Python is a broadly used high-level, general-purpose programming language. The language presents many advantages to builders, making it a well-liked selection for a variety of purposes together with internet growth, backend growth, machine studying purposes, and all cutting-edge software program know-how, and is most popular for each rookies in addition to skilled software program builders.
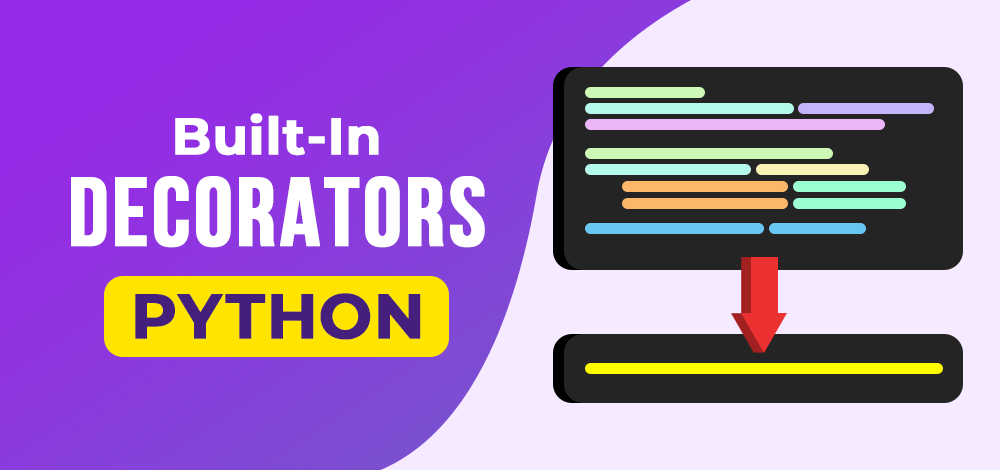
Python decorators are useful instruments for writing clear, versatile, reusable, and maintainable code, and in consequence, are used extensively by software program builders. Decorators mean you can lengthen the conduct of a perform or class with out altering its supply code. They’re additionally broadly utilized in many Python frameworks and libraries, corresponding to Flask, Django, and SQLAlchemy. Moreover, there are lots of superior built-in Python decorators that make our lives a lot simpler as a result of we are able to add difficult functionalities to current capabilities or courses with only one line of code. Decorators are generally utilized in Python for caching, logging, timing, authentication, authorization, and validation. With this being stated let’s proceed the article on the finest Python built-in decorators to help you in having a a lot simpler time studying and constructing with Python.
Prime 10 Python Constructed-In Decorators That Optimize PythonCode Considerably
1. @classmethod
Class strategies are strategies which might be certain to a specific class. These strategies usually are not tied to any situations of the category. The ‘@classmethod’ is a built-in decorator that’s used to create a category technique.
Instance:
Python3
|
Output:
This can be a Class technique Worth of sophistication variable 10
Within the above implementation, a category GFGClass and a category technique gfg_class_method are outlined. The @classmethod decorator is used to point that it is a class technique. The cls parameter within the technique definition refers back to the class itself, by which the class-level variables (instance: class_val) and strategies could be accessed. When gfg_class_method() technique is known as it logs the message, “This can be a Class technique” adopted by the worth of the class_var.
Additionally Learn: @classmethod in Python
2. @abstractmethod
Summary strategies are declared inside an Summary class with none technique definition. This technique is supposed to be applied by the bottom class who implements the mother or father summary class. The @abstractmethod decorator offered by abc module is used to implement summary strategies.
Python3
|
Output:
100
Within the above implementation, The Form class is outlined as an summary base class by inheriting from ABC and utilizing the @abstractmethod decorator to declare the space() technique as summary. The Sq. class is a concrete subclass of Form that implements the realm() technique to calculate the realm of a sq.. By inheriting from Form, Sq. should implement all the summary strategies declared in Form. On this case, the Sq. class implements space() by squaring the size of 1 aspect of the sq..
Additionally, Learn: @abstractmethod in Python
3. @staticmethod
Static strategies are merely utility strategies which might be not certain to a category or an occasion of the category. The ‘@staticmethod‘ is a built-in decorator that’s used to create a static technique.
