Whereas I’ve put React utility, there is not such a factor as React utility. I imply, there are
front-end functions written in JavaScript or TypeScript that occur to
use React as their views. Nevertheless, I believe it is not honest to name them React
functions, simply as we would not name a Java EE utility JSP
utility.
As a rule, individuals squeeze various things into React
parts or hooks to make the appliance work. Such a
less-organised construction is not an issue if the appliance is small or
largely with out a lot enterprise logic. Nevertheless, as extra enterprise logic shifted
to front-end in lots of circumstances, this everything-in-component reveals issues. To
be extra particular, the hassle of understanding such sort of code is
comparatively excessive, in addition to the elevated danger to code modification.
On this article, I wish to talk about a number of patterns and methods
you need to use to reshape your “React utility” into an everyday one, and solely
with React as its view (you possibly can even swap these views into one other view
library with out an excessive amount of efforts).
The important level right here is you need to analyse what function every a part of the
code is enjoying inside an utility (even on the floor, they may be
packed in the identical file). Separate view from no-view logic, cut up the
no-view logic additional by their duties and place them within the
proper locations.
The advantage of this separation is that it lets you make modifications in
the underlying area logic with out worrying an excessive amount of concerning the floor
views, or vice versa. Additionally, it may possibly improve the reusability of the area
logic in different places as they don’t seem to be coupled to some other elements.
React is a humble library for constructing views
It is simple to neglect that React, at its core, is a library (not a
framework) that helps you construct the person interface.
On this context, it’s emphasised that React is a JavaScript library
that concentrates on a specific facet of net growth, specifically UI
parts, and affords ample freedom by way of the design of the
utility and its total construction.
A JavaScript library for constructing person interfaces
It could sound fairly simple. However I’ve seen many circumstances the place
individuals write the info fetching, reshaping logic proper within the place the place
it is consumed. For instance, fetching knowledge inside a React element, within the
useEffect
block proper above the rendering, or performing knowledge
mapping/remodeling as soon as they received the response from the server facet.
useEffect(() => { fetch("https://deal with.service/api") .then((res) => res.json()) .then((knowledge) => { const addresses = knowledge.map((merchandise) => ({ road: merchandise.streetName, deal with: merchandise.streetAddress, postcode: merchandise.postCode, })); setAddresses(addresses); }); }, []); // the precise rendering...
Maybe as a result of there may be but to be a common customary within the frontend
world, or it is only a unhealthy programming behavior. Frontend functions ought to
not be handled too in another way from common software program functions. Within the
frontend world, you continue to use separation of issues typically to rearrange
the code construction. And all of the confirmed helpful design patterns nonetheless
apply.
Welcome to the true world React utility
Most builders had been impressed by React’s simplicity and the concept
a person interface will be expressed as a pure perform to map knowledge into the
DOM. And to a sure extent, it IS.
However builders begin to battle when they should ship a community
request to a backend or carry out web page navigation, as these unwanted effects
make the element much less “pure”. And when you take into account these completely different
states (both international state or native state), issues shortly get
difficult, and the darkish facet of the person interface emerges.
Other than the person interface
React itself doesn’t care a lot about the place to place calculation or
enterprise logic, which is honest because it’s solely a library for constructing person
interfaces. And past that view layer, a frontend utility has different
elements as properly. To make the appliance work, you have to a router,
native storage, cache at completely different ranges, community requests, Third-party
integrations, Third-party login, safety, logging, efficiency tuning,
and so on.
With all this additional context, attempting to squeeze every part into
React parts or hooks is mostly not a good suggestion. The reason being
mixing ideas in a single place typically results in extra confusion. At
first, the element units up some community request for order standing, and
then there may be some logic to trim off main area from a string and
then navigate some other place. The reader should continuously reset their
logic circulation and bounce backwards and forwards from completely different ranges of particulars.
Packing all of the code into parts may match in small functions
like a Todo or one-form utility. Nonetheless, the efforts to know
such utility shall be important as soon as it reaches a sure degree.
To not point out including new options or fixing current defects.
If we may separate completely different issues into recordsdata or folders with
buildings, the psychological load required to know the appliance would
be considerably diminished. And also you solely should concentrate on one factor at a
time. Fortunately, there are already some well-proven patterns again to the
pre-web time. These design rules and patterns are explored and
mentioned properly to resolve the frequent person interface issues – however within the
desktop GUI utility context.
Martin Fowler has an incredible abstract of the idea of view-model-data
layering.
On the entire I’ve discovered this to be an efficient type of
modularization for a lot of functions and one which I recurrently use and
encourage. It is greatest benefit is that it permits me to extend my
focus by permitting me to consider the three matters (i.e., view,
mannequin, knowledge) comparatively independently.
Layered architectures have been used to manage the challenges in giant
GUI functions, and definitely we will use these established patterns of
front-end group in our “React functions”.
The evolution of a React utility
For small or one-off tasks, you may discover that every one logic is simply
written inside React parts. You may even see one or only some parts
in whole. The code seems just about like HTML, with just some variable or
state used to make the web page “dynamic”. Some may ship requests to fetch
knowledge on useEffect
after the parts render.
As the appliance grows, and increasingly more code are added to codebase.
With out a correct approach to organise them, quickly the codebase will flip into
unmaintainable state, that means that even including small options will be
time-consuming as builders want extra time to learn the code.
So I’ll record a number of steps that may assist to reduction the maintainable
downside. It typically require a bit extra efforts, however it’ll repay to
have the construction in you utility. Let’s have a fast overview of those
steps to construct front-end functions that scale.
