In the event you spend an inexpensive period of time programming, you’re certain to come across code feedback. You could have even written some your self. These tiny items of textual content are embedded throughout the program, however they’re ignored by the compiler or interpreter. They’re meant for the people who work with the code, they usually have many purposes. They might even reshape your code or create documentation for others robotically. Need to know how you can use them correctly? Then you definately’re in the precise place!
Time to discover feedback, how you can use them and in addition how not to make use of them.
What You’ll Be taught:
By studying this text, you’ll study:
- What a remark is, varieties and how you can use them.
- The place they started and the place they’re now.
- The distinction between good and unhealthy feedback.
- Sensible purposes from the previous and current.
By the top of the article, you’ll know why code feedback are vital, greatest practices for writing efficient feedback, some frequent pitfalls and errors to keep away from and the instruments and sources you should utilize to optimize your code commenting course of, together with automated documentation mills, remark linters and code overview platforms.
You’ll begin with an in-depth have a look at what code feedback are.
What Are Code Feedback?
Feedback inside a software program program are human-readable annotations that programmers add to supply details about what the code is doing. Feedback take many types, relying on the programming language and the intent of the remark.
They’re categorized into two varieties: block feedback and line feedback.
-
Block feedback delimit a area throughout the code through the use of a sequence of characters at the beginning of the remark and one other character sequence on the finish. For instance, some use
/*
at the beginning and*/
on the finish. -
Line feedback use a sequence of characters to ask the compiler to disregard the whole lot from the beginning of the remark till the top of the road. For instance:
#
,–
or//
. How you can point out line feedback relies on the programming language you’re utilizing.
Right here’s an instance of how every sort of remark seems in Swift:
struct EventList: View {
// THIS IS A LINE COMMENT @EnvironmentObject var userData: UserData
@EnvironmentObject var eventData: EventData
/* THIS IS A BLOCK COMMENT
@State personal var isAddingNewEvent = false
@State personal var newEvent = Occasion()
*/
var physique: some View {
...
}
}
Some programming languages solely assist one sort of remark sequence, and a few require workarounds to make a sequence work. Right here’s how some languages deal with feedback:
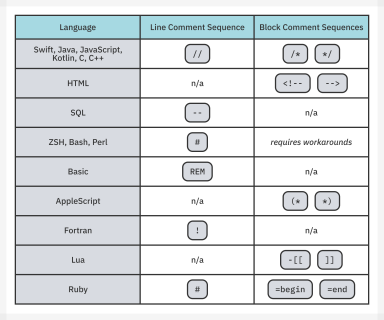
Take into consideration the way you’d use a sticky observe. For programmers, a remark is a sticky observe with limitless makes use of.
Feedback have taken on extra significance over time as their makes use of have expanded and standardized. You’ll find out about many of those makes use of on this article.
A Transient Historical past of the Remark
Not so way back, computer systems weren’t programmed utilizing a display and a keyboard. They had been programmed utilizing punch playing cards: small items of stiff paper with holes punched in a sequence to point directions for a pc. These would change into large stacks that had been fed right into a reader, and the pc would run this system.
It was arduous to know at a look what every card or a set of playing cards did. To unravel this, programmers would write notes on them. These notes had been ignored by the machine reader as a result of it solely learn the holes on the cardboard. Right here’s an instance:
Immediately, most feedback serve the identical objective: to help in understanding what code does.
Code With out Feedback
A standard saying amongst programmers is, “Good code doesn’t want a proof”. That is true to a sure extent, and a few programmers don’t write feedback of their code.
However what occurs when that code will get actually huge? Or it incorporates a number of enterprise guidelines and logic? Or different folks want to make use of it? It might be attainable to decipher the uncommented code, however at what value in effort and time?
When our focus is continually on writing code, we neglect that a couple of months — even a couple of weeks — after writing mentioned code, quite a lot of the logic has left our reminiscence. Why did we make sure selections? Why did we use sure conventions. With out feedback, we should undergo the costly train of determining what we had been pondering.
Feedback are extremely helpful, and good builders ought to use them. Nonetheless, all feedback aren’t created equal. Right here’s a have a look at what makes feedback good, ineffective and even counterproductive.
Good Feedback
Good feedback are easy and simple to learn. They make clear or point out a practice of thought versus how the code works.
They take many types, akin to annotations, hyperlinks and citations to sources, licenses, flags, duties, instructions, documentation, and many others.
Ineffective Feedback
Feedback are good, however you don’t want to make use of them all over the place. A standard pitfall is utilizing feedback to explain what code is doing, when that’s already clear.
For instance, in case you have code that’s iterating via an array and incrementing every worth, there’s no want to clarify that — it must be apparent to the programmer who’s reviewing the code. What isn’t apparent, although, is why you’re doing that. And that’s what a very good remark will clarify.
