Introduction
Resume parsing, a priceless instrument utilized in real-life situations to simplify and streamline the hiring course of, has grow to be important for busy hiring managers and human assets professionals. By automating the preliminary screening of resumes utilizing SpaCy‘s magic , a resume parser acts as a wise assistant, leveraging superior algorithms and pure language processing methods to extract key particulars reminiscent of contact info, training historical past, work expertise, and abilities.
This structured information permits recruiters to effectively consider candidates, seek for particular {qualifications}, and combine the parsing expertise with applicant monitoring programs or recruitment software program. By saving time, lowering errors, and facilitating knowledgeable decision-making, resume parsing expertise revolutionizes the resume screening course of and enhances the general recruitment expertise.
Take a look at the Github Depository right here.
Studying Targets
Earlier than we dive into the technical particulars, let’s define the educational aims of this information:
- Perceive the idea of resume parsing and its significance within the recruitment course of.
- Discover ways to arrange the event setting for constructing a resume parser utilizing spaCy.
- Discover methods to extract textual content from resumes in several codecs.
- Implement strategies to extract contact info, together with cellphone numbers and e-mail addresses, from resume textual content.
- Develop abilities to establish and extract related abilities talked about in resumes.
- Achieve data on extracting academic {qualifications} from resumes.
- Make the most of spaCy and its matcher to extract the candidate’s identify from resume textual content.
- Apply the discovered ideas to parse a pattern resume and extract important info.
- Recognize the importance of automating the resume parsing course of for environment friendly recruitment.
Now, let’s delve into every part of the information and perceive tips on how to accomplish these aims.
This text was revealed as part of the Knowledge Science Blogathon.
What’s SpaCy?
SpaCy, a strong open-source library for pure language processing (NLP) in Python, is a priceless instrument within the context of resume parsing. It gives pre-trained fashions for duties like named entity recognition (NER) and part-of-speech (POS) tagging, permitting it to successfully extract and categorize info from resumes. With its linguistic algorithms, rule-based matching capabilities, and customization choices, SpaCy stands out as a most well-liked alternative for its pace, efficiency, and ease of use.
By using SpaCy for resume parsing, recruiters can save effort and time by automating the extraction of key particulars from resumes. The library’s correct information extraction reduces human error and ensures constant outcomes, enhancing the general high quality of the candidate screening course of. Furthermore, SpaCy’s superior NLP capabilities allow refined evaluation, offering priceless insights and contextual info that support recruiters in making knowledgeable assessments.
One other benefit of SpaCy is its seamless integration with different libraries and frameworks, reminiscent of scikit-learn and TensorFlow. This integration opens up alternatives for additional automation and superior evaluation, permitting for the applying of machine studying algorithms and extra in depth information processing.
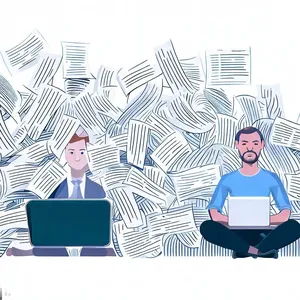
In abstract, SpaCy is a strong NLP library utilized in resume parsing because of its capacity to extract and analyze info from resumes successfully. Its pre-trained fashions, linguistic algorithms, and rule-based matching capabilities make it a priceless instrument for automating the preliminary screening of candidates, saving time, lowering errors, and enabling deeper evaluation.
Be aware: I’ve developed a resume parser utilizing two distinct approaches. The primary technique, accessible on my GitHub account, gives an easy method. Within the second technique, I leveraged the exceptional capabilities of spaCy, an distinctive pure language processing library. Via this integration, I’ve enhanced the resume parsing course of, effortlessly extracting priceless info from resumes.
Right here is the whole code from Github.
