Introduction
Internet frameworks/libraries in Python present a great basis for builders who wish to develop web site purposes by combining totally different options offered by these libraries in Python. Because of its simplicity, many builders have used Python, even for web site software improvement for the backend. Information Science and Machine Studying have lately develop into a key a part of these web site apps. So builders continuously search for libraries that enable them to combine these into their purposes.
Standard libraries in Python embody Flask and Django. Django is used for constructing large-scale apps. On the opposite aspect, Flask is for lighter apps. However these often operate just like the backend, the place the developer has to write down the web site’s HTML, CSS, and Javascript individually. That is the place new libraries like Streamlit and Pynecone are available, permitting the developer to create a full-fledged full-stack software immediately by way of Python while not having HTML, CSS, or Javascript recordsdata. On this article, we’ll discuss one such library known as Pynecone, which is comparatively new and gaining recognition day by day.
Studying Targets
- Be taught in regards to the Pynecone library
- Understanding tips on how to set up the Pynecone package deal
- To discover ways to get began with Pynecone to develop full-stack purposes
- Perceive the challenge format of a Pynecone challenge and tips on how to run them
This text was revealed as part of the Information Science Blogathon.
Desk of Contents
What’s Pynecone? Why a brand new Internet Framework?
Pinecone is a full-stack versatile library for constructing and deploying extremely scalable web site purposes. So why Pynecone? What makes it totally different from different Frameworks? Pinecone is created with the intent of utilizing Python for all the pieces. One doesn’t must be taught a brand new language aside from Python to develop a full-stack web site. Pynecone, new to the Internet Framework, is full of helpful options to simply get began with constructing from small information science purposes to giant, extremely scalable multi-page web sites.
Additionally, Pynecone is kind of versatile just like older libraries. Pynecone is a beginner-friendly framework however can show highly effective sufficient for growing and deploying advanced use instances. Pynecone takes care of all the pieces from the entrance finish to the again finish to the deployment of the appliance. Pynecone is designed to simplify the method of constructing a complete web site from scratch to deployment of that app fully by way of python while not having to be taught and write frontend language.
Use Circumstances and Purposes of Pynecone
1. To deploy web-based machine studying purposes
Pynecone works for each entrance and backend in web-based machine studying purposes. It integrates with a database which could be useful in storing prediction labels and different related information. This database may even be used to retailer the mannequin parameters in order that the mannequin can load its parameters and make predictions.
2. Within the information science subject, constructing visible dashboards
Visualizations are essential when coping with Information Science. They inform how nicely a enterprise is operating, how nicely a provide chain is being maintained, and the way nicely the purposes carry out. With Pynecone, these visualization dashboards could be made easy utilizing the chart parts obtainable in them.
3. Constructing web site prototypes in a brief interval of time
Time is crucial when constructing prototypes and presenting them to the shopper. The sooner one builds, the sooner one will get suggestions, and the faster one could make the suitable adjustments. Pynecone is greatest for constructing light-weight apps like enterprise prototypes very quickly.
4. In creating and deploying large-scale purposes
As mentioned, Pynecone is de facto helpful in constructing light-weight information science purposes. Nevertheless it doesn’t shrink back from constructing larger ones. Pynecone could be very a lot able to constructing giant multi-page web sites, due to its simple integration of React parts with Python.
Getting Began – Putting in Pynecone
To put in Pynecone, the Python model have to be 3.7 and above, and the NodeJS model have to be 12.22.0 and above. You’ll be able to obtain the most recent NodeJS from their official web site. Simply because we’re downloading NodeJS doesn’t imply we shall be writing Javascript code; it’s only a prerequisite to putting in Pynecone. After making certain we’ve the precise surroundings, we will go forward and set up Pynecone utilizing the pip command.
$ pip set up pynecone
It will then set up the Pynecone library and a few dependencies it would rely on.
Creating Our First Pynecone Challenge
On this part, we’ll discover ways to create a brand new Pynecone Challenge. Now open the CMD to create a brand new listing; let’s title it pyne_project. Now let’s transfer into the pyne_project listing and name the command computer init.