Single Element Utility
It may be known as just about a Single Element Utility:
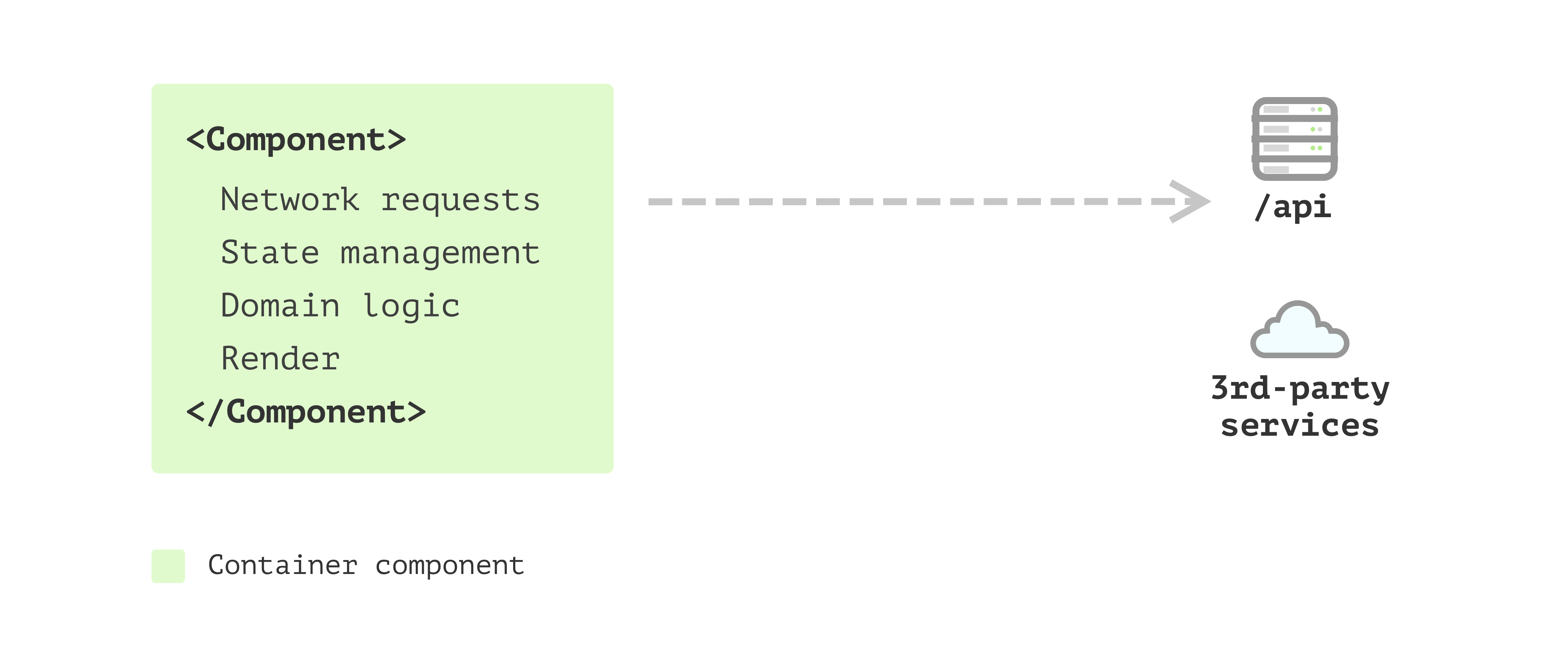
Determine 1: Single Element Utility
However quickly, you realise one single element requires a whole lot of time
simply to learn what’s going on. For instance, there may be logic to iterate
by means of a listing and generate every merchandise. Additionally, there may be some logic for
utilizing Third-party parts with only some configuration code, aside
from different logic.
A number of Element Utility
You determined to separate the element into a number of parts, with
these buildings reflecting what’s taking place on the outcome HTML is a
good thought, and it lets you concentrate on one element at a time.
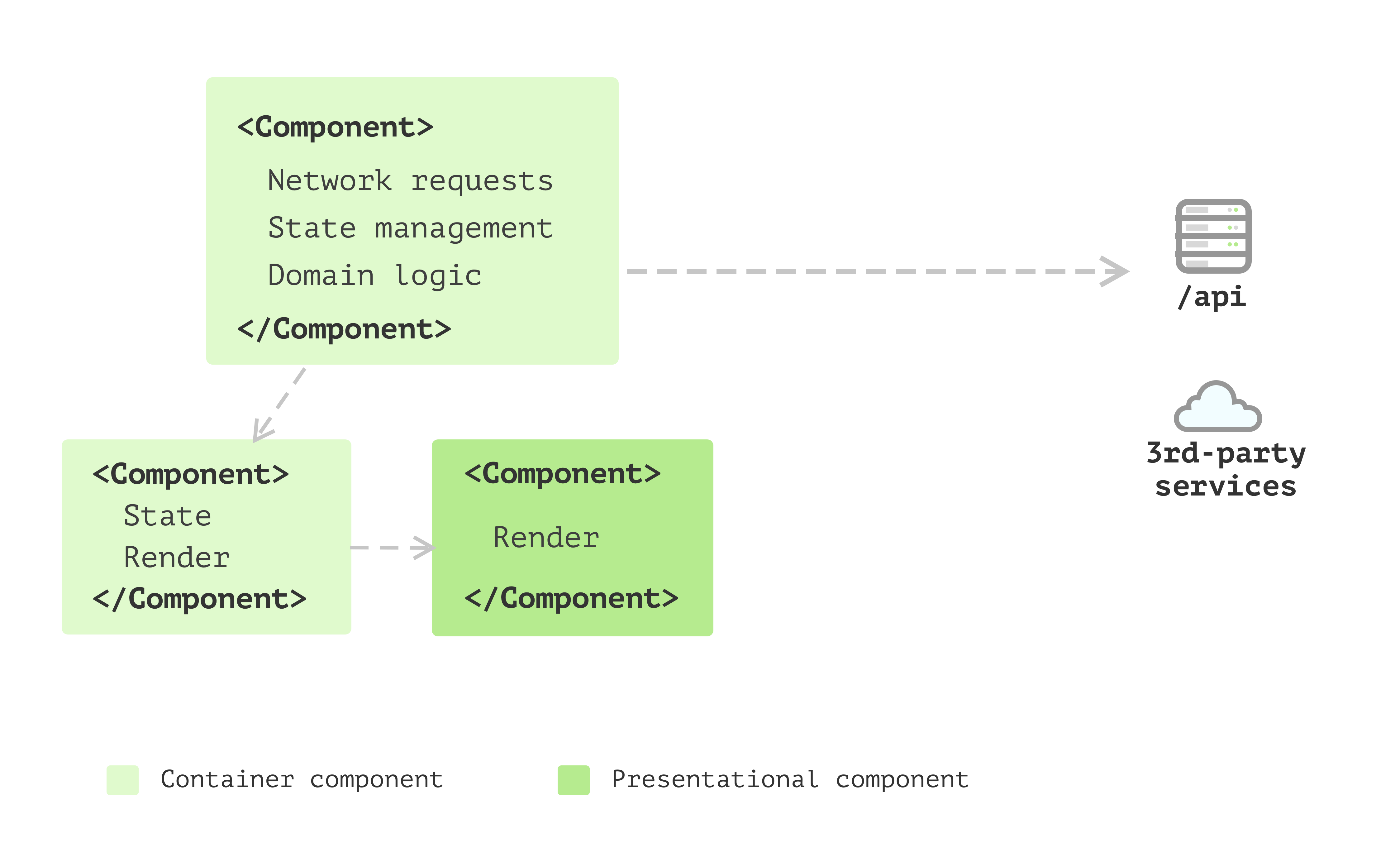
Determine 2: A number of Element Utility
And as your utility grows, other than the view, there are issues
