the to-do listing. Nevertheless, it serves as a very good place to begin to familiarize your self with the SwiftData framework.
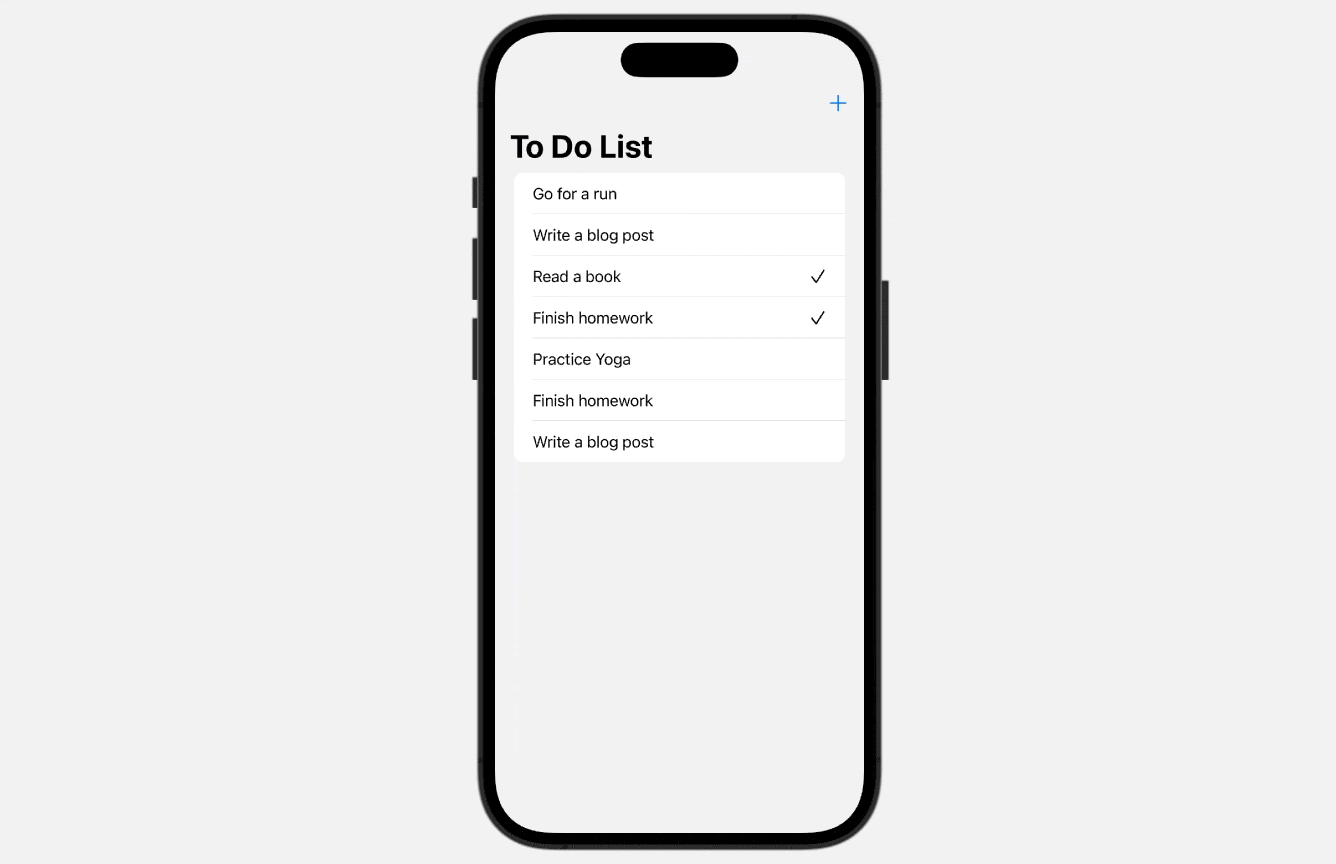
Assuming youāve created a SwiftUI venture in Xcode, letās first create the information mannequin of the app. Create a brand new file named ToDoItem
and replace the content material like this:
@Mannequin class ToDoItem: Identifiable {
var id: UUID
var identify: String
var isComplete: Bool
init(id: UUID = UUID(), identify: String = “”, isComplete: Bool = false) {
self.id = id
self.identify = identify
self.isComplete = isComplete
}
}
import Basis import SwiftData Ā @Mannequin class ToDoItem: Identifiable { Ā Ā Ā Ā var id: UUID Ā Ā Ā Ā var identify: String Ā Ā Ā Ā var isComplete: Bool Ā Ā Ā Ā Ā init(id: UUID = UUID(), identify: String = “”, isComplete: Bool = false) { Ā Ā Ā Ā Ā Ā Ā Ā self.id = id Ā Ā Ā Ā Ā Ā Ā Ā self.identify = identify Ā Ā Ā Ā Ā Ā Ā Ā self.isComplete = isComplete Ā Ā Ā Ā } } |
As mentioned earlier, SwiftData simplifies the method of defining a schema utilizing code. All it’s essential to do is annotate the mannequin class with theĀ @Mannequin
Ā macro. SwiftData will then mechanically allow persistence for the information class.