Instance:
Python3
|
Output:
This can be a static technique
Within the above implementation, a category GFGClass and a static technique gfg_static_method are outlined. The @staticmethod decorator is used to point that it is a static technique. Not like class strategies, static strategies don’t have a particular first parameter that refers back to the class itself. Static strategies are sometimes used for utility capabilities that don’t rely on the category or the occasion of the category. When the gfg_static_method() technique is known as on the GFGClass class, the message “This can be a static technique” is logged to the console.
Additionally, Learn: @staticmethod in Python
4. @atexit.register
The ‘@atexit.register’ decorator is used to name the perform when this system is exiting. The performance is offered by the ‘atexit’ module. This perform can be utilized to carry out the clean-up duties, earlier than this system exits. Clear-ups are the duties which have been completed in an effort to launch the assets corresponding to opened information or database connections, that have been getting used throughout this system execution.
Instance:
Python3
|
Output:
Hi there, Geeks! Bye, Geeks!
Within the above implementation, a perform gfg_exit_handler() is outlined which makes use of the @atexit.register decorator that registers it to be known as when this system is exiting. After we run this system, the message “Hi there, Geeks!” is logged into the console. When completes its execution both or by being interrupted, the gfg_exit_handler() perform is known as and the message “Bye, Geeks!” is logged to the console. The gfg_exit_handler() perform could be helpful for performing cleanup duties or closing assets (corresponding to information or database connections) when a program is exiting.
Additionally, Learn: @atexit.register in Python
5. @typing.last
The ‘@last’ decorator from the typing module is used to outline the ultimate class or technique. Ultimate courses can’t be inherited and in the same manner, last strategies can’t be overridden.
Instance:
Python3
|
Within the above implementation, the @typing.last decorator is used to point {that a} technique or class shouldn’t be overridden or subclassed, respectively. The Base class has a completed() technique marked as last utilizing @typing.last. The Youngster is a subclass of Base that makes an attempt to override the completed() technique, which raises an error when the code is executed. Equally, the Geek class is marked as last utilizing @typing.last, indicating that it shouldn’t be subclassed. The Different class makes an attempt to subclass Leaf, which additionally raises an error when the code is executed.
Utilizing @typing.last will help stop unintended adjustments to crucial elements of your code and make it extra strong by imposing constraints on how it may be used.
Additionally Learn: @typing.last in Python
6. @enum.distinctive
Enumeration or Enum is a set of distinctive names which have a singular worth. They’re helpful if we wish to outline a set of constants which have a selected that means. The ‘@distinctive’ decorator offered by enum module is used to make sure that enumeration members are distinctive.
Instance:
Python3
|
Within the above implementation, an enum class ‘Days’ is outlined utilizing the category ‘Enum‘. The category has 4 members, MONDAY, TUESDAY, WEDNESDAY, and THURSDAY, with values of 1,2,3,2 respectively.
Nonetheless, as a result of TUESDAY and THURSDAY each have the worth 2, the @distinctive decorator raises a ValueError with the message “duplicate values present in <enum ‘Days’>: THURSDAY -> TUESDAY”. This error happens as a result of the @distinctive decorator ensures that the values of the enumeration members are distinctive, and on this case, they don’t seem to be.
Additionally, Learn: @enum in Python
7. @property
Getters and Setters are used throughout the class to entry or replace the worth of the item variable inside that class. The ‘@property’ decorator is used to outline getters and setters for sophistication attributes.
Instance:
Python3
|
Output:
Setter known as Getter Referred to as 10
Within the above implementation, the Geek class has a personal attribute ‘_name‘. This system defines the getter technique ‘title‘ which permits the title to be retrieved utilizing the dot notation. The @title.setter decorator is used to create the setter technique for setting the ‘_name’ worth. The @property and @title.setter decorators outline the “getter” and “setter” strategies for sophistication attributes, which might make it simpler to work with class knowledge.
Additionally, Learn: @property in Python
8. @enum.confirm
Launched in Python 3.11, The ‘@enum.confirm‘ decorator in Python is used to make sure that the values of the members of an enumeration comply with a specific sample or constraint. Some predefined constraints offered by the enum embrace,
- CONTINUES: The CONTINUES modules assist be sure that there isn’t any lacking worth between the highest and lowest worth.