Organising the Improvement Setting
Earlier than we will begin constructing our resume parser, we have to arrange our improvement setting. Listed here are the steps to get began:
- Set up Python: Make sure that Python is put in in your system. You possibly can obtain the newest model of Python from the official Python web site (https://www.python.org) and observe the set up directions on your working system.
- Set up spaCy: Open a command immediate or terminal and use the next command to put in spaCy:
!pip set up spacy
- Obtain spaCy’s English Language Mannequin: spaCy offers pre-trained fashions for various languages. We’ll be utilizing the English language mannequin for our resume parser. Obtain the English language mannequin by operating the next command:
python -m spacy obtain en_core_web_sm
- Set up extra libraries: We’ll be utilizing the pdfminer.six library to extract textual content from PDF resumes. Set up it utilizing the next command:
pip set up pdfminer.six
After you have accomplished these steps, your improvement setting will likely be prepared for constructing the resume parser.
Step one in resume parsing is to extract the textual content from resumes in varied codecs, reminiscent of PDF or Phrase paperwork. We’ll be utilizing the pdfminer.six library to extract textual content from PDF resumes. Right here’s a operate that takes a PDF file path as enter and returns the extracted textual content:
import re
from pdfminer.high_level import extract_text
def extract_text_from_pdf(pdf_path):
return extract_text(pdf_path)
You possibly can name this operate with the trail to your PDF resume and acquire the extracted textual content.
Contact info, together with cellphone numbers, e-mail addresses, and bodily addresses, is essential for reaching out to potential candidates. Extracting this info precisely is an important a part of resume parsing. We will use common expressions to match patterns and extract contact info.
Let’s outline a operate to extract a contact quantity from the resume textual content:
import re
def extract_contact_number_from_resume(textual content):
contact_number = None
# Use regex sample to discover a potential contact quantity
sample = r"b(?:+?d{1,3}[-.s]?)?(?d{3})?[-.s]?d{3}[-.s]?d{4}b"
match = re.search(sample, textual content)
if match:
contact_number = match.group()
return contact_number
We outline a regex sample to match the contact quantity format we’re on the lookout for. The sample r”b(?:+?d{1,3}[-.s]?)?(?d{3})?[-.s]?d{3}[-.s]?d{4}b” is used on this case.
Sample Elements
Right here’s a breakdown of the sample parts:
- b: Matches a phrase boundary to make sure the quantity shouldn’t be half of a bigger phrase.
- (?:+?d{1,3}[-.s]?)?: Matches an optionally available nation code (e.g., +1 or +91) adopted by an optionally available separator (-, ., or area).
- (?: Matches an optionally available opening parenthesis for the world code.
- d{3}: Matches precisely three digits for the world code.
- )?: Matches an optionally available closing parenthesis for the world code.
- [-.s]?: Matches an optionally available separator between the world code and the following a part of the quantity.
- d{3}: Matches precisely three digits for the following a part of the quantity.
- [-.s]?: Matches an optionally available separator between the following a part of the quantity and the ultimate half.
- d{4}: Matches precisely 4 digits for the ultimate a part of the quantity.
- b: Matches a phrase boundary to make sure the quantity shouldn’t be half of a bigger phrase.
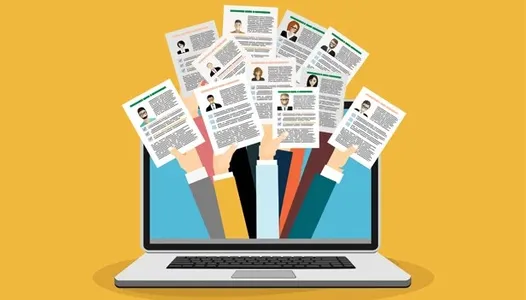
The supplied regex sample is designed to match a standard format for contact numbers. Nonetheless, it’s essential to notice that contact quantity codecs can fluctuate throughout completely different nations and areas. The sample supplied is a common sample that covers frequent codecs, however it could not seize all doable variations.