Earlier than we transfer onto constructing the UI of the app and dealing with the information persistent, letās create a helper perform for producing a random to-do merchandise:
let randomIndex = Int.random(in: 0..<duties.depend)
let randomTask = duties[randomIndex]
return ToDoItem(identify: randomTask, isComplete: Bool.random())
}
func generateRandomTodoItem() –> ToDoItem { Ā Ā Ā Ā let duties = [ “Buy groceries”, “Finish homework”, “Go for a run”, “Practice Yoga”, “Read a book”, “Write a blog post”, “Clean the house”, “Walk the dog”, “Attend a meeting” ] Ā Ā Ā Ā Ā let randomIndex = Int.random(in: 0..<duties.depend) Ā Ā Ā Ā let randomTask = duties[randomIndex] Ā Ā Ā Ā Ā return ToDoItem(identify: randomTask, isComplete: Bool.random()) } |
Subsequent, letās construct the primary UI of the to-do app. Within the ContentView.swift
file, replace the code like this:
struct ContentView: View {
@Question var todoItems: [ToDoItem]
var physique: some View {
NavigationStack {
Record {
ForEach(todoItems) { todoItem in
HStack {
Textual content(todoItem.identify)
Spacer()
if todoItem.isComplete {
Picture(systemName: “checkmark”)
}
}
}
}
.navigationTitle(“To Do Record”)
}
}
}
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
import SwiftData Ā struct ContentView: View { Ā Ā Ā Ā @Question var todoItems: [ToDoItem] Ā Ā Ā Ā Ā var physique: some View { Ā Ā Ā Ā Ā Ā Ā Ā NavigationStack { Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Record { Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā ForEach(todoItems) { todoItem in Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā HStack { Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Textual content(todoItem.identify) Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Spacer() Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā if todoItem.isComplete { Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Picture(systemName: “checkmark”) Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā } Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā } Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā } Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā } Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā .navigationTitle(“To Do Record”) Ā Ā Ā Ā Ā Ā Ā Ā } Ā Ā Ā Ā } } |
We mark theĀ todoItems
Ā array with theĀ @Question
Ā property wrapper. ThisĀ @Question
Ā property mechanically fetches the required information for you. Within the supplied code, we specify to fetch theĀ ToDoItem
Ā cases. As soon as we retrieve the to-do objects, we make the most of theĀ Record
Ā view to show the objects.
Arrange the mannequin container
To drive the information persistent operations, we additionally have to arrange the mannequin container. Change over to ToDoDemoAppApp.swift
and fasten the modelContainer
modifier like this:
struct ToDoDemoAppApp: App { Ā Ā Ā Ā var physique: some Scene { Ā Ā Ā Ā Ā Ā Ā Ā WindowGroup { Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā Ā ContentView() Ā Ā Ā Ā Ā Ā Ā Ā } Ā Ā Ā Ā Ā Ā Ā Ā .modelContainer(for: ToDoItem.self) Ā Ā Ā Ā } } |
Right here, we set a shared mannequin container for storing cases of ToDoItem
.
In case you preview the ContentView
, the listing view is empty. Clearly, we havenāt saved any to-do objects within the database. Now, letās add a āAdd merchandiseā button to insert a random to-do merchandise into the database.
Storing to-do objects into the database
In ContentView.swift
, declare the next variable to retrieve the mannequin context:
@Surroundings(.modelContext) non-public var modelContext |
After acquiring the mannequin context, we will simply insert information into the database. Weāll add a toolbar button for including a random to-do merchandise. Insert the next code contained in the NavigationStack
view (place it after navigationTitle
):
.toolbar { Ā Ā Ā Ā Button(“”, systemImage: “plus”) { Ā Ā Ā Ā Ā Ā Ā Ā modelContext.insert(generateRandomTodoItem()) Ā Ā Ā Ā } } |
To retailer an merchandise into database, you merely name the insert
methodology of the mannequin context.