- UNIQUE: The UNIQUE modules assist be sure that every worth has just one title
Instance:
Python3
|
Within the above implementation, two completely different enumeration courses, Days1 and Days2 are outlined utilizing the Enum class from the enum module and the confirm() decorator is utilized to every class.
Within the Days1 class, the confirm() decorator with the UNIQUE argument is used to make sure that every member of the Days1 enumeration class has a singular worth. Nonetheless, as TUESDAY and THURSDAY have the identical worth, a ValueError is raised with the message: “ValueError: aliases present in <enum ‘Days’>: THURSDAY -> TUESDAY“.
Within the Days2 class, the confirm() decorator with the CONTINUOUS argument is used to make sure that every member of the Days2 enumeration class types a steady sequence ranging from the primary member. Nonetheless, for the reason that worth of three is lacking and 4 is straight used, a ValueError is raised with the message: “ValueError: invalid enum ‘Days’: lacking values 3“.
Additionally Learn: @enum in Python
9. @singledispatch
A perform with the identical title however completely different conduct with respect to the kind of argument is a generic perform. The ‘@singledispatch’ decorator in Python is used to create a generic perform. This enables a person to create an overloaded perform that may work with a number of forms of parameters.
Instance:
Python3
|
Output:
Perform Referred to as with an integer Perform Referred to as with an inventory Perform Referred to as with a string Perform Name with single argument
Within the above implementation, a perform known as geek_func() is outlined utilizing the @singledispatch decorator. The @singledispatch decorator tells Python that this perform ought to be dispatched primarily based on the kind of its first argument. 4 extra implementations of geek_func() are outlined utilizing the @geek_func.register decorator. Every implementation is embellished with the kind of argument it handles. When geek_func() is known as with an argument of a specific kind, the implementation embellished with the corresponding kind is known as. When the perform name doesn’t match any of the registered implementations, it defaults to the preliminary implementation.
Additionally, Learn: @singledispatch in Python
10. @lru_cache
This decorator returns the identical as lru_cache(maxsize=None) by creating a skinny wrapper round a dictionary lookup for the perform arguments. As a result of it by no means must take away previous values, that is smaller and sooner than lru_cache() with a dimension restrict.
Instance:
Python3
|
Output:
55 --- 0.0009987354278564453 seconds --- 55 --- 0.0 seconds ---
Additionally, Learn: @lru_cache in Python
Within the above implementation of the Fibonacci sequence, we’re utilizing memoization with the lru_cache decorator from the functools module. The Fibonacci sequence is a sequence of numbers during which every quantity is the sum of the 2 previous ones, ranging from 0 and 1.
The fibonacci perform is taking an integer argument n and returns the n-th quantity within the Fibonacci sequence and If n is lower than 2, then the perform returns n itself. In any other case, it recursively computes the sum of the (n-1)-th and (n-2)-th numbers within the sequence.
Conclusion
Python is a flexible and widely-used programming language, making it an ideal selection for builders to implement code that’s used for quite a lot of functions. This text highlights a number of the hottest and widely-used decorators out there in Python. Decorators are helpful abstractions for extending your code with extra functionalities corresponding to caching, automated retry, charge limiting, logging, and extra. These not solely present highly effective performance but additionally simplify the code. Utilizing decorators can present builders with highly effective instruments for writing clear, concise, and environment friendly code in Python. Whether or not you’re a newbie or an skilled developer, utilizing these decorators will present a great start line for enhancing your code and leveraging the ability of Python.
FAQs on Python Decorators
Q1: What’s a decorator in Python?
Reply:
Python Decorators are very highly effective instruments that enable programmers to increase the conduct of a perform or class.
Q2: The way to create a decorator in Python?
Reply:
A decorator could be created by defining a perform that takes one other perform as an argument and returns a brand new perform that extends or modifies the unique perform’s conduct.
Q3: Can a number of decorators be used for a similar perform?
Reply:
Sure! It’s potential to have a number of decorators on the identical perform.