If you’re parsing resumes from particular areas or nations, it’s really useful to customise the regex sample to match the precise contact quantity codecs utilized in these areas. You could want to think about nation codes, space codes, separators, and quantity size variations.
It’s additionally value mentioning that cellphone quantity codecs can change over time, so it’s an excellent apply to periodically assessment and replace the regex sample to make sure it stays correct.
Discover Contact Quantity with Nation Code
# That is One other technique to seek out contact quantity with nation code +91
sample = [
{"ORTH": "+"},
{"ORTH": "91"},
{"SHAPE": "dddddddddd"}
]
For extra info go throght spaCy’s documentation.
On the finish of the article, we’re going to focus on some frequent issues relating to to completely different codes we want throughout resume parser coding.
Along with the contact quantity, extracting the e-mail handle is important for communication with candidates. We will once more use common expressions to match patterns and extract the e-mail handle. Right here’s a operate to extract the e-mail handle from the resume textual content:
import re
def extract_email_from_resume(textual content):
e-mail = None
# Use regex sample to discover a potential e-mail handle
sample = r"b[A-Za-z0-9._%+-][email protected][A-Za-z0-9.-]+.[A-Za-z]{2,}b"
match = re.search(sample, textual content)
if match:
e-mail = match.group()
return e-mail
The regex sample used on this code is r”b[A-Za-z0-9._%+-][email protected][A-Za-z0-9.-]+.[A-Za-z]{2,}b”. Let’s break down the sample:
- b: Represents a phrase boundary to make sure that the e-mail handle shouldn’t be half of a bigger phrase.
- [A-Za-z0-9._%+-]+: Matches a number of occurrences of alphabetic characters (each uppercase and lowercase), digits, intervals, underscores, p.c indicators, or hyphens. This half represents the native a part of the e-mail handle earlier than the “@” image.
- @: Matches the “@” image.
- [A-Za-z0-9.-]+: Matches a number of occurrences of alphabetic characters (each uppercase and lowercase), digits, intervals, or hyphens. This half represents the area identify (e.g., gmail, yahoo) of the e-mail handle.
- .: Matches a interval (dot) character.
- [A-Za-z]{2,}: Matches two or extra occurrences of alphabetic characters (each uppercase and lowercase). This half represents the top-level area (e.g., com, edu) of the e-mail handle.
- b: Represents one other phrase boundary to make sure the e-mail handle shouldn’t be half of a bigger phrase.
#Different code
def extract_email_from_resume(textual content):
e-mail = None
# Break up the textual content into phrases
phrases = textual content.break up()
# Iterate by way of the phrases and verify for a possible e-mail handle
for phrase in phrases:
if "@" in phrase:
e-mail = phrase.strip()
break
return e-mail
Whereas the choice code is easier to grasp for newbies, it could not deal with extra advanced e-mail handle codecs or think about e-mail addresses separated by particular characters. The preliminary code with the regex sample offers a extra complete method to establish potential e-mail addresses based mostly on frequent conventions.
Figuring out the talents talked about in a resume is essential for figuring out the candidate’s {qualifications}. We will create a listing of related abilities and match them in opposition to the resume textual content to extract the talked about abilities. Let’s outline a operate to extract abilities from the resume textual content:
import re
def extract_skills_from_resume(textual content, skills_list):
abilities = []
for ability in skills_list:
sample = r"b{}b".format(re.escape(ability))
match = re.search(sample, textual content, re.IGNORECASE)
if match:
abilities.append(ability)
return abilities
Right here’s a breakdown of the code and its sample:
- The operate takes two parameters: textual content (the resume textual content) and skills_list (a listing of abilities to seek for).
- It initializes an empty listing abilities to retailer the extracted abilities.
- It iterates by way of every ability within the skills_list.
- Contained in the loop, a regex sample is constructed utilizing re.escape(ability) to flee any particular characters current within the ability. This ensures that the sample will match the precise ability as an entire phrase.
