Progress bars or other forms of indicators play an important position in apps that execute time-consuming duties or operations. It enriches the person expertise by displaying a progress indicator, which permits the person to trace the progress of the operation and get an thought of how lengthy it is going to take to finish.
In SwiftUI, it comes with a built-in part referred to as ProgressView
for builders to current a progress bar or a round indicator to show the progress of a long-running operation, resembling downloading a file or importing knowledge to a server.
The default look of ProgressView
is easy and minimalistic, and it may not match the design and theme of your app. That is the place the ProgressViewStyle
protocol comes into play. You should utilize the ProgressViewStyle
protocol to create customized kinds for the ProgressView
part that match the design and theme of your app.
On this tutorial, I’ll stroll you thru the fundamentals of ProgressView
and present you the best way to customise the look & really feel of the progress view utilizing ProgressViewStyle
.
The Fundamental Implementation of ProgressView
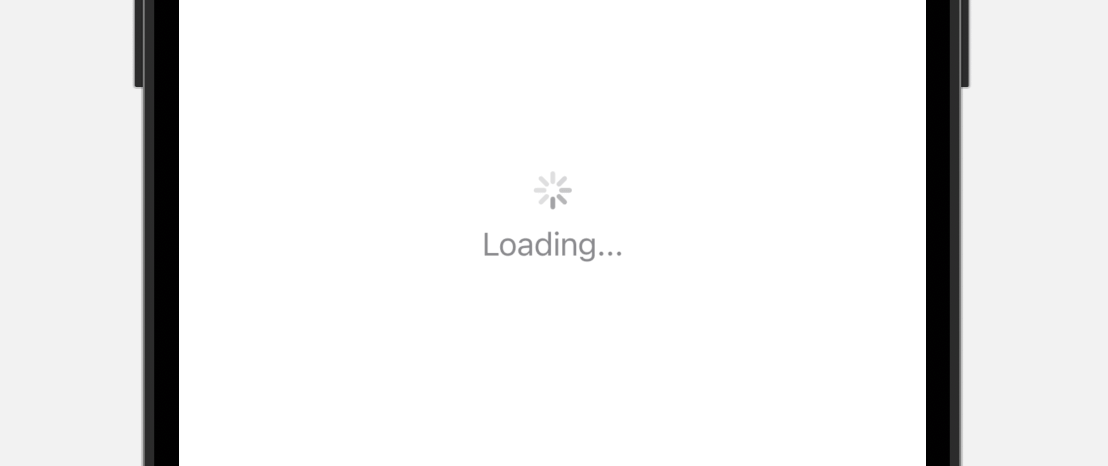
The ProgressView
part may be very simply to make use of. In your SwiftUI apps, you’ll be able to create a progress indicator by writing the next line of code:
Optionally, you’ll be able to add a label to show the standing message like this:
ProgressView() { Â Â Â Â Textual content(“Loading…”) } |
On iOS, the progress view is displayed as a round indicator.
Indeterminate Progress vs Determineate Progress
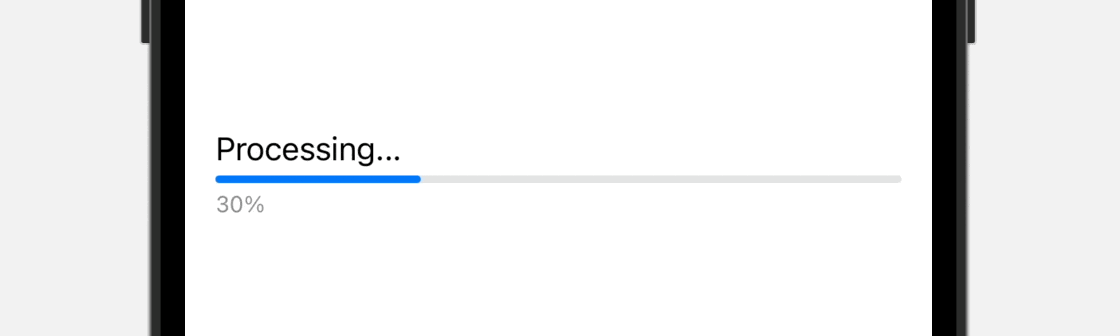
The ProgressView
part gives two kinds of progress indicator to point indeterminate progress and determineate progress. What we simply demonstrated within the earlier part is an indeterminate progress indicator. For duties which have an unknown length, resembling loading or syncing complicated knowledge, we use indeterminate progress indicators, which is displayed as a round exercise indicator.
