JavaScript is by far probably the most well-liked languages on the subject of net improvement, powering most web sites and net purposes. Not being restricted to solely the client-side JavaScript can also be probably the most well-liked languages that are used for creating server-side purposes. Organizations use Javascript to create interactive and dynamic net purposes for his or her prospects. At the moment, most fashionable net purposes depend on utilizing REST structure to enhance the web site’s dynamic capabilities.
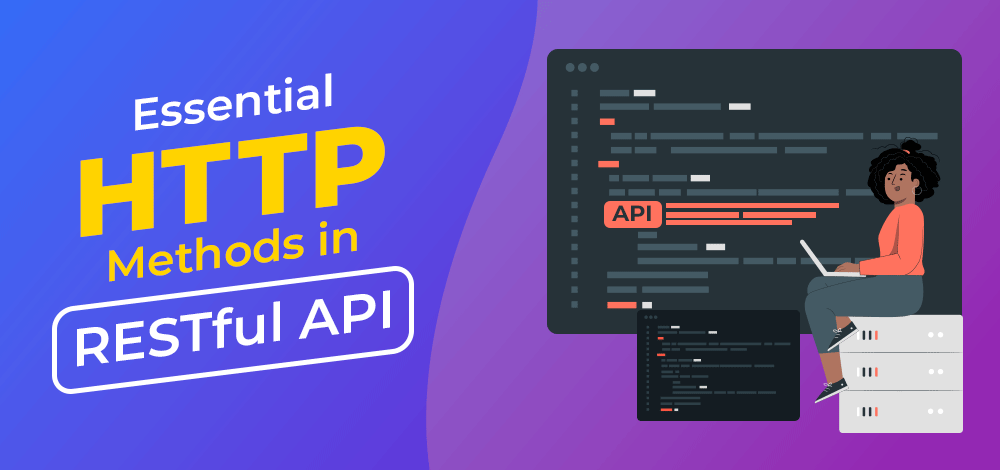
Thus, there are a few of the most important HTTP strategies that you will need to know as a developer, to develop RESTful APIs in your software. RESTful APIs are people who observe the REST (Representational State Switch) architectural model. With this being mentioned, let’s proceed with the article on the important RESTful strategies to help you to have with engaged on the server aspect utilizing JavaScript.
5 Important HTTP Strategies in RESTful API Improvement
1. GET
The GET technique is used to ‘retrieve’ a file or a set of information from the server. The beneath code exhibits the implementation of the GET technique in JavaScript.
Instance:
1.1. Backend (Node with Specific)
Javascript
|
Right here, the code defines a get() technique that’s used to retrieve the ‘college students’ (right here is an array of objects) information from the server. It defines a route that listens to the ‘/college students‘ endpoint. The second parameter is a callback operate that receives ‘req'(request) and ‘res‘ (response) objects as arguments. It makes use of the ‘res.json()’ technique to ship the information to the shopper.
1.2. Frontend (JavaScript)
Javascript
|
Right here, the code defines an async operate referred to as ‘getStudents()’ that makes a GET request to the API Endpoint (/college students) utilizing the fetch operate. The fetch operate returns a promise that’s resolved with await and the response object is saved within the ‘response’ variable. The json() technique is known as on the response to parse the information which once more returns a promise that’s resolved by await and the information is saved within the ‘information’ variable. The parsed information(record of scholars) is then logged into the console.
Should Learn: Specific | app.get()
2. POST
The POST technique sends information to create a ‘new file‘ on the server. The beneath code exhibits the implementation of the POST technique in JavaScript.
Instance:
2.1. Backend (Node with Specific)
Javascript
|
Right here, the code defines a submit() technique that’s used so as to add a brand new file i.e. ‘pupil’ information to the server. It defines a route that listens to the ‘/college students’ endpoint. The second parameter is a callback operate that receives ‘req'(request) and ‘res’ (response) objects as arguments. It extracts the information from the request utilizing ‘req.physique’, and appends it to the prevailing record utilizing the array push() technique. Lastly, it sends the acknowledgment message again to the shopper within the type of JSON information utilizing res.json().
2.2. Frontend (JavaScript)
Javascript
|
Right here, the code defines an async operate referred to as ‘addStudent()’ that makes a POST request to the API Endpoint (/college students) with the request physique containing the ‘pupil’ information. The fetch operate returns a promise which is resolved with await and the response object is saved within the ‘response’ variable. The json() technique is known as on the response to parse the information which once more returns a promise that’s resolved by await and the information is saved within the ‘information’ variable. The parsed information (acknowledgment message – Document Added) is then logged into the console.
Should Learn: Specific | app.submit()
3. PUT
The PUT technique sends information to replace an ‘present file‘ on the server. The beneath code exhibits the implementation of the PUT technique in JavaScript.
