Python is a strong programming language that has been gaining reputation lately. It’s recognized for its versatility and ease of use, making it an amazing selection for inexperienced persons and skilled programmers alike. Nonetheless, with so many sources accessible on the web, it may be troublesome to know the place to begin. That’s why we’ve put collectively this complete information to mastering Python in 2023.
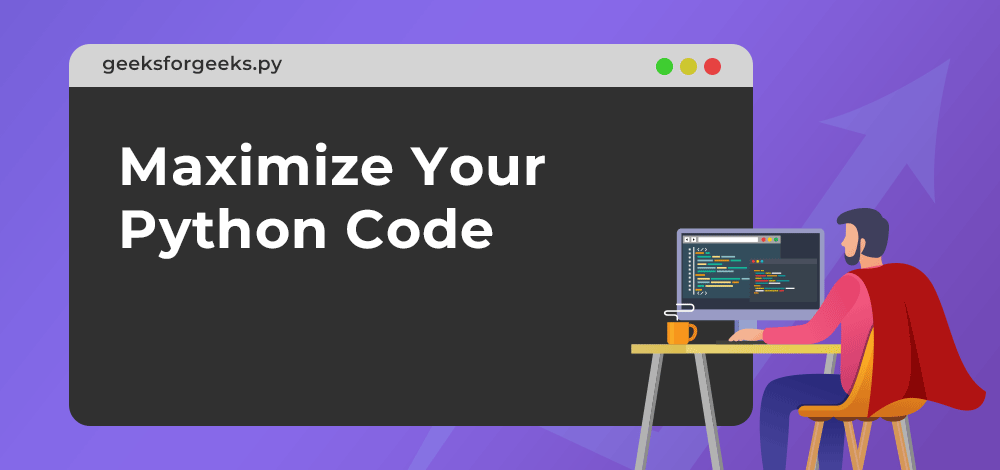
On this article, you’ll be capable to maximize your Python Efficiency with the ten suggestions in 2023, whether or not you’re simply beginning out or seeking to take your expertise to the subsequent stage. The following tips are designed that will help you navigate the complicated world of Python programming and provide the instruments you have to succeed. So, in case you’re able to take your Python expertise to the subsequent stage and change into a grasp of the language, learn on!
1. Ideas For Optimizing Code Efficiency and Velocity
- Use built-in capabilities and libraries: Python has a variety of built-in capabilities and libraries which might be extremely optimized and may prevent a variety of time and sources.
- Keep away from utilizing international variables: World variables can decelerate your code, as they are often accessed from anyplace in this system. As an alternative, use native variables each time doable.
- Use checklist comprehensions as a substitute of for loops: Listing comprehensions are quicker than for loops as a result of they’re extra concise and carry out the identical operations in fewer traces of code.
- Keep away from utilizing recursion: Recursive capabilities can decelerate your code as a result of they take up a variety of reminiscence. As an alternative, use iteration.
- Use NumPy and SciPy: NumPy and SciPy are highly effective libraries that may make it easier to optimize your code for scientific and mathematical computing.
- Use Cython to hurry up crucial components of the code. It’s a programming language that may be a superset of Python however could be compiled into C, which makes it quicker.
- Use “vectorized operations” and “broadcasting” when performing calculations, it’s going to make the code run quicker.
- Use multi-processing, multi-threading, or asyncio to make the most of a number of CPU cores and run a number of duties concurrently.
- Use a profiler and debuggers to establish bottlenecks within the code, and optimize these sections particularly.
- Hold the code easy and readable, it’s going to make it simpler to grasp, preserve and optimize.
2. Utilizing Superior Options Akin to Decorators, Turbines, and Metaclasses
- Decorators: Decorators are a approach to modify the habits of a perform or class. They’re usually used so as to add performance, equivalent to logging or memoization, with out altering the underlying code.
- Turbines: Turbines are a approach to create iterators in Python. They help you iterate over giant knowledge units with out loading all the knowledge set into reminiscence. This may be helpful for duties like studying giant recordsdata or processing giant quantities of knowledge.
