Introduction
As AI is taking up the world, Giant language fashions are in enormous demand in know-how. Giant Language Fashions generate textual content in a method a human does. They can be utilized to develop pure language processing (NLP) purposes various from chatbots and textual content summarizers to translation apps, digital assistants, and many others.
Google launched its next-generation mannequin named Palm 2. This mannequin excels in superior scientific and mathematical operations and is utilized in reasoning and language translations. This mannequin is educated over 100+ spoken phrase languages and 20+ programming languages.
As it’s educated in numerous programming languages, it may be used to translate one programming language to a different. For instance, if you wish to translate Python code to R or JavaScript code to TypeScript, and many others., you may simply use Palm 2 to do it for you. Other than these, it could generate idioms and phrases and simply break up a posh activity into less complicated duties, making it a lot better than the earlier giant language fashions.
Studying Goals
- Introduction to Google’s Palm API
- Discover ways to entry Palm API by producing an API key
- Utilizing Python, discover ways to generate easy textual content utilizing textual content mannequin
- Discover ways to create a easy chatbot utilizing Python
- Lastly, we focus on learn how to use Langchain with Palm API.
This text was revealed as part of the Knowledge Science Blogathon.
Palm API
Utilizing the Palm API, you may entry the capabilities of Google’s Generative AI fashions and develop fascinating AI-powered purposes. Nonetheless, if you wish to work together straight with the Palm 2 mannequin from the browser, you need to use the browser-based IDE “MakerSuite”. However you may entry the Palm 2 mannequin utilizing the Palm API to combine giant language fashions into your purposes and construct AI-driven purposes utilizing your organization’s information.
Three totally different immediate interfaces are designed, and you will get began with anybody amongst them utilizing the Palm API. They’re:
- Textual content Prompts: You should utilize the mannequin named “text-bison-001 to generate easy textual content. Utilizing textual content prompts, you may generate textual content, generate code, edit textual content, retrieve data, extract information, and many others..
- Knowledge Prompts: These assist you to assemble prompts in a tabular format.
- Chat Prompts: Chat prompts are used to construct conversations. You should utilize the mannequin named “chat-bison-001” to make use of chat providers.
Entry Palm API
Navigate to the web site https://builders.generativeai.google/ and be a part of the maker suite. You can be added to the waitlist and will probably be given entry in all probability inside 24 hours.
Generate an API key:
- You’ll want to get your personal API key to make use of the API.
- You may join your software to the Palm API and entry its providers utilizing the API key.
- As soon as your account is registered, it is possible for you to to generate it.
- Subsequent, go forward and generate your API key, as proven within the screenshot under:
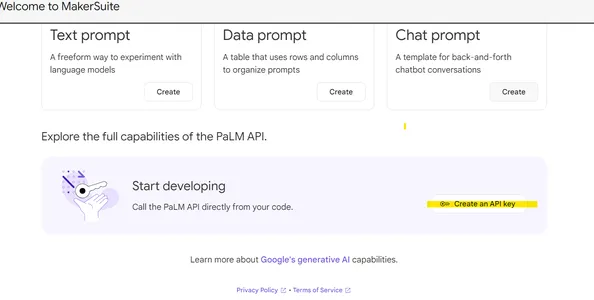
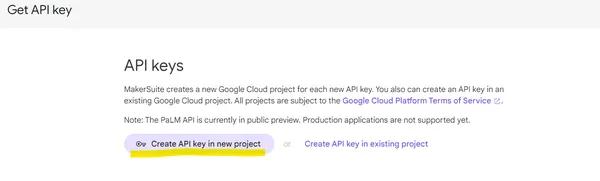
Save the API key as we are going to use it additional.
Setting the Setting
To make use of the API with Python, set up it utilizing the command:
pip set up google-generativeai
Subsequent, we configure it utilizing the API key that we generated earlier.
import google.generativeai as palm
palm.configure(api_key=API_KEY)
To record the obtainable fashions, we write the under code:
fashions = [model for model in palm.list_models()]
for mannequin in fashions:
print(mannequin.title)
Output:
fashions/chat-bison-001
fashions/text-bison-001
fashions/embedding-gecko-001
Generate Textual content
We use the mannequin “text-bison-001” to generate textual content and move GenerateTextRequest. The generate_text() operate takes in two parameters i.e., a mannequin and a immediate. We move the mannequin as “text-bison-001,” and the immediate comprises the enter string.
Clarification:
- Within the instance under, we move the model_id variable with the mannequin title and a immediate variable containing the enter textual content.
- We then move the model_id as mannequin and the immediate as immediate to the generate_text() technique.
- The temperature parameter signifies how random the response will probably be. In different phrases, if you would like the mannequin to be extra artistic, you may give it a worth of 0.3.