like sending community requests, changing knowledge into completely different shapes for
the view to eat, and gathering knowledge to ship again to the server. And
having this code inside parts doesn’t really feel proper as they’re not
actually about person interfaces. Additionally, some parts have too many
inside states.
State administration with hooks
It’s a greater thought to separate this logic right into a separate locations.
Fortunately in React, you possibly can outline your personal hooks. This can be a nice approach to
share these state and the logic of at any time when states change.
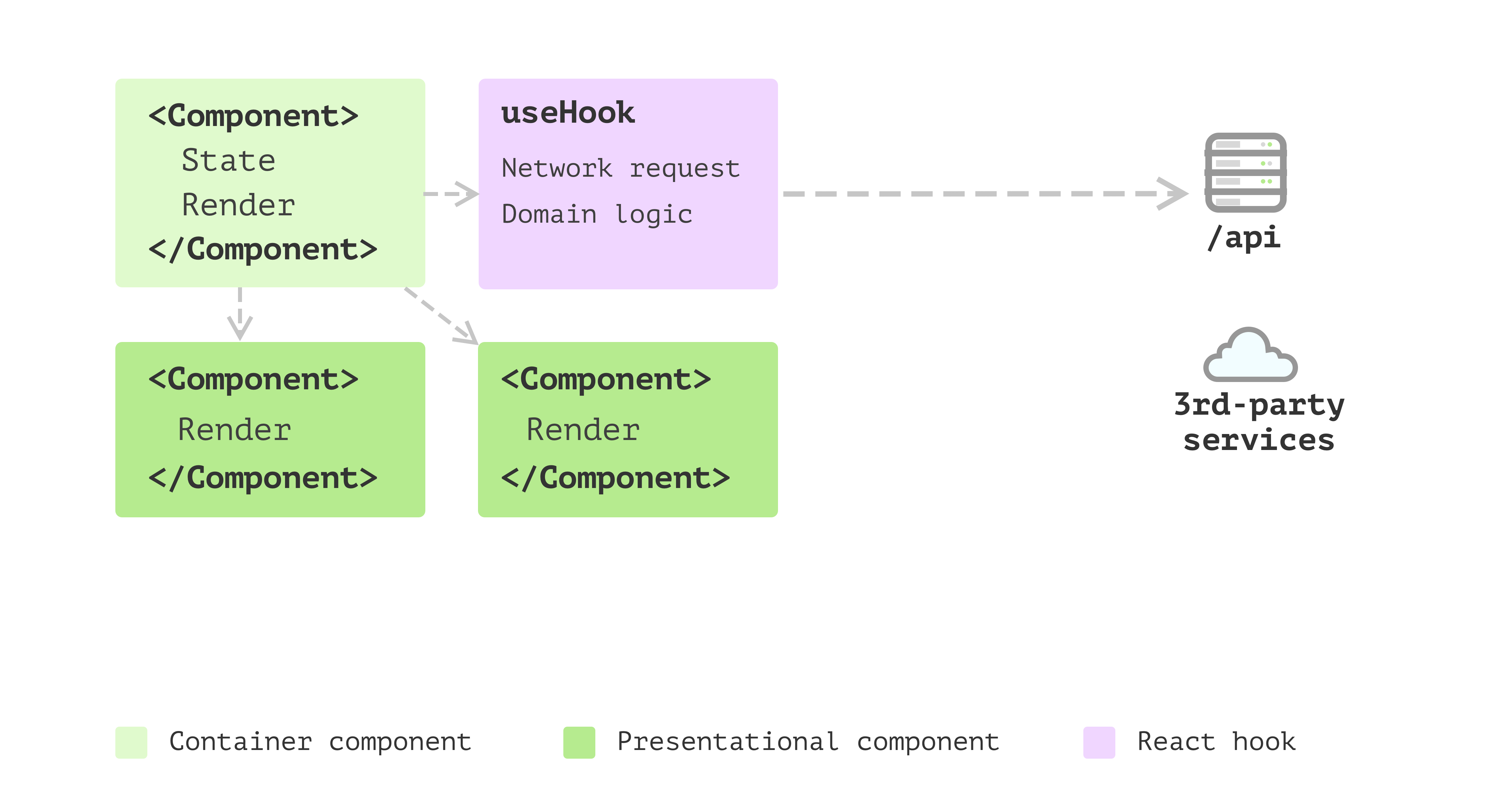
Determine 3: State administration with hooks
That’s superior! You could have a bunch of parts extracted out of your
single element utility, and you’ve got a number of pure presentational
parts and a few reusable hooks that make different parts stateful.