- Metaclasses: Metaclasses are a approach to create lessons that can be utilized to create different lessons. They can be utilized to outline customized habits for lessons, equivalent to including strategies or properties. They can be used to create metaprogramming, which lets you write code that generates different code.
- Coroutines: Coroutines are a approach to create concurrent and asynchronous code in Python. They help you carry out a number of duties concurrently, they usually can be utilized to create easy, light-weight threads.
- Operate annotations: Operate annotations are a approach so as to add metadata to a perform, they can be utilized to supply extra details about perform arguments, return values, and kinds, they usually can be used to specify the kind of perform’s argument, and return worth.
- Context Managers: Context managers are a approach to deal with sources, equivalent to recordsdata, sockets, and database connections, in a protected and environment friendly approach. They help you outline a context during which a useful resource is used, and mechanically deal with the opening and shutting of the useful resource.
- Enumerations: Enumerations are a approach to outline a set of named values, which can be utilized as replacements for integers and strings. They’re created utilizing the Enum class.
- Namedtuples: Namedtuples is a subclass of tuples with named fields, this fashion you possibly can entry the fields by title fairly than by index. They’re created utilizing the namedtuple perform.
These superior options may help you to make your code extra expressive, readable, maintainable, and environment friendly.
3. Methods for Debugging and Error Dealing with
- Use the built-in Python debugger (pdb): The built-in Python debugger is a strong instrument that lets you step by means of your code line by line, look at variables, and set breakpoints.
- Use print statements: Including print statements to your code may help you establish the supply of the issue by offering a transparent image of this system’s execution circulate and variable values.
- Use a linter: A linter is a instrument that checks your code for syntax errors and potential bugs. It could possibly make it easier to catch errors earlier than you run your code.
- Use a unit testing framework: Unit testing lets you take a look at small items of your code individually, making it simpler to pinpoint the supply of any errors.
- Use a logging library: A logging library lets you document details about your program’s execution, such because the values of variables and the circulate of execution. This may be helpful for monitoring down errors that happen sometimes or for understanding the habits of this system over time.
- Use try-except blocks: Strive-except blocks help you deal with errors gracefully, by catching them and offering another circulate of execution. They may help you to jot down strong and fault-tolerant code.
- Use assert assertion: assert assertion lets you examine if a given situation is true and lift an exception whether it is false. They’re used to examine the integrity of the enter and can be utilized as a debugging assist.
- Use the logging module: The logging module lets you log messages with completely different severity ranges, it may be used to log debug, information, warning, error, and important messages.
- Use the traceback module: The traceback module lets you extract the stack hint of an exception, which could be helpful for understanding the reason for the error and finding the purpose of failure within the code.
- Use a bug monitoring system: A bug monitoring system lets you document, observe, and handle bugs, and hold observe of the progress of bug fixing.
4. Finest Practices For Writing Clear and Readable Code
- Use significant variable and performance names: Use clear, descriptive names for variables and capabilities that precisely mirror their objective and utilization.
- Use whitespace and indentation: Use whitespace and indentation persistently to separate code blocks and make the construction of your code clear.
- Use feedback: Use feedback to clarify the aim of your code and any non-obvious components of it.
- Hold traces brief: Restrict the size of your traces of code to round 80 characters, this makes it simpler to learn the code on completely different gadgets and screens.
- Use significant perform and variable names: Use clear, descriptive names for variables and capabilities that precisely mirror their objective and utilization.
- Use significant naming conventions: Use naming conventions which might be constant and significant, equivalent to snake_case for variable names, and CamelCase for sophistication names.
- Hold capabilities small and targeted: Hold capabilities small and targeted on a single job, this makes them extra reusable and simpler to grasp.
- Keep away from utilizing international variables: World variables could make the code tougher to grasp and preserve, keep away from them when doable.
- Use docstrings: Use docstrings to doc the aim, and arguments, and return the worth of capabilities and lessons.
- Observe the PEP 8 type information: The Python Enhancement Proposal (PEP) 8 type information offers tips for writing clear and readable Python code. It covers subjects equivalent to indentation, naming conventions, and whitespace. Following these tips will make your code extra constant and simpler to learn for others.