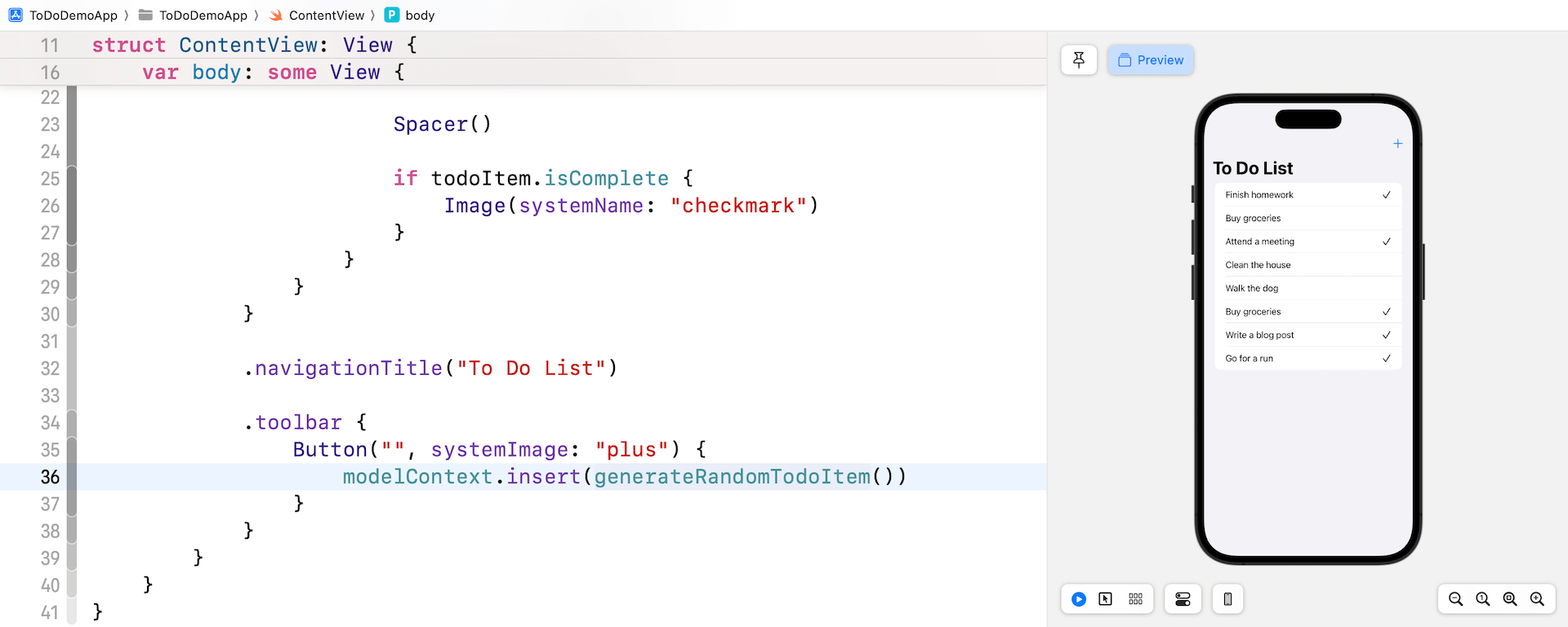
Now youāre prepared to check the app within the simulator. Nevertheless, when you intend to check it within the preview canvas, it’s essential to make one extra modification by including the mannequin container inside theĀ #Preview
Ā block:
#Preview { Ā Ā Ā Ā ContentView() Ā Ā Ā Ā Ā Ā Ā Ā .modelContainer(for: ToDoItem.self) } |
Whenever you faucet the ā+ā button, the app immediately shops the to-do merchandise. Concurrently, it retrieves the brand new merchandise from the database and shows it within the listing view.
Updating an present merchandise
SwiftData considerably reduces the quantity of labor required to deal with merchandise updates or modifications within the persistent retailer. By merely marking your mannequin objects with the @Mannequin
macro, SwiftData mechanically modifies the setters for change monitoring and remark. Which means that no code modifications are wanted to replace the to-do objects.
To check the replace habits, you’ll be able to merely run the app on a simulator. Whenever you faucet a to-do merchandise, it ought to be marked as full. This variation is now saved completely within the gadgetās database. Even after restarting the app, all of the objects will nonetheless be retained.
Deleting the merchandise from the database
Now that you know the way to carry out fetch, replace, and insert, how about information deletion? We are going to add a characteristic to the app for eradicating a to-do merchandise.
Within the ContentView
struct, connect the onDelete
modifier to the ForEach
loop:
.onDelete(carry out: { indexSet in Ā Ā Ā Ā for index in indexSet { Ā Ā Ā Ā Ā Ā Ā Ā let itemToDelete = todoItems[index] Ā Ā Ā Ā Ā Ā Ā Ā modelContext.delete(itemToDelete) Ā Ā Ā Ā } }) |
This closure takes an index set that shops the indices of the objects to be deleted. To take away an merchandise from the persistent retailer, merely name the delete
perform of the mannequin context and specify the merchandise to be deleted.
The onDelete
modifier mechanically allows the swipe-to-delete characteristic within the listing view. To do this out, merely run the app and swipe to delete an merchandise. It will fully take away the merchandise from the database.
Abstract
I hope that you simply now have a greater understanding of the best way to combine SwiftData right into a SwiftUI venture and the best way to carry out all fundamental CRUD (create, learn, replace & delete) operations. Apple has put quite a lot of efforts to make persistent information administration and information modeling simpler for Swift builders and new comers.
Whereas Core Knowledge stays an possibility for backward compatibility, itās time to be taught the SwiftData framework, particularly in case you are creating an app solely for iOS 17 or later. Embrace this new framework to leverage the improved capabilities and advantages SwiftData gives.