The one downside is that in hooks, other than the facet impact and state
administration, some logic doesn’t appear to belong to the state administration
however pure calculations.
Enterprise fashions emerged
So that you’ve began to turn out to be conscious that extracting this logic into but
one other place can deliver you a lot advantages. For instance, with that cut up,
the logic will be cohesive and impartial of any views. You then extract
a number of area objects.
These easy objects can deal with knowledge mapping (from one format to
one other), examine nulls and use fallback values as required. Additionally, because the
quantity of those area objects grows, you discover you want some inheritance
or polymorphism to make issues even cleaner. Thus you utilized many
design patterns you discovered useful from different locations into the front-end
utility right here.
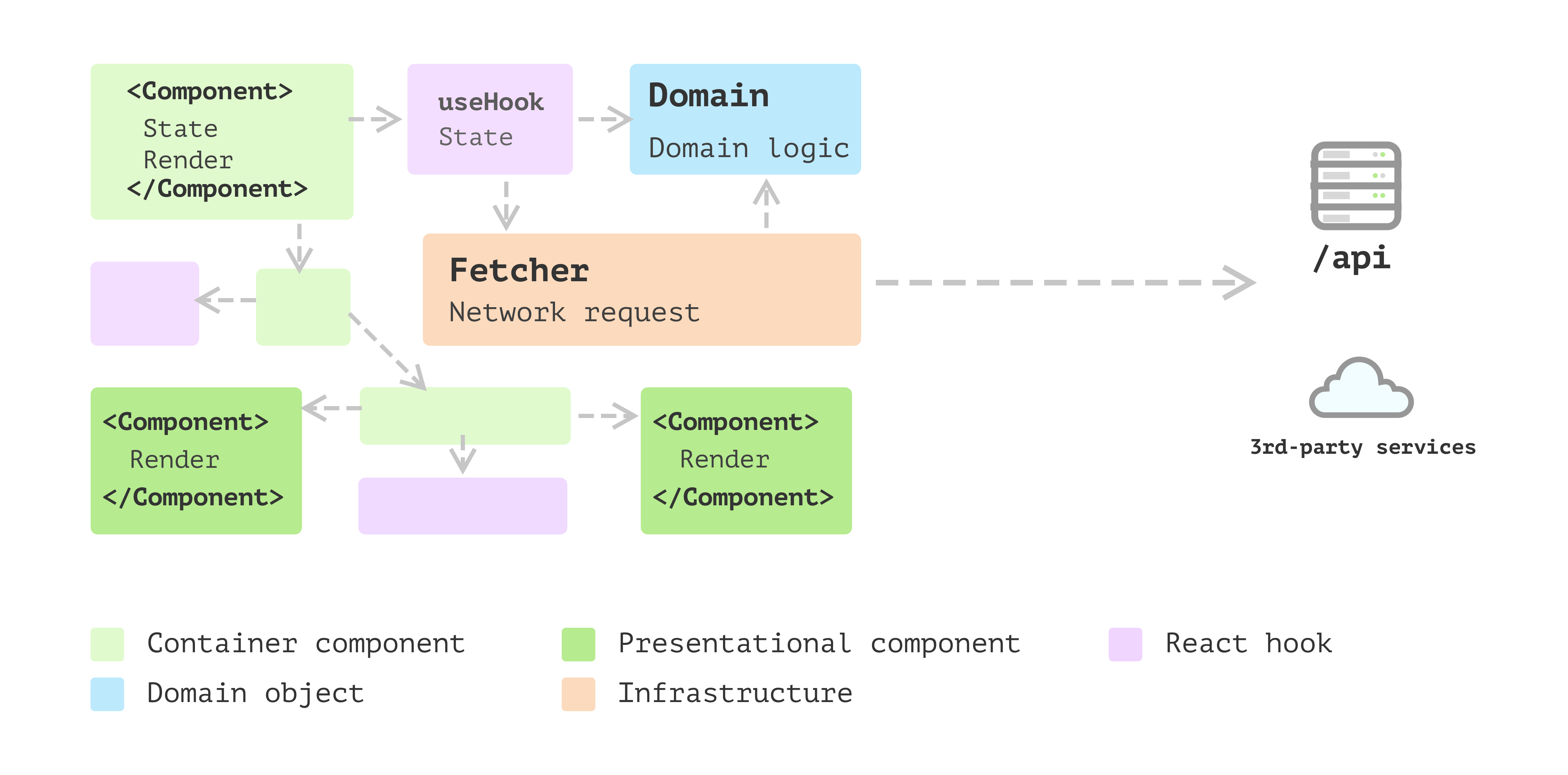
Determine 4: Enterprise fashions
Layered frontend utility
The appliance retains evolving, and then you definitely discover some patterns
emerge. There are a bunch of objects that don’t belong to any person
interface, they usually additionally don’t care about whether or not the underlying knowledge is
from distant service, native storage or cache. After which, you wish to cut up
them into completely different layers. Here’s a detailed clarification concerning the layer
splitting Presentation Area Information Layering.