$ mkdir pyne_project
$ cd pyne_project
$ computer init
When putting in Pynecone, with it the computer command line software shall be put in; this software is used to create and run new initiatives. The computer init command will create a brand new Pynecone challenge by creating some recordsdata and directories, which could be seen beneath (I’ve opened pyne_project in VS Code)
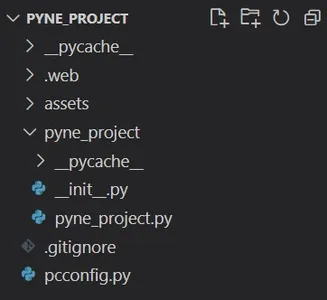
We see that computer init has created folders like pyne_project (just like the title of the folder we created), and recordsdata like pcconfig.py, pyne_project.py (right here once more, the .py file title is just like the title of the folder we’ve created), and so forth. The Property folder shops all our web sites’ static recordsdata, like photos we wish to show on the web site are saved right here. The .internet is the place the Pynecone entrance finish compiles to a NextJS app. The pcconfig.py accommodates the configurations for our software.
We don’t have to fret about these recordsdata; the one file we shall be enhancing is the pyne_project.py which is within the pyne_project folder. So mainly, we’ve created a folder pyne_project and known as computer init, which in flip created one other folder pyne_project and a file known as pyne_project.py which we shall be working upon. The pyne_project.py already has some demo code in it, so now, kind the beneath command within the CMD to run our demo web site.
$ computer run
It will run the code and can take some time whenever you first use this command computer run for a brand new challenge. Now we will entry the web site from the localhost:3000
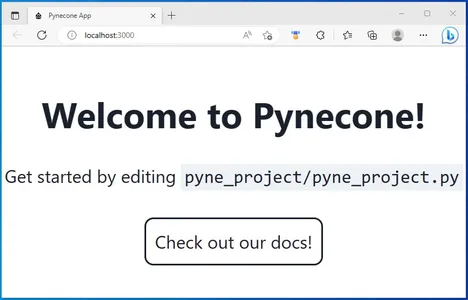
We are able to see that the webpage tells us to edit pyne_project.py situated within the pyne_project folder, which was created utilizing computer init. Press ctrl + c to cease the server.
Constructing a Easy Internet App – Multiply and Divide
On this part, we’ll create our new web site from scratch and perceive all the pieces that goes into constructing the appliance. The web site we’ll create is a straightforward software that accommodates 2 buttons known as Multiply, which multiplies a quantity by 2 after we click on on it, and Divide, which divides a quantity by 2 after we press the button.
The very first thing we shall be creating is a category known as State. This class compromises all of the variables that may change in our software, and we even outline the features that change these variables within the class itself. Let’s check out an instance code beneath:
import pynecone as computer
class State(computer.State):
starting_value = 1
def multiply(self):
self.starting_value *= 2
def divide(self):
self.starting_value /= 2
Right here we’re defining this class that inherits from the computer.State. As we create an software that may divide and multiply a quantity by 2, we have to set a beginning worth for this quantity. So within the above code, we outlined a variable starting_value after which handed a worth of 1. We even outlined the features multiply and divide, which change the worth of this variable known as starting_value, i.e., both multiply it by 2 or divide it by 2.
These features, multiply and divide, are identified by Occasion Handlers. Occasion handlers are the one doable approach to edit the state (and its variables) in a Pynecone software. These are known as in response to person actions; when a person clicks a Multiply button, this multiply operate is activated. These actions are known as occasions. So that is how we create this class, the place we outline all of the variables that shall be used within the software and the features that may change these variables.
Now we’ll write the frontend half for our software, creating buttons for multiplying and dividing and even displaying this starting_value within the browser. All of this shall be performed in Python utilizing the Pynecone library itself. For writing the frontend a part of the appliance, we’ll create a operate known as index and write the frontend half in it. The next is the code for the entrance of our software:
def index():
return computer.hstack(
computer.button(
"Multiply",
color_scheme="blue",
border_radius="1em",
on_click=State.multiply,
),
computer.textual content(State.starting_value , font_size="2em"),
computer.button(
"Divide",
color_scheme="crimson",
border_radius="1em",
on_click=State.divide,
),
)
The primary operate we’ve used is the hstack() operate from Pynecone. hstack() lets us create buttons and place them in a horizontal style. For vertical we’ll use one thing known as vstack().
To create the button, we used the button() from Pynecone. And the parameters used on this operate are self-explanatory, i.e., the primary parameter is the button title (“Multiply” and “Divide”, in our case), subsequent is the color_scheme, which defines the colour of the button, adopted by that’s the border_radius, which complies how curved the borders have to be. The final parameter is the on_click, which decides which operate to name/ what motion to take when the respective button is clicked. For the Multiply button, the on_click is about to multiply operate (multiply operate outlined within the class we created) and for the Divide button, it’s set to divide operate.