Instance:
3.1. Backend (Node with Specific)
Javascript
|
Right here, the code defines a put() technique that’s used to replace an present file i.e. ‘pupil with particular id’ on the server. It defines a route that listens to the ‘/college students/:id’ endpoint. The ‘:id’ here’s a URL parameter that’s extracted utilizing ‘req.params.id‘. The information handed contained in the request physique is extracted utilizing ‘req.physique’. The scholar’s information is traversed to seek out the coed with the matching id which on discovered will get the actual file changed with new information. Lastly, it sends the acknowledgment message again to the shopper within the type of JSON information utilizing res.json().
3.2. Frontend (JavaScript)
Javascript
|
Right here, the code defines an async operate referred to as ‘updateStudent()’ that makes a PUT request to the API Endpoint (/college students/3) with the request physique containing the ‘pupil‘ information. The fetch operate returns a promise which is resolved with await and the response object is saved within the ‘response’ variable. The json() technique is known as on the response to parse the information which once more returns a promise that’s resolved by await and the information is saved within the ‘information’ variable. The parsed information (acknowledgment message – “Document Up to date”) is then logged into the console.
Should Learn: Specific | app.put()
4. PATCH
Just like the PUT technique, PATCH can also be used to ship information to replace an ‘present file’ on the server. However the vital distinction between PUT and PATCH is that PATCH solely applies partial modifications to the file as a substitute of changing the entire file. The beneath code exhibits the implementation of the PATCH technique in JavaScript.
Instance:
4.1. Backend (Node with Specific)
Javascript
|
Right here, the code defines a patch() technique that’s used to partially replace an present file i.e. ‘pupil with particular id’ on the server. It defines a route that listens to the ‘/college students/:id’ endpoint. The ‘:id’ here’s a URL parameter that’s extracted utilizing ‘req.params.id’. The information handed contained in the request physique is extracted utilizing ‘req.physique’. The scholar’s information is traversed to seek out the coed with the matching id which on discovered will get the actual file up to date, right here as a substitute of updating the complete object solely the particular properties on the objects get up to date. Lastly, it sends the acknowledgment message again to the shopper within the type of JSON information utilizing res.json().
4.2. Frontend (JavaScript)
Javascript
|
Right here, the code defines an async operate referred to as ‘updateStudentPatch()‘ that makes a PATCH request to the API Endpoint (/college students/2) with the request physique containing the precise(‘identify’) property ‘pupil’ information. The fetch operate returns a promise which is resolved with await and the response object is saved within the ‘response’ variable. The json() technique is known as on the response to parse the information which once more returns a promise that’s resolved by await and the information is saved within the ‘information’ variable. The parsed information (acknowledgment message – ‘Document Up to date utilizing patch’) is then logged into the console.
Should Learn: Specific | put() vs patch()
5. DELETE
The DELETE technique is used to delete file(s) from the server. The beneath code exhibits the implementation of the DELETE technique in JavaScript.
Instance:
5.1. Backend (Node with Specific)
Javascript
|
Right here, the code defines a delete() technique that’s used to delete an present file (right here ‘pupil with particular id‘) on the server. It defines a route that listens to the ‘/college students/:id’ endpoint. The ‘:id’ here’s a URL parameter that’s extracted utilizing ‘req.params.id’. The scholar’s information (right here Array of scholars) is traversed to seek out the coed with the matching id which on discovered will get deleted utilizing the Array splice() technique in javascript. Lastly, it sends the acknowledgment message again to the shopper within the type of JSON information utilizing res.json().
5.2. Frontend (JavaScript)
Javascript
|
Right here, the code defines an async operate referred to as ‘deleteStudent()‘ that makes a PATCH request to the API Endpoint (/college students/3). The fetch operate returns a promise which is resolved with await and the response object is saved within the ‘response’ variable. The json() technique is known as on the response to parse the information which once more returns a promise that’s resolved by await and the information is saved within the ‘information’ variable. The parsed information (acknowledgment message – ‘Document Deleted‘) is then logged into the console.
Should Learn: Specific | app.delete()
Code Information
1. Backend Code
Javascript
|
2. Frontend Code
Javascript
|
Conclusion
Now that you understand how to implement RESTful HTTP strategies in javascript, begin utilizing them now! HTTP strategies equivalent to GET, POST, PUT, PATCH, and DELETE are utilized in RESTful API improvement to specify the kind of motion being carried out on a useful resource. RESTful HTTP strategies are an integral part of creating net APIs within the REST architectural model. They’re broadly utilized in fashionable net improvement as a result of they supply a normal interface for interacting with server sources.
FAQs
1. What’s REST?
Ans: REST (Representational State Switch) is a design sample for creating net companies. It establishes a set of constraints and ideas for creating net APIs which are versatile, scalable, and easy to keep up.
2. What’s the distinction between REST and RESTful?
Ans: REST is a set of architectural pointers for constructing APIs. RESTful APIs are APIs that adhere to REST pointers.
3. What’s the distinction between RESTful and Non-Restful APIs?
Ans: RESTful APIs observe REST pointers. Quite the opposite, Non-Restful APIs use different strategies/protocols like SOAP(Easy Object Entry Protocol) for communication.
4. What’s the distinction between Node and Specific?
Ans: Node is a runtime constructed on Chrome’s V8 javascript runtime engine. Specific is a framework for constructing net purposes on prime of Node.js