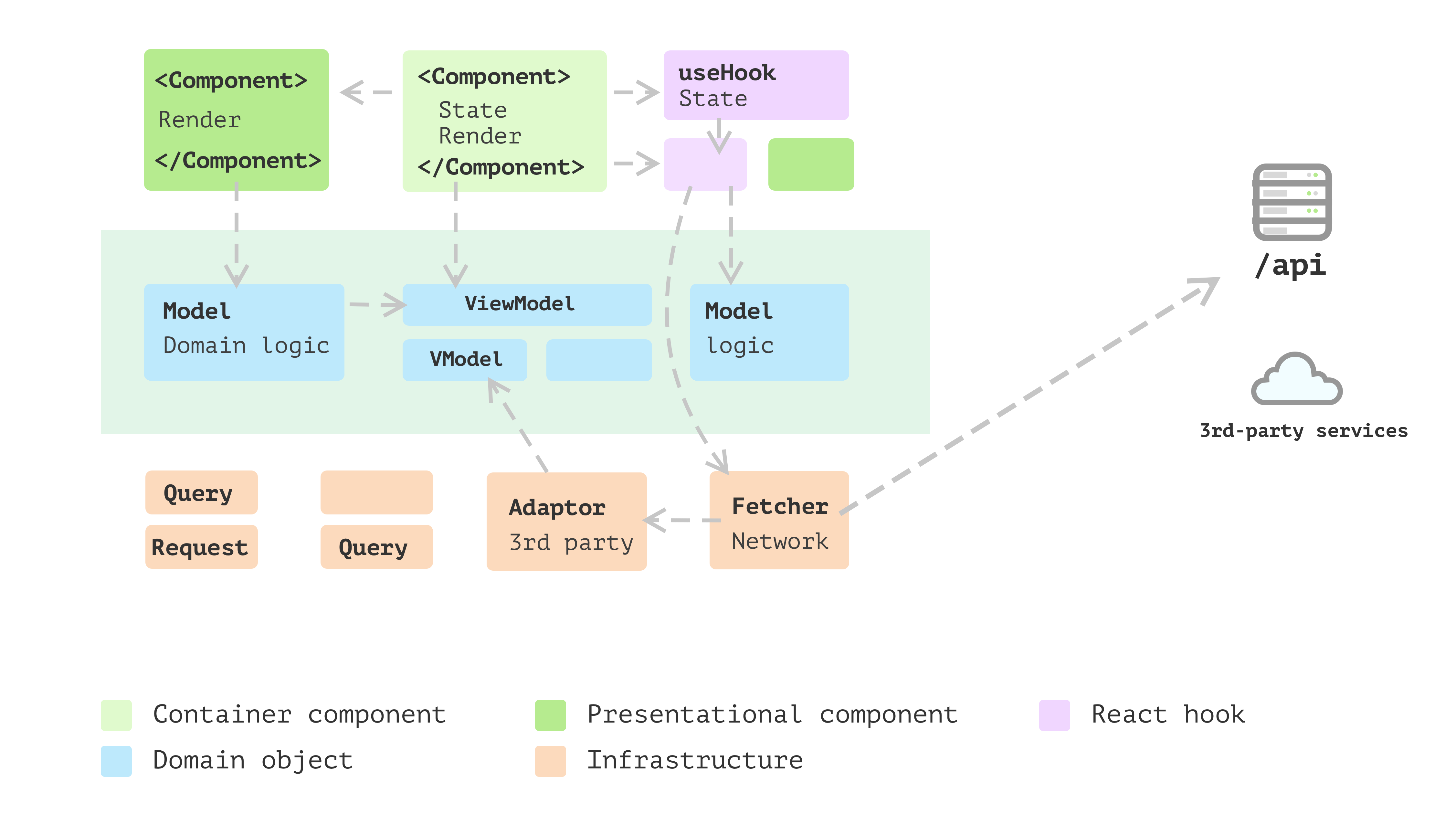
Determine 5: Layered frontend utility
The above evolution course of is a high-level overview, and you need to
have a style of how you need to construction your code or not less than what the
path needs to be. Nevertheless, there shall be many particulars it’s essential
take into account earlier than making use of the speculation in your utility.
Within the following sections, I’ll stroll you thru a characteristic I
extracted from an actual challenge to reveal all of the patterns and design
rules I believe helpful for large frontend functions.
Introduction of the Cost characteristic
I’m utilizing an oversimplified on-line ordering utility as a beginning
level. On this utility, a buyer can decide up some merchandise and add
them to the order, after which they might want to choose one of many fee
strategies to proceed.