5. Utilizing Superior Information Buildings Akin to Units, Dictionaries, and Tuples
Python offers a number of superior knowledge constructions that can be utilized to retailer and manipulate knowledge in highly effective and environment friendly methods. These knowledge constructions embrace units, dictionaries, and tuples.
- Units: A set is an unordered assortment of distinctive parts. Units are generally used for membership testing, eradicating duplicates from an inventory, and mathematical operations equivalent to intersection and union. They’re outlined utilizing curly braces {} or the set() constructor. For instance my_set = {1, 2, 3, 4}
- Dictionaries: A dictionary is an unordered assortment of key-value pairs. Dictionaries are generally used for lookups, counting, and sorting. They’re outlined utilizing curly braces {} and their keys and values are separated by a colon. For instance my_dict = {‘geeks’: 1, ‘for’: 2, ‘geeks’: 3}
- Tuples: A tuple is an ordered assortment of parts. Tuples are just like lists however they’re immutable, that means their parts can’t be modified as soon as created. They’re outlined utilizing parentheses () or the tuple() constructor. For instance my_tuple = (1, 2, 3, 4)
These knowledge constructions can be utilized in quite a lot of methods to resolve completely different issues. For instance, you need to use units to shortly examine if a component is already current in an information set, use dictionaries to effectively retailer and retrieve knowledge, and use tuples to group a number of values collectively and use them as a single entity.
It’s vital to remember the fact that every knowledge construction has its personal strengths and weaknesses, and choosing the proper one for a particular job can enormously enhance the efficiency and readability of your code.
6. Utilizing Constructed-in Libraries For Information Evaluation and Manipulation
Python has an enormous ecosystem of built-in libraries that can be utilized for knowledge evaluation and manipulation. These libraries embrace:
- NumPy: NumPy is a library for working with giant arrays and matrices of numerical knowledge. It offers capabilities for performing mathematical operations on these arrays, equivalent to linear algebra, Fourier transforms, and statistical operations.
- Pandas: Pandas is a library for working with tabular knowledge, equivalent to knowledge in a CSV file. It offers knowledge constructions such because the DataFrame and Sequence, which permit for straightforward manipulation and evaluation of knowledge. Pandas additionally present capabilities for studying and writing knowledge from varied file codecs, equivalent to CSV, Excel, and SQL.
- Matplotlib: Matplotlib is a library for creating static, animated, and interactive visualizations. It offers capabilities for creating a variety of plots and charts, equivalent to line plots, scatter plots, histograms, and warmth maps.
- Scikit-learn: Scikit-learn is a library for machine studying. It offers a variety of algorithms for duties equivalent to classification, regression, clustering, and dimensionality discount. It additionally consists of instruments for mannequin choice, analysis, and preprocessing.
- Seaborn: Seaborn is a library constructed on high of Matplotlib that gives a high-level interface for creating lovely and informative statistical graphics. It additionally offers capabilities for visualizing complicated relationships between a number of variables.
- Scipy: Scipy is a library that gives algorithms for optimization, sign and picture processing, interpolation, integration, and extra.
These libraries are broadly used within the knowledge science group, and lots of extra libraries can be found for particular duties equivalent to pure language processing, pc imaginative and prescient, and deep studying. With these libraries, you possibly can carry out complicated knowledge evaluation and manipulation duties shortly and simply, with out having to jot down low-level code.
It’s vital to notice that mastering these libraries takes time and follow. It’s good to begin with the fundamentals, be taught the syntax and essentially the most generally used capabilities, after which transfer on to extra superior subjects. Additionally, it’s a good suggestion to learn the documentation and examples offered by the libraries, in addition to tutorials and different sources accessible on-line.
7. Ideas For Working With Massive Datasets and Reminiscence Administration
Working with giant datasets generally is a difficult job, and it requires correct reminiscence administration to keep away from working out of reminiscence and to make sure the code runs effectively. Listed here are some suggestions for working with giant datasets and managing reminiscence:
- Use memory-efficient knowledge constructions: When working with giant datasets, it’s vital to make use of memory-efficient knowledge constructions. For instance, as a substitute of utilizing Python’s built-in checklist knowledge construction, you need to use NumPy arrays that are extra reminiscence environment friendly.