When doable, use a determinate progress indicator. An indeterminate progress indicator reveals {that a} course of is happening, nevertheless it doesn’t assist individuals estimate how lengthy a job will take. A determinate progress indicator may help individuals resolve whether or not to do one thing else whereas ready for the duty to finish, restart the duty at a distinct time, or abandon the duty.
For a determinate progress indicator, it’s used for duties with a well-defined length resembling file downloads. On iOS, a determinate progress indicator is introduced within the type of a progress bar. To implement it, you simply must instantiate a ProgressView
with the present worth of the progress. Right here is an instance:
By default, the whole worth is 1.0. You may present a price between 0.0 and 1.0 the place 1.0 means 100% full. If you need to use a distinct vary with a distinct whole, you’ll be able to write the code by utilizing one other initializer:
ProgressView(worth: 30, whole: 100) |
Each traces of the codes current the identical progress indicator that reveals 30% full.
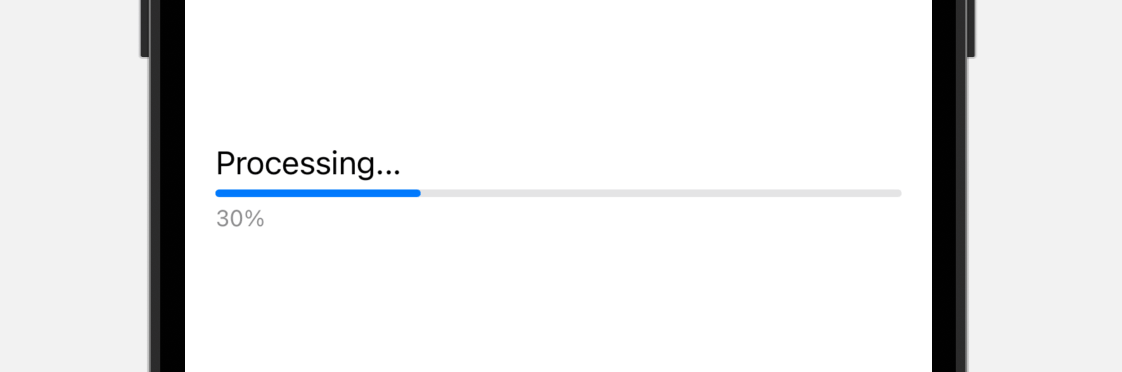
Optionally, you’ll be able to add some labels to show the standing message and present progress by utilizing the label
and currentValueLabel
parameters:
ProgressView(worth: 0.3, Â Â Â Â Â Â Â Â Â Â Â Â label: { Textual content(“Processing…”) }, Â Â Â Â Â Â Â Â Â Â Â Â currentValueLabel: { Textual content(“30%”) }) |
To be able to replace the worth of the progress, you normally create a state variable to carry the present worth of the progress. Here’s a pattern code snippet:
@State non-public var progress = 0.1
var physique: some View {
ProgressView(worth: progress,
label: { Textual content(“Processing…”) },
currentValueLabel: { Textual content(progress.formatted(.%.precision(.fractionLength(0)))) })
.padding()
.job {
Timer.scheduledTimer(withTimeInterval: 1, repeats: true) { _ in
self.progress += 0.1
if self.progress > 1.0 {
