Introduction
Within the intricate world of synthetic intelligence (AI), the Hill Climbing Algorithm emerges as a basic methodology for problem-solving. Impressed by the metaphorical ascent up a hill, this method is essential for navigating the advanced terrain of optimization issues in AI. It’s a strategic method to discovering the best resolution amongst many prospects, making it a cornerstone in numerous AI purposes.
How Does the Hill Climbing Algorithm Work?
The Hill Climbing Algorithm initiates its course of at a base level, analogous to standing on the foot of a hill, and embarks on an iterative exploration of adjoining options. Like a climber assessing the subsequent finest step, every algorithm transfer is an incremental change scrutinized towards an goal perform. This perform guides the algorithm in direction of the height, guaranteeing development.
As an illustration, a maze-solving utility could be nice. On this situation, every step the algorithm executes symbolizes a strategic transfer inside the maze, concentrating on the shortest path to the exit. The algorithm evaluates every potential step for its effectiveness in advancing it nearer to the exit, much like a climber gauging which step will elevate it nearer to the height of a hill.
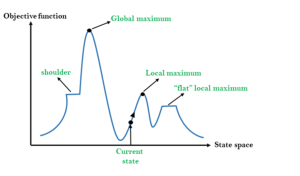
Options of Hill Climbing Algorithm
Key options of the Hill Climbing Algorithm embody:
- Generate and Take a look at Strategy: This function entails producing neighboring options and evaluating their effectiveness, at all times aiming for an upward transfer within the resolution area.
- Grasping Native Search: The algorithm makes use of an inexpensive technique, choosing speedy useful strikes that promise native enhancements.
- No Backtracking: Not like different algorithms, Hill Climbing doesn’t revisit or rethink earlier selections, persistently shifting ahead within the quest for the optimum resolution.
Sorts of Hill Climbing Algorithm
The Hill Climbing Algorithm presents itself in numerous varieties, every appropriate for particular eventualities:
Easy Hill Climbing
This model evaluates neighboring options and selects the primary one which improves the present state. For instance, optimizing supply routes would possibly decide the primary alternate route that shortens supply time, even when it’s not optimum.
Algorithm:
Step 1: Begin with an preliminary state.
Step 2: Examine if the preliminary state is the purpose. In that case, return success and exit.
Step 3: Enter a loop to seek for a greater state repeatedly.
- Choose a neighboring state inside the loop by making use of an operator to the present state.
- Consider this new state:
- If it’s the purpose state, return success and exit.
- If it’s higher than the present state, replace the present state to this new state.
- If it’s not higher, discard it and proceed the loop.
Step 4: Finish the method if no higher state is discovered and the purpose isn’t achieved.
Steepest-Ascent Hill Climbing
This variant assesses all neighboring options, selecting the one with essentially the most vital enchancment. In allocating sources, for example, it evaluates all potential distributions to establish essentially the most environment friendly one.
Algorithm:
Step 1: Consider the preliminary state. If it’s the purpose, you may return success; in any other case, set it as the present state.
Step 2: Repeat till an answer is discovered or no additional enchancment is feasible.
- Initialize “BEST_SUCCESSOR” as the perfect potential enchancment over the present state.
- For every operator, apply to the present state, then consider the brand new state.
- If it’s the purpose, return success.
- If higher than “BEST_SUCCESSOR,” replace “BEST_SUCCESSOR” to this new state.
- If “BEST_SUCCESSOR” is an enchancment, replace the present state.
Step 3: Cease the algorithm if no resolution is discovered or additional enchancment is feasible.
Stochastic Hill Climbing
It introduces randomness by selecting a random neighbor for exploration. This methodology broadens the search, stopping the lure of native optima. In an AI chess recreation, this would possibly imply randomly selecting a transfer from a set of excellent choices to shock the opponent.
Sensible Examples
Let’s dive proper into some sensible examples for every and attempt to remedy the issue of discovering the utmost quantity in a listing utilizing all three kinds of Hill Climbing Algorithms.
Discovering the Most Quantity within the checklist utilizing Easy Hill Climbing
Code:
def simple_hill_climbing(numbers):
current_index = 0
whereas True:
# Examine if subsequent index is inside the checklist vary
if current_index + 1 < len(numbers):
# Evaluate with the subsequent quantity
if numbers[current_index] < numbers[current_index + 1]:
current_index += 1
else:
# Present quantity is larger than the subsequent
return numbers[current_index]
else:
# Finish of the checklist
return numbers[current_index]
# Instance checklist of numbers
numbers = [1, 3, 7, 12, 9, 5]
max_number = simple_hill_climbing(numbers)
print(f”The utmost quantity within the checklist is: {max_number}”)
Output: The utmost quantity within the checklist is: 12
On this code:
- We begin from the primary quantity within the checklist.
- We evaluate it with the subsequent quantity. If the subsequent quantity is bigger, we transfer to it.
- The method repeats till we discover a quantity that’s not smaller than the subsequent one, indicating we’ve discovered the utmost within the reached section of the checklist.
Discovering the Most Quantity within the checklist utilizing Steepest-Ascent Hill Climbing
Code:
def steepest_ascent_hill_climbing(numbers):
current_max = numbers[0]
for num in numbers:
if num > current_max:
current_max = num
return current_max
# Instance checklist of numbers
numbers = [1, 3, 7, 12, 9, 5]
max_number = steepest_ascent_hill_climbing(numbers)
print(f"The utmost quantity within the checklist is: {max_number}")
Output: The utmost quantity within the checklist is 12.