- Use knowledge sampling: When working with giant datasets, it’s usually helpful to work with a smaller subset of the information first. This may be completed utilizing methods equivalent to random sampling, which may help to cut back the quantity of reminiscence required to load and course of the information.
- Use lazy loading: Lazy loading is a way for loading knowledge into reminiscence solely when it’s wanted, fairly than loading all the dataset without delay. This may help to cut back the quantity of reminiscence utilized by this system and make it extra environment friendly.
- Use iterators and mills: Iterators and mills are a approach to work with giant datasets with out loading all the dataset into reminiscence without delay. They help you course of the information one piece at a time, which may help to cut back the quantity of reminiscence utilized by this system.
- Use out-of-core and on-line studying algorithms: Out-of-core and on-line studying algorithms are designed to work with giant datasets that don’t match into reminiscence. These algorithms can course of the information in smaller chunks and could be extra reminiscence environment friendly than conventional algorithms.
- Use disk-based storage: When working with giant datasets that may’t match into reminiscence, it’s usually helpful to retailer the information on disk. In style libraries equivalent to HDF5 and Parquet help you retailer giant datasets on disk and entry it in a memory-efficient approach.
- Monitor reminiscence utilization: Repeatedly monitoring the reminiscence utilization of your program may help you establish and repair reminiscence leaks, and optimize the reminiscence utilization of your program. Python offers libraries equivalent to memory_profiler and psutil to watch reminiscence utilization.
By following the following pointers, you possibly can work with giant datasets extra effectively and successfully, whereas minimizing the chance of working out of reminiscence.
8. Methods For Creating and Utilizing Modules and Packages
Modules and packages are a approach to arrange and reuse code in Python. They can be utilized to group associated capabilities, lessons, and variables collectively, and to make them accessible to be used in different components of this system. Listed here are some methods for creating and utilizing modules and packages:
- Create modules: A module is a single Python file that accommodates Python code. To create a module, merely create a brand new Python file with a .py extension. For instance, you possibly can create a file named mymodule.py, and put your capabilities and lessons on this file.
- Use the import assertion: To make use of a module in one other Python file, you need to use the import assertion. For instance, you need to use import mymodule to make all of the capabilities and lessons in mymodule.py accessible to be used within the present file.
- Use the from … import assertion: The import assertion can be utilized to import particular capabilities or lessons from a module utilizing the from … import assertion. For instance, you need to use from mymodule import myfunction to import solely the myfunction from mymodule.py
- Create packages: A bundle is a set of modules which might be organized in a listing construction. To create a bundle, create a brand new listing and add an init.py file contained in the listing. This file could be empty or can include an initialization code for the bundle. You possibly can then add modules as subdirectories contained in the bundle listing.
- Use the import assertion for packages: To make use of a module inside a bundle, you need to use the import assertion and specify the bundle and module title separated by a dot. For instance, you need to use import mypackage.mymodule to import the mymodule module from the mypackage bundle.
- Use the from … import assertion for packages: To import particular modules from a bundle, you need to use the from … import assertion, in addition to specify the bundle title earlier than the module title. For instance, you need to use from mypackage import mymodule to import mymodule from mypackage
- Use the init.py file: When making a bundle, you need to use the init.py file to outline variables and capabilities that needs to be accessible to be used all through the bundle. This file is executed when the bundle is imported, and any variables or capabilities outlined in it will likely be accessible to be used in all modules inside the bundle.
Through the use of modules and packages, you possibly can arrange your code in a logical and reusable approach, making it extra readable and maintainable. It additionally lets you distribute your code and share it with others.
9. Utilizing Object-Oriented Programming Ideas in Python
Object-oriented programming (OOP) is a programming paradigm that’s primarily based on the idea of objects, that are situations of lessons. OOP lets you mannequin real-world ideas in your code, making it extra organized, reusable, and maintainable. Listed here are some methods for utilizing object-oriented programming ideas in Python:
- Create lessons: In Python, a category is a blueprint for creating objects. You possibly can outline a category through the use of the category key phrase after which outline the attributes and strategies for the category inside the category definition.