self.progress = 0.0
}
}
}
}
}
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
struct ContentView: View {      @State non-public var progress = 0.1      var physique: some View {         ProgressView(worth: progress,                     label: { Textual content(“Processing…”) },                     currentValueLabel: { Textual content(progress.formatted(.%.precision(.fractionLength(0)))) })             .padding()             .job {                 Timer.scheduledTimer(withTimeInterval: 1, repeats: true) { _ in                      self.progress += 0.1                      if self.progress > 1.0 {                         self.progress = 0.0                     }                 }             }     } } |
Altering the Progress View’s Shade and Model
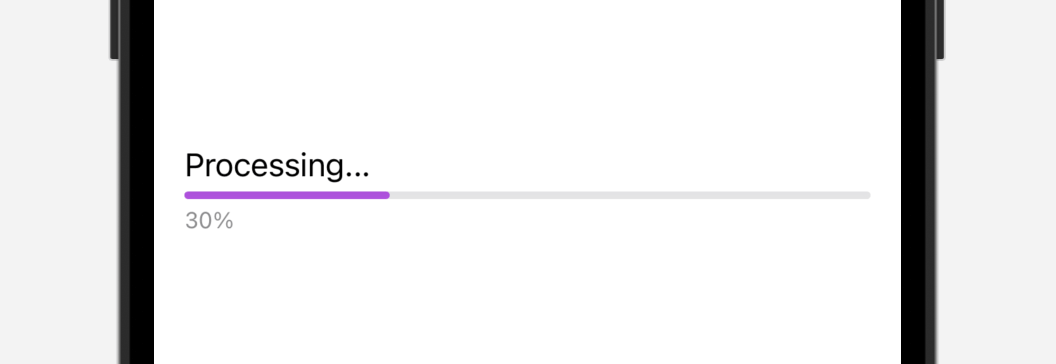
The default progress indicator has a easy design with restricted customization choices. Nevertheless, it’s doable to change the colour of the progress bar by making use of the tint
modifier:
ProgressView(worth: 0.3) Â Â Â Â .tint(.purple) |
A determinate progress indicator is usually displayed as a progress bar. In the event you want to modify its look, you should use the progressViewStyle
modifier. As an illustration, you’ll be able to change from the default linear
model to a round
model, like so:
ProgressView(worth: 0.3, label: { Textual content(“Processing…”) }, currentValueLabel: { Textual content(“30%”) }) Â Â Â Â .progressViewStyle(.round) |
This transforms the progress bar right into a round indicator.
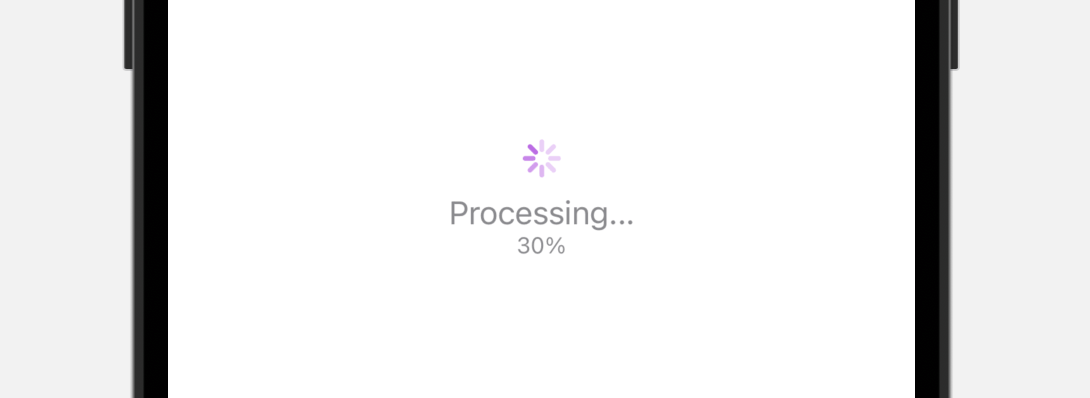
Utilizing ProgressViewStyle for Superior Customizations
Though the default model of the progress view is ample for many purposes, chances are you’ll wish to create distinctive and visually interesting progress bars that match their app’s design and theme. If you wish to create a personalized progress bar that aligns together with your app’s aesthetic, you should use the ProgressViewStyle
protocol in SwiftUI.