- The sample is enclosed between b phrase boundaries. This ensures that the ability shouldn’t be half of a bigger phrase and is handled as a separate entity.
- The re.IGNORECASE flag is used with re.search() to carry out a case-insensitive search. This permits matching abilities no matter their case (e.g., “Python” or “python”).
- The re.search() operate is used to seek for the sample inside the resume textual content.
- If a match is discovered, indicating the presence of the ability within the resume, the ability is appended to the talents listing.
- After iterating by way of all the talents within the skills_list, the operate returns the extracted abilities as a listing.
Be aware: The regex sample used on this code assumes that abilities are represented as complete phrases and never as elements of bigger phrases. It might not deal with variations in ability representations or account for abilities talked about in a unique format.
If you wish to discover some particular abilities from resume, then this code will likely be usefull.
if __name__ == '__main__':
textual content = extract_text_from_pdf(pdf_path)
# Record of predefined abilities
skills_list = ['Python', 'Data Analysis', 'Machine Learning', 'Communication', 'Project Management', 'Deep Learning', 'SQL', 'Tableau']
extracted_skills = extract_skills_from_resume(textual content, skills_list)
if extracted_skills:
print("Abilities:", extracted_skills)
else:
print("No abilities discovered")
Exchange pdf_path together with your file location. skills_list will be up to date as your want.
Training {qualifications} play an important position within the recruitment course of. We will match particular training key phrases in opposition to the resume textual content to establish the candidate’s academic background. Right here’s a operate to extract training info from the resume textual content:
import re
def extract_education_from_resume(textual content):
training = []
# Record of training key phrases to match in opposition to
education_keywords = ['Bsc', 'B. Pharmacy', 'B Pharmacy', 'Msc', 'M. Pharmacy', 'Ph.D', 'Bachelor', 'Master']
for key phrase in education_keywords:
sample = r"(?i)b{}b".format(re.escape(key phrase))
match = re.search(sample, textual content)
if match:
training.append(match.group())
return training
#Different Code:
def extract_text_from_pdf(pdf_path):
return extract_text(pdf_path)
def extract_education_from_resume(textual content):
training = []
# Use regex sample to seek out training info
sample = r"(?i)(?:Bsc|bB.w+|bM.w+|bPh.D.w+|bBachelor(?:'s)?|bMaster(?:'s)?|bPh.D)s(?:w+s)*w+"
matches = re.findall(sample, textual content)
for match in matches:
training.append(match.strip())
return training
if __name__ == '__main__':
textual content = extract_text_from_pdf(r"C:UsersSANKETDownloadsUntitled-resume.pdf")
extracted_education = extract_education_from_resume(textual content)
if extracted_education:
print("Training:", extracted_education)
else:
print("No training info discovered")
#Be aware : You have to create sample as per your requirement.
Figuring out the candidate’s identify from the resume is crucial for personalization and identification. We will use spaCy and its sample matching capabilities to extract the candidate’s identify. Let’s outline a operate to extract the identify utilizing spaCy:
import spacy
from spacy.matcher import Matcher
def extract_name(resume_text):
nlp = spacy.load('en_core_web_sm')
matcher = Matcher(nlp.vocab)
# Outline identify patterns
patterns = [
[{'POS': 'PROPN'}, {'POS': 'PROPN'}], # First identify and Final identify
[{'POS': 'PROPN'}, {'POS': 'PROPN'}, {'POS': 'PROPN'}], # First identify, Center identify, and Final identify
[{'POS': 'PROPN'}, {'POS': 'PROPN'}, {'POS': 'PROPN'}, {'POS': 'PROPN'}] # First identify, Center identify, Center identify, and Final identify
# Add extra patterns as wanted
]
for sample in patterns:
matcher.add('NAME', patterns=[pattern])
doc = nlp(resume_text)
matches = matcher(doc)
for match_id, begin, finish in matches:
span = doc[start:end]
return span.textual content
return None
#Different Technique:
def extract_text_from_pdf(pdf_path):
return extract_text(pdf_path)
def extract_name_from_resume(textual content):
identify = None
# Use regex sample to discover a potential identify
sample = r"(b[A-Z][a-z]+b)s(b[A-Z][a-z]+b)"
match = re.search(sample, textual content)
if match:
identify = match.group()
return identify
if __name__ == '__main__':
textual content = extract_text_from_pdf(pdf_path)
identify = extract_name_from_resume(textual content)
if identify:
print("Identify:", identify)
else:
print("Identify not discovered")
The regex sample r”(b[A-Z][a-z]+b)s(b[A-Z][a-z]+b)” is used to discover a potential identify sample within the resume textual content.