On this code:
- The algorithm begins with the primary quantity as the present most.
- It iterates via the checklist, updating the present most every time it finds a bigger quantity.
- The biggest quantity discovered after checking all components is returned as the utmost.
This instance illustrates the essence of Steepest-Ascent Hill Climbing, the place all potential “strikes” (or, on this case, all components within the checklist) are evaluated to search out the perfect one.
Discovering the Most Quantity within the checklist utilizing Stochastic Hill Climbing
Code:
import random
def stochastic_hill_climbing(numbers):
current_index = random.randint(0, len(numbers) - 1)
current_max = numbers[current_index]
iterations = 100 # Restrict the variety of iterations to keep away from infinite loops
for _ in vary(iterations):
next_index = random.randint(0, len(numbers) - 1)
if numbers[next_index] > current_max:
current_max = numbers[next_index]
return current_max
# Instance checklist of numbers
numbers = [1, 3, 7, 12, 9, 5]
max_number = stochastic_hill_climbing(numbers)
print(f"The utmost quantity within the checklist is: {max_number}")
Output: The utmost quantity within the checklist is: 12
On this code:
- We begin from a random place within the checklist.
- The algorithm then randomly selects one other index and compares the numbers.
- If the brand new quantity is bigger, it turns into the present most.
- This course of is repeated for a set variety of iterations (to keep away from probably infinite loops).
Since this method entails randomness, it won’t at all times yield absolutely the most, particularly with restricted iterations, however it affords a unique method of exploring the checklist.
A Enjoyable Instance
Think about discovering the best level on a panorama representing happiness ranges all through the day. We’ll use a easy perform to simulate the ‘happiness’ degree at completely different occasions.
Right here’s the Python code with explanations:
Code
import random
# A easy perform to simulate happiness ranges
def happiness(time):
return -((time - 12)**2) + 50
# Hill Climbing algorithm to search out the time with the best happiness
def hill_climbing():
current_time = random.uniform(0, 24) # Beginning at a random time
current_happiness = happiness(current_time)
whereas True:
# Making an attempt a brand new time near the present time
new_time = current_time + random.uniform(-1, 1)
new_happiness = happiness(new_time)
# If the brand new time is happier, it turns into the brand new present time
if new_happiness > current_happiness:
current_time, current_happiness = new_time, new_happiness
else:
# If not happier, we have discovered the happiest time
return current_time, current_happiness
# Operating the algorithm
best_time, best_happiness = hill_climbing()
print(f"The happiest time is round {best_time:.2f} hours with a happiness degree of {best_happiness:.2f}")
Output
The happiest time is round 16.57 hours, with a happiness degree of 29.13
On this code:
- The happiness perform represents our day by day happiness degree, peaking round midday.
- The hill_climbing perform begins randomly and explores close by occasions to see in the event that they make us ‘happier.’
- If a close-by time is happier, it turns into our new ‘present time.’
- The method repeats till no close by time is happier.
This simplistic instance reveals how the Hill Climbing algorithm can discover an optimum resolution (the happiest time of the day) by making small adjustments and checking in the event that they enhance the end result.
Functions of Hill Climbing Algorithm
The flexibility of the Hill Climbing Algorithm is highlighted by its wide selection of purposes:
- Advertising and marketing: The Hill Climbing algorithm is a game-changer for advertising managers crafting top-notch methods. It’s instrumental in fixing the basic Touring-Salesman issues, optimizing gross sales routes, and lowering journey time. This results in extra environment friendly gross sales operations and higher useful resource utilization.
- Robotics: The algorithm performs a essential position in robotics, enhancing the efficiency and coordination of assorted robotic parts. This results in extra subtle and environment friendly robotic programs performing advanced duties.
- Job Scheduling: Inside computing programs, Hill Climbing is vital in job scheduling, optimizing the allocation of system sources for numerous duties. Effectively managing the distribution of jobs throughout completely different nodes ensures optimum use of computational sources, enhancing general system effectivity.
- Sport Idea: In AI-based gaming, the algorithm is pivotal in growing subtle methods figuring out strikes that maximize profitable probabilities or scores.
Benefits and Disadvantages of Hill Climbing Algorithms
Benefits | Disadvantages |
Simplicity: The algorithm is simple to grasp and implement. | Susceptibility to Native Optima: The algorithm can turn into caught at regionally optimum options that aren’t the perfect general. |
Reminiscence Effectivity: It’s memory-efficient, sustaining solely the present state’s information. | Restricted Exploration: Its tendency to give attention to the speedy neighborhood limits its exploration, probably overlooking globally optimum options. |
Fast Convergence: It usually converges swiftly to an answer, which is useful in eventualities the place time is essential. | Dependence on Preliminary State: The standard and effectiveness of the answer discovered closely rely upon the start line. |
Conclusion
The Hill Climbing Algorithm, with its easy but efficient method, stands as a vital device in AI. Its adaptability throughout numerous domains highlights its significance in AI and optimization. Regardless of its inherent limitations, as AI continues to evolve, the position of this algorithm in navigating advanced issues stays indispensable.