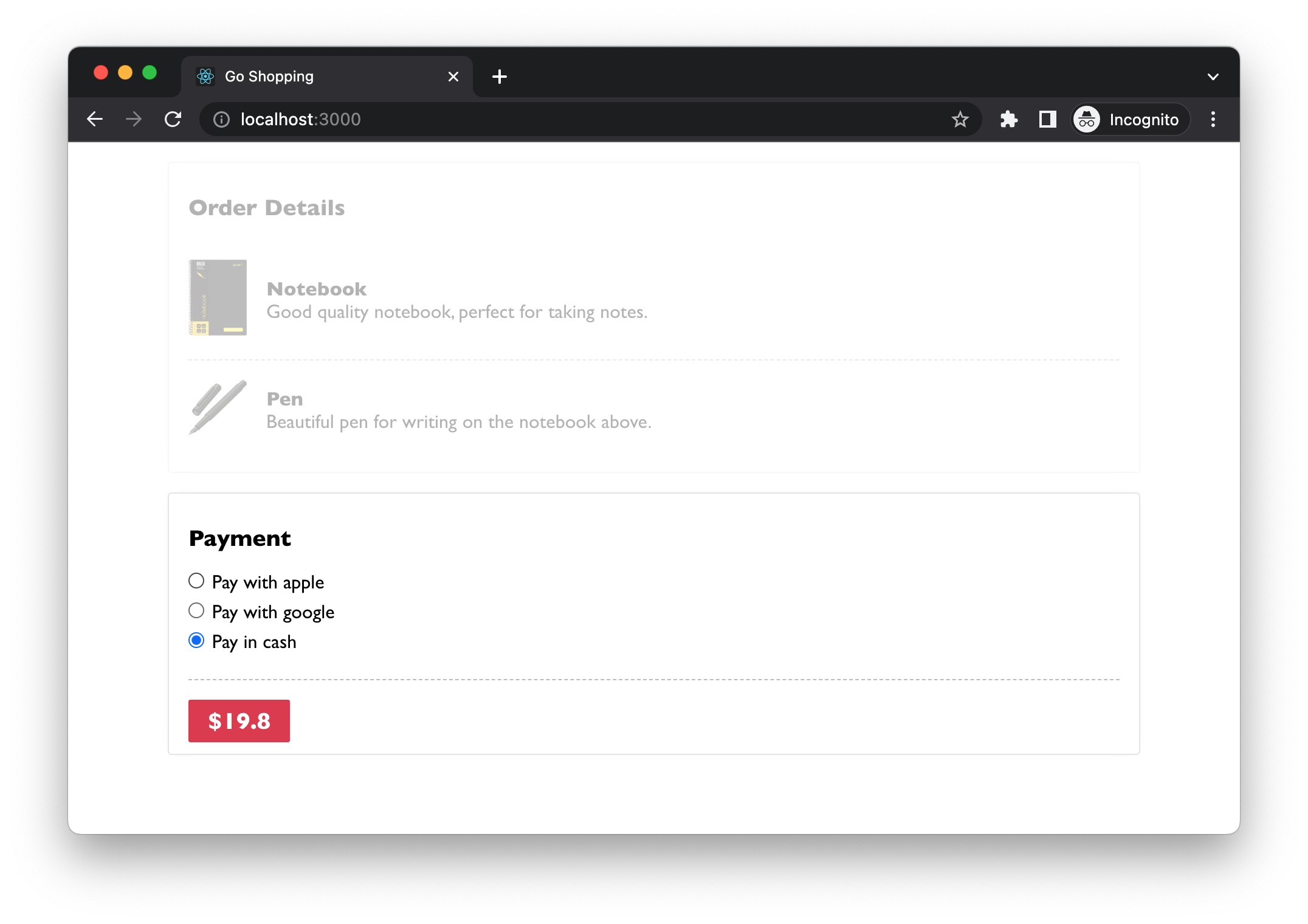
Determine 6: Cost part
These fee technique choices are configured on the server facet, and
prospects from completely different nations may even see different choices. For instance,
Apple Pay could solely be widespread in some nations. The radio buttons are
data-driven – no matter is fetched from the backend service shall be
surfaced. The one exception is that when no configured fee strategies
are returned, we don’t present something and deal with it as “pay in money” by
default.
For simplicity, I’ll skip the precise fee course of and concentrate on the
Cost
element. Let’s say that after studying the React howdy world
doc and a few stackoverflow searches, you got here up with some code
like this:
src/Cost.tsx…
export const Cost = ({ quantity }: { quantity: quantity }) => { const [paymentMethods, setPaymentMethods] = useState<LocalPaymentMethod[]>( [] ); useEffect(() => { const fetchPaymentMethods = async () => { const url = "https://online-ordering.com/api/payment-methods"; const response = await fetch(url); const strategies: RemotePaymentMethod[] = await response.json(); if (strategies.size > 0) { const prolonged: LocalPaymentMethod[] = strategies.map((technique) => ({ supplier: technique.identify, label: `Pay with ${technique.identify}`, })); prolonged.push({ supplier: "money", label: "Pay in money" }); setPaymentMethods(prolonged); } else { setPaymentMethods([]); } }; fetchPaymentMethods(); }, []); return ( <div> <h3>Cost</h3> <div> {paymentMethods.map((technique) => ( <label key={technique.supplier}> <enter sort="radio" identify="fee" worth={technique.supplier} defaultChecked={technique.supplier === "money"} /> <span>{technique.label}</span> </label> ))} </div> <button>${quantity}</button> </div> ); };
The code above is fairly typical. You might need seen it within the get
began tutorial someplace. And it is not mandatory unhealthy. Nevertheless, as we
talked about above, the code has combined completely different issues all in a single
element and makes it a bit tough to learn.
The issue with the preliminary implementation
The primary situation I wish to deal with is how busy the element
is. By that, I imply Cost
offers with various things and makes the
code tough to learn as it’s a must to change context in your head as you
learn.