The sample consists of two elements enclosed in parentheses:
- (b[A-Z][a-z]+b): This half matches a phrase beginning with an uppercase letter adopted by a number of lowercase letters. It represents the primary identify.
- s: This half matches a single whitespace character to separate the primary and final names.
- (b[A-Z][a-z]+b): This half matches a phrase beginning with an uppercase letter adopted by a number of lowercase letters. It represents the final identify.
Exchange pdf_path together with your file path.
Parsing a Pattern Resume
To place every part collectively, let’s create a pattern resume and parse it utilizing our resume parser capabilities. Right here’s an instance:
if __name__ == '__main__':
resume_text = "John DoennContact Data: 123-456-7890, [email protected]nnSkills: Python, Knowledge Evaluation, CommunicationnnEducation: Bachelor of Science in Pc SciencennExperience: Software program Engineer at XYZ Firm"
print("Resume:")
print(resume_text)
identify = extract_name(resume_text)
if identify:
print("Identify:", identify)
else:
print("Identify not discovered")
contact_number = extract_contact_number_from_resume(resume_text)
if contact_number:
print("Contact Quantity:", contact_number)
else:
print("Contact Quantity not discovered")
e-mail = extract_email_from_resume(resume_text)
if e-mail:
print("Electronic mail:", e-mail)
else:
print("Electronic mail not discovered")
skills_list = ['Python', 'Data Analysis', 'Machine Learning', 'Communication']
extracted_skills = extract_skills_from_resume(resume_text, skills_list)
if extracted_skills:
print("Abilities:", extracted_skills)
else:
print("No abilities discovered")
extracted_education = extract_education_from_resume(resume_text)
if extracted_education:
print("Training:", extracted_education)
else:
print("No training info discovered")
Challenges in Resume Parcer Improvement
Creating a resume parser is usually a advanced job with a number of challenges alongside the way in which. Listed here are some frequent issues we encountered and strategies for addressing them in a extra human-friendly method:
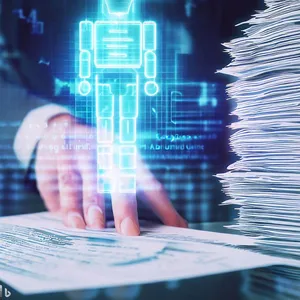
One of many predominant challenges is extracting textual content precisely from resumes, particularly when coping with PDF codecs. At occasions, the extraction course of could distort or introduce errors within the extracted textual content, ensuing within the retrieval of incorrect info. To beat this, we have to depend on dependable libraries or instruments particularly designed for PDF textual content extraction, reminiscent of pdfminer, to make sure correct outcomes.
Coping with Formatting Variations
Resumes are available in varied codecs, layouts, and constructions, making it tough to extract info constantly. Some resumes could use tables, columns, or unconventional formatting, which might complicate the extraction course of. To deal with this, we have to think about these formatting variations and make use of methods like common expressions or pure language processing to precisely extract the related info.