The textual content() from Pynecone is used to show the worth of the starting_value. All these features hstack(), button(), and textual content() are known as Elements that construct the frontend. Our Multiply and Divide web site is sort of full. For the web site to run, we have to outline the routing and compiling which is finished as follows:
app = computer.App(state=State)
app.add_page(index)
app.compile()
Firstly, we outline which state to make use of within the computer.App() operate. As we’ve just one, we cross this to the variable within the computer.App(). Subsequent shall be our root URL, which is our dwelling web page, and this would be the index as a result of, within the index() operate, we’ve outlined the frontend for our software, thus we cross the operate title i.e., index to the app.add_page(). Lastly, we compile our app utilizing the app.compile()
Testing – Mutiply and Divide App
On this part, we’ll run our software and guarantee it really works correctly. Lastly, we’ve written all of the code and created a frontend for our software. Now to run the web site, use the command computer run.
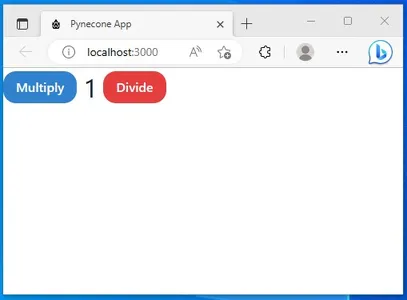
We are able to see that the web site is being run efficiently. There are two buttons known as Multiply and Divide, and between these two buttons, we see the worth of the variable starting_value, which was outlined within the class we’ve created. After clicking multiply 3 occasions, we get the next output.
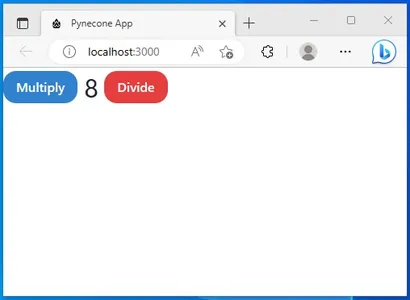
After clicking on divide 5 occasions, we get the next output
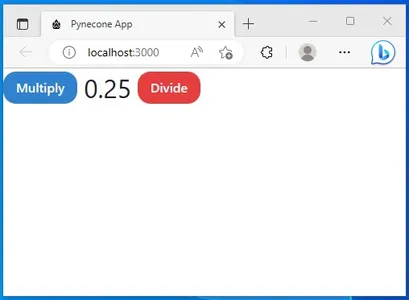
Thus our easy Multiply and Divide App is working completely advantageous. To alter the title of the appliance, we will add a parameter known as title within the app.add_page() and provides it the title of the web site
app.add_page(index, title="Multiply and Divide App")
By altering the title, we will change the app title of our web site, now after we re-load the web page, the title will get modified
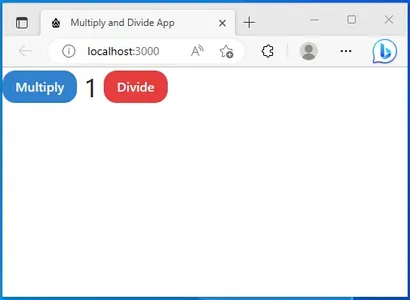
What Makes Pynecone Completely different from Others like Flask?
Pynecone is a comparatively new Internet Framework designed to construct purposes fully in Python. Flask is likely one of the well-liked libraries used to construct web sites in Python. Python with Flask is especially for the backend of an software, the place the developer has to write down the frontend code. What makes Pynecone totally different is its capacity to even write the frontend a part of the web site in Python itself, thus lowering the overhead of studying HTML, CSS, and Javascript.
One other factor that differentiates Pynecone from different libraries like Flask and Django is that Pynecone compiles your complete code right into a NextJS/ReactJS app, which shall be actually useful as a result of, with that, the react libraries could be mixed in Python in hours in comparison with different libraries which take for much longer time. The Pynecone comes with greater than 50+ Elements, thus offering sufficient of them to start out constructing information science purposes simply.
Conclusion
On this article, we’ve gone by way of a brand new Internet Framework known as Pynecone, which may fully construct web sites from the entrance finish to the again finish by way of Python. Sooner or later, Pynecone will even deploy these website-based purposes. We now have seen a walkthrough of tips on how to set up Pynecone and get began with it. Additionally, we appeared into the challenge format and totally different Elements that go into the Pynecone software.
A number of the key takeaways from this embody:
- Pynecone is a Full-Stack Internet Framework for Python.
- It gives a simple-to-use API, making constructing the frontend a part of the purposes simple.
- With Pynecone, it’s simple so as to add totally different ReactJS libraries in Python.
- Pynecone will even function the deployment of purposes within the close to future with a greater internet framework.
The media proven on this article just isn’t owned by Analytics Vidhya and is used on the Writer’s discretion.