Describing the apparent is a standard pitfall… and feedback that do that are simply taking over area and probably inflicting confusion.
Dangerous Feedback
Dangerous feedback are extra harmful than ineffective. They trigger confusion, or worse, attempt to excuse convoluted or badly written code. Don’t attempt to use feedback as an alternative choice to good code. For instance:
var n = 0 // Line quantity
As a substitute, do:
var lineNumber = 0
Abusing feedback, akin to cluttering code with TODO, BUG and FIXME feedback, is unhealthy observe. The most effective answer is to resolve these previous to committing code when engaged on a staff — or just create a problem in a ticketing system.
Since feedback are free-form, it’s useful to have some conventions or templates to find out what a remark ought to seem like, however mixing conventions falls into the class of unhealthy feedback.
Did you touch upon code throughout growth? Don’t commit it — resolve the issue and take out the remark earlier than you confuse others within the staff.
Past Annotations
Up so far, you’ve realized about utilizing feedback as annotations. Nonetheless, since feedback haven’t any predefined format, you possibly can lengthen their use by remodeling your humble remark annotation into a knowledge file or specialised doc. Listed here are some good examples.
LICENSE Feedback
LICENSE is a kind of remark that signifies phrases and circumstances for the code. You’ll see these particularly usually in open-source code. Right here’s an instance from the MIT LICENSE:
/*
THE SOFTWARE IS PROVIDED “AS IS”, WITHOUT WARRANTY OF ANY KIND, EXPRESS
OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,
DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE
USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
Since feedback are free-form, the best way the remark is typed can take many shapes. Right here’s an instance from Apple:
//===---------------------------------------------------------------===//
//
// This supply file is a part of the Swift open supply challenge
//
// Copyright (c) 2015-2016 Apple Inc. and the Swift challenge authors
// Licensed beneath Apache License v2.0 with Runtime Library Exception
//
// See http://swift.org/LICENSE.txt for license info
// See http://swift.org/CONTRIBUTORS.txt for the checklist of Swift challenge authors
//
//===---------------------------------------------------------------===//
TEMPORARY CODE-OUT Feedback
Programmers use the TEMPORARY CODE-OUT remark as a software for debugging. By taking a block or line of code out of the compile course of, you possibly can alter which code executes and which doesn’t. This technique is often used when doing a process-of-elimination debug. For instance (solely constructing for iOS):
let bundle = Package deal(
title: "CommentOut",
platforms: [
.iOS(.v14), /* .tvOS(.v14), .watchOS(.v7), .macOS(.v11) */
],
merchandise: [
.library(name: "CommentOut", targets: ["CommentOut"])
],
targets: [
.target(name: "CommentOut")
]
)
DECORATIVE Feedback
A DECORATIVE or SEPARATOR remark can separate a file or blocks of code by some class or perform. This helps customers discover code extra simply. Right here’s an instance:
/**************************************************
* Huffman Implementation Helpers *
**************************************************/
LOGIC Feedback
LOGIC feedback doc the rationale you selected when creating the code. This goes past what the code exhibits, like “What was that worth assigned?”
For instance (from swift-package-manager/Sources/Instructions/SwiftRunTool.swift, traces 287-288):
personal func execute(path: String, args: [String]) throws -> By no means {
#if !os(Home windows)
// On platforms aside from Home windows, sign(SIGINT, SIG_IGN) is used for dealing with SIGINT by DispatchSourceSignal,
// however this course of is about to get replaced by exec, so SIG_IGN have to be returned to default.
sign(SIGINT, SIG_DFL)
#endif
attempt TSCBasic.exec(path: path, args: args)
}
On this case, the execute
perform accommodates compile conditional directions that may both embody or skip the sign name. The remark accommodates the reason as to why it does this.
Superior Types of Feedback
Feedback can maintain specialised content material, usually formatted identical to a knowledge file could be: a file throughout the code. They will also be easy flags, which supply code parser instruments can reply to in several methods.
Flag Feedback
Flag feedback are usually utilized by linters; they allow or disable options. Right here’s how SwiftLint disables options utilizing flag feedback:
struct GitHubUserInfo: Content material {
let title: String
// swiftlint:disable identifier_name
let avatar_url: String?
// swiftlint:allow identifier_name
}
Compilers themselves additionally use flag feedback as a type of setup. Within the instance beneath, you see a Python script run as a CLI script and in UTF-8 format:
#!/usr/bin/env python3
# -*- coding: UTF-8 -*-
Word: The primary remark line in scripts has a proper title: the shebang. Its objective is to point which interpreter the working system ought to use when it executes the script. It all the time begins with a #!.