Extracting the candidate’s identify precisely is usually a problem, particularly if the resume comprises a number of names or advanced identify constructions. Completely different cultures and naming conventions additional add to the complexity. To deal with this, we will make the most of approaches like named entity recognition (NER) utilizing machine studying fashions or rule-based matching. Nonetheless, it’s essential to deal with completely different naming conventions correctly to make sure correct extraction.
Extracting contact info reminiscent of cellphone numbers and e-mail addresses will be liable to false positives or lacking particulars. Common expressions will be useful for sample matching, however they could not cowl all doable variations. To reinforce accuracy, we will incorporate strong validation methods or leverage third-party APIs to confirm the extracted contact info.
Figuring out abilities talked about within the resume precisely is a problem because of the huge array of doable abilities and their variations. Utilizing a predefined listing of abilities or using methods like key phrase matching or pure language processing can support in extracting abilities successfully. Nonetheless, it’s essential to frequently replace and refine the ability listing to accommodate rising abilities and industry-specific terminology.
Extracting training particulars from resumes will be advanced as they are often talked about in varied codecs, abbreviations, or completely different orders. Using a mixture of normal expressions, key phrase matching, and contextual evaluation will help establish training info precisely. It’s important to think about the restrictions of sample matching and deal with variations appropriately.
Dealing with Multilingual Resumes
Coping with resumes in several languages provides one other layer of complexity. Language detection methods and language-specific parsing and extraction strategies allow the dealing with of multilingual resumes. Nonetheless, it’s essential to make sure language assist for the libraries or fashions used within the parser.
When growing a resume parser, combining methods like rule-based matching, common expressions, and pure language processing can improve info extraction accuracy. We advocate testing and refining the parser by utilizing numerous resume samples to establish and handle potential points. Take into account using open-source resume parser libraries like spaCy or NLTK, which supply pre-trained fashions and parts for named entity recognition, textual content extraction, and language processing. Bear in mind, constructing a sturdy resume parser is an iterative course of that improves with person suggestions and real-world information.
Conclusion
In conclusion, resume parsing with spaCy gives vital advantages for recruiters by saving time, streamlining the hiring course of, and enabling extra knowledgeable selections. Methods reminiscent of textual content extraction, contact element capturing, and leveraging spaCy’s sample matching with common expressions and key phrase matching guarantee correct retrieval of data, together with abilities, training, and candidate names. Palms-on expertise confirms the sensible software and potential of resume parsing, in the end revolutionizing recruitment practices. By implementing a spaCy resume parser, recruiters can improve effectivity and effectiveness, main to raised hiring outcomes.
Keep in mind that constructing a resume parser requires a mixture of technical abilities, area data, and a focus to element. With the best method and instruments, you may develop a strong resume parser that automates the extraction of essential info from resumes, saving effort and time within the recruitment course of.
Incessantly Requested Questions
A. Resume parsing is a expertise that enables automated extraction and evaluation of data from resumes. It includes parsing or breaking down a resume into structured information, enabling recruiters to effectively course of and search by way of numerous resumes.
A. Resume parsing sometimes includes utilizing pure language processing (NLP) methods to extract particular information factors from resumes. It makes use of algorithms and rule-based programs to establish and extract info reminiscent of contact particulars, abilities, work expertise, and training.
A. Resume parsing gives a number of advantages, together with time-saving for recruiters by automating the extraction of crucial info, improved accuracy in capturing information, streamlined candidate screening and matching, and enhanced general effectivity within the recruitment course of.
A. Some challenges in resume parsing embody precisely decoding and extracting info from resumes with various codecs and layouts, coping with inconsistencies in how candidates current their info, and dealing with potential errors or misinterpretations within the parsing course of.
Sure, there are numerous specialised instruments and software program accessible for resume parsing. Some well-liked choices embody Applicant Monitoring Techniques (ATS), which frequently embody resume parsing capabilities, and devoted resume parsing software program that may combine with current recruitment programs.
The media proven on this article shouldn’t be owned by Analytics Vidhya and is used on the Creator’s discretion.