ProgressViewStyle
is a strong instrument for creating personalized progress indicators in SwiftUI. With ProgressViewStyle
, you’ll be able to create distinctive and visually interesting progress bars that completely match the design and theme of your app. There are a number of built-in kinds accessible, resembling CircularProgressViewStyle
and LinearProgressViewStyle
. By conforming to the ProgressViewStyle
protocol, you’ll be able to create your personal kinds and apply them to the ProgressView
part. This permits for superior customizations that transcend altering the colour of the progress bar.
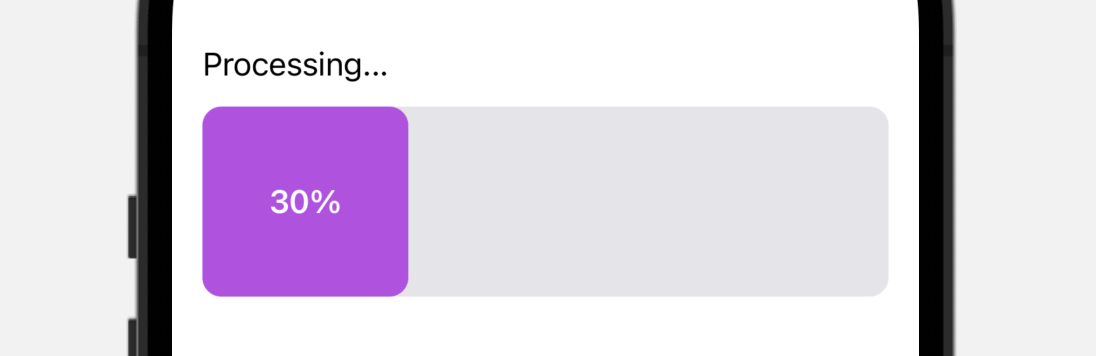
To provide you a greater thought about ProgressViewStyle
, let’s construct a customized progress bar just like the one proven above. What it’s essential do is to create a brand new struct that conforms to the ProgressViewStyle
protocol and implement the next required technique:
@ViewBuilder func makeBody(configuration: Self.Configuration) –> Self.Physique |
When you’ve got expertise with ButtonStyle
, the implementation of ProgressViewStyle
may be very comparable because it follows the identical sample.
Let’s create a brand new model named BarProgressStyle
like this:
var coloration: Shade = .purple
var peak: Double = 20.0
var labelFontStyle: Font = .physique
func makeBody(configuration: Configuration) -> some View {
let progress = configuration.fractionCompleted ?? 0.0
GeometryReader { geometry in
VStack(alignment: .main) {
configuration.label
.font(labelFontStyle)
RoundedRectangle(cornerRadius: 10.0)
.fill(Shade(uiColor: .systemGray5))
.body(peak: peak)
.body(width: geometry.measurement.width)
.overlay(alignment: .main) {
RoundedRectangle(cornerRadius: 10.0)
.fill(coloration)
.body(width: geometry.measurement.width * progress)
.overlay {
if let currentValueLabel = configuration.currentValueLabel {
currentValueLabel
.font(.headline)
.foregroundColor(.white)
}
}
}
}
}
}
}
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
struct BarProgressStyle: ProgressViewStyle {      var coloration: Shade = .purple     var peak: Double = 20.0     var labelFontStyle: Font = .physique      func makeBody(configuration: Configuration) –> some View {          let progress = configuration.fractionCompleted ?? 0.0          GeometryReader { geometry in              VStack(alignment: .main) {                  configuration.label                     .font(labelFontStyle)                  RoundedRectangle(cornerRadius: 10.0)                     .fill(Shade(uiColor: .systemGray5))                     .body(peak: peak)                     .body(width: geometry.measurement.width)                     .overlay(alignment: .main) {                         RoundedRectangle(cornerRadius: 10.0)                             .fill(coloration)                             .body(width: geometry.measurement.width * progress)                             .overlay {                                 if let currentValueLabel = configuration.currentValueLabel {                                      currentValueLabel                                         .font(.headline)                                         .foregroundColor(.white)                                 }                             }                     }              }          }     } } |
This tradition model takes in three parameters for configuring the bar coloration, peak, and font model. I’m not going to elucidate the code within the makeBody
technique line by line. In short, we implement our personal progress bar model by overlaying a purple RoundedRectangle
view on high of one other RoundedRectangle
view in gentle grey.
