The SwiftUI framework comes with a built-in checkbox, but it surely’s solely obtainable on the macOS platform. If you wish to use checkboxes on iOS, it’s a must to construct your individual element. Beforehand, we’ve lined ToggleStyle
to create customized toggles. By making use of the identical method, it’s not onerous to construct a checkbox to your iOS tasks.
Utilizing Checkbox on macOS
Let’s first have a look how you utilize checkbox on macOS. To create a checkbox, you create a Toggle
view and alter it to the .checkbox
fashion. Right here is an instance:
@State non-public var isChecked = false
var physique: some View {
VStack {
Toggle(isOn: $isChecked) {
Textual content(“Purchase a pack of Airtags”)
}
.toggleStyle(.checkbox)
}
.padding()
}
}
struct ContentView: View {
@State non-public var isChecked = false
var physique: some View { VStack { Toggle(isOn: $isChecked) { Textual content(“Purchase a pack of Airtags”) } .toggleStyle(.checkbox) } .padding() } } |
In the event you run the code on macOS, it renders a regular checkbox.
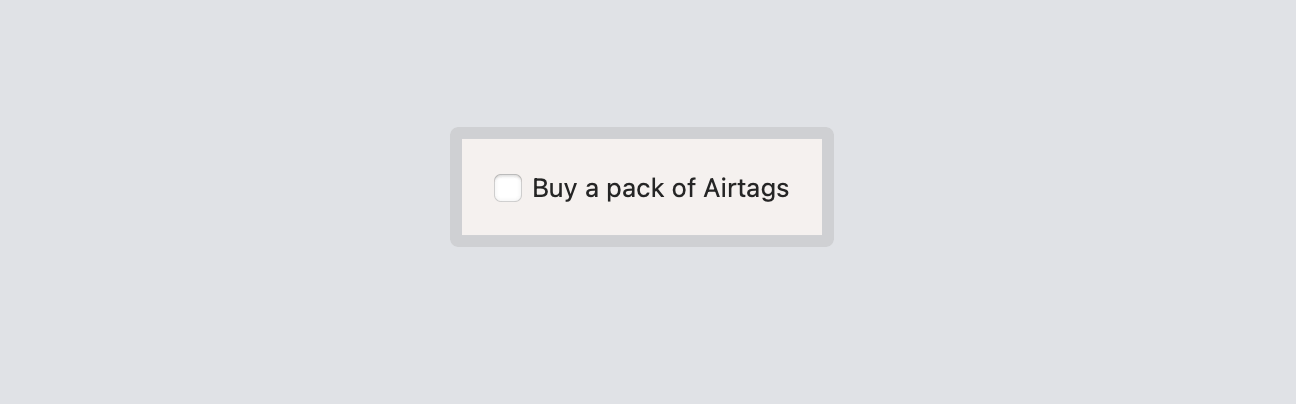
Creating Checkbox Toggle Type on iOS
The .checkbox
fashion doesn’t include the iOS SDK. That mentioned, it’s fairly straightforward to create such a toggle fashion utilizing the ToggleStyle
protocol. If you wish to know easy methods to work with ToggleStyle
, please try our earlier tutorial.
On iOS, we are able to create a brand new fashion named CheckboxToggleStyle
which adopts the ToggleStyle
protocol and implement the required methodology:
RoundedRectangle(cornerRadius: 5.0)
.stroke(lineWidth: 2)
.body(width: 25, top: 25)
.cornerRadius(5.0)
.overlay {
Picture(systemName: configuration.isOn ? “checkmark” : “”)
}
.onTapGesture {
withAnimation(.spring()) {
configuration.isOn.toggle()
}
}
configuration.label
}
}
}
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
struct CheckboxToggleStyle: ToggleStyle { func makeBody(configuration: Configuration) –> some View { HStack {
RoundedRectangle(cornerRadius: 5.0) .stroke(lineWidth: 2) .body(width: 25, top: 25) .cornerRadius(5.0) .overlay { Picture(systemName: configuration.isOn ? “checkmark” : “”) } .onTapGesture { withAnimation(.spring()) { configuration.isOn.toggle() } }
configuration.label
} } } |
Within the makebody
methodology, we used a RoundedRectangle
view and overlay it with a “checkmark” picture when the toggle is enabled.

To make use of this toggle fashion, you merely connect the toggleStyle
modifier and go an occasion of CheckboxToggleStyle
like this:
Toggle(isOn: $isChecked) { Textual content(“Purchase a pack of Airtags”) } .toggleStyle(CheckboxToggleStyle()) |
If you wish to use the dot-syntax, you’ll be able to prolong ToggleStyle
and declare a corresponding static variable like this:
static var checkmark: CheckboxToggleStyle { CheckboxToggleStyle() }
}
extension ToggleStyle the place Self == CheckboxToggleStyle {
static var checkmark: CheckboxToggleStyle { CheckboxToggleStyle() } } |
Now you’ll be able to apply the fashion utilizing the dot syntax.
Toggle(isOn: $isChecked) { Textual content(“Purchase a pack of Airtags”) } .toggleStyle(.checkmark) |