With a view to make any modifications it’s a must to comprehend
the way to initialise community request
,
the way to map the info to an area format that the element can perceive
,
the way to render every fee technique
,
and
the rendering logic for Cost
element itself
.
src/Cost.tsx…
export const Cost = ({ quantity }: { quantity: quantity }) => { const [paymentMethods, setPaymentMethods] = useState<LocalPaymentMethod[]>( [] ); useEffect(() => { const fetchPaymentMethods = async () => { const url = "https://online-ordering.com/api/payment-methods"; const response = await fetch(url); const strategies: RemotePaymentMethod[] = await response.json(); if (strategies.size > 0) { const prolonged: LocalPaymentMethod[] = strategies.map((technique) => ({ supplier: technique.identify, label: `Pay with ${technique.identify}`, })); prolonged.push({ supplier: "money", label: "Pay in money" }); setPaymentMethods(prolonged); } else { setPaymentMethods([]); } }; fetchPaymentMethods(); }, []); return ( <div> <h3>Cost</h3> <div> {paymentMethods.map((technique) => ( <label key={technique.supplier}> <enter sort="radio" identify="fee" worth={technique.supplier} defaultChecked={technique.supplier === "money"} /> <span>{technique.label}</span> </label> ))} </div> <button>${quantity}</button> </div> ); };
It isn’t an enormous downside at this stage for this straightforward instance.
Nevertheless, because the code will get greater and extra complicated, we’ll have to
refactoring them a bit.
It’s good observe to separate view and non-view code into separate
locations. The reason being, typically, views are altering extra regularly than
non-view logic. Additionally, as they cope with completely different facets of the
utility, separating them lets you concentrate on a specific
self-contained module that’s way more manageable when implementing new
options.
The cut up of view and non-view code
In React, we will use a customized hook to keep up state of a element
whereas retaining the element itself kind of stateless. We will
use
to create a perform known as usePaymentMethods
(the
prefix use
is a conference in React to point the perform is a hook
and dealing with some states in it):
src/Cost.tsx…
const usePaymentMethods = () => {
const [paymentMethods, setPaymentMethods] = useState<LocalPaymentMethod[]>(
[]
);
useEffect(() => {
const fetchPaymentMethods = async () => {
const url = "https://online-ordering.com/api/payment-methods";
const response = await fetch(url);
const strategies: RemotePaymentMethod[] = await response.json();
if (strategies.size > 0) {
const prolonged: LocalPaymentMethod[] = strategies.map((technique) => ({
supplier: technique.identify,
label: `Pay with ${technique.identify}`,
}));
prolonged.push({ supplier: "money", label: "Pay in money" });
setPaymentMethods(prolonged);
} else {
setPaymentMethods([]);
}
};
fetchPaymentMethods();
}, []);
return {
paymentMethods,
};
};
This returns a paymentMethods
array (in sort LocalPaymentMethod
) as
inside state and is prepared for use in rendering. So the logic in
Cost
will be simplified as:
src/Cost.tsx…
export const Cost = ({ quantity }: { quantity: quantity }) => {
const { paymentMethods } = usePaymentMethods();
return (
<div>
<h3>Cost</h3>
<div>
{paymentMethods.map((technique) => (
<label key={technique.supplier}>
<enter
sort="radio"
identify="fee"
worth={technique.supplier}
defaultChecked={technique.supplier === "money"}
/>
<span>{technique.label}</span>
</label>
))}
</div>
<button>${quantity}</button>
</div>
);
};
This helps relieve the ache within the Cost
element. Nevertheless, in case you
have a look at the block for iterating by means of paymentMethods
, it appears a
idea is lacking right here. In different phrases, this block deserves its personal
element. Ideally, we would like every element to concentrate on, just one
factor.
Information modelling to encapsulate logic
Up to now, the modifications we now have made are all about splitting view and
non-view code into completely different locations. It really works properly. The hook handles knowledge
fetching and reshaping. Each Cost
and PaymentMethods
are comparatively
small and straightforward to know.
Nevertheless, in case you look intently, there may be nonetheless room for enchancment. To
begin with, within the pure perform element PaymentMethods
, we now have a bit
of logic to examine if a fee technique needs to be checked by default:
src/Cost.tsx…
const PaymentMethods = ({
paymentMethods,
}: {
paymentMethods: LocalPaymentMethod[];
}) => (
<>
{paymentMethods.map((technique) => (
<label key={technique.supplier}>
<enter
sort="radio"
identify="fee"
worth={technique.supplier}
defaultChecked={technique.supplier === "money"}
/>
<span>{technique.label}</span>
</label>
))}
</>
);
These check statements in a view will be thought-about a logic leak, and
progressively they are often scatted elsewhere and make modification
more durable.
One other level of potential logic leakage is within the knowledge conversion
the place we fetch knowledge:
src/Cost.tsx…
const usePaymentMethods = () => { const [paymentMethods, setPaymentMethods] = useState<LocalPaymentMethod[]>( [] ); useEffect(() => { const fetchPaymentMethods = async () => { const url = "https://online-ordering.com/api/payment-methods"; const response = await fetch(url); const strategies: RemotePaymentMethod[] = await response.json(); if (strategies.size > 0) { const prolonged: LocalPaymentMethod[] = strategies.map((technique) => ({ supplier: technique.identify, label: `Pay with ${technique.identify}`, })); prolonged.push({ supplier: "money", label: "Pay in money" }); setPaymentMethods(prolonged); } else { setPaymentMethods([]); } }; fetchPaymentMethods(); }, []); return { paymentMethods, }; };
Be aware the nameless perform inside strategies.map
does the conversion
silently, and this logic, together with the technique.supplier === "money"
above will be extracted into a category.