The configuration
parameter gives you with the frequent properties of a progress view together with:
fractionCompleted
– The finished fraction of the duty represented by the progress view, from0.0
(not but began) to1.0
(totally full).label
– The standing label.currentValueLabel
– the label that reveals the present progress.
Now that we’ve created our personal model, you’ll be able to apply it by attaching the progressViewStyle
modifier to the progress view:
ProgressView(worth: 0.3, label: { Textual content(“Processing…”) }, currentValueLabel: { Textual content(“30%”) }) Â Â Â Â .progressViewStyle(BarProgressStyle(peak: 100.0)) |
Train
After gaining an understanding of ProgressViewStyle
, it’s time to place that information into follow and create a brand new customized model. You may problem your self by trying to construct a mode that resembles the one proven within the picture under. This is not going to solely enable you reinforce what you may have discovered but additionally push you to assume creatively about the best way to obtain your required feel and look. Don’t be afraid to experiment and have enjoyable with it!
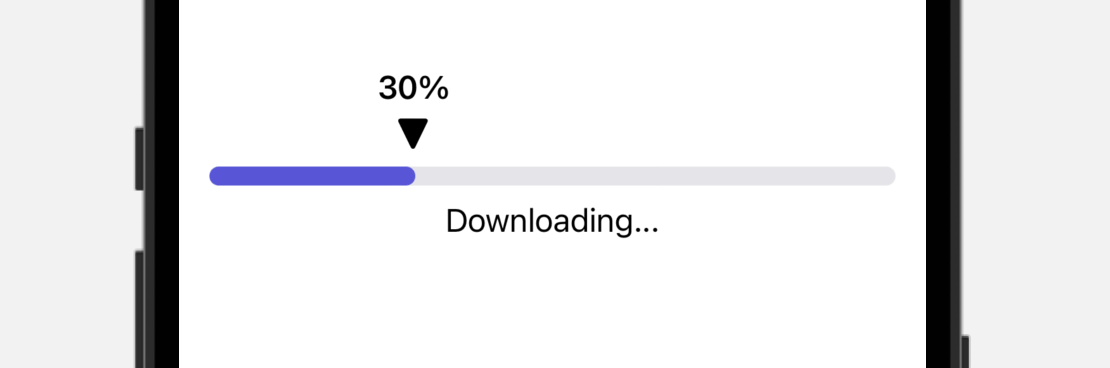
Abstract
All through this tutorial, now we have lined the basics of utilizing ProgressView
to show the progress of time-consuming duties or operations in your app. It is suggested to make use of a determinate progress indicator each time doable, because it gives customers with a extra correct understanding of the progress being made.
Whereas the default progress view is sufficient for many purposes, now we have additionally explored the best way to create a personalized progress view utilizing the ProgressViewStyle
protocol. By following the steps outlined on this tutorial, you must now have a stable understanding of the best way to create your personal distinctive model of progress view that aligns with the design and theme of your app.