TASK Feedback
Built-in growth environments (IDEs), textual content editors and a few CLI instruments are in a position to learn TASK feedback left by programmers in a format that signifies an motion to be carried out by the programmer sooner or later, akin to TODO:
// TODO: Swap Mannequin permissions
// FIXME: Convert strings to Enum
DOCUMENT GENERATION Feedback
DOCUMENT GENERATION feedback permit a software to parse the supply code and output a formatted doc — usually HTML — that finish customers can use to browse courses, strategies, and many others. Right here’s an instance from JavaDoc:
/**
* @param string the string to be transformed
* @param sort the sort to transform the string to
* @param <T> the kind of the aspect
* @param <V> the worth of the aspect
*/
<T, V extends T> V convert(String string, Class<T> sort) {
}
Different instruments can generate documentation that’s seen by IDEs and proven to the person throughout the code. One such software is Documentation Markup (DocC). That is significantly helpful for APIs Apple makes use of with its personal libraries. Right here’s an instance from the open-source SwiftGD challenge:
/// Exports the picture as `Information` object in specified raster format.
///
/// - Parameter format: The raster format of the returning picture knowledge (e.g. as jpg, png, ...). Defaults to `.png`
/// - Returns: The picture knowledge
/// - Throws: `Error` if the export of `self` in specified raster format failed.
public func export(as format: ExportableFormat = .png) throws -> Information {
return attempt format.knowledge(of: internalImage)
}
CDATA Feedback
CDATA feedback embed formatted knowledge inside XML and XHTML recordsdata. You should utilize a number of differing kinds with these feedback, akin to pictures, code, XML inside XML, and many others.:
<![CDATA[<sender>John Smith</sender>]]>
From Wikipedia’s article on Pc Programming Feedback, you might have the RESOURCE inclusion sort of remark: “Logos, diagrams, and flowcharts consisting of ASCII artwork constructions could be inserted into supply code formatted as a remark”. For instance:
<useful resource id="ProcessDiagram000">
<![CDATA[
HostApp (Main_process)
|
V
script.wsf (app_cmd) --> ClientApp (async_run, batch_process)
|
|
V
mru.ini (mru_history)
]]>
</useful resource>
Having Enjoyable With Feedback
Simply because feedback aren’t included with an app doesn’t imply you possibly can’t have enjoyable with them. Life classes, jokes, poetry, tic-tac-toe video games and a few coworker banter have all made it into code feedback. Right here’s an instance from py_easter_egg_zen.py:
import this
# The Zen of Python, by Tim Peters
# Lovely is best than ugly.
# Specific is best than implicit.
# Easy is best than advanced.
# Complicated is best than difficult.
# Flat is best than nested.
# Sparse is best than dense.
# Readability counts.
# Particular circumstances aren't particular sufficient to interrupt the foundations.
# Though practicality beats purity.
# Errors ought to by no means cross silently.
# Until explicitly silenced.
# Within the face of ambiguity, refuse the temptation to guess.
# There must be one-- and ideally just one --obvious technique to do it.
# Though that approach will not be apparent at first until you are Dutch.
# Now could be higher than by no means.
# Though by no means is commonly higher than *proper* now.
# If the implementation is tough to clarify, it is a unhealthy concept.
# If the implementation is simple to clarify, it might be a good suggestion.
Vital: Easter egg feedback aren’t welcome in all supply codes. Please confer with the challenge or repository coverage or your worker handbook earlier than doing any of those.
Key Takeaways
Immediately, you realized that there’s extra to feedback than it appears. As soon as thought-about an afterthought, they’re now acknowledged a useful communication software for programming.
- They assist doc and protect data for future code upkeep.
- There are good feedback and unhealthy feedback.
- Feedback may help create automated documentation.
Now, it’s time to observe! The one technique to get good at feedback is by leaping in and including them.
By making feedback a part of your programming thought course of, they not change into an additional step to carry out. Listed here are some methods to observe:
- Doc your present initiatives and be aware of any requirements set for the challenge or repository.
- Use a linter on all of your initiatives to maintain your formatting and conventions in test.
- Discover a doc generator and publish your work.
You’ll be able to observe and contribute whereas supporting an open-source challenge that wants assist with feedback and documentation.
The place to Go From Right here?
In the event you’d prefer to study extra about commenting, take a look at these articles and sources. A lot of them had been consulted within the writing of this text.
We hope you’ve loved this have a look at commenting! Do you might have questions, recommendations or tricks to share? Be part of us within the discussion board for this text. You’ll be able to all study from one another.
In regards to the Creator
Roberto Machorro has been programming professionally for over 25 years, and commenting code simply as lengthy. He has championed documenting in-house, third-party and open supply code out of frustration for having to take care of badly written code or battle with unavailable documentation. He bought began with library paperwork utilizing HeaderDoc, JavaDoc and now having fun with DocC.