We may have a category PaymentMethod
with the info and behavior
centralised right into a single place:
src/PaymentMethod.ts…
class PaymentMethod {
non-public remotePaymentMethod: RemotePaymentMethod;
constructor(remotePaymentMethod: RemotePaymentMethod) {
this.remotePaymentMethod = remotePaymentMethod;
}
get supplier() {
return this.remotePaymentMethod.identify;
}
get label() {
if(this.supplier === 'money') {
return `Pay in ${this.supplier}`
}
return `Pay with ${this.supplier}`;
}
get isDefaultMethod() {
return this.supplier === "money";
}
}
With the category, I can outline the default money fee technique:
const payInCash = new PaymentMethod({ identify: "money" });
And throughout the conversion – after the fee strategies are fetched from
the distant service – I can assemble the PaymentMethod
object in-place. And even
extract a small perform known as convertPaymentMethods
:
src/usePaymentMethods.ts…
const convertPaymentMethods = (strategies: RemotePaymentMethod[]) => {
if (strategies.size === 0) {
return [];
}
const prolonged: PaymentMethod[] = strategies.map(
(technique) => new PaymentMethod(technique)
);
prolonged.push(payInCash);
return prolonged;
};
Additionally, within the PaymentMethods
element, we don’t use the
technique.supplier === "money"
to examine anymore, and as a substitute name the
getter
:
src/PaymentMethods.tsx…
export const PaymentMethods = ({ choices }: { choices: PaymentMethod[] }) => (
<>
{choices.map((technique) => (
<label key={technique.supplier}>
<enter
sort="radio"
identify="fee"
worth={technique.supplier}
defaultChecked={technique.isDefaultMethod}
/>
<span>{technique.label}</span>
</label>
))}
</>
);
Now we’re restructuring our Cost
element right into a bunch of smaller
elements that work collectively to complete the work.
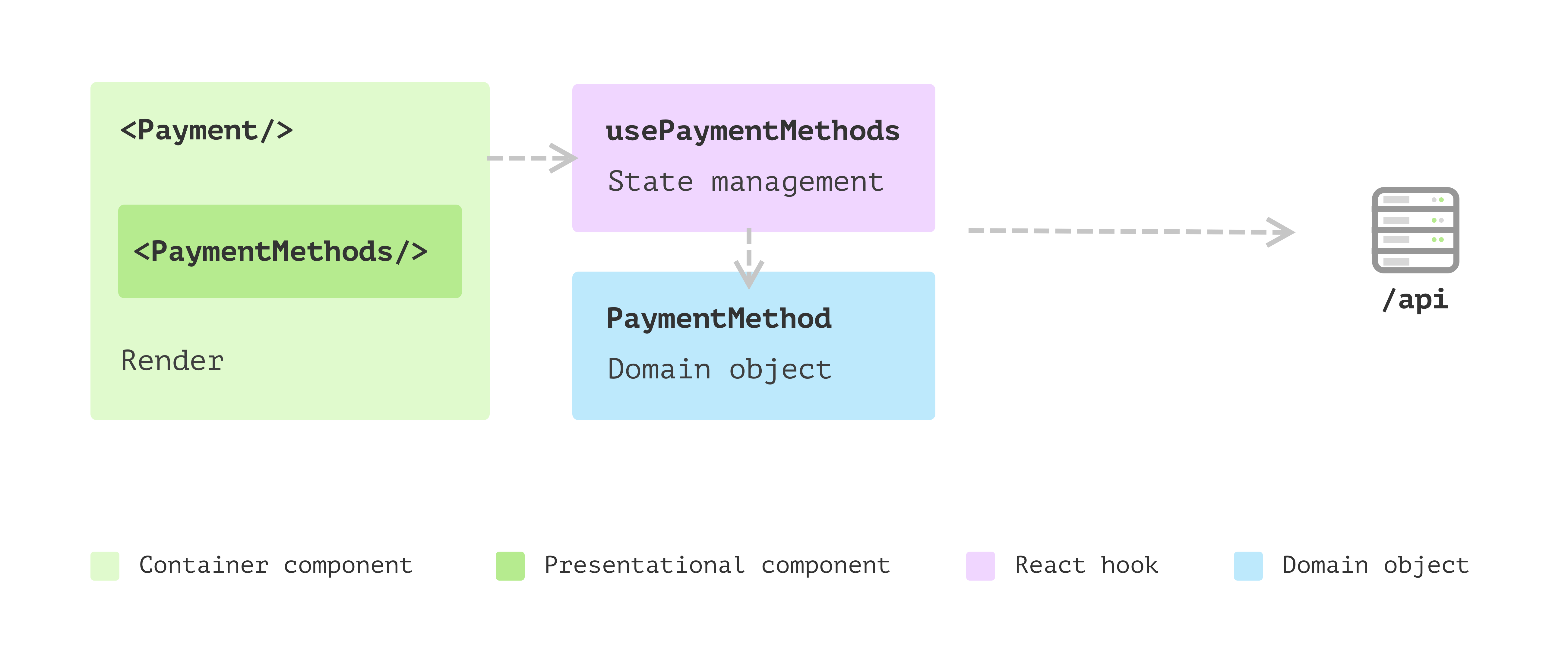
Determine 7: Refactored Cost with extra elements that may be composed simply
The advantages of the brand new construction
- Having a category encapsulates all of the logic round a fee technique. It’s a
area object and doesn’t have any UI-related info. So testing and
probably modifying logic right here is way simpler than when embedded in a
view. - The brand new extracted element
PaymentMethods
is a pure perform and solely
is dependent upon a site object array, which makes it tremendous straightforward to check and reuse
elsewhere. We would have to cross in aonSelect
callback to it, however even in
that case, it’s a pure perform and doesn’t have to the touch any exterior
states. - Every a part of the characteristic is obvious. If a brand new requirement comes, we will
navigate to the correct place with out studying all of the code.
I’ve to make the instance on this article sufficiently complicated in order that
many patterns will be extracted. All these patterns and rules are
there to assist simplify our code’s modifications.