- Lastly, the parameter “max_tokens” signifies the utmost variety of tokens the mannequin’s output can include. A token can include roughly 4 tokens. Nonetheless, for those who don’t specify, a default worth of 64 will probably be assigned to it.
Instance 1
model_id="fashions/text-bison-001"
immediate=""'write a canopy letter for a knowledge science job applicaton.
Summarize it to 2 paragraphs of fifty phrases every. '''
completion=palm.generate_text(
mannequin=model_id,
immediate=immediate,
temperature=0.99,
max_output_tokens=800,
)
print(completion.outcome)
Output:
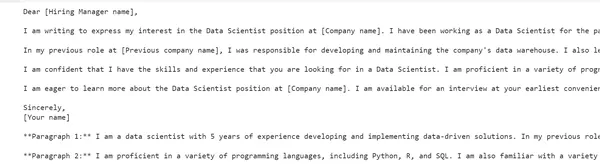
We outline some time loop that asks for enter and generates a reply. The response.final assertion prints the response.
model_id="fashions/chat-bison-001"
immediate="I need assistance with a job interview for a knowledge analyst job. Are you able to assist me?"
examples=[
('Hello', 'Hi there mr. How can I be assistant?'),
('I want to get a High paying Job','I can work harder')
]
response=palm.chat(messages=immediate, temperature=0.2, context="Converse like a CEO", examples=examples)
for messages in response.messages:
print(messages['author'],messages['content'])
whereas True:
s=enter()
response=response.reply(s)
print(response.final)
Output:
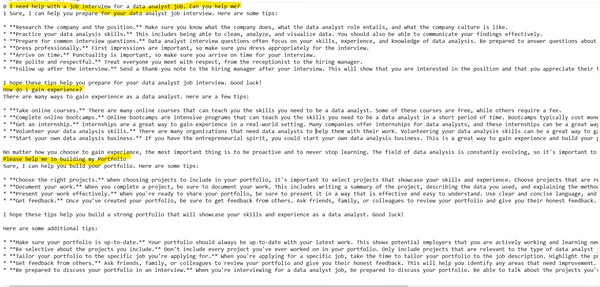
Utilizing Palm API with LangChain
LangChain is an open-source framework that means that you can join giant language fashions to your purposes. To make use of Palm API with langchain, we import GooglePalmEmbeddings from langchain.embeddings. The langchain has an embedding class that gives an ordinary interface for numerous textual content embedding fashions equivalent to OpenAI, HuggingFace, and many others.
We move the prompts as an array, as proven within the under instance. Then, we name llm._generate() operate and move the prompts array as a parameter.
from langchain.embeddings import GooglePalmEmbeddings
from langchain.llms import GooglePalm
llm=GooglePalm(google_api_key=API_KEY)
llm.temperature=0.2
prompts=["How to Calculate the area of a triangle?","How many sides are there for a polygon?"]
llm_result= llm._generate(prompts)
res=llm_result.generations
print(res[0][0].textual content)
print(res[1][0].textual content)
Output:
Immediate 1
1.
**Discover the bottom and peak of the triangle.
** The bottom is the size of the aspect of the triangle that's parallel to the bottom, and the peak is the size of the road phase that's perpendicular to the bottom and intersects the alternative vertex.
2.
**Multiply the bottom and peak and divide by 2.
** The system for the world of a triangle is A = 1/2 * b * h.
For instance, if a triangle has a base of 5 cm and a peak of 4 cm, its space can be 1/2 * 5 * 4 = 10 cm2.
Immediate 2
3
Conclusion
On this article, we’ve launched Google’s newest Palm 2 mannequin and the way it’s higher than the earlier fashions. We then realized learn how to use Palm API with Python Programming Language. We then mentioned learn how to develop easy purposes and generate textual content and chats. Lastly, we lined learn how to embed it utilizing Langchain framework.
Key Takeaways
- Palm API permits customers to develop purposes utilizing Giant Language Fashions
- Palm API gives a number of text-generation providers, equivalent to textual content service to generate textual content and a chat service to generate chat conversations.
- Google generative-ai is the Palm API Python Library and might be simply put in utilizing the pip command.
Continuously Requested Questions
A. To rapidly get began with palm API in python, you may set up a library utilizing the pip command – pip set up google generative-ai.
A. Sure, you may entry Google’s Giant Language Fashions and develop purposes utilizing Palm API.
A. Sure, Google’s Palm API and MakerSuite can be found for public preview.
A. Google’s Palm 2 mannequin was educated in over 20 programming languages and may generate code in numerous programming languages.
A. Palm API comes with each textual content and chat providers. It gives a number of textual content technology capabilities.
The media proven on this article shouldn’t be owned by Analytics Vidhya and is used on the Creator’s discretion.