- Create objects: As soon as a category is outlined, you possibly can create objects (or situations) of that class. To create an object, you name the category title adopted by parentheses. For instance, my_object = MyClass() creates an object of the MyClass class.
- Use attributes: Attributes are variables that retailer knowledge inside an object. You possibly can outline attributes inside a category utilizing the self key phrase. For instance, self.title = “John” would create an attribute named title with the worth “John” for an object of the category.
- Use strategies: Strategies are capabilities which might be related to a category. You possibly can outline strategies inside a category utilizing the self key phrase. Strategies can be utilized to carry out operations on the article’s attributes.
- Use inheritance: Inheritance is a mechanism that lets you create a brand new class that inherits the attributes and strategies of an present class. The brand new class is named a subclass, and the present class is named the superclass.
- Use polymorphism: Polymorphism is a mechanism that lets you use a single perform or methodology to work with several types of objects. In Python, this may be achieved through the use of duck typing, which implies that the kind of an object is set by its habits (strategies) fairly than by its class.
- Use encapsulation: Encapsulation is a mechanism that lets you cover the implementation particulars of a category from the skin world. You possibly can obtain encapsulation in Python through the use of underscores earlier than the attribute or methodology title. For instance, _private_attribute can be non-public and shouldn’t be accessed outdoors the category.
Through the use of OOP ideas, you possibly can design extra modular, versatile, and maintainable code. It lets you outline a transparent and constant interface on your lessons, encapsulate implementation particulars, and supply a approach to arrange and reuse code.
10. Superior Methods For Working with Strings, Numbers, and Different Information Sorts
Python offers a variety of built-in capabilities and strategies for working with strings, numbers, and different knowledge sorts. Listed here are some superior methods for working with these knowledge sorts:
- String formatting: Python offers superior string formatting methods utilizing the format() methodology and f-strings. These methods help you insert dynamic values into strings and make them extra readable. For instance, you need to use “My title is {}”.format(“John”) to insert the worth “John” into the string.
- Common expressions: Python offers a module known as re that lets you work with common expressions. Common expressions are a strong instrument for looking out, matching, and manipulating strings. They can be utilized for duties equivalent to discovering patterns in textual content, validating e-mail addresses, and extra.
- String strategies: Python offers a variety of string strategies that can be utilized to govern strings. These strategies embrace however usually are not restricted to .strip() to take away whitespace from the start and finish of a string, .cut up() to separate a string into an inventory of substrings, and .substitute() to exchange a particular substring with one other string.
- Quantity formatting: Python offers superior quantity formatting methods utilizing the format() methodology and f-strings, just like what we will do with strings. These methods help you management the variety of decimal locations, the presence of a thousand separators, and different formatting choices.
- Kind casting: Python offers capabilities equivalent to int(), float(), and str() that can be utilized to transform one knowledge sort to a different. This may be helpful when working with several types of knowledge, equivalent to changing a string to an integer or a floating-point quantity to a string.
- Decimal precision: Python’s decimal module offers a Decimal class that can be utilized to carry out high-precision decimal arithmetic. This may be helpful for monetary and financial calculations the place the precision of floating-point numbers may not be enough.
- Superior mathematical operations: Python offers a math module that gives superior mathematical capabilities equivalent to trigonometric capabilities, logarithms, exponents, and extra. The module NumPy additionally offers an environment friendly implementation of those operations and others, equivalent to matrix operations and extra.
Through the use of these superior methods, you possibly can carry out complicated operations on strings, numbers, and different knowledge sorts, and make your code extra environment friendly and readable. It’s vital to notice that it’s all the time a good suggestion to check and benchmark your code to make sure that it runs effectively when working with giant knowledge units.
Conclusion
Mastering Python programming in 2023 shouldn’t be solely a useful talent however a vital one in as we speak’s tech-driven world. With the proper strategy and methods, you possibly can obtain mastery very quickly. The information outlined on this article, mixed together with your dedication and dedication, will make it easier to attain your objectives and unlock the complete potential of Python. Embrace the ability of Python and see your expertise soar to new heights within the coming yr! So, be able to unlock the key of Python and elevate your coding expertise to the subsequent stage.